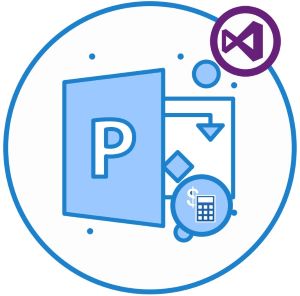
We can calculate project schedules or costs using calculation modes defined in Project options for Microsoft Project. A project schedule shows the start and end date of all project tasks. Microsoft Project allows the project calculation manually or automatically. As a C# developer, we can easily set project calculation mode in .NET applications and calculate project properties. In this article, we will learn how to calculate a project programmatically using C#.
The following topics shall be covered in this article:
- C# API to Calculate Project Programmatically
- Manual Project Calculation
- Automatic Project Calculation
- No Project Calculation Mode
C# API to Calculate Project Programmatically
For setting project calculation modes, we will be using the Aspose.Tasks for .NET API. It provides three project calculation modes, to calculate the values of dependent properties. These calculation modes are:
- None – it sets only necessary properties and does not recalculate project dates and costs.
- Manual – it sets only dependent object properties without recalculation of project dates and costs.
- Automatic – it recalculates project dates and cost properties automatically.
The API defines above-mentioned calculation modes in the CalculationMode enumeration. The CalculationMode property of the Project class allows setting or getting the value of the CalculationMode. The API also allows manipulating an existing project in order to add some modifications. Moreover, it facilitates performing basic as well as advanced project management operations seamlessly. Please either download the DLL of the API or install it using NuGet.
PM> Install-Package Aspose.Tasks
Manual Project Calculation using C#
We can set the calculation mode to manual and verify it by following the steps given below:
- Firstly, create an instance of the Project class.
- Next, set calculation mode to Manual.
- Then, specify the project start date.
- Next, add new tasks, e.g., Task 1, and Task 2.
- Then, read task properties set in manual mode.
- After that, initialize an object of the TaskLinks class and link tasks.
- Finally, verify the start and end date of Task 2.
The following code sample shows how to manually calculate a project using C#.
Task1.Id Equals 1 : True
Task1 OutlineLevel Equals 1 : True
Task1 Start Equals 15/04/2015 08:00 AM : True
Task1 Finish Equals 15/04/2015 05:00 PM : True
Task1 Duration Equals 1 day : True
Task2 Start Equals 15/04/2015 08:00 AM : True
Task2 Finish Equals 15/04/2015 05:00 PM : True
Task2 Duration Equals 1 day : True
Task1 Start Equals Task2 Start : True
Task1 Finish Equals Task2 Finish : True
Automatic Project Calculation using C#
We can set the calculation mode to automatic and verify it by following the steps given below:
- Firstly, create an instance of the Project class.
- Next, set calculation mode to Automatic.
- Then, set the project start date.
- Now, add new tasks, e.g., Task 1, and Task 2.
- After that, initialize an object of the TaskLinks class and link tasks.
- Finally, verify the recalculated dates.
The following code sample shows how to calculate the project automatically using C#.
Task1 Start + 1 Equals Task2 Start : True
Task1 Finish + 1 Equals Task2 Finish : True
RootTask Finish Equals Task2 Finish : True
Project Finish Date Equals Task2 Finish : True
No Project Calculation Mode in C#
We can set the calculation mode to none and verify it by following the steps given below:
- Firstly, create an instance of the Project class.
- Next, set calculation mode to None.
- Then, specify the project start date.
- Next, add a new task, e.g., Task 1.
- Then, read task properties.
- After that, set task duration in days.
- Finally, verify the start and end date of the Task.
The following code sample shows how to set project calculation to none using C#.
Task.Id Equals 0 : True
Task.OutlineLevel Equals 0 : False
Task Start Equals DateTime.MinValue : True
Task Finish Equals DateTime.MinValue : True
Task Duration Equals 0 mins : True
Task Duration Equals 2 days : True
Task Start Equals DateTime.MinValue : True
Task Finish Equals DateTime.MinValue : True
Get a Free License
You can get a free temporary license to try the library without evaluation limitations.
Conclusion
In this article, we have learned how to set project calculation modes programmatically. We have also seen how values of dependent properties are calculated in each calculation mode using C#. Besides, you can learn more about Aspose.Tasks for .NET API using the documentation. In case of any ambiguity, please feel free to contact us on the forum.