Trong bài viết này, bạn sẽ học cách xuất dữ liệu Excel sang Google Trang tính theo lập trình bằng Python.
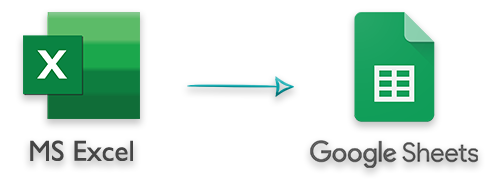
Tệp Excel được sử dụng rộng rãi để lưu trữ dữ liệu và thực hiện nhiều loại hoạt động khác nhau trên đó, chẳng hạn như tạo biểu đồ, áp dụng công thức. Mặt khác, Google Sheets là một ứng dụng trực tuyến phổ biến để tạo và thao tác các bảng tính. Google Trang tính cũng cung cấp tính năng chia sẻ bảng tính trong thời gian thực với nhiều người. Trong một số trường hợp nhất định, bạn có thể cần xuất tệp Excel XLS hoặc XLSX sang Google Trang tính theo lập trình. Để đạt được điều đó, bài viết này cung cấp hướng dẫn đầy đủ về cách thiết lập dự án Google và xuất dữ liệu từ tệp Excel sang Google Trang tính bằng Python.
Điều kiện tiên quyết - Xuất Dữ liệu Excel sang Google Trang tính bằng Python
Dự án đám mây của Google
Để giao tiếp với Google Trang tính, chúng tôi sẽ phải tạo một dự án trên Google Cloud và bật API Google Trang tính. Ngoài ra, chúng ta cần tạo thông tin xác thực được sử dụng để cho phép các hành động mà chúng ta sẽ thực hiện với mã của mình. Bạn có thể đọc hướng dẫn về cách tạo dự án Google Cloud và bật API Google Trang tính.
Sau khi tạo dự án Google Cloud và bật API Google Trang tính, chúng tôi có thể tiến hành cài đặt các API sau trong ứng dụng Python của mình.
Thư viện Python để xuất tệp Excel sang Google Trang tính
Để xuất dữ liệu từ tệp Excel XLS / XLSX sang Google Trang tính, chúng tôi sẽ cần các API sau.
- Aspose.Cells dành cho Python - To read the data from Excel files.
- Thư viện khách hàng của Google - To create and update spreadsheets on Google Sheets.
Xuất dữ liệu từ Excel sang Google Trang tính bằng Python
Sau đây là hướng dẫn từng bước về cách đọc dữ liệu từ tệp Excel XLSX và ghi dữ liệu đó vào Google Trang tính trong ứng dụng Python.
Tạo một ứng dụng Python mới.
Cài đặt Aspose.Cells và thư viện ứng dụng khách Google trong dự án.
pip install aspose.cells
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib
Đặt tệp JSON (chúng tôi đã tải xuống sau khi tạo thông tin đăng nhập trong Google Cloud) vào thư mục của dự án.
Viết một phương thức có tên là tạo bảng tính để tạo một bảng tính mới trên Google Trang tính, đặt tên cho trang tính mặc định và trả về ID của bảng tính.
def create_spreadsheet(_service, _title, _sheetName):
# Chi tiết bảng tính
spreadsheetBody = {
'properties': {
'title': "{0}".format(_title)
},
'sheets': {
'properties': {
'title' : "{0}".format(_sheetName)
}
}
}
# Tạo bảng tính
spreadsheet = _service.spreadsheets().create(body=spreadsheetBody,
fields='spreadsheetId').execute()
print('Spreadsheet ID: {0}'.format(spreadsheet.get('spreadsheetId')))
print('Spreadsheet URL: "https://docs.google.com/spreadsheets/d/{0}'.format(spreadsheet.get('spreadsheetId')))
# Mở trong trình duyệt web
webbrowser.open_new_tab("https://docs.google.com/spreadsheets/d/{0}".format(spreadsheet.get('spreadsheetId')))
return spreadsheet.get('spreadsheetId')
- Viết một phương thức khác có tên là bảng bổ sung để thêm một trang tính mới trong bảng tính Google.
def add_sheet(_service, _spreadsheetID, _sheetName):
data = {'requests': [
{
'addSheet':{
'properties':{'title': '{0}'.format(_sheetName)}
}
}
]}
# Thực hiện yêu cầu
res = _service.spreadsheets().batchUpdate(spreadsheetId=_spreadsheetID, body=data).execute()
- Bây giờ, khởi tạo dịch vụ Google Trang tính bằng thông tin đăng nhập (tệp JSON) và xác định phạm vi của ứng dụng. Tham số phạm vi được sử dụng để chỉ định quyền truy cập vào Google Trang tính và các thuộc tính của chúng.
