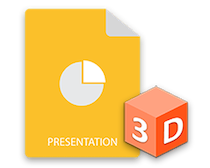
Hiệu ứng 3D trong PowerPoint được sử dụng để làm cho bài thuyết trình hấp dẫn hơn và thu hút sự chú ý của người dùng Do đó, bạn có thể bắt gặp yêu cầu thêm các đối tượng 3D vào bài thuyết trình theo chương trình. Trong bài viết này, bạn sẽ học cách tạo hiệu ứng 3D trong PowerPoint PPT hoặc PPTX trong Java. Chúng tôi sẽ hướng dẫn bạn cách tạo văn bản và hình dạng 3D và áp dụng hiệu ứng 3D cho hình ảnh.
- Java API để tạo hiệu ứng 3D trong PowerPoint
- Tạo văn bản 3D trong PowerPoint trong Java
- Tạo Hình dạng 3D trong PowerPoint trong Java
- Đặt Gradient cho Hình dạng 3D
- Áp dụng hiệu ứng 3D cho hình ảnh trong PowerPoint
API Java để áp dụng hiệu ứng 3D trong PowerPoint PPT
Aspose.Slides dành cho Java là một API mạnh mẽ bao gồm một loạt các tính năng thao tác trình bày. Sử dụng API, bạn có thể tạo các bản trình bày tương tác và thao tác liền mạch với các tệp PPT / PPTX hiện có. Để tạo hiệu ứng 3D trong bản trình bày PowerPoint, chúng tôi sẽ sử dụng API này.
Bạn có thể tải xuống API của JAR hoặc cài đặt nó bằng cách sử dụng các cấu hình Maven sau.
Kho:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
Sự phụ thuộc:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-slides</artifactId>
<version>22.1</version>
<classifier>jdk16</classifier>
</dependency>
Tạo văn bản 3D trong PowerPoint trong Java
Sau đây là các bước để tạo một đoạn văn bản 3D trong PowerPoint PPT bằng Java.
- Đầu tiên, tạo một PPT mới hoặc tải một PPT hiện có bằng cách sử dụng lớp Trình bày.
- Sau đó, thêm một hình chữ nhật mới bằng phương thức addAutoShape().
- Đặt các thuộc tính của hình dạng như kiểu tô, văn bản, v.v.
- Nhận tham chiếu của văn bản bên trong hình dạng thành một đối tượng Portion.
- Áp dụng định dạng cho phần văn bản.
- Tham khảo hình dạng bên trong TextFrame.
- Áp dụng hiệu ứng 3D bằng cách sử dụng IThreeDFormat được trả về bởi phương thức TextFrame.getTextFrameFormat(). GetThreeDFormat().
- Cuối cùng, lưu bản trình bày bằng phương thức Presentation.save (String, SaveFormat).
Mẫu mã sau đây cho thấy cách tạo văn bản 3D trong PowerPoint PPT bằng Java.
// Tạo bản trình bày
Presentation pres = new Presentation();
try {
// Thêm hình chữ nhật
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// Đặt văn bản
shape.getFillFormat().setFillType(FillType.NoFill);
shape.getLineFormat().getFillFormat().setFillType(FillType.NoFill);
shape.getTextFrame().setText("3D Text");
// Thêm phần văn bản và đặt thuộc tính của nó
Portion portion = (Portion)shape.getTextFrame().getParagraphs().get_Item(0).getPortions().get_Item(0);
portion.getPortionFormat().getFillFormat().setFillType(FillType.Pattern);
portion.getPortionFormat().getFillFormat().getPatternFormat().getForeColor().setColor(new Color(255, 140, 0));
portion.getPortionFormat().getFillFormat().getPatternFormat().getBackColor().setColor(Color.WHITE);
portion.getPortionFormat().getFillFormat().getPatternFormat().setPatternStyle(PatternStyle.LargeGrid);
// Đặt kích thước phông chữ cho văn bản của hình dạng
shape.getTextFrame().getParagraphs().get_Item(0).getParagraphFormat().getDefaultPortionFormat().setFontHeight(128);
// Nhận khung văn bản
ITextFrame textFrame = shape.getTextFrame();
// Thiết lập hiệu ứng chuyển đổi WordArt "Arch Up"
textFrame.getTextFrameFormat().setTransform(TextShapeType.ArchUp);
// Áp dụng hiệu ứng 3D
textFrame.getTextFrameFormat().getThreeDFormat().setExtrusionHeight(3.5f);
textFrame.getTextFrameFormat().getThreeDFormat().setDepth(3);
textFrame.getTextFrameFormat().getThreeDFormat().setMaterial(MaterialPresetType.Plastic);
textFrame.getTextFrameFormat().getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
textFrame.getTextFrameFormat().getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Balanced);
textFrame.getTextFrameFormat().getThreeDFormat().getLightRig().setRotation(0, 0, 40);
textFrame.getTextFrameFormat().getThreeDFormat().getCamera().setCameraType(CameraPresetType.PerspectiveContrastingRightFacing);
// Lưu bản trình bày
pres.save("3D-Text.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
Ảnh chụp màn hình sau đây cho thấy đầu ra của mẫu mã ở trên.
