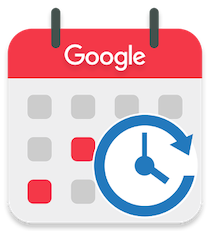
Lịch Google là dịch vụ lập lịch cho phép bạn tạo và theo dõi các sự kiện, chẳng hạn như cuộc họp. Bạn có thể ghi lại các sự kiện trên lịch và nhận lời nhắc về những sự kiện sắp tới. Google cũng cho phép bạn sử dụng dịch vụ lập lịch của mình theo chương trình. Do đó, bạn có thể quản lý các sự kiện của mình bằng Lịch Google từ trong các ứng dụng của mình. Trong bài viết này, bạn sẽ học cách tạo, cập nhật và xóa Lịch Google trong C#.
- API C# để tạo và cập nhật Lịch Google
- Tạo Lịch Google bằng C#
- Cập nhật Lịch Google trong C#
- Xóa Lịch Google trong C#
C# API để tạo và cập nhật Lịch Google - Tải xuống miễn phí
Để làm việc với dịch vụ Lịch Google, chúng tôi sẽ sử dụng Aspose.Email for .NET. Đây là một API mạnh mẽ cung cấp một loạt các tính năng để xử lý email, làm việc với các ứng dụng email và sử dụng các dịch vụ cộng tác của Google. Bạn có thể tải xuống DLL của API hoặc cài đặt nó từ NuGet bằng lệnh sau.
PM> Install-Package Aspose.Email
Trước khi bắt đầu làm việc, bạn cần tạo một dự án trên Bảng điều khiển dành cho nhà phát triển của Google, dự án này sẽ cho phép ứng dụng của bạn giao tiếp với các dịch vụ của Google. Để tạo một tài khoản, bạn có thể làm theo hướng dẫn này.
Để truy cập và thao tác với Lịch Google, chúng ta cần viết một số mã để xử lý thông tin của người dùng và thực hiện xác thực. Đối với người dùng Google, trước tiên chúng tôi sẽ tạo một lớp có tên TestUser và sau đó kế thừa nó từ lớp GoogleUser. Sau đây là cách thực hiện đầy đủ của cả hai lớp.
using System;
namespace Aspose.Email
{
internal enum GrantTypes
{
authorization_code,
refresh_token
}
public class TestUser
{
internal TestUser(string name, string eMail, string password, string domain)
{
Name = name;
EMail = eMail;
Password = password;
Domain = domain;
}
public readonly string Name;
public readonly string EMail;
public readonly string Password;
public readonly string Domain;
public static bool operator ==(TestUser x, TestUser y)
{
if ((object)x != null)
return x.Equals(y);
if ((object)y != null)
return y.Equals(x);
return true;
}
public static bool operator !=(TestUser x, TestUser y)
{
return !(x == y);
}
public static implicit operator string(TestUser user)
{
return user == null ? null : user.Name;
}
public override int GetHashCode()
{
return ToString().GetHashCode();
}
public override bool Equals(object obj)
{
return obj != null && obj is TestUser && this.ToString().Equals(obj.ToString(), StringComparison.OrdinalIgnoreCase);
}
public override string ToString()
{
return string.IsNullOrEmpty(Domain) ? Name : string.Format("{0}/{1}", Domain, Name);
}
}
public class GoogleUser : TestUser
{
public GoogleUser(string name, string eMail, string password)
: this(name, eMail, password, null, null, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret)
: this(name, eMail, password, clientId, clientSecret, null)
{ }
public GoogleUser(string name, string eMail, string password, string clientId, string clientSecret, string refreshToken)
: base(name, eMail, password, "gmail.com")
{
ClientId = clientId;
ClientSecret = clientSecret;
RefreshToken = refreshToken;
}
public readonly string ClientId;
public readonly string ClientSecret;
public readonly string RefreshToken;
}
}
Bây giờ, chúng ta cần tạo một lớp trợ giúp sẽ đảm nhận việc xác thực tài khoản Google. Chúng tôi sẽ đặt tên lớp này là GoogleOAuthHelper. Sau đây là cách thực hiện hoàn chỉnh của lớp này.
