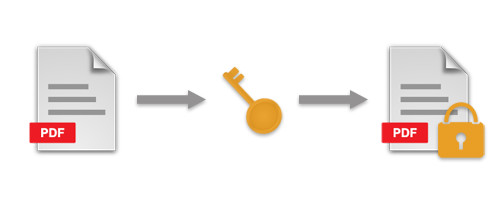
PDF encryption is used to secure the PDF document from unauthorized access. If a PDF document is encrypted, you have to decrypt it to access its content, otherwise, it will remain unreadable. Most often, the PDF documents are encrypted with a password that is required to open the document. On the other hand, you can also limit the access permissions to various operations such as printing, editing, copying, etc. Aspose’ PDF library, Aspose.PDF for .NET, provides some simple ways to encrypt and decrypt PDF files using C# and VB.NET. In this article, I’ll show you how to perform PDF encryption and decryption operations using C#.
C# PDF Encryption and Decryption API - Installation
Aspose.PDF for .NET is hosted on NuGet and can easily be installed using the NuGet Package Manager. Alternatively, you can download the API’s DLL from the Downloads section.
Encrypt a PDF Files in C#
In order to encrypt a PDF file, you need to set a password that will be required to open and view the document. In addition, you have to specify the desired cryptographic algorithm as the encryption method. Aspose.PDF for .NET supports the following encryption methods:
- RC4 with a 40-bit key.
- RC4 with a 128-bit key.
- AES with a 128-bit key.
- AES with a 256-bit key.
Steps to Encrypt a PDF File
The following are the simple steps to encrypt a PDF document using C#.
- Load the PDF document using Document class.
- Encrypt the PDF document with a password and cryptographic algorithm using Document.Encrypt method.
- Save the encrypted PDF document using Document.Save method.
The following code sample shows how to encrypt a PDF document using C#.
When you will open this encrypted PDF document in Adobe Reader, it will popup the following dialogue.
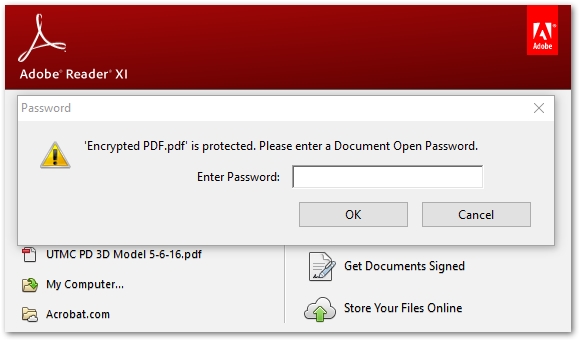
Decrypt a PDF File using C#
In order to decrypt a PDF document, you must have the user or owner password of the document. The following are the simple steps to decrypt a PDF document:
- Load PDF document using Document class by specifying the user’s or owner’s password.
- Call Document.Decrypt() method.
- Save the decrypted PDF document.
The following code sample shows how to decrypt a PDF document using C#.
Change Security Permissions of a PDF File in C#
You can enhance the security of a PDF document by limiting the user’s permission. In such a case, you can specify the operations which are allowed for the users. The following is the list of the permissions that you can set to allow for a user.
- Print Document - Allows printing the document.
- Modify Content - Allows modifying the contents of the document.
- Extract Content - Allows copying the content from the document.
- Modify Text Annotations - Allows adding or modifying text annotations.
- Fill Form - Allows filling in the interactive form fields.
- Extract Content with Disabilities - Allows extracting text and graphics (for users with disabilities).
- Assemble Document - Allows to insert, rotate, or delete pages and create bookmarks or thumbnail images.
- Printing Quality - Allows printing documents with high resolution.
Steps to Change Security Permissions of a PDF File
The following are the steps to change the security permissions of a PDF document.
- Load the PDF document.
- Set security permissions using Document.Encrypt method.
- Save the encrypted PDF document.
The following code sample shows how to change permissions of a PDF document using C#.
Another way to set or modify the privileges of PDF documents is by using DocumentPrivilege class. The DocumentPrivilege class lets you define the permissions or privileges for the users. The following code sample shows how to set the security permissions of a PDF document using DocumentPrivilege class in C#.
The following are the security details of the PDF document we have encrypted in this section.
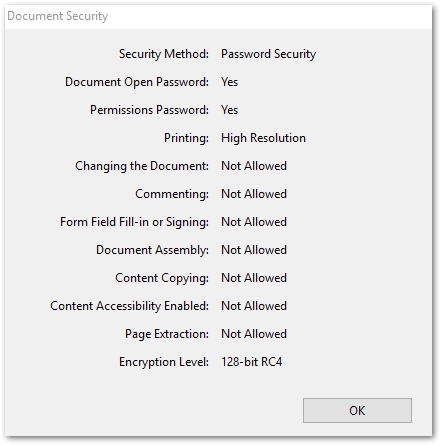
Conclusion
In this article, you have gone through the steps of how to encrypt and decrypt PDF documents using C#. Furthermore, you have also learned how to set or modify the security permissions of a PDF document in C#. These security features let you protect the sensitive PDF documents using the password and cryptographic algorithms as well as limit the user’s access to the document related operations. You may consult the documentation to learn more about our .NET PDF Library.