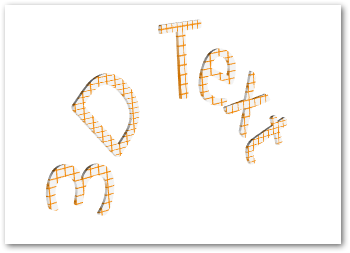
Tạo Hình dạng 3D trong PowerPoint trong Java
Tương tự như văn bản, bạn có thể áp dụng hiệu ứng 3D cho các hình dạng trong bản trình bày PowerPoint. Sau đây là các bước để tạo hình dạng 3D trong PowerPoint PPT bằng Java.
- Đầu tiên, tạo một PPT mới bằng cách sử dụng lớp Trình bày.
- Thêm một hình dạng hình chữ nhật mới bằng cách sử dụng phương thức addAutoShape().
- Đặt văn bản của hình dạng bằng phương pháp IAutoShape.getTextFrame.setText().
- Áp dụng hiệu ứng 3D cho hình dạng bằng cách sử dụng IThreeDFormat được trả về bởi phương thức IAutoShape.getThreeDFormat().
- Cuối cùng, lưu bản trình bày bằng phương thức Presentation.save (String, SaveFormat).
Mẫu mã sau đây cho thấy cách áp dụng hiệu ứng 3D cho các hình dạng trong PowerPoint bằng Java.
// Tạo bản trình bày
Presentation pres = new Presentation();
try {
// Thêm hình chữ nhật
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// Đặt văn bản cho hình dạng
shape.getTextFrame().setText("3D");
shape.getTextFrame().getParagraphs().get_Item(0).getParagraphFormat().getDefaultPortionFormat().setFontHeight(64);
// Áp dụng hiệu ứng 3D
shape.getThreeDFormat().getCamera().setCameraType(CameraPresetType.OrthographicFront);
shape.getThreeDFormat().getCamera().setRotation(20, 30, 40);
shape.getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Flat);
shape.getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
shape.getThreeDFormat().setMaterial(MaterialPresetType.Flat);
shape.getThreeDFormat().setExtrusionHeight(100);
shape.getThreeDFormat().getExtrusionColor().setColor(Color.BLUE);
// Lưu bản trình bày
pres.save("3D-Shape.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
Sau đây là hình dạng 3D mà chúng ta nhận được sau khi thực hiện đoạn mã này.
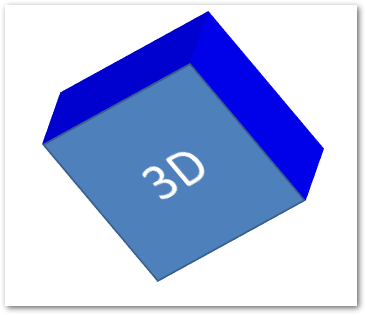
Tạo Gradient cho Hình dạng 3D
Bạn cũng có thể áp dụng hiệu ứng gradient cho các hình dạng theo các bước bên dưới.
- Đầu tiên, tạo một PPT mới bằng cách sử dụng lớp Trình bày.