# Nếu sửa đổi các phạm vi này, hãy xóa tệp token.json.
SCOPES = ['https://www.googleapis.com/auth/spreadsheets']
creds = None
# Tệp token.json lưu trữ mã thông báo truy cập và làm mới của người dùng và
# được tạo tự động khi quy trình ủy quyền hoàn tất cho lần đầu tiên
# thời gian.
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json', SCOPES)
# Nếu không có thông tin xác thực (hợp lệ) nào khả dụng, hãy để người dùng đăng nhập.
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials1.json', SCOPES)
creds = flow.run_local_server(port=0)
# Lưu thông tin đăng nhập cho lần chạy tiếp theo
with open('token.json', 'w') as token:
token.write(creds.to_json())
service = build('sheets', 'v4', credentials=creds)
- Sau đó, tải tệp Excel XLS hoặc XLSX bằng Aspose.Cells và lấy tên của trang tính đầu tiên trong sổ làm việc.
# Tải sổ làm việc Excel
wb = Workbook(fileName)
# Nhận bộ sưu tập trang tính
collection = wb.getWorksheets()
collectionCount = collection.getCount()
# Nhận bảng tính và tên trang tính đầu tiên
spreadsheetName = wb.getFileName()
firstSheetName = collection.get(0).getName()
- Gọi phương thức tạo bảng tính để tạo bảng tính mới trên Google Trang tính.
# Tạo bảng tính trên Google Trang tính
spreadsheetID = create_spreadsheet(service, spreadsheetName, firstSheetName)
- Lặp qua các trang tính trong tệp Excel. Trong mỗi lần lặp, hãy đọc dữ liệu từ trang tính và thêm nó vào một mảng.
# Lặp lại tất cả các trang tính
for worksheetIndex in range(collectionCount):
# Nhận trang tính bằng cách sử dụng chỉ mục của nó
worksheet = collection.get(worksheetIndex)
# Đặt phạm vi trang tính
if(worksheetIndex==0):
sheetRange= "{0}!A:Y".format(firstSheetName)
else:
add_sheet(service, spreadsheetID, worksheet.getName())
sheetRange= "{0}!A:Y".format(worksheet.getName())
# Nhận số hàng và cột
rows = worksheet.getCells().getMaxDataRow()
cols = worksheet.getCells().getMaxDataColumn()
# Danh sách để lưu trữ dữ liệu của trang tính
worksheetDatalist = []
# Lặp qua các hàng
for i in range(rows):
# Danh sách để lưu trữ từng hàng trong trang tính
rowDataList = []
# Lặp qua từng cột trong hàng đã chọn
for j in range(cols):
cellValue = worksheet.getCells().get(i, j).getValue()
if( cellValue is not None):
rowDataList.append(str(cellValue))
else:
rowDataList.append("")
# Thêm vào dữ liệu trang tính
worksheetDatalist.append(rowDataList)
- Đối với mỗi trang tính trong tệp Excel, hãy tạo yêu cầu ghi dữ liệu vào Google Trang tính.
# Đặt giá trị
body = {
'values': worksheetDatalist
}
# Thực hiện yêu cầu
result = service.spreadsheets().values().update(
spreadsheetId=spreadsheetID, range=sheetRange,
valueInputOption='USER_ENTERED', body=body).execute()
# In số lượng ô đã cập nhật
print('{0} cells updated.'.format(result.get('updatedCells')))
Sau đây là toàn bộ hàm xuất dữ liệu từ tệp Excel sang bảng tính trong Google Trang tính.
