Bài viết này hướng dẫn cách chuyển đổi MS Excel XLS hoặc XLSX sang Google Trang tính theo lập trình trong Java.
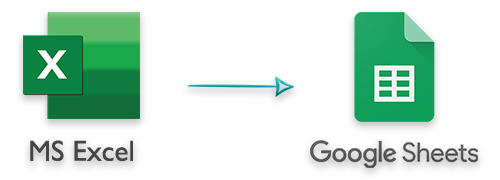
Google Trang tính là một ứng dụng trực tuyến phổ biến cho phép bạn tạo và thao tác các tài liệu bảng tính. Hơn nữa, nó cho phép bạn chia sẻ bảng tính với nhiều người trong thời gian thực. Do đó, bạn thường phải xuất dữ liệu từ sổ làm việc Excel sang bảng tính trong Google Trang tính theo chương trình. Trong bài viết này, chúng tôi sẽ giới thiệu đầy đủ về cách xuất dữ liệu Excel sang Google Trang tính bằng Java.
Điều kiện tiên quyết - Xuất Dữ liệu Excel sang Google Trang tính bằng Java
Dự án đám mây của Google
Google Cloud là một nền tảng điện toán đám mây, cung cấp nhiều loại dịch vụ khác nhau mà chúng tôi có thể sử dụng trong các ứng dụng của mình. Để sử dụng API Google Trang tính, chúng tôi sẽ phải tạo một dự án trên bảng điều khiển Google Cloud và bật API Google Trang tính. Bạn có thể đọc hướng dẫn từng bước về cách tạo dự án Google Cloud và bật API Trang tính.
API Java để xuất dữ liệu Excel sang Google Trang tính
Để xuất dữ liệu từ tệp Excel XLS / XLSX sang Google Trang tính, chúng tôi sẽ cần các API sau.
- Aspose.Cells dành cho Java - To read the data from Excel files.
- Google Client APIs dành cho Java - Để tạo và cập nhật bảng tính trên Google Trang tính.
Để cài đặt các API này, bạn có thể sử dụng các cấu hình sau trong tệp pom.xml của mình.
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>com.google.api-client</groupId>
<artifactId>google-api-client-gson</artifactId>
<version>1.33.0</version>
</dependency>
<dependency>
<groupId>com.google.oauth-client</groupId>
<artifactId>google-oauth-client-jetty</artifactId>
<version>1.32.1</version>
</dependency>
<dependency>
<groupId>com.google.apis</groupId>
<artifactId>google-api-services-sheets</artifactId>
<version>v4-rev20210629-1.32.1</version>
</dependency>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-cells</artifactId>
<version>22.2</version>
</dependency>
</dependencies>
Xuất Dữ liệu Excel sang Google Trang tính bằng Java
Sau đây là các bước để đọc tệp Excel và sau đó xuất dữ liệu của tệp đó sang Google Trang tính trong ứng dụng bảng điều khiển Java.
Tạo một ứng dụng Java mới (máy tính để bàn).
Cài đặt Aspose.Cells for Java và API máy khách Google trong dự án, như đã đề cập trong phần trước.
Sao chép tệp JSON (tệp chúng tôi đã tải xuống sau khi tạo thông tin đăng nhập trong Google Cloud) và dán vào thư mục của dự án.
Chỉ định phạm vi ứng dụng xác định quyền truy cập vào trang tính. Ngoài ra, hãy tạo các biến cho đường dẫn thư mục mã thông báo và nhà máy JSON.
/**
* Phiên bản toàn cầu của phạm vi được yêu cầu. Nếu sửa đổi các phạm vi này, hãy xóa
* thư mục / mã thông báo đã lưu trước đó của bạn.
*/
private static final String APPLICATION_NAME = "Google Sheets API Java Quickstart";
private static final JsonFactory JSON_FACTORY = GsonFactory.getDefaultInstance();
private static final String TOKENS_DIRECTORY_PATH = "tokens";
private static final List<String> SCOPES = Collections.singletonList(SheetsScopes.SPREADSHEETS);
- Tạo phương thức getCredentials cho phép người dùng sử dụng tệp thông tin xác thực.
private static Credential getCredentials(final NetHttpTransport HTTP_TRANSPORT) throws IOException {
// Tải thông tin bí mật của khách hàng
InputStream in = new FileInputStream(new File("credentials.json"));
GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new InputStreamReader(in));
// Xây dựng quy trình và kích hoạt yêu cầu ủy quyền người dùng
GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder(HTTP_TRANSPORT, JSON_FACTORY,
clientSecrets, SCOPES)
.setDataStoreFactory(new FileDataStoreFactory(new java.io.File(TOKENS_DIRECTORY_PATH)))
.setAccessType("offline").build();
LocalServerReceiver receiver = new LocalServerReceiver.Builder().setPort(8888).build();
return new AuthorizationCodeInstalledApp(flow, receiver).authorize("user");
}
- Tạo phương thức createS Spreadsheet để tạo một bảng tính mới trên Google Sheets và đặt tên cho trang tính mặc định. Phương thức này trả về một đối tượng Bảng tính.
