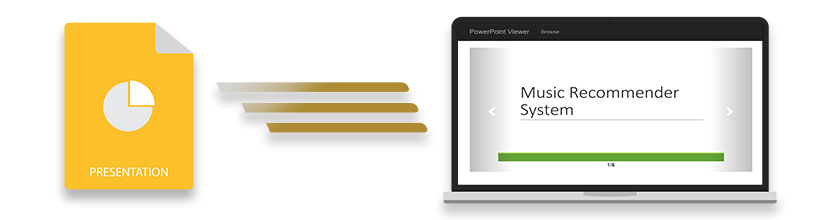
คุณกำลังมองหา PowerPoint Viewer เพื่อดูหรือฝังงานนำเสนอในเว็บแอปพลิเคชัน ASP.NET Core ของคุณหรือไม่ ถ้าใช่ โปรดอ่านบทความนี้ต่อและเรียนรู้วิธีสร้าง ASP.NET Core PowerPoint Viewer และแสดงงานนำเสนอ PPT/PPTX โดยใช้ C# มาเริ่มกันเลย
คุณสมบัติของ ASP.NET PowerPoint Viewer
ASP.NET PowerPoint Viewer ของเราจะใช้ Aspose.Slides for .NET API เพื่อเรนเดอร์สไลด์งานนำเสนอเป็นภาพ PNG เมื่อเรนเดอร์สไลด์แล้ว เราจะแสดงโดยใช้ Bootstrap Carousel ต่อไปนี้จะเป็นคุณสมบัติของแอปพลิเคชัน:
- เรียกดูและดูงานนำเสนอ PowerPoint (PPT/PPTX)
- ตั้งค่าไฟล์ PowerPoint เริ่มต้นที่จะแสดงในการโหลดหน้า
- แถบเลื่อนเพื่อนำทางระหว่างสไลด์
ขั้นตอนในการสร้าง PowerPoint Viewer ใน ASP.NET Core
ต่อไปนี้เป็นขั้นตอนง่ายๆ ในการสร้าง PowerPoint Viewer ใน ASP.NET Core
- สร้าง ASP.NET Core Web Application ใหม่ใน Visual Studio
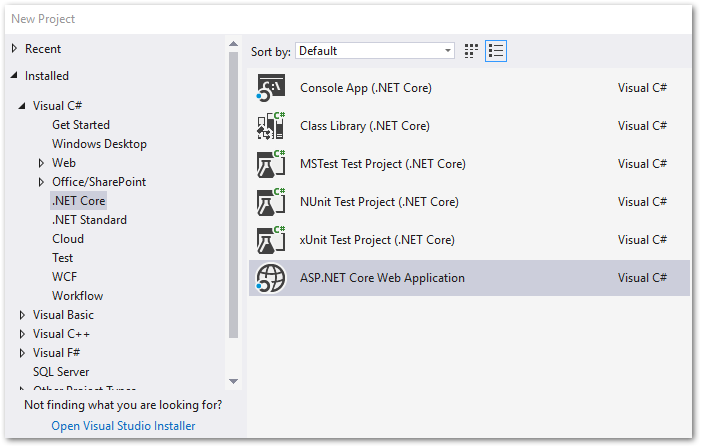
- เปิด NuGet Package Manager และติดตั้งแพ็คเกจ Aspose.Slides for .NET
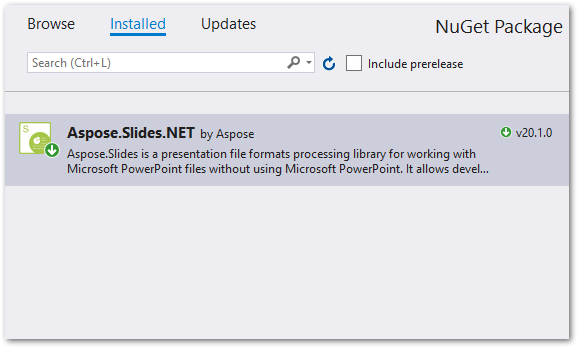
- สร้างโฟลเดอร์ Presentations และ Slides ใน wwwroot เพื่อเก็บไฟล์ PowerPoint และสไลด์ที่แสดงผลตามลำดับ
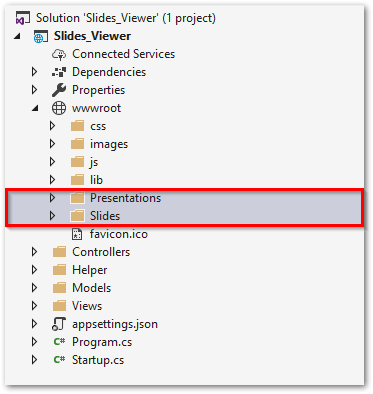
สร้างโฟลเดอร์ใหม่ด้วยชื่อ “ผู้ช่วยเหลือ” ในโฟลเดอร์รูท
สร้างคลาสใหม่ชื่อ “สไลด์” ในโฟลเดอร์ “ตัวช่วย” เพื่อเก็บข้อมูลของสไลด์นำเสนอ
public class Slide
{
public int SlideNumber { get; set; }
public string Path { get; set; }
}
- เปิด “HomeController.cs” และแทนที่รหัสด้วยสิ่งต่อไปนี้ (อัปเดตชื่อไฟล์ PowerPoint เริ่มต้นในการดำเนินการดัชนี)
public class HomeController : Controller
{
public List<Helper.Slide> slides;
public IHostingEnvironment _env;
public HomeController(IHostingEnvironment env)
{
_env = env;
}
[HttpGet]
public ActionResult Index(string fileName)
{
slides = new List<Helper.Slide>();
if (fileName == null)
{
// แสดงไฟล์ PowerPoint เริ่มต้นเมื่อโหลดหน้า
slides = RenderPresentationAsImage("presentation.pptx");
}
else
{
slides = RenderPresentationAsImage(fileName);
}
return View(slides);
}
public List<Helper.Slide> RenderPresentationAsImage(string FileName)
{
var webRoot = _env.WebRootPath;
// โหลดงานนำเสนอ PowerPoint
Presentation presentation = new Presentation(Path.Combine(webRoot, "Presentations", FileName));
var slides = new List<Helper.Slide>();
string imagePath = "";
// บันทึกและดูสไลด์นำเสนอ
for (int j = 0; j < presentation.Slides.Count; j++)
{
ISlide sld = presentation.Slides[j];
Bitmap bmp = sld.GetThumbnail(1f, 1f);
imagePath = Path.Combine(webRoot, "Slides", string.Format("slide_{0}.png", j));
bmp.Save(imagePath, System.Drawing.Imaging.ImageFormat.Png);
// เพิ่มในรายการ
slides.Add(new Helper.Slide { SlideNumber = j, Path = string.Format("slide_{0}.png", j) });
}
return slides;
}
}
- เปิด Views/Home/index.cshtml และแทนที่เนื้อหาด้วยสิ่งต่อไปนี้
@{
ViewData["Title"] = "PowerPoint Viewer";
}
@{
Layout = null;