using System;
using System.Diagnostics;
using System.IO;
using System.Net;
using System.Text;
using System.Threading;
using System.Windows.Forms;
namespace Aspose.Email
{
internal class GoogleOAuthHelper
{
public const string TOKEN_REQUEST_URL = "https://accounts.google.com/o/oauth2/token";
public const string SCOPE = "https%3A%2F%2Fwww.googleapis.com%2Fauth%2Fcalendar" + "+" + "https%3A%2F%2Fwww.google.com%2Fm8%2Ffeeds%2F" + "+" + "https%3A%2F%2Fmail.google.com%2F"; // IMAP & SMTP
public const string REDIRECT_URI = "urn:ietf:wg:oauth:2.0:oob";
public const string REDIRECT_TYPE = "code";
internal static string GetAccessToken(TestUser user)
{
return GetAccessToken((GoogleUser)user);
}
internal static string GetAccessToken(GoogleUser user)
{
string access_token;
string token_type;
int expires_in;
GetAccessToken(user, out access_token, out token_type, out expires_in);
return access_token;
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string token_type, out int expires_in)
{
access_token = null;
token_type = null;
expires_in = 0;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&client_secret={1}&refresh_token={2}&grant_type={3}",
System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(user.RefreshToken), System.Web.HttpUtility.UrlEncode(GrantTypes.refresh_token.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
}
}
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", user.EMail));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token)
{
string token_type;
int expires_in;
GoogleOAuthHelper.GetAccessToken(user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(TestUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
GetAccessToken((GoogleUser)user, out access_token, out refresh_token, out token_type, out expires_in);
}
internal static void GetAccessToken(GoogleUser user, out string access_token, out string refresh_token, out string token_type, out int expires_in)
{
string authorizationCode = GoogleOAuthHelper.GetAuthorizationCode(user, GoogleOAuthHelper.SCOPE, GoogleOAuthHelper.REDIRECT_URI, GoogleOAuthHelper.REDIRECT_TYPE);
GoogleOAuthHelper.GetAccessToken(authorizationCode, user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, TestUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
GetAccessToken(authorizationCode, (GoogleUser)user, out access_token, out token_type, out expires_in, out refresh_token);
}
internal static void GetAccessToken(string authorizationCode, GoogleUser user, out string access_token, out string token_type, out int expires_in, out string refresh_token)
{
access_token = null;
token_type = null;
expires_in = 0;
refresh_token = null;
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(TOKEN_REQUEST_URL);
request.CookieContainer = new CookieContainer();
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
string encodedParameters = string.Format("client_id={0}&code={1}&client_secret={2}&redirect_uri={3}&grant_type={4}", System.Web.HttpUtility.UrlEncode(user.ClientId), System.Web.HttpUtility.UrlEncode(authorizationCode), System.Web.HttpUtility.UrlEncode(user.ClientSecret), System.Web.HttpUtility.UrlEncode(REDIRECT_URI), System.Web.HttpUtility.UrlEncode(GrantTypes.authorization_code.ToString()));
byte[] requestData = Encoding.UTF8.GetBytes(encodedParameters);
request.ContentLength = requestData.Length;
if (requestData.Length > 0)
using (Stream stream = request.GetRequestStream())
stream.Write(requestData, 0, requestData.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
string responseText = null;
using (TextReader reader = new StreamReader(response.GetResponseStream(), Encoding.ASCII))
responseText = reader.ReadToEnd();
foreach (string sPair in responseText.Replace("{", "").Replace("}", "").Replace("\"", "").Split(new string[] { ",\n" }, StringSplitOptions.None))
{
string[] pair = sPair.Split(':');
string name = pair[0].Trim().ToLower();
string value = System.Web.HttpUtility.UrlDecode(pair[1].Trim());
switch (name)
{
case "access_token":
access_token = value;
break;
case "token_type":
token_type = value;
break;
