В этой статье вы узнаете, как программно экспортировать данные Excel в Google Таблицы на Python.
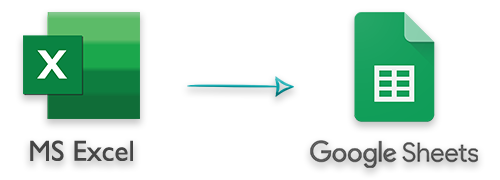
Файлы Excel широко используются для хранения данных и выполнения с ними различных типов операций, таких как создание диаграмм, применение формул. С другой стороны, Google Sheets – это популярное онлайн-приложение для создания электронных таблиц и управления ими. Google Sheets также обеспечивает обмен электронными таблицами в режиме реального времени с несколькими людьми. В некоторых случаях вам может потребоваться программно экспортировать файлы Excel XLS или XLSX в Google Таблицы. Для этого в этой статье представлено полное руководство по настройке проекта Google и экспорту данных из файлов Excel в Google Таблицы на Python.
Предварительные требования — экспорт данных Excel в таблицы Google на Python
Облачный проект Google
Для связи с Google Sheets нам нужно будет создать проект в Google Cloud и включить Google Sheets API. Кроме того, нам нужно создать учетные данные, которые используются для авторизации действий, которые мы собираемся выполнить с нашим кодом. Вы можете прочитать рекомендации о том, как создать проект Google Cloud и включить Google Sheets API.
После создания проекта Google Cloud и включения API Google Таблиц мы можем приступить к установке следующих API в нашем приложении Python.
Библиотеки Python для экспорта файлов Excel в Google Таблицы
Для экспорта данных из файлов Excel XLS/XLSX в Google Таблицы нам потребуются следующие API.
- Aspose.Cells для Python - To read the data from Excel files.
- Клиентские библиотеки Google - To create and update spreadsheets on Google Sheets.
Экспорт данных из Excel в Google Таблицы на Python
Ниже приведено пошаговое руководство о том, как считывать данные из файла Excel XLSX и записывать их в Google Sheets в приложении Python.
Создайте новое приложение Python.
Установите в проект Aspose.Cells и клиентские библиотеки Google.
pip install aspose.cells
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib
Поместите файл JSON (мы загрузили его после создания учетных данных в Google Cloud) в каталог проекта.
Напишите метод с именем createspreadsheet, который создает новую электронную таблицу в Google Sheets, задает имя таблицы по умолчанию и возвращает идентификатор электронной таблицы.
def create_spreadsheet(_service, _title, _sheetName):
# Spreadsheet details
spreadsheetBody = {
'properties': {
'title': "{0}".format(_title)
},
'sheets': {
'properties': {
'title' : "{0}".format(_sheetName)
}
}
}
# Create spreadsheet
spreadsheet = _service.spreadsheets().create(body=spreadsheetBody,
fields='spreadsheetId').execute()
print('Spreadsheet ID: {0}'.format(spreadsheet.get('spreadsheetId')))
print('Spreadsheet URL: "https://docs.google.com/spreadsheets/d/{0}'.format(spreadsheet.get('spreadsheetId')))
# Open in web browser
webbrowser.open_new_tab("https://docs.google.com/spreadsheets/d/{0}".format(spreadsheet.get('spreadsheetId')))
return spreadsheet.get('spreadsheetId')
- Напишите еще один метод с именем addsheet, чтобы добавить новый лист в электронную таблицу Google.
def add_sheet(_service, _spreadsheetID, _sheetName):
data = {'requests': [
{
'addSheet':{
'properties':{'title': '{0}'.format(_sheetName)}
}
}
]}
# Execute request
res = _service.spreadsheets().batchUpdate(spreadsheetId=_spreadsheetID, body=data).execute()
- Теперь инициализируйте службу Google Таблиц, используя учетные данные (файл JSON) и определите области действия приложения. Параметр scopes используется для указания прав доступа к Google Sheets и их свойствам.
# If modifying these scopes, delete the file token.json.
SCOPES = ['https://www.googleapis.com/auth/spreadsheets']
creds = None
# The file token.json stores the user's access and refresh tokens, and is
# created automatically when the authorization flow completes for the first
# time.
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json', SCOPES)
# If there are no (valid) credentials available, let the user log in.
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials1.json', SCOPES)
creds = flow.run_local_server(port=0)
# Save the credentials for the next run
with open('token.json', 'w') as token:
token.write(creds.to_json())
service = build('sheets', 'v4', credentials=creds)
- Затем загрузите файл Excel XLS или XLSX с помощью Aspose.Cells и получите имя первого листа в книге.
