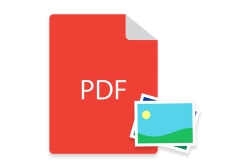
While generating PDF files programmatically, you may need to work with the images. This could be adding an image, removing/replacing an image, or extracting images from the PDF in C#. This article covers all these features and lets you implement them in easy-to-follow steps and a few lines of code. So let’s see how to work with images in PDF files in C#.
- C# Library to Work with Images in PDF
- Add an Image in a PDF in C#
- Extract Images from a PDF in C#
- C# Remove Images from a PDF
- Replace an Image in a PDF in C#
C# Library to Add and Manipulate Images in PDF
To add, remove, and replace images in PDF, we will use Aspose.PDF for .NET. It is a C# class library that lets you create and manipulate PDF documents from within the .NET applications. Using the library, you can perform basic as well as advanced PDF automation features quite easily.
The library can be downloaded as DLL or installed via NuGet.
PM> Install-Package Aspose.PDF
Add Image in a PDF using C#
The following are the steps to add an image to a PDF file in C#.
- Use Document class to create a new or load an existing PDF file.
- Get the reference of the desired page in Page object.
- Add the image to Resources collection of the page.
- Use the following operators to place the image on the page:
- GSave operator to save the current graphical state.
- ConcatenateMatrix operator to specify where the image is to be placed.
- Do operator to draw the image on the page.
- GRestore operator to save the updated graphical state.
- Save the updated PDF file using Document.Save(String) method.
The following code sample shows how to add an image to a PDF file using C#.
Extract Images from PDF in C#
In case you want to extract all the images from a PDF file, you can do it by following the below steps.
- Use Document class to load an existing PDF file.
- Get the desired image in the XImage object from a particular page’s Resources collection using the index.
- Save the extracted image in the desired format using XImage.Save(FileStream, ImageFormat) method.
The following code sample shows how to extract images from PDF in C#.
Remove Images from PDF in C#
Once you have got access to the resources of a page in PDF, you can remove the images from it. The following are the steps to remove images from a PDF file in C#.
- Load the PDF file using Document class.
- Remove image(s) using one of the following methods.
- Delete() - Delete all images.
- Delete(Int32) - Delete image by index.
- Delete(String) - Delete image by name.
- Save the updated PDF file using Document.Save(String) method.
The following code sample shows how to remove images from a PDF using C#.
Replace Image in PDF in C#
Aspose.PDF for .NET also lets you replace a particular image in the PDF. For this, you can replace the image in the image collection of the page. The following are the steps to replace an image in PDF using C#.
- Load the PDF file using Document class.
- Replace the desired image using [Document.Pages1.Resources.Images.Replace(Int32, Stream, Int32, Boolean)]24 method.
- Save the updated PDF file using Document.Save(String) method.
The following code sample shows how to replace an image in PDF using C#.
Free C# Library to Manipulate PDF Images
You can get a free temporary license and work with images in PDF files without any limitations.
Explore C# PDF Library
You can explore more about the C# PDF library using the documentation.
Conclusion
Images and graphical objects are important elements of PDF documents. Therefore, in this article, we have covered how to manipulate images in a PDF using C#. The step-by-step tutorial and code samples have shown how to add, extract, remove and replace images in PDF files in C#.