private static Spreadsheet createSpreadsheet(Sheets _service, String spreadsheetName, String _defaultSheetName)
throws IOException {
// Tạo một bảng tính mới
Spreadsheet spreadsheet = new Spreadsheet()
.setProperties(new SpreadsheetProperties().setTitle(spreadsheetName));
// Tạo một trang tính mới
Sheet sheet = new Sheet();
sheet.setProperties(new SheetProperties());
sheet.getProperties().setTitle(_defaultSheetName);
// Thêm trang tính vào danh sách
List<Sheet> sheetList = new ArrayList<Sheet>();
sheetList.add(sheet);
spreadsheet.setSheets(sheetList);
// Thực hiện yêu cầu
spreadsheet = _service.spreadsheets().create(spreadsheet).setFields("spreadsheetId").execute();
System.out.println("Spreadsheet ID: " + spreadsheet.getSpreadsheetId());
return spreadsheet;
}
- Tạo phương thức addSheet để thêm một trang tính mới trong bảng tính Google.
private static void addSheet(Sheets _service, String _spreadSheetID, String _sheetName) {
try {
// Thêm trang tính mới
AddSheetRequest addSheetRequest = new AddSheetRequest();
addSheetRequest.setProperties(new SheetProperties());
addSheetRequest.getProperties().setTitle(_sheetName);
// Tạo yêu cầu cập nhật
BatchUpdateSpreadsheetRequest batchUpdateSpreadsheetRequest = new BatchUpdateSpreadsheetRequest();
Request req = new Request();
req.setAddSheet(addSheetRequest);
batchUpdateSpreadsheetRequest.setRequests(new ArrayList<Request>());
batchUpdateSpreadsheetRequest.getRequests().add(req);
// Thực hiện yêu cầu
_service.spreadsheets().batchUpdate(_spreadSheetID, batchUpdateSpreadsheetRequest).execute();
} catch (Exception e) {
System.out.println("Error in creating sheet: " + e.getMessage());
}
}
- Bây giờ, hãy tạo phương thức exportExcelToGoogle và trong phương pháp này, khởi chạy dịch vụ Trang tính.
// Xây dựng một dịch vụ ứng dụng khách API được ủy quyền mới.
final NetHttpTransport HTTP_TRANSPORT = GoogleNetHttpTransport.newTrustedTransport();
Sheets service = new Sheets.Builder(HTTP_TRANSPORT, JSON_FACTORY, getCredentials(HTTP_TRANSPORT))
.setApplicationName(APPLICATION_NAME).build();
- Sau đó, tải tệp Excel XLS / XLSX bằng Aspose.Cells for Java. Ngoài ra, lấy tên của trang tính đầu tiên trong sổ làm việc.
// Tải sổ làm việc Excel
Workbook wb = new Workbook(_excelFileName);
// Lấy tên của trang tính đầu tiên
String defaultWorksheetName = wb.getWorksheets().get(0).getName().trim();
// Nhận tất cả các trang tính
WorksheetCollection collection = wb.getWorksheets();
- Gọi phương thức createS Spreadsheet để tạo một bảng tính mới trên Google Trang tính.
// Tạo bảng tính Google mới với trang tính mặc định
Spreadsheet spreadsheet = createSpreadsheet(service, wb.getFileName(), defaultWorksheetName);
- Đọc dữ liệu từ mỗi trang tính và lưu vào danh sách.