case "expires_in":
expires_in = Convert.ToInt32(value);
break;
case "refresh_token":
refresh_token = value;
break;
}
}
Debug.WriteLine(string.Format("Authorization code: '{0}'", authorizationCode));
Debug.WriteLine(string.Format("Access token: '{0}'", access_token));
Debug.WriteLine(string.Format("Refresh token: '{0}'", refresh_token));
Debug.WriteLine(string.Format("Token type: '{0}'", token_type));
Debug.WriteLine(string.Format("Expires in: '{0}'", expires_in));
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("");
}
internal static string GetAuthorizationCode(TestUser acc, string scope, string redirectUri, string responseType)
{
return GetAuthorizationCode((GoogleUser)acc, scope, redirectUri, responseType);
}
internal static string GetAuthorizationCode(GoogleUser acc, string scope, string redirectUri, string responseType)
{
Debug.WriteLine("");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine("-----------OAuth 2.0 authorization information-----------");
Debug.WriteLine("---------------------------------------------------------");
Debug.WriteLine(string.Format("Login: '{0}'", acc.EMail));
string authorizationCode = null;
string error = null;
string approveUrl = string.Format("https://accounts.google.com/o/oauth2/auth?redirect_uri={0}&response_type={1}&client_id={2}&scope={3}", redirectUri, responseType, acc.ClientId, scope);
AutoResetEvent are0 = new AutoResetEvent(false);
Thread t = new Thread(delegate()
{
bool doEvents = true;
WebBrowser browser = new WebBrowser();
browser.AllowNavigation = true;
browser.DocumentCompleted += delegate (object sender, WebBrowserDocumentCompletedEventArgs e) { doEvents = false; };
Form f = new Form();
f.FormBorderStyle = FormBorderStyle.FixedToolWindow;
f.ShowInTaskbar = false;
f.StartPosition = FormStartPosition.Manual;
f.Location = new System.Drawing.Point(-2000, -2000);
f.Size = new System.Drawing.Size(1, 1);
f.Controls.Add(browser);
f.Load += delegate (object sender, EventArgs e)
{
try
{
browser.Navigate("https://accounts.google.com/Logout");
doEvents = true;
while (doEvents) Application.DoEvents();
browser.Navigate("https://accounts.google.com/ServiceLogin?sacu=1");
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement loginForm = browser.Document.Forms["gaia_loginform"];
if (loginForm != null)
{
HtmlElement userName = browser.Document.All["Email"];
userName.SetAttribute("value", acc.EMail);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
loginForm = browser.Document.Forms["gaia_loginform"];
HtmlElement passwd = browser.Document.All["Passwd"];
passwd.SetAttribute("value", acc.Password);
loginForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Login form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
browser.Navigate(approveUrl);
doEvents = true;
while (doEvents) Application.DoEvents();
HtmlElement approveForm = browser.Document.Forms["connect-approve"];
if (approveForm != null)
{
HtmlElement submitAccess = browser.Document.All["submit_access"];
submitAccess.SetAttribute("value", "true");
approveForm.InvokeMember("submit");
doEvents = true;
while (doEvents)
Application.DoEvents();
}
else
{
error = "Approve form is not found in \n" + browser.Document.Body.InnerHtml;
return;
}
HtmlElement code = browser.Document.All["code"];
if (code != null)
authorizationCode = code.GetAttribute("value");
else
error = "Authorization code is not found in \n" + browser.Document.Body.InnerHtml;
}
catch (Exception ex)
{
error = ex.Message;
}
finally
{
f.Close();
}
};
Application.Run(f);
are0.Set();
});
t.SetApartmentState(ApartmentState.STA);
t.Start();
are0.WaitOne();
if (error != null)
throw new Exception(error);
return authorizationCode;
}
}
}
Tạo Lịch Google bằng C#
Khi bạn đã thực hiện xong cấu hình trên, bạn có thể tiếp tục làm việc với dịch vụ Lịch Google. Sau đây là các bước để tạo và cập nhật Lịch Google trong C#.
- Lấy một phiên bản của lớp GmailClient vào đối tượng IGmailClient bằng phương thức GmailClient.GetInstance (String, String).
- Tạo một thể hiện của lớp Lịch và khởi tạo nó với tên, mô tả và các thuộc tính khác.
