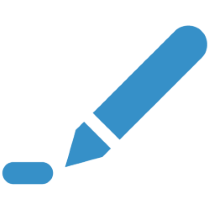
In this guide, we will show you how to add a digital signature to a PDF file in C#, including the necessary code and steps to get started. Whether you are a developer or a user, this guide will provide you with the information you need to add a digital signature to a PDF document in C#. Also, we will demonstrate how to verify a digitally signed PDF file programmatically in C#.
- C# Library to Digitally Sign PDF
- Add digital signatures in PDF documents using C#
- Digitally sign PDF documents with a Timestamp server in C#
- Verify digital signature in PDF using C#
C# Library for Digital Signatures in PDF
To add and verify digital signatures in PDF, we’ll use Aspose.PDF for .NET. It is a powerful PDF manipulation library for creating, editing, converting, and digitally signing PDF documents. Not only this, you can get a free license to sign your PDF files without any limitations.
You can download Aspose.PDF for .NET or install it using NuGet.
PM> Install-Package Aspose.PDF
How to Add Digital Signature to PDF in C#
The following are the steps to add a digital signature to a PDF in C#.
- Create an object of the Document class and initialize it with the PDF document’s path.
- Create an object of PdfFileSignature class and initialize it with the Document class’s object.
- Create an object of PKCS7 class and initialize it with a certificate path and password.
- Create and initialize the object of DocMDPSignature class for the MDP signature type.
- Create a Rectangle for signature placement.
- Digitally sign PDF document using PdfFileSignature.Certify() method.
- Save the document using PdfFileSignature.Save() method.
C# Code for Adding Digital Signature to PDF
The following code sample shows how to add a digital signature to a PDF in C#.
C# Digitally Sign PDF with Timestamp Server
You can also add a digital signature to a PDF document with the TimeStamp server by providing its details using the TimestampSettings class.
The following code sample shows how to digitally sign a PDF document with a TimeStamp server in C#.
Verify Digitally Signed PDF in C#
The following are the steps to verify the digital signature in a PDF document:
- Create an object of the Document class and initialize it with the PDF document’s path.
- Create an object of PdfFileSignature class and initialize it with the Document class’s object.
- Access all the signatures of the PDF document.
- Verify the validity of the signature using PdfFileSignature.VerifySigned() method.
The following code sample shows how to verify the digital signature in PDF using C#.
Free PDF Digital Signature Library
You can get a free temporary license and add digital signatures to PDF files without any limitations.
Conclusion
In this article, you have learned how to add digital signatures to PDF files in C#.NET. Furthermore, you have seen how to verify the digitally signed PDF files programmatically in C#. You can simply install Aspose.PDF for .NET in your applications and digitally sign your PDF files seamlessly.