String range;
// Lặp qua các trang tính
for (int worksheetIndex = 0; worksheetIndex < collection.getCount(); worksheetIndex++) {
// Tham khảo bảng tính
Worksheet ws = collection.get(worksheetIndex);
if (worksheetIndex == 0) {
// Trang tính đầu tiên được tạo theo mặc định, vì vậy chỉ đặt phạm vi
range = defaultWorksheetName + "!A:Y";
} else {
// Thêm một trang tính mới
addSheet(service, spreadsheet.getSpreadsheetId(), ws.getName().trim());
range = ws.getName().trim() + "!A:Y";
}
// Nhận số hàng và cột
int rows = ws.getCells().getMaxDataRow();
int cols = ws.getCells().getMaxDataColumn();
List<List<Object>> worksheetData = new ArrayList<List<Object>>();
// Lặp qua các hàng
for (int i = 0; i <= rows; i++) {
List<Object> rowData = new ArrayList<Object>();
// Lặp qua từng cột trong hàng đã chọn
for (int j = 0; j <= cols; j++) {
if (ws.getCells().get(i, j).getValue() == null)
rowData.add("");
else
rowData.add(ws.getCells().get(i, j).getValue());
}
// Thêm vào dữ liệu trang tính
worksheetData.add(rowData);
}
// VIỆC CẦN LÀM: Thực hiện yêu cầu đăng dữ liệu lên Google Trang tính
}
- Đối với mỗi trang tính trong tệp Excel, hãy tạo yêu cầu ghi dữ liệu vào bảng tính trong Google Trang tính.
// Đặt dải
ValueRange body = new ValueRange();
body.setRange(range);
// Đặt giá trị
body.setValues(worksheetData);
// Xuất giá trị sang Google Trang tính
UpdateValuesResponse result = service.spreadsheets().values()
.update(spreadsheet.getSpreadsheetId(), range, body).setValueInputOption("USER_ENTERED")
.execute();
// In đầu ra
System.out.printf("%d cells updated.", result.getUpdatedCells());
Dưới đây là phương pháp hoàn chỉnh để đọc và xuất dữ liệu từ tệp Excel sang bảng tính trong Google Trang tính.
private static void exportExcelToGoogle(String _excelFileName) {
try {
// Xây dựng một dịch vụ ứng dụng khách API được ủy quyền mới.
final NetHttpTransport HTTP_TRANSPORT = GoogleNetHttpTransport.newTrustedTransport();
Sheets service = new Sheets.Builder(HTTP_TRANSPORT, JSON_FACTORY, getCredentials(HTTP_TRANSPORT))
.setApplicationName(APPLICATION_NAME).build();
// Tải sổ làm việc Excel
Workbook wb = new Workbook(_excelFileName);
// Lấy tên của trang tính đầu tiên
String defaultWorksheetName = wb.getWorksheets().get(0).getName().trim();
// Nhận tất cả các trang tính
WorksheetCollection collection = wb.getWorksheets();
// Tạo bảng tính Google mới với trang tính mặc định
Spreadsheet spreadsheet = createSpreadsheet(service, wb.getFileName(), defaultWorksheetName);
System.out.println("Spreadsheet URL: " + spreadsheet.getSpreadsheetUrl());
System.out.println("ID: " + spreadsheet.getSpreadsheetId());
String range;
// Lặp qua các trang tính
for (int worksheetIndex = 0; worksheetIndex < collection.getCount(); worksheetIndex++) {
// Tham khảo bảng tính
Worksheet ws = collection.get(worksheetIndex);
if (worksheetIndex == 0) {
// Trang tính đầu tiên được tạo theo mặc định, vì vậy chỉ đặt phạm vi
range = defaultWorksheetName + "!A:Y";
} else {
// Thêm một trang tính mới
addSheet(service, spreadsheet.getSpreadsheetId(), ws.getName().trim());
range = ws.getName().trim() + "!A:Y";
}
// Nhận số hàng và cột
int rows = ws.getCells().getMaxDataRow();
int cols = ws.getCells().getMaxDataColumn();
List<List<Object>> worksheetData = new ArrayList<List<Object>>();
// Lặp qua các hàng
for (int i = 0; i <= rows; i++) {
List<Object> rowData = new ArrayList<Object>();
// Lặp qua từng cột trong hàng đã chọn
for (int j = 0; j <= cols; j++) {
if (ws.getCells().get(i, j).getValue() == null)
rowData.add("");
else
rowData.add(ws.getCells().get(i, j).getValue());
}
// Thêm vào dữ liệu trang tính
worksheetData.add(rowData);
}
// Đặt dải
ValueRange body = new ValueRange();
body.setRange(range);
// Đặt giá trị
body.setValues(worksheetData);
// Xuất giá trị sang Google Trang tính
UpdateValuesResponse result = service.spreadsheets().values()
.update(spreadsheet.getSpreadsheetId(), range, body).setValueInputOption("USER_ENTERED")
.execute();
// In đầu ra
System.out.printf("%d cells updated.", result.getUpdatedCells());
}
// Mở bảng tính Google trong trình duyệt
Desktop desk = Desktop.getDesktop();
desk.browse(new URI("https://docs.google.com/spreadsheets/d/" + spreadsheet.getSpreadsheetId()));
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
Hoàn thành mã nguồn
Sau đây là mã nguồn hoàn chỉnh để chuyển đổi tệp Excel XLSX sang Google Trang tính trong Java.
