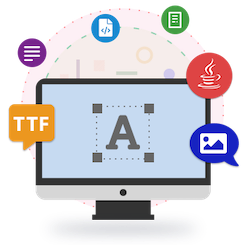
ใน บทความก่อนหน้า คุณได้เห็นวิธีการโหลดฟอนต์ CFF, TrueType และ Type1 โดยทางโปรแกรมโดยใช้ Java วันนี้เราจะพูดถึงคุณสมบัติที่น่าสนใจอีกอย่างของ API การจัดการฟอนต์ Java ของเรา นั่นคือการแสดงข้อความโดยใช้ฟอนต์ ในตอนท้ายของบทความนี้ คุณจะสามารถแสดงข้อความโดยใช้ฟอนต์ TrueType และ Type1 จากภายในแอปพลิเคชัน Java ของคุณ มาเริ่มกันเลย
- เกี่ยวกับ Java Font Manipulation API
- ใช้วิธีการเรนเดอร์ข้อความ
- แสดงผลข้อความโดยใช้ TrueType Fonts ใน Java
- แสดงผลข้อความโดยใช้แบบอักษร Type1 ใน Java
API การจัดการฟอนต์ Java
Aspose.Font for Java มอบคุณสมบัติในการโหลดและบันทึกฟอนต์ ตลอดจนรับเมตริกของประเภทฟอนต์ยอดนิยม ได้แก่ CFF, TrueType, OpenType และ Type1 นอกจากนี้ API ยังให้คุณแสดงข้อความโดยใช้ฟอนต์ TrueType หรือ Type1 ที่ให้มา คุณสามารถติดตั้ง API โดยใช้การกำหนดค่า Maven หรือ ดาวน์โหลด JAR ของ API
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-font</artifactId>
<version>20.10</version>
</dependency>
ใช้วิธีการเรนเดอร์ข้อความ
ในการแสดงข้อความ Aspose.Font for Java คุณต้องใช้เมธอด drawText() ซึ่งจะวาดข้อความที่ให้มา ต่อไปนี้เป็นคำจำกัดความที่สมบูรณ์ของเมธอด drawText()
// สำหรับตัวอย่างและไฟล์ข้อมูลทั้งหมด โปรดไปที่ https://github.com/aspose-font/Aspose.Font-for-Java
static void drawText(String text, IFont font, double fontSize,
Paint backgroundBrush, Paint textBrush, String outFile) throws Exception
{
//รับตัวระบุสัญลักษณ์สำหรับทุกสัญลักษณ์ในบรรทัดข้อความ
GlyphId[] gids = new GlyphId[text.length()];
for (int i = 0; i < text.length(); i++)
gids[i] = font.getEncoding().decodeToGid(text.charAt(i));
// ตั้งค่าการวาดทั่วไป
double dpi = 300;
double resolutionCorrection = dpi / 72; // 72 is font's internal dpi
// เตรียมบิตแมปเอาต์พุต
BufferedImage outBitmap = new BufferedImage(960, 720, BufferedImage.TYPE_INT_BGR);
//outBitmap.getRaster().SetResolution((ลอย)dpi, (ลอย)dpi);
java.awt.Graphics2D outGraphics = (java.awt.Graphics2D) outBitmap.getGraphics();
outGraphics.setPaint(backgroundBrush);
outGraphics.fillRect(0, 0, outBitmap.getWidth(), outBitmap.getHeight());
outGraphics.setRenderingHint(RenderingHints.KEY_RENDERING, RenderingHints.VALUE_RENDER_QUALITY);
//ประกาศตัวแปรพิกัดและ gid ก่อนหน้า
GlyphId previousGid = null;
double glyphXCoordinate = 0;
double glyphYCoordinate = fontSize * resolutionCorrection;
//วนซ้ำซึ่งวาดภาพทุกสัญลักษณ์ใน gids
for (GlyphId gid : gids)
{
// หากแบบอักษรมี gid
if (gid != null)
{
Glyph glyph = font.getGlyphAccessor().getGlyphById(gid);
if (glyph == null)
continue;
// เส้นทางที่ยอมรับคำแนะนำการวาดภาพ
GeneralPath path = new GeneralPath();
// สร้างการใช้งาน IGlyphOutlinePainter
GlyphOutlinePainter outlinePainter = new GlyphOutlinePainter(path);
// สร้างตัวเรนเดอร์
IGlyphRenderer renderer = new GlyphOutlineRenderer(outlinePainter);
// รับคุณสมบัติของสัญลักษณ์ทั่วไป
double kerning = 0;
// รับค่าการจัดช่องไฟ
if (previousGid != null)
{
kerning = (font.getMetrics().getKerningValue(previousGid, gid) /
glyph.getSourceResolution()) * fontSize * resolutionCorrection;
kerning += fontWidthToImageWdith(font.getMetrics().getGlyphWidth(previousGid),
glyph.getSourceResolution(), fontSize, 300);
}
// ตำแหน่งสัญลักษณ์ - เพิ่มพิกัดสัญลักษณ์ X ตามระยะการจัดช่องไฟ
glyphXCoordinate += kerning;
// เมทริกซ์ตำแหน่งสัญลักษณ์
TransformationMatrix glyphMatrix =