# Load Excel workbook
wb = Workbook(fileName)
# Get worksheets collection
collection = wb.getWorksheets()
collectionCount = collection.getCount()
# Get workbook and first sheet's name
spreadsheetName = wb.getFileName()
firstSheetName = collection.get(0).getName()
- Вызовите метод createpreadsheet, чтобы создать новую электронную таблицу в Google Sheets.
# Create spreadsheet on Google Sheets
spreadsheetID = create_spreadsheet(service, spreadsheetName, firstSheetName)
- Прокрутите рабочие листы в файле Excel. На каждой итерации считывайте данные с рабочего листа и добавляйте их в массив.
# Loop through all the worksheets
for worksheetIndex in range(collectionCount):
# Get worksheet using its index
worksheet = collection.get(worksheetIndex)
# Set worksheet range
if(worksheetIndex==0):
sheetRange= "{0}!A:Y".format(firstSheetName)
else:
add_sheet(service, spreadsheetID, worksheet.getName())
sheetRange= "{0}!A:Y".format(worksheet.getName())
# Get number of rows and columns
rows = worksheet.getCells().getMaxDataRow()
cols = worksheet.getCells().getMaxDataColumn()
# List to store worksheet's data
worksheetDatalist = []
# Loop through rows
for i in range(rows):
# List to store each row in worksheet
rowDataList = []
# Loop through each column in selected row
for j in range(cols):
cellValue = worksheet.getCells().get(i, j).getValue()
if( cellValue is not None):
rowDataList.append(str(cellValue))
else:
rowDataList.append("")
# Add to worksheet data
worksheetDatalist.append(rowDataList)
- Для каждого рабочего листа в файле Excel создайте запрос на запись данных в Google Sheets.
# Set values
body = {
'values': worksheetDatalist
}
# Execute request
result = service.spreadsheets().values().update(
spreadsheetId=spreadsheetID, range=sheetRange,
valueInputOption='USER_ENTERED', body=body).execute()
# Print number of updated cells
print('{0} cells updated.'.format(result.get('updatedCells')))
Ниже приведена полная функция для экспорта данных из файла Excel в электронную таблицу в Google Sheets.
def export_to_google(fileName):
# If modifying these scopes, delete the file token.json.
SCOPES = ['https://www.googleapis.com/auth/spreadsheets']
creds = None
# The file token.json stores the user's access and refresh tokens, and is
# created automatically when the authorization flow completes for the first
# time.
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json', SCOPES)
# If there are no (valid) credentials available, let the user log in.
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials1.json', SCOPES)
creds = flow.run_local_server(port=0)
# Save the credentials for the next run
with open('token.json', 'w') as token:
token.write(creds.to_json())
try:
service = build('sheets', 'v4', credentials=creds)
# Load Excel workbook
wb = Workbook(fileName)
# Get worksheets collection
collection = wb.getWorksheets()
collectionCount = collection.getCount()
# Get workbook and first sheet's name
spreadsheetName = wb.getFileName()
firstSheetName = collection.get(0).getName()
# Create spreadsheet on Google Sheets
spreadsheetID = create_spreadsheet(service, spreadsheetName, firstSheetName)
# To set worksheet range
sheetRange = None
# Loop through all the worksheets
for worksheetIndex in range(collectionCount):
# Get worksheet using its index
worksheet = collection.get(worksheetIndex)
# Set worksheet range
if(worksheetIndex==0):
sheetRange= "{0}!A:Y".format(firstSheetName)
else:
add_sheet(service, spreadsheetID, worksheet.getName())
sheetRange= "{0}!A:Y".format(worksheet.getName())
# Get number of rows and columns
rows = worksheet.getCells().getMaxDataRow()
cols = worksheet.getCells().getMaxDataColumn()
# List to store worksheet's data
worksheetDatalist = []
# Loop through rows
for i in range(rows):
# List to store each row in worksheet
rowDataList = []
# Loop through each column in selected row
for j in range(cols):
cellValue = worksheet.getCells().get(i, j).getValue()
if( cellValue is not None):
rowDataList.append(str(cellValue))
else:
rowDataList.append("")
# Add to worksheet data
worksheetDatalist.append(rowDataList)
# Set values
body = {
'values': worksheetDatalist
}
# Execute request
result = service.spreadsheets().values().update(
spreadsheetId=spreadsheetID, range=sheetRange,
valueInputOption='USER_ENTERED', body=body).execute()
# Print number of updated cells
print('{0} cells updated.'.format(result.get('updatedCells')))
except HttpError as err:
print(err)
print("Workbook has been exported to Google Sheets.")