package excel_to_google_sheets;
import com.aspose.cells.Workbook;
import com.aspose.cells.Worksheet;
import com.aspose.cells.WorksheetCollection;
import com.google.api.client.auth.oauth2.Credential;
import com.google.api.client.extensions.java6.auth.oauth2.AuthorizationCodeInstalledApp;
import com.google.api.client.extensions.jetty.auth.oauth2.LocalServerReceiver;
import com.google.api.client.googleapis.auth.oauth2.GoogleAuthorizationCodeFlow;
import com.google.api.client.googleapis.auth.oauth2.GoogleClientSecrets;
import com.google.api.client.googleapis.javanet.GoogleNetHttpTransport;
import com.google.api.client.http.javanet.NetHttpTransport;
import com.google.api.client.json.JsonFactory;
import com.google.api.client.json.gson.GsonFactory;
import com.google.api.client.util.store.FileDataStoreFactory;
import com.google.api.services.sheets.v4.Sheets;
import com.google.api.services.sheets.v4.SheetsScopes;
import com.google.api.services.sheets.v4.model.AddSheetRequest;
import com.google.api.services.sheets.v4.model.BatchUpdateSpreadsheetRequest;
import com.google.api.services.sheets.v4.model.Request;
import com.google.api.services.sheets.v4.model.Sheet;
import com.google.api.services.sheets.v4.model.SheetProperties;
import com.google.api.services.sheets.v4.model.Spreadsheet;
import com.google.api.services.sheets.v4.model.SpreadsheetProperties;
import com.google.api.services.sheets.v4.model.UpdateValuesResponse;
import com.google.api.services.sheets.v4.model.ValueRange;
import java.awt.Desktop;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.URI;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class ExportExcelToGoogle {
/**
* Phiên bản toàn cầu của phạm vi được yêu cầu. Nếu sửa đổi các phạm vi này, hãy xóa
* thư mục / mã thông báo đã lưu trước đó của bạn.
*/
private static final String APPLICATION_NAME = "Google Sheets API Java Quickstart";
private static final JsonFactory JSON_FACTORY = GsonFactory.getDefaultInstance();
private static final String TOKENS_DIRECTORY_PATH = "tokens";
private static final List<String> SCOPES = Collections.singletonList(SheetsScopes.SPREADSHEETS);
public static void main(String[] args) throws Exception {
// Xuất dữ liệu Excel sang Google Trang tính
exportExcelToGoogle("workbook.xlsx");
}
private static void exportExcelToGoogle(String _excelFileName) {
try {
// Xây dựng một dịch vụ ứng dụng khách API được ủy quyền mới.
final NetHttpTransport HTTP_TRANSPORT = GoogleNetHttpTransport.newTrustedTransport();
Sheets service = new Sheets.Builder(HTTP_TRANSPORT, JSON_FACTORY, getCredentials(HTTP_TRANSPORT))
.setApplicationName(APPLICATION_NAME).build();
// Tải sổ làm việc Excel
Workbook wb = new Workbook(_excelFileName);
// Lấy tên của trang tính đầu tiên
String defaultWorksheetName = wb.getWorksheets().get(0).getName().trim();
// Nhận tất cả các trang tính
WorksheetCollection collection = wb.getWorksheets();
// Tạo bảng tính Google mới với trang tính mặc định
Spreadsheet spreadsheet = createSpreadsheet(service, wb.getFileName(), defaultWorksheetName);
String range;
// Lặp qua các trang tính
for (int worksheetIndex = 0; worksheetIndex < collection.getCount(); worksheetIndex++) {
// Tham khảo bảng tính
Worksheet ws = collection.get(worksheetIndex);
if (worksheetIndex == 0) {
// Trang tính đầu tiên được tạo theo mặc định, vì vậy chỉ đặt phạm vi
range = defaultWorksheetName + "!A:Y";
} else {
// Thêm một trang tính mới
addSheet(service, spreadsheet.getSpreadsheetId(), ws.getName().trim());
range = ws.getName().trim() + "!A:Y";
}
// Nhận số hàng và cột
int rows = ws.getCells().getMaxDataRow();
int cols = ws.getCells().getMaxDataColumn();
List<List<Object>> worksheetData = new ArrayList<List<Object>>();
// Lặp qua các hàng
for (int i = 0; i <= rows; i++) {
List<Object> rowData = new ArrayList<Object>();
// Lặp qua từng cột trong hàng đã chọn
for (int j = 0; j <= cols; j++) {
