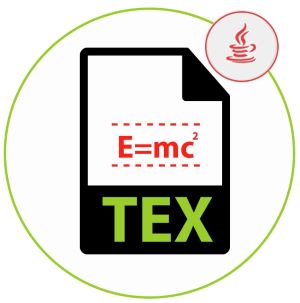
We can embed formulas and mathematical equations in the TeX file. LaTeX uses the TeX file as a source document that contains a set of commands. These commands specify the document’s format, including text, symbols, mathematical expressions, and graphics. We can write and render any kind of equations and mathematical formulas programmatically without using LaTeX. In this article, will learn how to render LaTeX math formulas and equations in Java.
The following topics shall be covered in this article:
- Java API to Render LaTeX Math Formulas & Equations
- Render LaTeX Math Formulas as PNG
- Render Complex Equations
- Display Long Equations
- Align Several Equations
- Group and Center Equations
- Render Parenthesis and Brackets
- Matrices in LaTeX
- Render Fractions and Binomials
- Mathematical Operators in LaTeX
- Render LaTeX Math Formulas as SVG
Java API to Render LaTeX Math Formulas & Equations
For rendering LaTeX math formulas, we will be using the Aspose.TeX for Java API. It provides the PngMathRendererOptions class for specifying the rendering options to save the Math formula as a PNG. Similarly, it also provides the SvgMathRendererOptions class for specifying the rendering options to save the Math formula as an SVG. Moreover, for the rendering of math formulas, it provides PngMathRenderer and SvgMathRenderer classes derived from the MathRenderer class. The render() method of this class renders the provided math formula. Furthermore, the API also allows typesetting TeX files to different file formats like PDF, XPS, or images.
Please either download the JAR of the API or add the following pom.xml configuration in a Maven-based Java application.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-tex</artifactId>
<version>22.4</version>
<type>jar</type>
</dependency>
Render LaTeX Math Formulas as PNG using Java
We can render simple inline math formulas or equations by following the steps given below:
- Firstly, create an instance of the PngMathRendererOptions class.
- Next, specify the image resolutions, such as 150 DPI.
- Then, specify the LaTeX document preamble.
- Optionally, set various properties such as Scale, TextColor, BackgroundColor, etc.
- After that, create the output stream for the formula image.
- Finally, call the render() method to render the formula. It takes formula string, stream, rendering options, and the output image size as arguments.
The following code sample shows how to render a math formula as a PNG programmatically using Java.

Render LaTeX Math Formulas as PNG using Java
Render Complex Equations using Java
We can render any complex equations or formulas by following the steps mentioned earlier. However, we just need to set the formula string in step # 5 as shown below:
mathRenderer.render("\\begin{equation*}"
+ "(1+x)^n = 1 + nx + \\frac{n(n-1)}{2!}x^{\\color{red}2}"
+ "+ \\frac{n(n-1)(n-2)}{3!}x^{\\color{red}3}"
+ "+ \\frac{n(n-1)(n-2)(n-3)}{4!}x^{\\color{red}4}"
+ "+ \\cdots"
+ "\\end{equation*}", stream, options, size);

Render Complex Equations using Java
Display Long Equations using Java
We can display long equations on multiple lines by following the steps mentioned earlier. However, we just need to set the formula string in step # 5 as shown below:
mathRenderer.render("\\begin{multline*}"
+ "p(x) = 3x^6 + 14x^5y + 590x^4y^2 + 19x^3y^3\\\\"
+ "- 12x^2y^4 - 12xy^5 + 2y^6 - a^3b^3"
+ "\\end{multline*}", stream, options, size);

Display Long Equations using Java.
Align Several Equations using Java
We can also align and render several equations or formulas at once by following the steps mentioned earlier. However, we just need to set the formula string in step # 5 as shown below:
mathRenderer.render("\\begin{align}"
+ "f(u) & =\\sum_{j=1}^{n} x_jf(u_j)\\\\"
+ "& =\\sum_{j=1}^{n} x_j \\sum_{i=1}^{m} a_{ij}v_i\\\\"
+ "& =\\sum_{j=1}^{n} \\sum_{i=1}^{m} a_{ij}x_jv_i"
+ "\\end{align}", stream, options, size);

