
Szukasz programu PowerPoint Viewer do przeglądania lub osadzania prezentacji w aplikacji internetowej ASP.NET Core? Jeśli tak, czytaj dalej ten artykuł i dowiedz się, jak utworzyć prostą przeglądarkę programu ASP.NET Core PowerPoint Viewer i wyświetlać prezentacje PPT/PPTX przy użyciu języka C#. Więc zacznijmy.
Funkcje przeglądarki ASP.NET PowerPoint Viewer
Nasza przeglądarka programu ASP.NET PowerPoint Viewer użyje interfejsu API Aspose.Slides for .NET do renderowania slajdów prezentacji jako obrazów PNG. Po wyrenderowaniu slajdów wyświetlimy je za pomocą karuzeli Bootstrap. Następujące funkcje będą dostępne w aplikacji:
- Przeglądaj i przeglądaj prezentacje programu PowerPoint (PPT/PPTX).
- Ustaw domyślny plik PowerPoint, który ma być wyświetlany podczas ładowania strony.
- Suwak do poruszania się między slajdami.
Kroki, aby utworzyć przeglądarkę programu PowerPoint w ASP.NET Core
Poniżej przedstawiono kilka prostych kroków, aby utworzyć przeglądarkę programu PowerPoint w ASP.NET Core.
- Utwórz nową aplikację internetową ASP.NET Core w programie Visual Studio.
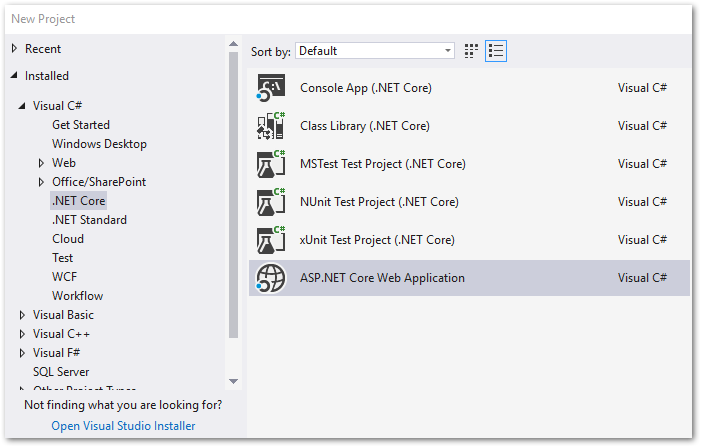
- Otwórz Menedżera pakietów NuGet i zainstaluj pakiet Aspose.Slides for .NET.

- Utwórz foldery Prezentacje i Slajdy w katalogu głównym www, aby przechowywać odpowiednio pliki programu PowerPoint i wyrenderowane slajdy.
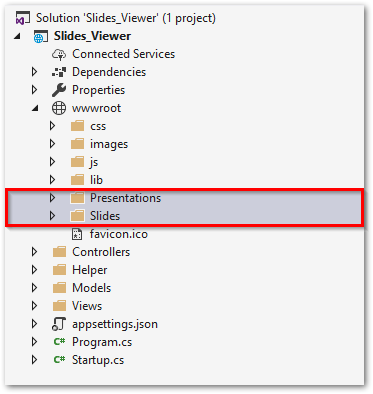
Utwórz nowy folder o nazwie „Pomocnicy” w folderze głównym.
Utwórz nową klasę o nazwie „Slajd” w folderze „Pomocnicy”, aby przechowywać informacje o slajdach prezentacji.
public class Slide
{
public int SlideNumber { get; set; }
public string Path { get; set; }
}
- Otwórz plik „HomeController.cs” i zastąp jego kod następującym (Zaktualizuj nazwę domyślnego pliku programu PowerPoint w akcji Indeks).
