
Converter MP4 para MP3
Se você está procurando uma ferramenta de alta qualidade para converter MP4 para MP3, use nosso conversor online de MP4 para MP3. Com uma interface amigável, nosso conversor MP4 online permite extrair o áudio dos arquivos de vídeo em algumas etapas.
Converta seus arquivos MP4 para MP3 com este Free MP4 to MP3 Converter sem criar uma conta. Livre-se dos conversores de MP4 instaláveis.
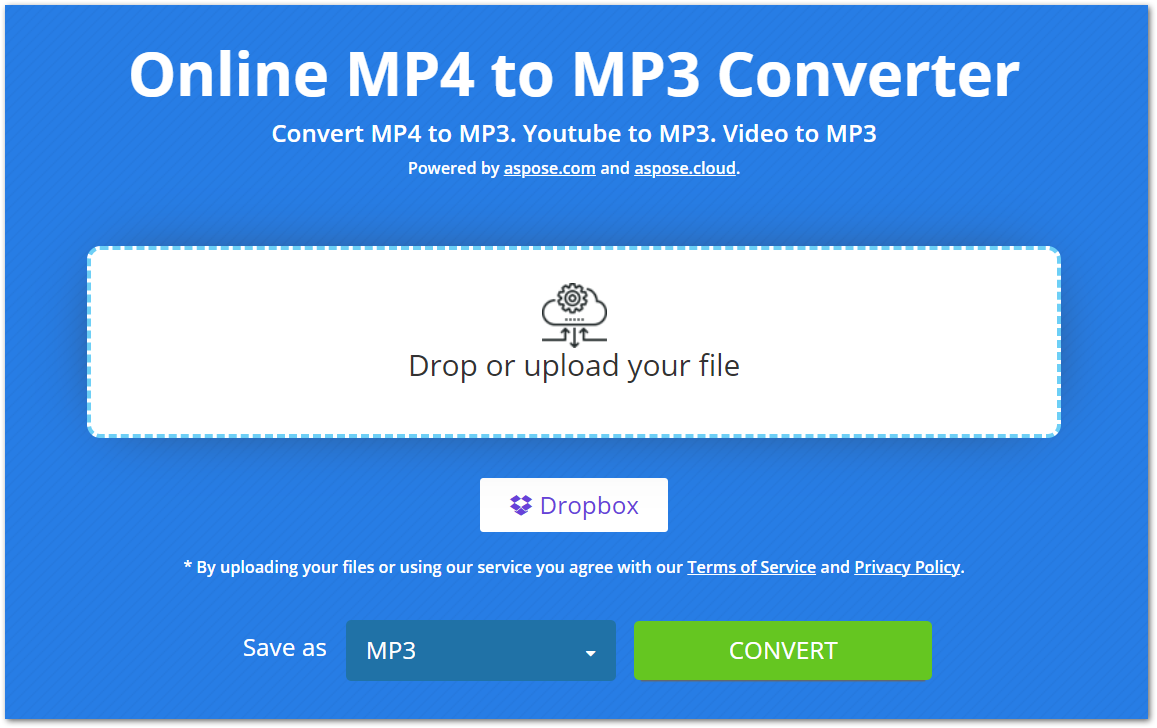
Usando o Conversor Online de MP4 para MP3
Com o nosso conversor online, a conversão de MP4 para MP3 tornou-se fácil. Você precisa executar apenas algumas etapas para extrair o áudio de um arquivo MP4, conforme mencionado abaixo.
- Carregue seu arquivo MP4 do seu computador ou Dropbox.
- Uma vez carregado, pressione o botão “CONVERT” para iniciar a conversão.
- Após a conversão, o arquivo MP3 estará disponível para download.
Observe que mantemos seus arquivos MP4/MP3 carregados e convertidos em segurança e os excluímos de nossos servidores após 24 horas.
Por que converter MP4 para MP3 online?
Existem várias razões pelas quais este conversor online de MP4 para MP3 é útil, incluindo:
Fácil de acessar: por ser um conversor baseado na web, você pode acessá-lo de qualquer dispositivo com conexão à internet.
Grátis: É totalmente gratuito converter seus arquivos MP4 para MP3 usando este conversor. Além disso, não há limite para o número de conversões que você pode realizar.