Align Several Equations using Java.
Group and Center Equations using Java
We can group and center multiple equations while rendering by following the steps mentioned earlier. However, we just need to set the formula string in step # 5 as shown below:
mathRenderer.render("\\begin{gather*}"
+ "2x - 5y = 8 \\\\"
+ "3x^2 + 9y = 3a + c\\\\"
+ "(a-b) = a^2 + b^2 -2ab"
+ "\\end{gather*}", stream, options, size);
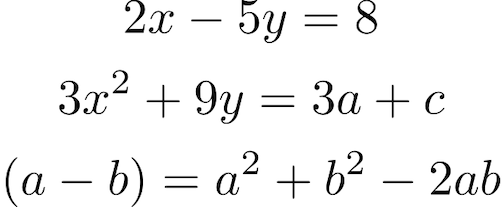
Group and Center Equations using Java.
Render Parenthesis and Brackets using Java
We can also render parenthesis and brackets by following the steps mentioned earlier. However, we just need to set the formula string in step # 5 as shown below:
mathRenderer.render("\\begin{document}"
+ "\["
+ " \\left[ \\frac{ N } { \\left( \\frac{L}{p} \\right) - (m+n) } \\right]"
+ "\]"
+ "\\end{document}", stream, options, size);
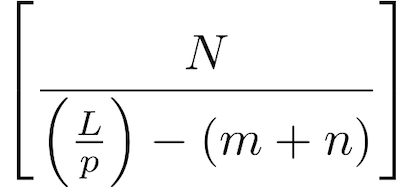
Render Parenthesis and Brackets using Java.
Matrices in LaTeX using Java
Similarly, we can render matrices by following the steps mentioned earlier. However, we just need to set the formula string in step # 5 as shown below:
mathRenderer.render("\\begin{document}"
+ "\["
+ "\\left \\{"
+ "\\begin{tabular}{ccc}"
+ " 1 & 4 & 7 \\\\"
+ " 2 & 5 & 8 \\\\"
+ " 3 & 6 & 9 "
+ "\\end{tabular}"
+ "\\right \\}"
+ "\]"
+ "\\end{document}", stream, options, size);
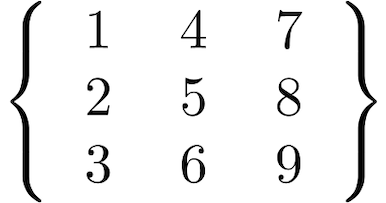
Matrices in LaTeX using Java.
Render Fractions and Binomials using Java
We can render fractions and binomials as well by following the steps mentioned earlier. However, we just need to set the formula string in step # 5 as shown below:
mathRenderer.render("\\begin{document}"
+ "\["
+ "\\binom{n}{k} = \\frac{n!}{k!(n-k)!}"
+ "\]"
+ "\\end{document}", stream, options, size);
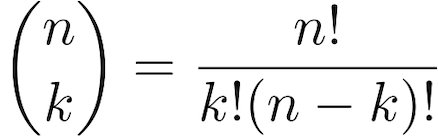
Render Fractions and Binomials using Java.
Mathematical Operators in LaTeX using Java
The mathematical operator symbols represent various mathematical functions such as log, cos, sin, etc. We can render trigonometrical functions and logarithms in LaTeX by following the steps mentioned earlier. However, we just need to set the formula string in step # 5 as shown below:
mathRenderer.render("\\begin{document}"
+ "\["
+ "\\sin(a + b) = \\sin a \\cos b + \\cos b \\sin a"
+ "\]"
+ "\\end{document}", stream, options, size);

Mathematical Operators in LaTeX using Java.
Render LaTeX Math Formulas in SVG using Java
We can also save the rendered math formulas or equations in SVG image format by following the steps given below:
- Firstly, create an instance of the SvgMathRendererOptions class.
- Next, specify the LaTeX document preamble.
- Optionally, set various properties such as Scale, TextColor, BackgroundColor, etc.
- After that, create the output stream for the formula image.
- Finally, call the render() method to render the formula. It takes formula string, stream, rendering options, and the output image size as arguments.
The following code sample shows how to render a math formula in an SVG image using Java.
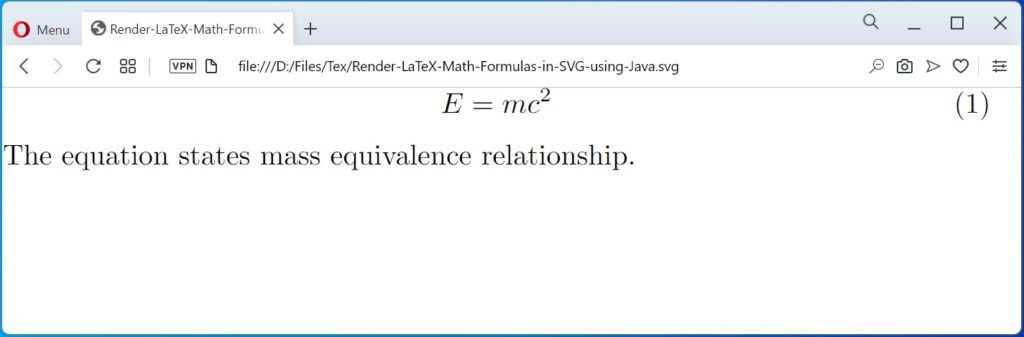
Render LaTeX Math Formulas in SVG using Java.
Get a Free License
You can get a free temporary license to try the library without evaluation limitations.
Conclusion
In this article, we have learned how to:
- render simple and complex mathematical formulas and equations in Java;
- align and group equations programmatically;
- render Matrices, Parenthesis, Brackets, Fractions, and Binomials;
- save the rendered formula images in PNG or SVG using Java.
Besides, you can learn more about Aspose.TeX for Java API using the documentation. In case of any ambiguity, please feel free to contact us on the forum.