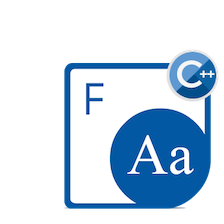
Caracteres são símbolos que explicam os significados de palavras diferentes. Você pode renderizar diferentes caracteres de texto com fontes TrueType usando C++ com a API Aspose.Font for C++. Ele suporta diferentes recursos de renderização de texto, incluindo TrueType, Type1, OTF, EOT e outras fontes. Vamos explorar como usar os recursos de renderização de texto com algumas chamadas de API simples. Estaremos abordando o tema sob os seguintes títulos:
- API de renderização de fonte C++ TrueType – Instalação
- Implementar interface para desenhar glifo para renderizar fontes TrueType usando C++
- Renderizar texto com fonte TrueType usando C++
API de renderização de fonte C++ TrueType – Instalação
Considerando a popularidade e a demanda da plataforma C++, introduzimos recursos muito úteis na API Aspose.Font for C++. Para renderizar fontes TrueType em seus aplicativos, você precisa configurar a API baixando-a em New Releases, ou pode instalá-la via NuGet usando o seguinte comando:
Install-Package Aspose.Font.Cpp
Implementar interface para desenhar glifo para renderizar fontes TrueType usando C++
Em primeiro lugar, você precisa implementar IGlyphOutlinePainter no namespace Aspose.Font.Rendering. Siga os passos abaixo para a implementação de métodos e classes:
- Crie uma classe chamada GlyphOutlinePainter
- Use System.Drawing.Drawing2D.GraphicsPath para desenhar gráficos
Abaixo está o trecho de código C++ para a implementação da interface para desenhar glifos:
For complete examples and data files, please go to https://github.com/aspose-font/Aspose.Font-for-C
RenderingText::GlyphOutlinePainter::GlyphOutlinePainter(System::SharedPtr<System::Drawing::Drawing2D::GraphicsPath> path)
{
_path = path;
}
void RenderingText::GlyphOutlinePainter::MoveTo(System::SharedPtr<Aspose::Font::RenderingPath::MoveTo> moveTo)
{
_path->CloseFigure();
_currentPoint.set_X((float)moveTo->get_X());
_currentPoint.set_Y((float)moveTo->get_Y());
}
void RenderingText::GlyphOutlinePainter::LineTo(System::SharedPtr<Aspose::Font::RenderingPath::LineTo> lineTo)
{
float x = (float)lineTo->get_X();
float y = (float)lineTo->get_Y();
_path->AddLine(_currentPoint.get_X(), _currentPoint.get_Y(), x, y);
_currentPoint.set_X(x);
_currentPoint.set_Y(y);
}
void RenderingText::GlyphOutlinePainter::CurveTo(System::SharedPtr<Aspose::Font::RenderingPath::CurveTo> curveTo)
{
float x3 = (float)curveTo->get_X3();
float y3 = (float)curveTo->get_Y3();
_path->AddBezier(_currentPoint.get_X(), _currentPoint.get_Y(), (float)curveTo->get_X1(), (float)curveTo->get_Y1(), (float)curveTo->get_X2(), (float)curveTo->get_Y2(), x3, y3);
_currentPoint.set_X(x3);
_currentPoint.set_Y(y3);
}
void RenderingText::GlyphOutlinePainter::ClosePath()
{
_path->CloseFigure();
}
System::Object::shared_members_type Aspose::Font::Examples::WorkingWithTrueTypeAndOpenTypeFonts::RenderingText::GlyphOutlinePainter::GetSharedMembers()
{
auto result = System::Object::GetSharedMembers();
result.Add("Aspose::Font::Examples::WorkingWithTrueTypeAndOpenTypeFonts::RenderingText::GlyphOutlinePainter::_path", this->_path);
result.Add("Aspose::Font::Examples::WorkingWithTrueTypeAndOpenTypeFonts::RenderingText::GlyphOutlinePainter::_currentPoint", this->_currentPoint);
return result;
}
Em segundo lugar, crie um método com o nome DrawText com os seguintes passos:
- Percorrer símbolos na string de texto
- Obter GID como glifo identificado para cada símbolo
- Crie GlyphOutlinePainter e passe para o objeto GlyphOutlineRenderer
- Especifique as coordenadas Glyph com o objeto Matrix
Além disso, após seguir todas essas etapas, crie um método utilitário para cálculo das dimensões da fonte para a imagem conforme elaborado no seguinte trecho de código C++:
For complete examples and data files, please go to https://github.com/aspose-font/Aspose.Font-for-C
double RenderingText::FontWidthToImageWith(double width, int32_t fontSourceResulution, double fontSize, double dpi /* = 300*/)
{
double resolutionCorrection = dpi / 72;
// 72 é o dpi interno da fonte
return (width / fontSourceResulution) * fontSize * resolutionCorrection;
}
Renderizar texto com fonte TrueType usando C++
Implementamos a funcionalidade necessária para a renderização de texto com fontes TrueType. Agora vamos chamar os métodos usando Aspose.Font for C++ API como o trecho de código abaixo:
For complete examples and data files, please go to https://github.com/aspose-font/Aspose.Font-for-C
System::String dataDir = RunExamples::GetDataDir_Data();
System::String fileName1 = dataDir + u"Montserrat-Bold.ttf";
//Nome do arquivo de fonte com caminho completo
System::SharedPtr<FontDefinition> fd1 = System::MakeObject<FontDefinition>(Aspose::Font::FontType::TTF, System::MakeObject<FontFileDefinition>(u"ttf", System::MakeObject<FileSystemStreamSource>(fileName1)));
System::SharedPtr<TtfFont> ttfFont1 = System::DynamicCast_noexcept<Aspose::Font::Ttf::TtfFont>(Aspose::Font::Font::Open(fd1));
System::String fileName2 = dataDir + u"Lora-Bold.ttf";
//Nome do arquivo de fonte com caminho completo
System::SharedPtr<FontDefinition> fd2 = System::MakeObject<FontDefinition>(Aspose::Font::FontType::TTF, System::MakeObject<FontFileDefinition>(u"ttf", System::MakeObject<FileSystemStreamSource>(fileName2)));
System::SharedPtr<TtfFont> ttfFont2 = System::DynamicCast_noexcept<Aspose::Font::Ttf::TtfFont>(Aspose::Font::Font::Open(fd2));
DrawText(u"Hello world", ttfFont1, 14, System::Drawing::Brushes::get_White(), System::Drawing::Brushes::get_Black(), dataDir + u"hello1_montserrat_out.jpg");
DrawText(u"Hello world", ttfFont2, 14, System::Drawing::Brushes::get_Yellow(), System::Drawing::Brushes::get_Red(), dataDir + u"hello2_lora_out.jpg");
Conclusão
Neste artigo, você aprendeu como renderizar texto usando fontes TrueType com C++. Interessado em saber mais sobre os recursos oferecidos pelo Aspose.Font for C++ API? Você pode obter mais detalhes explorando referências de API ou Documentação do produto. No entanto, se você enfrentar qualquer ambiguidade ou precisar de ajuda, sinta-se à vontade para entrar em contato conosco por meio de Fóruns de suporte gratuito. Estamos ansiosos para interagir com você!