Sem inscrição: Não pedimos que você crie uma conta antes de usar este conversor de MP4 online. Basta abri-lo em seu navegador e começar a converter os arquivos.
Fácil de usar: A interface deste conversor é amigável para que você possa converter seus arquivos sem esforço.
Guia do desenvolvedor
Este conversor online é baseado em nossa API, Aspose.Slides, que está disponível para .NET, Java, C++, Python, PHP e outras plataformas. Abaixo está a demonstração de como você pode usar esta API e converter uma apresentação do PowerPoint para um formato de vídeo.
C
- Instalar Aspose.Slides para .NET in your application.
- Baixe e instale o pacote ffmpeg.
- Use o código abaixo para criar um vídeo de PPT:
using System.Collections.Generic;
using Aspose.Slides;
using FFMpegCore; // Will use FFmpeg binaries we extracted to "c:\tools\ffmpeg" before
using Aspose.Slides.Animation;
using (Presentation presentation = new Presentation())
{
// Adds a smile shape and then animates it
IAutoShape smile = presentation.Slides[0].Shapes.AddAutoShape(ShapeType.SmileyFace, 110, 20, 500, 500);
IEffect effectIn = presentation.Slides[0].Timeline.MainSequence.AddEffect(smile, EffectType.Fly, EffectSubtype.TopLeft, EffectTriggerType.AfterPrevious);
IEffect effectOut = presentation.Slides[0].Timeline.MainSequence.AddEffect(smile, EffectType.Fly, EffectSubtype.BottomRight, EffectTriggerType.AfterPrevious);
effectIn.Timing.Duration = 2f;
effectOut.PresetClassType = EffectPresetClassType.Exit;
const int Fps = 33;
List<string> frames = new List<string>();
using (var animationsGenerator = new PresentationAnimationsGenerator(presentation))
using (var player = new PresentationPlayer(animationsGenerator, Fps))
{
player.FrameTick += (sender, args) =>
{
string frame = $"frame_{(sender.FrameIndex):D4}.png";
args.GetFrame().Save(frame);
frames.Add(frame);
};
animationsGenerator.Run(presentation.Slides);
}
// Configure ffmpeg binaries folder. See this page: https://github.com/rosenbjerg/FFMpegCore#installation
GlobalFFOptions.Configure(new FFOptions { BinaryFolder = @"c:\tools\ffmpeg\bin", });
// Converts frames to webm video
FFMpeg.JoinImageSequence("smile.webm", Fps, frames.Select(frame => ImageInfo.FromPath(frame)).ToArray());
}
Java
- Instalar Aspose.Slides para Java in your application.
- Adicione a seguinte dependência.
<dependency>
<groupId>net.bramp.ffmpeg</groupId>
<artifactId>ffmpeg</artifactId>
<version>0.7.0</version>
</dependency>
- Copie e cole o código abaixo para criar um vídeo de PPT:
Presentation presentation = new Presentation();
try {
// Adds a smile shape and then animates it
IAutoShape smile = presentation.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.SmileyFace, 110, 20, 500, 500);
ISequence mainSequence = presentation.getSlides().get_Item(0).getTimeline().getMainSequence();
IEffect effectIn = mainSequence.addEffect(smile, EffectType.Fly, EffectSubtype.TopLeft, EffectTriggerType.AfterPrevious);
IEffect effectOut = mainSequence.addEffect(smile, EffectType.Fly, EffectSubtype.BottomRight, EffectTriggerType.AfterPrevious);
effectIn.getTiming().setDuration(2f);
effectOut.setPresetClassType(EffectPresetClassType.Exit);
final int fps = 33;
ArrayList<String> frames = new ArrayList<String>();
PresentationAnimationsGenerator animationsGenerator = new PresentationAnimationsGenerator(presentation);
try
{
PresentationPlayer player = new PresentationPlayer(animationsGenerator, fps);
try {
player.setFrameTick((sender, arguments) ->
{
try {
String frame = String.format("frame_%04d.png", sender.getFrameIndex());
ImageIO.write(arguments.getFrame(), "PNG", new java.io.File(frame));
frames.add(frame);
} catch (IOException e) {
throw new RuntimeException(e);
}
});
animationsGenerator.run(presentation.getSlides());
} finally {
if (player != null) player.dispose();
}
} finally {
if (animationsGenerator != null) animationsGenerator.dispose();
}
// Configure ffmpeg binaries folder. See this page: https://github.com/rosenbjerg/FFMpegCore#installation
FFmpeg ffmpeg = new FFmpeg("path/to/ffmpeg");
FFprobe ffprobe = new FFprobe("path/to/ffprobe");
FFmpegBuilder builder = new FFmpegBuilder()
.addExtraArgs("-start_number", "1")
.setInput("frame_%04d.png")
.addOutput("output.avi")
.setVideoFrameRate(FFmpeg.FPS_24)
.setFormat("avi")
.done();
FFmpegExecutor executor = new FFmpegExecutor(ffmpeg, ffprobe);
executor.createJob(builder).run();
} catch (IOException e) {
e.printStackTrace();
}
C++
- Instalar Aspose.Slides para C++ in your application.