Полный исходный код
Ниже приведен полный исходный код для экспорта файла Excel XLSX в Google Таблицы на Python.
from __future__ import print_function
import jpype
import webbrowser
import os.path
from google.auth.transport.requests import Request
from google.oauth2.credentials import Credentials
from google_auth_oauthlib.flow import InstalledAppFlow
from googleapiclient.discovery import build
from googleapiclient.errors import HttpError
import asposecells
jpype.startJVM()
from asposecells.api import Workbook, License
def export_to_google(fileName):
# If modifying these scopes, delete the file token.json.
SCOPES = ['https://www.googleapis.com/auth/spreadsheets']
creds = None
# The file token.json stores the user's access and refresh tokens, and is
# created automatically when the authorization flow completes for the first
# time.
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json', SCOPES)
# If there are no (valid) credentials available, let the user log in.
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials1.json', SCOPES)
creds = flow.run_local_server(port=0)
# Save the credentials for the next run
with open('token.json', 'w') as token:
token.write(creds.to_json())
try:
service = build('sheets', 'v4', credentials=creds)
# Load Excel workbook
wb = Workbook(fileName)
# Get worksheets collection
collection = wb.getWorksheets()
collectionCount = collection.getCount()
# Get workbook and first sheet's name
spreadsheetName = wb.getFileName()
firstSheetName = collection.get(0).getName()
# Create spreadsheet on Google Sheets
spreadsheetID = create_spreadsheet(service, spreadsheetName, firstSheetName)
# To set worksheet range
sheetRange = None
# Loop through all the worksheets
for worksheetIndex in range(collectionCount):
# Get worksheet using its index
worksheet = collection.get(worksheetIndex)
# Set worksheet range
if(worksheetIndex==0):
sheetRange= "{0}!A:Y".format(firstSheetName)
else:
add_sheet(service, spreadsheetID, worksheet.getName())
sheetRange= "{0}!A:Y".format(worksheet.getName())
# Get number of rows and columns
rows = worksheet.getCells().getMaxDataRow()
cols = worksheet.getCells().getMaxDataColumn()
# List to store worksheet's data
worksheetDatalist = []
# Loop through rows
for i in range(rows):
# List to store each row in worksheet
rowDataList = []
# Loop through each column in selected row
for j in range(cols):
cellValue = worksheet.getCells().get(i, j).getValue()
if( cellValue is not None):
rowDataList.append(str(cellValue))
else:
rowDataList.append("")
# Add to worksheet data
worksheetDatalist.append(rowDataList)
# Set values
body = {
'values': worksheetDatalist
}
# Execute request
result = service.spreadsheets().values().update(
spreadsheetId=spreadsheetID, range=sheetRange,
valueInputOption='USER_ENTERED', body=body).execute()
# Print number of updated cells
print('{0} cells updated.'.format(result.get('updatedCells')))
except HttpError as err:
print(err)
print("Workbook has been exported to Google Sheets.")
def create_spreadsheet(_service, _title, _sheetName):
# Spreadsheet details
spreadsheetBody = {
'properties': {
'title': "{0}".format(_title)
},
'sheets': {
'properties': {
'title' : "{0}".format(_sheetName)
}
}
}
# Create spreadsheet
spreadsheet = _service.spreadsheets().create(body=spreadsheetBody,
fields='spreadsheetId').execute()
# Open in web browser
webbrowser.open_new_tab("https://docs.google.com/spreadsheets/d/{0}".format(spreadsheet.get('spreadsheetId')))
return spreadsheet.get('spreadsheetId')
def add_sheet(_service, _spreadsheetID, _sheetName):
data = {'requests': [
{
'addSheet':{
'properties':{'title': '{0}'.format(_sheetName)}
}
}
]}
# Execute request
res = _service.spreadsheets().batchUpdate(spreadsheetId=_spreadsheetID, body=data).execute()
# Create a Aspose.Cells icense object
license = License()
# Set the license of Aspose.Cells to avoid the evaluation limitations
license.setLicense("D:\\Licenses\\Conholdate.Total.Product.Family.lic")
export_to_google("Book1.xlsx")
Получите бесплатную лицензию Aspose.Cells
Вы можете получить бесплатную временную лицензию и использовать Aspose.Cells for Python без ограничений на пробную версию.
Вывод
В этой статье вы узнали, как экспортировать данные Excel в Google Таблицы на Python. Мы рассмотрели, как создать проект в Google Cloud, включить API Google Таблиц, прочитать файлы Excel и экспортировать данные из файлов Excel в Google Таблицы. Чтобы узнать больше об Aspose.Cells для Python, вы можете посетить документацию. Также вы можете задать свои вопросы на нашем форуме.