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Slides Viewer</title>
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.css" />
<link rel="stylesheet" href="~/css/site.css" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
</head>
<body>
<div class="container">
<nav class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="sr-only">Toggle navigation</span>
</button>
<a asp-area="" asp-controller="Home" asp-action="Index" class="navbar-brand">PowerPoint Viewer</a>
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li style="cursor: pointer;"><a data-toggle="modal" data-target="#exampleModal">Browse</a></li>
</ul>
</div>
</div>
</nav>
<br />
<div id="myCarousel" class="carousel slide" data-interval="false">
<div class="carousel-inner" role="listbox" style="width:100%; height: auto !important;">
@for (int i = 0; i < Model.Count; i++)
{
if (i == 0)
{
<div class="item active">
<img src="~/Slides/@Model[i].Path" style="width: 80%; height: auto; margin:auto !important" class="img-responsive " />
<p style="text-align:center;">
<strong> @(Model[i].SlideNumber + 1)/@Model.Count</strong>
</p>
</div>
}
else
{
<div class="item">
<img src="~/Slides/@Model[i].Path" style="width: 80%; height: auto; margin:auto !important" class="img-responsive " />
<p style="text-align:center;">
<strong> @(Model[i].SlideNumber + 1)/@Model.Count</strong>
</p>
</div>
}
}
</div>
<a class="left carousel-control" href="#myCarousel" role="button" data-slide="prev">
<span class="glyphicon glyphicon-chevron-left" aria-hidden="true"></span>
<span class="sr-only">Previous</span>
</a>
<a class="right carousel-control" href="#myCarousel" role="button" data-slide="next">
<span class="glyphicon glyphicon-chevron-right" aria-hidden="true"></span>
<span class="sr-only">Next</span>
</a>
</div>
</div>
<!-- Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Select a file</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<div class="list-group">
@foreach (string file in System.IO.Directory.EnumerateFiles("wwwroot/Presentations","*"))
{
string fileName = System.IO.Path.GetFileName(file);
@Html.ActionLink(fileName, "Index", "Home", new { fileName = fileName }, new { @class = "list-group-item" })
}
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
<script src="~/lib/jquery/dist/jquery.js"></script>
<script src="~/lib/bootstrap/dist/js/bootstrap.js"></script>
<script src="~/js/site.min.js" asp-append-version="true"></script>
</body>
</html>
- สร้างแอปพลิเคชันและเรียกใช้งานในเบราว์เซอร์ที่คุณชื่นชอบ
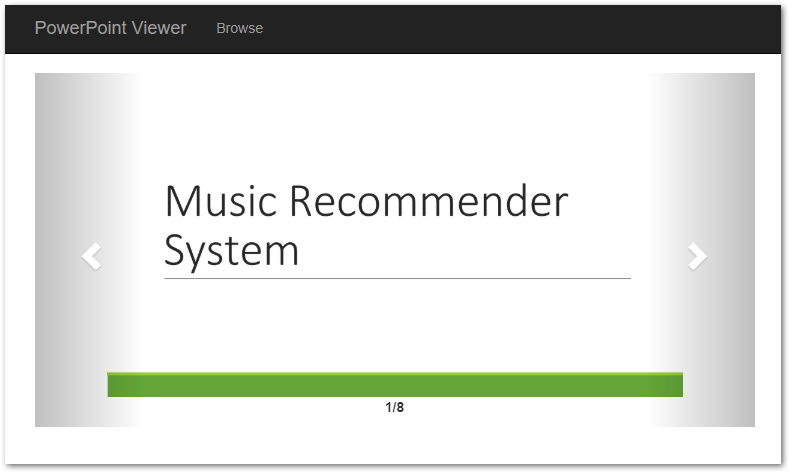
ดูสิ่งนี้ด้วย
รับใบอนุญาตชั่วคราวสำหรับ Aspose.Slides สำหรับ .NET
คุณสามารถรับ ใบอนุญาตชั่วคราว ของ Aspose.Slides สำหรับ .NET เพื่อหลีกเลี่ยงข้อจำกัดในการทดลองใช้งาน
เคล็ดลับ: คุณอาจต้องการดู การใช้งานจริงของการดำเนินการดูสำหรับงานนำเสนอ