- Baixe e instale o pacote ffmpeg.
- Use o código abaixo para criar um vídeo de PPT:
void OnFrameTick(System::SharedPtr<PresentationPlayer> sender, System::SharedPtr<FrameTickEventArgs> args)
{
System::String fileName = System::String::Format(u"frame_{0}.png", sender->get_FrameIndex());
args->GetFrame()->Save(fileName);
}
void Run()
{
auto presentation = System::MakeObject<Presentation>();
auto slide = presentation->get_Slide(0);
// Adds a smile shape and then animates it
System::SharedPtr<IAutoShape> smile = slide->get_Shapes()->AddAutoShape(ShapeType::SmileyFace, 110.0f, 20.0f, 500.0f, 500.0f);
auto sequence = slide->get_Timeline()->get_MainSequence();
System::SharedPtr<IEffect> effectIn = sequence->AddEffect(smile, EffectType::Fly, EffectSubtype::TopLeft, EffectTriggerType::AfterPrevious);
System::SharedPtr<IEffect> effectOut = sequence->AddEffect(smile, EffectType::Fly, EffectSubtype::BottomRight, EffectTriggerType::AfterPrevious);
effectIn->get_Timing()->set_Duration(2.0f);
effectOut->set_PresetClassType(EffectPresetClassType::Exit);
const int32_t fps = 33;
auto animationsGenerator = System::MakeObject<PresentationAnimationsGenerator>(presentation);
auto player = System::MakeObject<PresentationPlayer>(animationsGenerator, fps);
player->FrameTick += OnFrameTick;
animationsGenerator->Run(presentation->get_Slides());
const System::String ffmpegParameters = System::String::Format(
u"-loglevel {0} -framerate {1} -i {2} -y -c:v {3} -pix_fmt {4} {5}",
u"warning", m_fps, "frame_%d.png", u"libx264", u"yuv420p", "video.mp4");
auto ffmpegProcess = System::Diagnostics::Process::Start(u"ffmpeg", ffmpegParameters);
ffmpegProcess->WaitForExit();
}
Explorar bibliotecas do PowerPoint
Se você quiser explorar mais sobre nossas bibliotecas de manipulação do PowerPoint, abaixo estão alguns recursos úteis.
perguntas frequentes
Como converter MP4 para MP3?
Para converter um arquivo MP4 para MP3, basta carregar o arquivo e iniciar a conversão pressionando o botão CONVERT. Depois de convertido, o arquivo MP3 estará pronto para download.
É seguro carregar meus arquivos MP4 aqui?
Sim, mantemos seus arquivos seguros e os excluímos de nossos servidores após 24 horas.
Este conversor converte MP4 para MP3 com alta qualidade?
Absolutamente! O conversor garante a conversão de alta qualidade dos arquivos MP4 para MP3.
Conclusão
Neste artigo, você aprendeu como converter arquivos MP4 para MP3 usando nosso conversor online de MP4 para MP3. Assim, você pode converter seus arquivos MP4 a qualquer hora e em qualquer lugar. Além disso, fornecemos nossas bibliotecas independentes que você pode usar para manipular vídeos em apresentações do PowerPoint.