
Adobe’s popular Photoshop Application uses PSD (Photoshop Document) as a native image file format. It is commonly used to create logos, brochures, and other images where the PSD file contains several layers. We can easily protect the design by adding the text or image watermark to a PSD file programmatically. In this article, we will learn how to add a watermark to a PSD in C#.
This article shall cover the following topics:
- C# Photoshop API to Add Watermark to PSD
- Add a Text Watermark to PSD
- Create a Diagonal Watermark in PSD
- Add an Image Watermark to PSD
C# Photoshop API to Add Watermark to PSD
To add a text or image watermark in a PSD file, we will be using the Aspose.PSD for .NET API. It is an easy-to-use Adobe Photoshop file format manipulation API. It allows the loading and reading of PSD and PSB files in .NET applications. The API enables us to update layer properties, add watermarks, perform compression, rotation, scale or render PSD and several other supported file formats without needing to install Adobe Photoshop.
The PsdImage class of the API allows loading, editing, and saving PSD files. The Graphics class represents the graphics in the image. We have the DrawString(string, Font, Brush, RectangleF, StringFormat) method of this class that draws the specified text string in the specified rectangle with the specified brush and font objects. The Layer class represents the PSD layer. The DrawImage(Point, RasterImage) method of this class draws the image on the layer. We can save the PSD to the specified file location using the Save(string, ImageOptionsBase) method.
Please either download the DLL of the API or install it using NuGet.
PM> Install-Package Aspose.PSD
Add Text Watermark to PSD using C#
We can add any text as a watermark to a PSD file by following the steps given below:
- Firstly, load the PSD file as PsdImage using the Image class.
- Next, create an instance of the Graphics class.
- Then, define a Font class object to draw the watermark width.
- Next, create an instance of the SolidBrush class with color.
- Then, specify string alignment.
- After that, call the DrawString() method.
- Finally, save the output file using the Save() method.
The following code sample shows how to add a text watermark to a PSD file in C#.
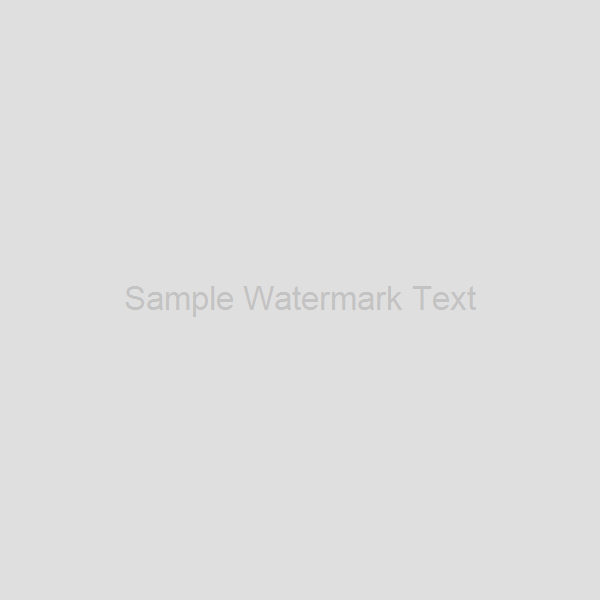
Add Text Watermark to PSD using C#
We can also save the output as a PSD file using the following code sample:
psdImage.Save(@"C:\Files\AddWatermark_output.psd", new PsdOptions());
Create Diagonal Watermark in PSD using C#
We can add a diagonal text watermark to a PSD file by following the steps given below:
- Firstly, load the PSD file as PsdImage using the Image class.
- Next, create an instance of the Graphics class.
- Then, define a Font class object to draw the watermark width.
- Meanwhile, create an instance of the SolidBrush class with color.
- Then, specify the transform matrix to rotate the watermark.
- Later, specify string alignment.
- After that, call the DrawString() method.
- Finally, save the output file using the Save() method.
The following code sample shows how to add a diagonal text watermark to a PSD file in C#.

Create Diagonal Watermark in PSD using C#
Add Image Watermark to PSD using C#
We can also add an image as a watermark to a PSD file by following the steps given below:
- Firstly, load the PSD file as PsdImage using the Image class.
- Next, create an instance of the Layer class.
- Then, set the layer’s height, width and Opacity.
- Next, call the AddLayer() method to add the layer to PSD.
- After that, load a watermark image into a layer.
- Then, add an image watermark to the layer.
- After that, call the DrawImage() method. It takes the location and watermark image layer as arguments.
- Finally, save the output file using the Save() method.
The following code sample shows how to add an image watermark to a PSD file in C#.
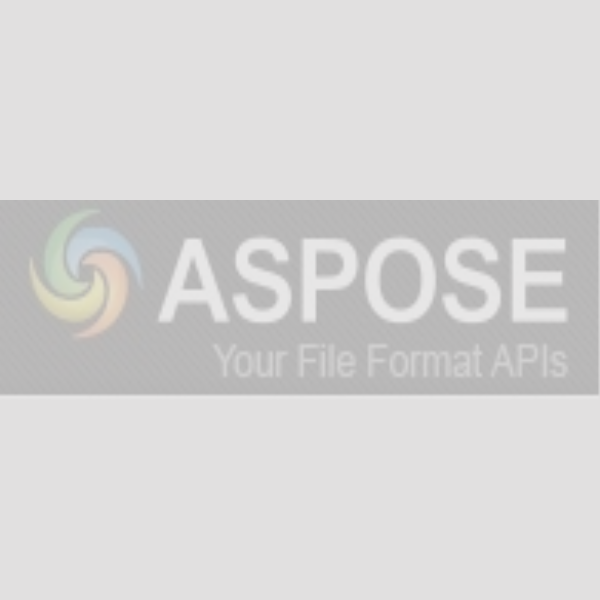
Add Image Watermark to PSD using C#
Get Free Temporary License
You can get a free temporary license to try Aspose.PSD for .NET without evaluation limitations.
Conclusion
In this article, we have learned how to:
- add a new layer to a PSD image;
- add watermark text or image to the PSD;
- save PSD as a PNG or PSD image using C#.
Besides adding a watermark to PSD in C#, you can learn more about Aspose.PSD for .NET using documentation and explore different features supported by the API. In case of any ambiguity, please feel free to contact us on our free support forum.