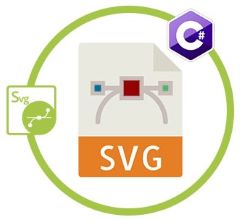
SVG (Scalable Vector Graphics) is an XML-based web-friendly vector file format. It enables developers and designers to create vector graphics using points, lines, paths, and shapes. You can use it to design logos, icons, simple graphics, and animations. Any text editor can create and edit SVG images since they are resolution-independent. Design is one of the most significant aspects of creating SVG, but the color is also equally critical. Colors can be applied to SVG shapes, lines, paths, and texts. It is possible to color, texture, shade, or build SVG graphics from partially transparent overlapping layers. In this article, we will learn how to work with SVG Fill Color and SVG Stroke Color in C#.
The following topics shall be covered in this article:
- SVG C# API for SVG Fill and Stroke Color
- What is SVG Fill
- What is SVG Stroke
- How to Use SVG Fill and SVG Stroke?
- How to Use SVG Fill Color and SVG Stroke Color
- Fill and Stroke Circle in SVG
- Fill and Stroke Path in SVG
- Apply Fill and Stroke using Style
SVG C# API for SVG Fill and Stroke Color
To work with SVG Fill Color and SVG Stroke Color in C#, we will be using the Aspose.SVG for .NET API. It allows loading, parsing, rendering, creation, and conversion of SVG files to popular formats without any software dependencies.
The SVGDocument class of the API represents the root of the SVG hierarchy and holds the entire content. The Save() method of this class allows saving SVG to the specified file path. The QuerySelector() method returns the first element in the document matching the selector. The API supports working with popular shapes such as rectangles, circles, ellipses, Line, polylines, etc. The API provides separate classes to represent these supported shapes such as SVGCircleElement for circle, SVGPolygonElement for polygon, etc.
Please either download the DLL of the API or install it using NuGet.
PM> Install-Package Aspose.SVG
What is SVG Fill?
The fill property is one of the basic SVG presentation attributes used to set the color of the shape inside its outline.
We can define the following fill attributes:
- fill: Sets the color to fill. Specify none or transparent if there is no filling.
- fill-opacity: Sets the opacity value between 0 to 1.
- fill-rule: Defines how the inside area of a shape is determined such as nonzero, evenodd.
What is SVG Stroke?
The stroke property defines the outline or a border of SVG shapes. The stroke presentation attribute sets the color of the outline of the shape.
We can set the following stroke attributes:
- stroke: Sets the color value.
- stroke-dasharray: Specifies the dashed line types.
- stroke-dashoffset
- stroke-linecap: Controls the shape of the ends of lines such as butt, square, and round.
- stroke-linejoin: Specifies how two lines join such as miter, bevel, and round.
- stroke-miterlimit: Must be greater than or equal to 1 if used.
- stroke-opacity: Sets the opacity value between 0 to 1.
- stroke-width: Sets the width.
How to Use SVG Fill and SVG Stroke?
Both the fill and stroke are used for adding color, gradients or patterns to graphics in SVG. The fill paints the interior of the SVG element, whereas, the stroke paints its outline. We can specify both fill and stroke in the style attribute or use them as presentation attributes.
We can set SVG fills and SVG strokes in the style attribute as shown below:
style="stroke:#00ff00; stroke-width:2; fill:#ff0000"
We can also use the same style properties in the presentation attributes as shown below:
stroke="green" stroke-width="2" fill="#ff0000"
How to Use SVG Fill Color and SVG Stroke Color
Color specification involves a variety of rules, such as:
The default color is Black, if the fill attribute is not specified.
The shape will not be filled with any color if the fill attribute has a none or transparent value.
There will be no stroke if the stroke attribute is not specified even if the stroke-width attribute is specified.