def export_to_google(fileName):
# Nếu sửa đổi các phạm vi này, hãy xóa tệp token.json.
SCOPES = ['https://www.googleapis.com/auth/spreadsheets']
creds = None
# Tệp token.json lưu trữ các mã thông báo truy cập và làm mới của người dùng, đồng thời
# được tạo tự động khi quy trình ủy quyền hoàn tất cho lần đầu tiên
# thời gian.
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json', SCOPES)
# Nếu không có thông tin xác thực (hợp lệ) nào khả dụng, hãy để người dùng đăng nhập.
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials1.json', SCOPES)
creds = flow.run_local_server(port=0)
# Lưu thông tin đăng nhập cho lần chạy tiếp theo
with open('token.json', 'w') as token:
token.write(creds.to_json())
try:
service = build('sheets', 'v4', credentials=creds)
# Tải sổ làm việc Excel
wb = Workbook(fileName)
# Nhận bộ sưu tập trang tính
collection = wb.getWorksheets()
collectionCount = collection.getCount()
# Nhận bảng tính và tên trang tính đầu tiên
spreadsheetName = wb.getFileName()
firstSheetName = collection.get(0).getName()
# Tạo bảng tính trên Google Trang tính
spreadsheetID = create_spreadsheet(service, spreadsheetName, firstSheetName)
# Để đặt phạm vi trang tính
sheetRange = None
# Lặp lại tất cả các trang tính
for worksheetIndex in range(collectionCount):
# Nhận trang tính bằng cách sử dụng chỉ mục của nó
worksheet = collection.get(worksheetIndex)
# Đặt phạm vi trang tính
if(worksheetIndex==0):
sheetRange= "{0}!A:Y".format(firstSheetName)
else:
add_sheet(service, spreadsheetID, worksheet.getName())
sheetRange= "{0}!A:Y".format(worksheet.getName())
# Nhận số hàng và cột
rows = worksheet.getCells().getMaxDataRow()
cols = worksheet.getCells().getMaxDataColumn()
# Danh sách lưu trữ dữ liệu của trang tính
worksheetDatalist = []
# Lặp qua các hàng
for i in range(rows):
# Danh sách để lưu trữ từng hàng trong trang tính
rowDataList = []
# Lặp qua từng cột trong hàng đã chọn
for j in range(cols):
cellValue = worksheet.getCells().get(i, j).getValue()
if( cellValue is not None):
rowDataList.append(str(cellValue))
else:
rowDataList.append("")
# Thêm vào dữ liệu trang tính
worksheetDatalist.append(rowDataList)
# Đặt giá trị
body = {
'values': worksheetDatalist
}
# Thực hiện yêu cầu
result = service.spreadsheets().values().update(
spreadsheetId=spreadsheetID, range=sheetRange,
valueInputOption='USER_ENTERED', body=body).execute()
# In số lượng ô đã cập nhật
print('{0} cells updated.'.format(result.get('updatedCells')))
except HttpError as err:
print(err)
print("Workbook has been exported to Google Sheets.")
Hoàn thành mã nguồn
Sau đây là mã nguồn hoàn chỉnh để xuất tệp Excel XLSX sang Google Trang tính bằng Python.
from __future__ import print_function
import jpype
import webbrowser
import os.path
from google.auth.transport.requests import Request
from google.oauth2.credentials import Credentials
from google_auth_oauthlib.flow import InstalledAppFlow
from googleapiclient.discovery import build
from googleapiclient.errors import HttpError
import asposecells
jpype.startJVM()
from asposecells.api import Workbook, License
def export_to_google(fileName):
# Nếu sửa đổi các phạm vi này, hãy xóa tệp token.json.
SCOPES = ['https://www.googleapis.com/auth/spreadsheets']
creds = None
# Tệp token.json lưu trữ mã thông báo truy cập và làm mới của người dùng và
# được tạo tự động khi quy trình ủy quyền hoàn tất cho lần đầu tiên
# thời gian.
if os.path.exists('token.json'):
creds = Credentials.from_authorized_user_file('token.json', SCOPES)