if (ws.getCells().get(i, j).getValue() == null)
rowData.add("");
else
rowData.add(ws.getCells().get(i, j).getValue());
}
// Thêm vào dữ liệu trang tính
worksheetData.add(rowData);
}
// Đặt dải
ValueRange body = new ValueRange();
body.setRange(range);
// Đặt giá trị
body.setValues(worksheetData);
// Xuất giá trị sang Google Trang tính
UpdateValuesResponse result = service.spreadsheets().values()
.update(spreadsheet.getSpreadsheetId(), range, body).setValueInputOption("USER_ENTERED")
.execute();
// In đầu ra
System.out.printf("%d cells updated.", result.getUpdatedCells());
}
// Mở bảng tính Google trong trình duyệt
Desktop desk = Desktop.getDesktop();
desk.browse(new URI("https://docs.google.com/spreadsheets/d/" + spreadsheet.getSpreadsheetId()));
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
private static Spreadsheet createSpreadsheet(Sheets _service, String spreadsheetName, String _defaultSheetName)
throws IOException {
// Tạo một bảng tính mới
Spreadsheet spreadsheet = new Spreadsheet()
.setProperties(new SpreadsheetProperties().setTitle(spreadsheetName));
// Tạo một trang tính mới
Sheet sheet = new Sheet();
sheet.setProperties(new SheetProperties());
sheet.getProperties().setTitle(_defaultSheetName);
// Thêm trang tính vào danh sách
List<Sheet> sheetList = new ArrayList<Sheet>();
sheetList.add(sheet);
spreadsheet.setSheets(sheetList);
// Thực hiện yêu cầu
spreadsheet = _service.spreadsheets().create(spreadsheet).setFields("spreadsheetId").execute();
System.out.println("Spreadsheet ID: " + spreadsheet.getSpreadsheetId());
return spreadsheet;
}
private static void addSheet(Sheets _service, String _spreadSheetID, String _sheetName) {
try {
// Thêm trang tính mới
AddSheetRequest addSheetRequest = new AddSheetRequest();
addSheetRequest.setProperties(new SheetProperties());
addSheetRequest.getProperties().setTitle(_sheetName);
// Tạo yêu cầu cập nhật
BatchUpdateSpreadsheetRequest batchUpdateSpreadsheetRequest = new BatchUpdateSpreadsheetRequest();
Request req = new Request();
req.setAddSheet(addSheetRequest);
batchUpdateSpreadsheetRequest.setRequests(new ArrayList<Request>());
batchUpdateSpreadsheetRequest.getRequests().add(req);
// Thực hiện yêu cầu
_service.spreadsheets().batchUpdate(_spreadSheetID, batchUpdateSpreadsheetRequest).execute();
} catch (Exception e) {
System.out.println("Error in creating sheet: " + e.getMessage());
}
}
private static Credential getCredentials(final NetHttpTransport HTTP_TRANSPORT) throws IOException {
// Tải thông tin bí mật của khách hàng
InputStream in = new FileInputStream(new File("credentials.json"));
GoogleClientSecrets clientSecrets = GoogleClientSecrets.load(JSON_FACTORY, new InputStreamReader(in));
// Xây dựng quy trình và kích hoạt yêu cầu ủy quyền người dùng
GoogleAuthorizationCodeFlow flow = new GoogleAuthorizationCodeFlow.Builder(HTTP_TRANSPORT, JSON_FACTORY,
clientSecrets, SCOPES)
.setDataStoreFactory(new FileDataStoreFactory(new java.io.File(TOKENS_DIRECTORY_PATH)))
.setAccessType("offline").build();
LocalServerReceiver receiver = new LocalServerReceiver.Builder().setPort(8888).build();
return new AuthorizationCodeInstalledApp(flow, receiver).authorize("user");
}
}
Demo - Chuyển đổi Excel sang Google Trang tính trong Java
Java Excel sang API Google Trang tính - Nhận Giấy phép Miễn phí
Bạn có thể nhận giấy phép tạm thời miễn phí và sử dụng Aspose.Cells for Java mà không có giới hạn đánh giá.
Sự kết luận
Trong bài viết này, bạn đã học cách xuất tệp Excel XLS hoặc XLSX sang Google Trang tính theo lập trình bằng Java. Chúng tôi đã cung cấp hướng dẫn đầy đủ về cách tạo dự án Google Cloud, bật API Google Trang tính, đọc tệp Excel và xuất dữ liệu từ tệp Excel sang Google Trang tính. Bạn cũng có thể truy cập tài liệu để đọc thêm về Aspose.Cells for Java. Trong trường hợp bạn có bất kỳ câu hỏi hoặc thắc mắc nào, hãy cho chúng tôi biết qua diễn đàn của chúng tôi.