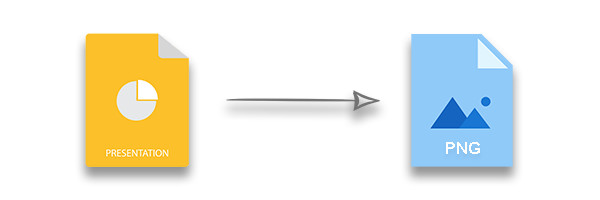
Often, you may need to display the PowerPoint PPTX or PPT presentations in your Python applications. The easiest way to achieve this is the conversion of slides to PNG images. In this article, you will learn how to convert PPT or PPTX to PNG images in Python. Furthermore, we will discuss how to generate PNG images of custom scale or size programmatically.
- Python Library for PPT to PNG Conversion
- Convert a PPT to PNG
- Image Scaling in PPT to PNG
- Custom Image Size in PPT to PNG
Python Library to Convert PPT to PNG
To convert the slides in PowerPoint presentations to PNG images, we will use Aspose.Slides for Python via .NET. It is a powerful Python library that lets you create and manipulate presentations seamlessly. Furthermore, it allows you to convert the presentations to other document and image formats. You can install the library from PyPI using the following pip command.
> pip install aspose.slides
Info: Aspose.Slides provides classes and methods that allow you to convert PPT to JPG and also perform the PNG to PPT and JPG to PPT reverse tasks. Similarly, you can use Aspose.Slides to convert JPG to PPT, convert PNG to PPT, convert HTML to PPT, convert PDF to PPT, etc.
Convert a PPT to PNG in Python
The following are the steps to convert a PPT file to PNG in Python.
- Load the PPT file using Presentation class.
- Start a loop to access each slide in the presentation.
- Get reference of each ISlide from Pesentation.slides collection using index.
- Convert slide to PNG using ISlide.get_thumbnail().save(string, ImageFormat.png) method.
The following code sample shows how to convert a PPTX to PNG in Python.
Image Scaling - Python PPTX to PNG
In the previous section, we generated PNG images of default dimensions. However, in certain cases, you need to scale the images to the desired dimensions. You can achieve this by providing the X and Y values to the get_thumbnail() method. The following are the steps to scale images in PPT to PNG conversion.
- Load the PPTX file using Presentation class.
- Create two variables to define X and Y values.
- Start a loop to access each slide in the presentation.
- Get reference of each ISlide from Pesentation.slides collection using index.
- Convert slide to PNG using ISlide.get_thumbnail(scale_x, scale_y).save(string, ImageFormat.png) method.
The following code sample generates PNG images from PPTX using custom dimensions in Python.
Custom Image Size in PPTX to PNG Conversion
You can also customize PPT to PNG conversion to generate images of desired width and height. The following are the steps to convert a PPT to PNG with custom image size in Python.
- Load the PPT file using Presentation class.
- Create a Size object containing width and height of the image.
- Start a loop to access each slide in the presentation.
- Get reference of each ISlide from Pesentation.slides collection using index.
- Convert slide to PNG using ISlide.get_thumbnail(Size).save(string, ImageFormat.png) method.
The following code sample shows how to convert a PPTX to PNG with custom image size in Python.
Get a Free License
You can use Aspose.Slides for Python via .NET without evaluation limitations by getting a temporary license.
Conclusion
PPT to PNG conversion could be useful in various scenarios such as when creating a PowerPoint slideshow. In this article, you have learned how to convert PPT or PPTX to PNG in Python. Furthermore, we have also covered how to scale the resultant images or generate images of desired width and height. You can explore other features of Aspose.Slides for Python via .NET using the documentation. In case you would have any queries, contact us on our forum.
See Also
Info: Using the API here, Aspose developed a free online PPT to PNG converter (that allows you to convert PowerPoint slide to PNG images) and PNG to PPT converter (that allows you to generate images based on slides in a presentation).