For specifying the SVG color, we can use color names such as red, blue, etc. We can also use RGB values or HEX values for the colors. Please find below a few of the examples showing different ways to set fill and stroke colors:
- SVG Color Names
SVG specification defines 147 color names. We can set any color by specifying its name as shown below:
stroke="Green"
fill="Red"
- HEX Color Codes
We can also specify six (6) digit color code represented in the form of #RRGGBB. There are two-digit hexadecimal pairs for three colors (Red, Green, and Blue) with values ranging from 00 to FF. We can set the green and red colors using HEX value as shown below:
stroke="#00FF00"
fill="#FF0000"
- RGB(Red, Blue, Green) Color Codes
We can specify RGB color values ranging from 0 to 255. We can set the green and red RGB colors values as shown below:
stroke="rgb(0,255,0)"
fill="rgb(255,0,0)"
- RGBA(Red, Blue, Green, Alpha) Color Codes
The RGBA color values are RGB color values enhanced by an alpha channel to determine their opacity. It is a number between 0.0 and 1.0 that specifies transparency. We can get the green and red RGB colors as shown below:
stroke="rgba(0,255,0,1.0)"
fill="rgba(255,0,0,1.0)"
- HSL Color Codes
HSL stands for Hue, Saturation and Lightness. Each color in HSL has an angle on the RGB color wheel as well as an intensity and saturation value as a percentage. We can get HSL codes for green and red colors as shown below:
stroke="hsl(120, 100%, 50%)"
fill="hsl(0, 100%, 50%)"
- HSLA(Hue, Saturation, Lightness, Alpha) Color Codes
Addition of Alpha channel value in HSL color value allows specifying the opacity of the color. We can get HSLA codes for green and red colors as shown below:
stroke="hsla(120, 100%, 50%, 1.0)"
fill="hsla(0, 100%, 50%, 1.0)"
Fill and Stroke Circle in C#
We can set SVG fill and SVG stroke properties for a circle by following the steps given below:
- Firstly, load an existing SVG using the SVGDocument class.
- Next, get the document’s root element.
- Then, find all the circle elements using the QuerySelectorAll() method.
- After that, set the fill attributes for the selected SVGCircleElement.
- Optionally, set the stroke attributes for the selected SVGCircleElement.
- Finally, save the output SVG image using the Save() method.
The following code sample shows how to set fill and stroke for a circle element of SVG in C#.
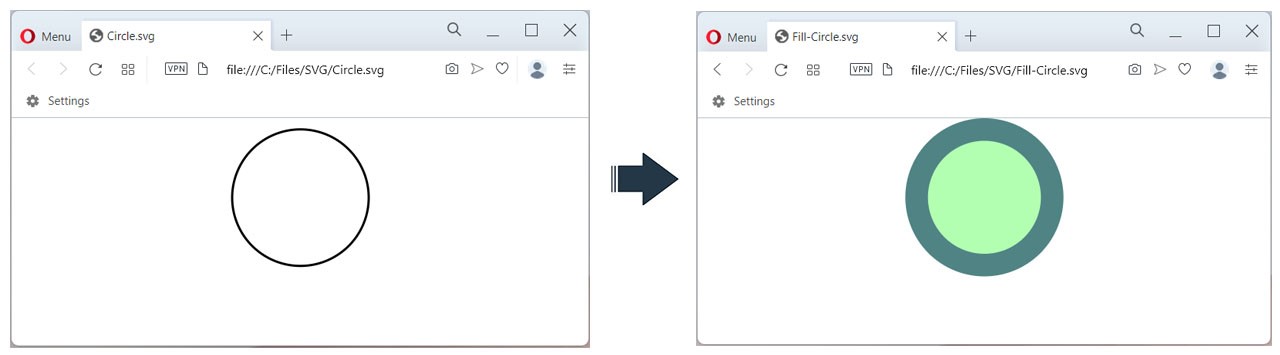
Set fill and stroke for circle element in C#.
Please find below the content of the Circle.svg image.
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 100 100">
<g stroke="black">
<circle r="30" cx="50" cy="35" fill="none"/>
</g>
</svg>
Here goes the content of the Fill-Circle.svg image.
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 100 100">
<g stroke="black">
<circle r="30" cx="50" cy="35" fill="#0F0" fill-opacity="0.3" stroke="#508484" stroke-width="10"/>
</g>
</svg>
Fill and Stroke Path in C#
We can set SVG fill and SVG stroke properties for a path element in SVG by following the steps given below:
- Firstly, load an existing SVG using the SVGDocument class.
