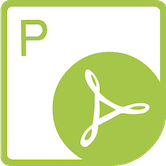
In this article, we will be converting XML files to PDF in C#. Aspose.PDF for .NET library extensively supports the XML conversion feature because of the inclusion of many related enhancements, as requested by the users. You can elevate your .NET applications with this efficient feature of exporting XML to PDF. Also, the good thing is, you can perform free XML to PDF conversion.
Let us consider the following use cases related to XML to PDF conversion:
C# PDF Library
First, install the XML to PDF converter library using the following NPM command.
PM> Install-Package Aspose.PDF
How to Convert XML to PDF in C#
For converting an XML to PDF, you need to follow the XML Schema of Aspose.PDF for .NET API which is available as XSD file. Following is an XML file that we will be converting to PDF as a Hello World demonstration.
<?xml version="1.0" encoding="utf-8" ?>
<Document xmlns="Aspose.Pdf">
<Page id="mainPage">
<TextFragment>
<TextSegment>Hello</TextSegment>
</TextFragment>
<TextFragment>
<TextSegment>World!</TextSegment>
</TextFragment>
</Page>
</Document>
You need to follow the steps below to convert XML data to PDF:
The following code snippet shows how to convert XML to PDF in C#:
C# XML to PDF using HTML
Sometimes you might need to use HTML in XML before conversion to HTML. Aspose.PDF for .NET API supports this feature as well. However, HTML and XML tags are quite similar. Therefore, you need to specify the CDATA tag so that the HTML is not parsed as XML markup. Below sample XML file includes HTML denoted by CDATA to avoid any anomaly:
<?xml version="1.0" encoding="utf-8" ?>
<Document xmlns="Aspose.Pdf">
<Page id="mainSection">
<HtmlFragment>
<![CDATA[
<font style="font-family:Tahoma; font-size:40px;">This is Html String.</font>
]]>
</HtmlFragment>
</Page>
</Document>
You can convert this XML file to PDF with the following steps:
- Instantiate Document class object
- Load the input XML file
- Save the output PDF file
Below code snippet shows how to convert an XML file, containing HTML, to PDF in C#:
Export XML and XSLT to PDF in C#
Sometimes you may have an existing XML file that contains important application data and you want to generate PDF reports using that XML file. In such scenarios, you can create XSLT file to transform your existing XML document to Aspose.PDF’s compatible XML document. Then you can proceed to convert XML to PDF. Let us learn this with a simple and basic examples:
<?xml version="1.0" encoding="utf-8" ?>
<Contents>
<Content>Hello World!</Content>
</Contents>
<?xml version="1.0" encoding="utf-8" ?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="text()"/>
<xsl:template match="/Contents">
<html>
<Document xmlns="Aspose.Pdf" IsAutoHyphenated="false">
<PageInfo>
<DefaultTextState
Font = "Helvetica" FontSize="8" LineSpacing="4"/>
<Margin Left="5cm" Right="5cm" Top="3cm" Bottom="15cm" />
</PageInfo>
<Page id="mainSection">
<TextFragment>
<TextSegment>
<xsl:value-of select="Content"/>
</TextSegment>
</TextFragment>
</Page>
</Document>
</html>
</xsl:template>
</xsl:stylesheet>
You can notice that the XML file does not follow the XML schema of Aspose.PDF for .NET API. However, the XSLT file transforms it to the required compatibility. Now you can follow the steps below to convert such XML using XSLT to PDF:
The code snippet below is based on these steps which show how to convert XML to PDF in C#:
Free C# XML to PDF Converter
You can get a free temporary license and convert XML data to PDF without any limitations.
Conclusion
We have learned how to convert XML to PDF file in C# provided the XML follows the mentioned schema of the API. Considering the popularity and usability of HTML tags, we have also considered the scenario when you need to include some HTML in the source XML file. Moreover, we have also explored how to convert XML and XSLT to PDF when the existing XML file does not follow the schema.