public class HomeController : Controller
{
public List<Helper.Slide> slides;
public IHostingEnvironment _env;
public HomeController(IHostingEnvironment env)
{
_env = env;
}
[HttpGet]
public ActionResult Index(string fileName)
{
slides = new List<Helper.Slide>();
if (fileName == null)
{
// Wyświetl domyślny plik programu PowerPoint podczas ładowania strony
slides = RenderPresentationAsImage("presentation.pptx");
}
else
{
slides = RenderPresentationAsImage(fileName);
}
return View(slides);
}
public List<Helper.Slide> RenderPresentationAsImage(string FileName)
{
var webRoot = _env.WebRootPath;
// Załaduj prezentację PowerPoint
Presentation presentation = new Presentation(Path.Combine(webRoot, "Presentations", FileName));
var slides = new List<Helper.Slide>();
string imagePath = "";
// Zapisz i przeglądaj slajdy prezentacji
for (int j = 0; j < presentation.Slides.Count; j++)
{
ISlide sld = presentation.Slides[j];
Bitmap bmp = sld.GetThumbnail(1f, 1f);
imagePath = Path.Combine(webRoot, "Slides", string.Format("slide_{0}.png", j));
bmp.Save(imagePath, System.Drawing.Imaging.ImageFormat.Png);
// Dodaj do listy
slides.Add(new Helper.Slide { SlideNumber = j, Path = string.Format("slide_{0}.png", j) });
}
return slides;
}
}
- Otwórz Views/Home/index.cshtml i zastąp jego zawartość następującą.
@{
ViewData["Title"] = "PowerPoint Viewer";
}
@{
Layout = null;
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Slides Viewer</title>
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.css" />
<link rel="stylesheet" href="~/css/site.css" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
</head>
<body>
<div class="container">
<nav class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="sr-only">Toggle navigation</span>
</button>
<a asp-area="" asp-controller="Home" asp-action="Index" class="navbar-brand">PowerPoint Viewer</a>
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li style="cursor: pointer;"><a data-toggle="modal" data-target="#exampleModal">Browse</a></li>
</ul>
</div>
</div>
</nav>
<br />
<div id="myCarousel" class="carousel slide" data-interval="false">
<div class="carousel-inner" role="listbox" style="width:100%; height: auto !important;">
@for (int i = 0; i < Model.Count; i++)
{
if (i == 0)
{
<div class="item active">
<img src="~/Slides/@Model[i].Path" style="width: 80%; height: auto; margin:auto !important" class="img-responsive " />
<p style="text-align:center;">
<strong> @(Model[i].SlideNumber + 1)/@Model.Count</strong>
</p>
</div>
}
else
{
<div class="item">
<img src="~/Slides/@Model[i].Path" style="width: 80%; height: auto; margin:auto !important" class="img-responsive " />
<p style="text-align:center;">
<strong> @(Model[i].SlideNumber + 1)/@Model.Count</strong>
</p>
</div>
}
}
</div>
<a class="left carousel-control" href="#myCarousel" role="button" data-slide="prev">
<span class="glyphicon glyphicon-chevron-left" aria-hidden="true"></span>
<span class="sr-only">Previous</span>
</a>
<a class="right carousel-control" href="#myCarousel" role="button" data-slide="next">
<span class="glyphicon glyphicon-chevron-right" aria-hidden="true"></span>
<span class="sr-only">Next</span>
</a>
</div>
</div>
<!-- Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Select a file</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<div class="list-group">
@foreach (string file in System.IO.Directory.EnumerateFiles("wwwroot/Presentations","*"))
{
string fileName = System.IO.Path.GetFileName(file);
@Html.ActionLink(fileName, "Index", "Home", new { fileName = fileName }, new { @class = "list-group-item" })
}
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
<script src="~/lib/jquery/dist/jquery.js"></script>
<script src="~/lib/bootstrap/dist/js/bootstrap.js"></script>
<script src="~/js/site.min.js" asp-append-version="true"></script>
</body>
</html>
- Zbuduj aplikację i uruchom ją w swojej ulubionej przeglądarce.

Zobacz też
Uzyskaj tymczasową licencję na Aspose.Slides for .NET
Możesz uzyskać tymczasową licencję Aspose.Slides for .NET, aby uniknąć ograniczeń próbnych.
Wskazówka: możesz sprawdzić implementację na żywo operacji przeglądania prezentacji.