# Nếu không có thông tin xác thực (hợp lệ) nào khả dụng, hãy để người dùng đăng nhập.
if not creds or not creds.valid:
if creds and creds.expired and creds.refresh_token:
creds.refresh(Request())
else:
flow = InstalledAppFlow.from_client_secrets_file(
'credentials1.json', SCOPES)
creds = flow.run_local_server(port=0)
# Lưu thông tin đăng nhập cho lần chạy tiếp theo
with open('token.json', 'w') as token:
token.write(creds.to_json())
try:
service = build('sheets', 'v4', credentials=creds)
# Tải sổ làm việc Excel
wb = Workbook(fileName)
# Nhận bộ sưu tập trang tính
collection = wb.getWorksheets()
collectionCount = collection.getCount()
# Nhận bảng tính và tên trang tính đầu tiên
spreadsheetName = wb.getFileName()
firstSheetName = collection.get(0).getName()
# Tạo bảng tính trên Google Trang tính
spreadsheetID = create_spreadsheet(service, spreadsheetName, firstSheetName)
# Để đặt phạm vi trang tính
sheetRange = None
# Lặp lại tất cả các trang tính
for worksheetIndex in range(collectionCount):
# Nhận trang tính bằng cách sử dụng chỉ mục của nó
worksheet = collection.get(worksheetIndex)
# Đặt phạm vi trang tính
if(worksheetIndex==0):
sheetRange= "{0}!A:Y".format(firstSheetName)
else:
add_sheet(service, spreadsheetID, worksheet.getName())
sheetRange= "{0}!A:Y".format(worksheet.getName())
# Nhận số hàng và cột
rows = worksheet.getCells().getMaxDataRow()
cols = worksheet.getCells().getMaxDataColumn()
# Danh sách lưu trữ dữ liệu của trang tính
worksheetDatalist = []
# Lặp qua các hàng
for i in range(rows):
# Danh sách để lưu trữ từng hàng trong trang tính
rowDataList = []
# Lặp qua từng cột trong hàng đã chọn
for j in range(cols):
cellValue = worksheet.getCells().get(i, j).getValue()
if( cellValue is not None):
rowDataList.append(str(cellValue))
else:
rowDataList.append("")
# Thêm vào dữ liệu trang tính
worksheetDatalist.append(rowDataList)
# Đặt giá trị
body = {
'values': worksheetDatalist
}
# Thực hiện yêu cầu
result = service.spreadsheets().values().update(
spreadsheetId=spreadsheetID, range=sheetRange,
valueInputOption='USER_ENTERED', body=body).execute()
# In số lượng ô đã cập nhật
print('{0} cells updated.'.format(result.get('updatedCells')))
except HttpError as err:
print(err)
print("Workbook has been exported to Google Sheets.")
def create_spreadsheet(_service, _title, _sheetName):
# Chi tiết bảng tính
spreadsheetBody = {
'properties': {
'title': "{0}".format(_title)
},
'sheets': {
'properties': {
'title' : "{0}".format(_sheetName)
}
}
}
# Tạo bảng tính
spreadsheet = _service.spreadsheets().create(body=spreadsheetBody,
fields='spreadsheetId').execute()
# Mở trong trình duyệt web
webbrowser.open_new_tab("https://docs.google.com/spreadsheets/d/{0}".format(spreadsheet.get('spreadsheetId')))
return spreadsheet.get('spreadsheetId')
def add_sheet(_service, _spreadsheetID, _sheetName):
data = {'requests': [
{
'addSheet':{
'properties':{'title': '{0}'.format(_sheetName)}
}
}
]}
# Thực hiện yêu cầu
res = _service.spreadsheets().batchUpdate(spreadsheetId=_spreadsheetID, body=data).execute()
# Tạo một đối tượng Aspose.Cells icense
license = License()
# Đặt giấy phép của Aspose.Cells để tránh các giới hạn đánh giá
license.setLicense("D:\\Licenses\\Conholdate.Total.Product.Family.lic")
export_to_google("Book1.xlsx")
Nhận giấy phép Aspose.Cells miễn phí
Bạn có thể nhận giấy phép tạm thời miễn phí và sử dụng Aspose.Cells for Python mà không có giới hạn đánh giá.
Sự kết luận
Trong bài viết này, bạn đã học cách xuất dữ liệu Excel sang Google Trang tính bằng Python. Chúng tôi đã trình bày cách tạo dự án trên Google Cloud, bật API Google Trang tính, đọc tệp Excel và xuất dữ liệu từ tệp Excel sang Google Trang tính. Để khám phá thêm về Aspose.Cells for Python, bạn có thể truy cập tài liệu. Ngoài ra, bạn có thể đặt câu hỏi của mình qua diễn đàn của chúng tôi.