- Next, get the document’s root element.
- Then, get the path element using the QuerySelector() method.
- After that, set the fill attributes for the selected SVGPathElement.
- Optionally, set the stroke attributes for the selected SVGPathElement.
- Finally, save the output SVG image using the Save() method.
The following code sample shows how to set fill and stroke for a path element of SVG in C#.
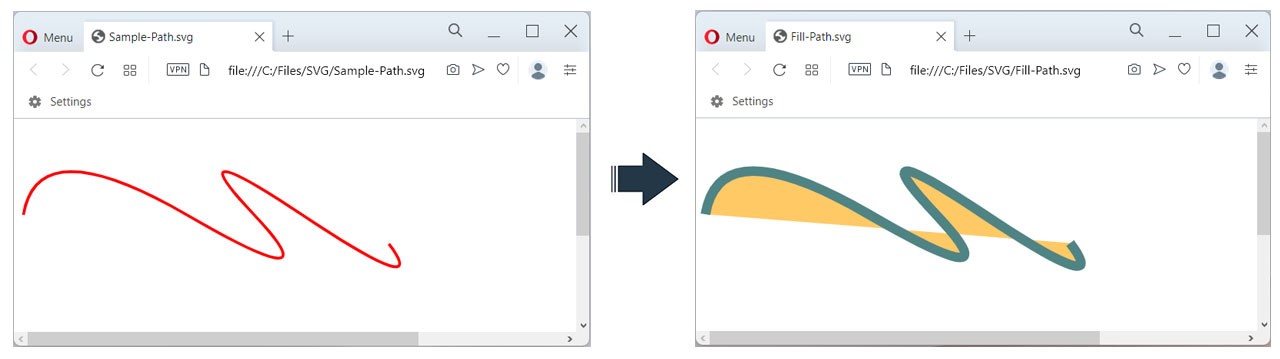
Set fill and stroke for path element in C#.
Please find below the content of the Sample-Path.svg image.
<svg height="400" width="800" xmlns="http://www.w3.org/2000/svg">
<g stroke="black">
<path d="M 10 100 Q 25 10 180 100 T 250 100 T 300 100 T 390 130" stroke="red" stroke-width="3" fill="none" />
</g>
</svg>
Please find below the content of the Fill-Path.svg image.
<svg height="400" width="800" xmlns="http://www.w3.org/2000/svg">
<g stroke="black">
<path d="M 10 100 Q 25 10 180 100 T 250 100 T 300 100 T 390 130" stroke="#508484" stroke-width="10" fill="orange" fill-opacity="0.6"/>
</g>
</svg>
Apply SVG Fill and SVG Stroke using Style in C#
We can also apply the SVG fill and SVG stroke properties using the CSS style attribute by following the steps given below:
- Firstly, create an instance of the SVGDocument class.
- Next, get the document’s root element.
- Then, create a circle element using the CreateElementNS() method.
- Next, set the circle’s basic properties such as Cx, Cy, and R.
- Then, apply the style attribute using the SetAttribute() method.
- After that, append the child using the AppendChild() method.
- Finally, save the output SVG image using the Save() method.
The following code sample shows how to apply the SVG fill and stroke using the CSS style in C#.
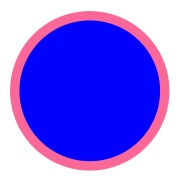
Apply the fill and stroke using CSS style
<svg xmlns="http://www.w3.org/2000/svg">
<g>
<circle cx="50" cy="50" r="40" style="fill: blue; stroke: rgb(251, 103, 150); stroke-width: 5;"/>
</g>
</svg>
Get Free Temporary License
You can get a free temporary license to try Aspose.SVG for .NET without evaluation limitations.
Conclusion
In this article, we have learned how to:
- create a new SVG image;
- load an existing SVG image;
- edit an SVG image;
- set fill and stroke attributes for shapes of SVG;
- set style attributes for the shapes in C#.
Besides, you can learn more about Aspose.SVG for .NET in the documentation and explore different features supported by the API. In case of any ambiguity, please feel free to contact us on our forum.