- Gọi phương thức IGmailClient.CreateCalendar (Lịch) để tạo Lịch Google.
- Lấy ID trả lại của lịch.
Mẫu mã sau đây cho biết cách tạo Lịch Google trong C#.
// Khởi tạo người dùng Google
GoogleUser User = new GoogleUser("user", "email address", "password", "clientId", "client secret");
string accessToken;
string refreshToken;
// Nhận mã thông báo truy cập
GoogleOAuthHelper.GetAccessToken(User, out accessToken, out refreshToken);
// Nhận phiên bản của ứng dụng khách Gmail
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// Chèn và tải lịch
Aspose.Email.Clients.Google.Calendar calendar = new Aspose.Email.Clients.Google.Calendar("summary - " + Guid.NewGuid().ToString(), null, null, "America/Los_Angeles");
// Chèn lịch và truy xuất id
string id = client.CreateCalendar(calendar);
Aspose.Email.Clients.Google.Calendar cal = client.FetchCalendar(id);
}
C# Cập nhật Lịch Google
Sau đây là các bước để cập nhật Lịch Google theo chương trình trong C#.
- Lấy một phiên bản của lớp GmailClient vào đối tượng IGmailClient bằng phương thức GmailClient.GetInstance (String, String).
- Sử dụng phương thức IGmailClient.FetchCalendar (String) để tìm nạp phiên bản lịch bằng cách sử dụng ID của nó.
- Cập nhật các thuộc tính của lịch và gọi phương thức IGmailClient.UpdateCalendar (Lịch) để cập nhật lịch.
Mẫu mã sau đây cho biết cách cập nhật Lịch Google trong C#.
// Nhận phiên bản của ứng dụng khách Gmail
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
string id = "<<calendar ID>>";
// Tìm nạp lịch
Aspose.Email.Clients.Google.Calendar cal = client.FetchCalendar(id);
// Thay đổi thông tin trong lịch đã tìm nạp và cập nhật lịch
cal.Description = "Description - " + Guid.NewGuid().ToString();
cal.Location = "Location - " + Guid.NewGuid().ToString();
// Cập nhật lịch
client.UpdateCalendar(cal);
}
C# Xóa Lịch Google
Bạn cũng có thể xóa một lịch cụ thể bằng cách sử dụng Aspose.Email for .NET. Sau đây là các bước để thực hiện thao tác này.
- Lấy một phiên bản của lớp GmailClient vào đối tượng IGmailClient bằng phương thức GmailClient.GetInstance (String, String).
- Nhận danh sách lịch bằng phương thức IGmailClient.ListCalendars().
- Lặp lại danh sách và lọc danh sách mong muốn.
- Xóa lịch bằng phương pháp IGmailClient.DeleteCalendar (Calendar.Id).
Mẫu mã sau đây cho biết cách xóa Lịch Google trong C#.
// Nhận phiên bản của ứng dụng khách Gmail
using (IGmailClient client = GmailClient.GetInstance(accessToken, User.EMail))
{
// Truy cập và xóa lịch có tóm tắt bắt đầu từ "Tóm tắt lịch -"
string summary = "Calendar summary - ";
// Nhận danh sách lịch
ExtendedCalendar[] lst0 = client.ListCalendars();
foreach (ExtendedCalendar extCal in lst0)
{
// Xóa các lịch đã chọn
if (extCal.Summary.StartsWith(summary))
client.DeleteCalendar(extCal.Id);
}
}
C# API cho Lịch Google - Nhận Giấy phép Miễn phí
Bạn có thể nhận giấy phép tạm thời miễn phí để sử dụng Aspose.Email for .NET mà không có giới hạn đánh giá.
Sự kết luận
Trong bài viết này, bạn đã học cách tạo Lịch Google theo chương trình trong C#. Hơn nữa, bạn đã thấy cách cập nhật và xóa một Lịch Google cụ thể trong C#. Ngoài ra, bạn có thể khám phá tài liệu để đọc thêm về Aspose.Email for .NET. Ngoài ra, bạn có thể đặt câu hỏi của mình qua diễn đàn của chúng tôi.