new TransformationMatrix(
new double[]
{
fontSize*resolutionCorrection,
0,
0,
// ลบเนื่องจากระบบพิกัดบิตแมปเริ่มต้นจากด้านบน
- fontSize*resolutionCorrection,
glyphXCoordinate,
glyphYCoordinate
});
// แสดงสัญลักษณ์ปัจจุบัน
renderer.renderGlyph(font, gid, glyphMatrix);
// เติมเส้นทาง
path.setWindingRule(GeneralPath.WIND_NON_ZERO);
outGraphics.setPaint(textBrush);
outGraphics.fill(path);
}
//ตั้งค่า gid ปัจจุบันเป็นก่อนหน้าเพื่อรับการจัดช่องไฟที่ถูกต้องสำหรับสัญลักษณ์ถัดไป
previousGid = gid;
}
//บันทึกผลลัพธ์
ImageIO.write(outBitmap, "jpg", new File(outFile));
}
ต่อไปนี้เป็นวิธียูทิลิตี้ที่ใช้ในการคำนวณความกว้างของสัญลักษณ์สำหรับระบบพิกัดบิตแมป
// สำหรับตัวอย่างและไฟล์ข้อมูลทั้งหมด โปรดไปที่ https://github.com/aspose-font/Aspose.Font-for-Java
static double fontWidthToImageWidth(double width, int fontSourceResulution, double fontSize, double dpi)
{
double resolutionCorrection = dpi / 72; // 72 is font's internal dpi
return (width / fontSourceResulution) * fontSize * resolutionCorrection;
}
ขั้นตอนในการ Render ข้อความด้วย Font ใน Java
ต่อไปนี้เป็นขั้นตอนของวิธีแสดงข้อความที่ระบุเป็นไฟล์รูปภาพโดยใช้เมธอด darwText() ที่กล่าวถึงข้างต้น
- โหลดฟอนต์โดยใช้คลาส FontDefinition
- เข้าถึงแบบอักษรโดยใช้คลาส เช่น Type1Font หรือ TtfFont
- เรียกใช้เมธอด drawText() โดยระบุรายละเอียดในรูปแบบของพารามิเตอร์
Render ข้อความด้วย TrueType Font โดยใช้ Java
ตัวอย่างโค้ดต่อไปนี้แสดงวิธีใช้เมธอด drawText() เพื่อแสดงข้อความโดยใช้ฟอนต์ TrueType ผลการเรนเดอร์จะถูกสร้างเป็นภาพ JPEG
// สำหรับตัวอย่างและไฟล์ข้อมูลทั้งหมด โปรดไปที่ https://github.com/aspose-font/Aspose.Font-for-Java
String fileName1 = Utils.getDataDir() + "Montserrat-Bold.ttf"; //Font file name with full path
FontDefinition fd1 = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName1)));
TtfFont font1 = (TtfFont) Font.open(fd1);
String fileName2 = Utils.getDataDir() + "Lora-Bold.ttf"; //Font file name with full path
FontDefinition fd2 = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName2)));
TtfFont font2 = (TtfFont) Font.open(fd2);
try {
drawText("Hello world", font1, 14, java.awt.Color.WHITE, java.awt.Color.BLACK, Utils.getDataDir() + "hello1_montserrat_out.jpg");
drawText("Hello world", font2, 14, java.awt.Color.YELLOW, java.awt.Color.RED, Utils.getDataDir() + "hello2_lora_out.jpg");
} catch (Exception ex) {
ex.printStackTrace();
}
แสดงผลข้อความโดยใช้ฟอนต์ Type1 ใน Java
ตัวอย่างโค้ดต่อไปนี้แสดงวิธีแสดงข้อความเป็นรูปภาพ JPEG ด้วยฟอนต์ Type1 โดยใช้ Java
// สำหรับตัวอย่างและไฟล์ข้อมูลทั้งหมด โปรดไปที่ https://github.com/aspose-font/Aspose.Font-for-Java
String fileName = Utils.getDataDir() + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = (Type1Font) Font.open(fd);
try {
drawText("Hello world", font, 14, java.awt.Color.WHITE, java.awt.Color.BLACK, Utils.getDataDir() + "hello1_type1_out.jpg");
drawText("Hello world", font, 14, java.awt.Color.YELLOW, java.awt.Color.RED, Utils.getDataDir() + "hello2_type1_out.jpg");
} catch (Exception ex) {
ex.printStackTrace();
}
บทสรุป
ในบทความนี้ คุณได้เห็นวิธีแสดงข้อความเป็นรูปภาพ JPEG โดยใช้ฟอนต์ TrueType หรือ Type1 จากภายในแอปพลิเคชัน Java หากต้องการเรียนรู้เพิ่มเติมเกี่ยวกับ API การจัดการฟอนต์ Java คุณสามารถไปที่ เอกสารประกอบ และประเมินคุณลักษณะของ API โดยใช้ ตัวอย่างซอร์สโค้ด