- Thêm một hình dạng hình chữ nhật mới bằng cách sử dụng phương thức addAutoShape().
- Đặt văn bản của hình dạng bằng thuộc tính IAutoShape.getTextFrame(). SetText().
- Đặt kiểu tô của hình dạng thành FillType.Gradient và đặt màu gradient.
- Áp dụng hiệu ứng 3D cho hình dạng bằng cách sử dụng IThreeDFormat được trả về bởi phương thức IAutoShape.getThreeDFormat().
- Cuối cùng, lưu bản trình bày bằng phương thức Presentation.save (String, SaveFormat).
Mẫu mã sau đây cho thấy cách áp dụng hiệu ứng gradient cho hình dạng trong PowerPoint PPT.
// Tạo bản trình bày
Presentation pres = new Presentation();
try {
// Thêm hình chữ nhật
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// Đặt văn bản cho hình dạng
shape.getTextFrame().setText("3D");
shape.getTextFrame().getParagraphs().get_Item(0).getParagraphFormat().getDefaultPortionFormat().setFontHeight(64);
shape.getFillFormat().setFillType(FillType.Gradient);
shape.getFillFormat().getGradientFormat().getGradientStops().add(0, Color.BLUE);
shape.getFillFormat().getGradientFormat().getGradientStops().add(100, Color.MAGENTA);
// Áp dụng hiệu ứng 3D
shape.getThreeDFormat().getCamera().setCameraType(CameraPresetType.OrthographicFront);
shape.getThreeDFormat().getCamera().setRotation(10, 20, 30);
shape.getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Flat);
shape.getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
shape.getThreeDFormat().setExtrusionHeight(150);
shape.getThreeDFormat().getExtrusionColor().setColor(new Color(255, 140, 0));
// Lưu bản trình bày
pres.save("3D-Shape-Gradient.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
Sau đây là hình dạng 3D sau khi áp dụng hiệu ứng gradient.
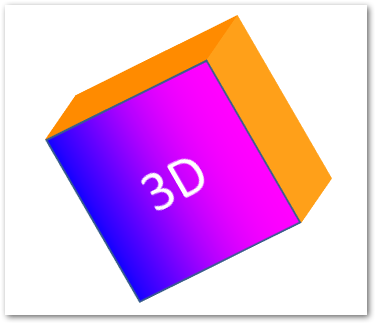
Áp dụng hiệu ứng 3D cho hình ảnh trong PowerPoint trong Java
Aspose.Slides for Java cũng cho phép bạn áp dụng các hiệu ứng 3D cho hình ảnh. Sau đây là các bước để thực hiện thao tác này trong Java.
- Tạo một PPT mới bằng cách sử dụng lớp Trình bày.
- Thêm một hình dạng hình chữ nhật mới bằng cách sử dụng phương thức addAutoShape().
- Đặt kiểu tô của hình dạng thành FillType.Picture và thêm hình ảnh.
- Áp dụng hiệu ứng 3D cho hình dạng bằng cách sử dụng IThreeDFormat được trả về bởi phương thức IAutoShape.getThreeDFormat().
- Lưu bản trình bày bằng phương thức Presentation.save (String, SaveFormat).
Sau đây là các bước để áp dụng hiệu ứng 3D cho hình ảnh trong PPT bằng Java.
// Tạo bản trình bày
Presentation pres = new Presentation();
try {
// Thêm hình chữ nhật
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// Đặt hình ảnh cho hình dạng
shape.getFillFormat().setFillType(FillType.Picture);
IPPImage picture = null;
try {
picture = pres.getImages().addImage(Files.readAllBytes(Paths.get("tiger.bmp")));
} catch (IOException e) { }
shape.getFillFormat().getPictureFillFormat().getPicture().setImage(picture);
shape.getFillFormat().getPictureFillFormat().setPictureFillMode(PictureFillMode.Stretch);
// Áp dụng hiệu ứng 3D
shape.getThreeDFormat().getCamera().setCameraType(CameraPresetType.OrthographicFront);
shape.getThreeDFormat().getCamera().setRotation(10, 20, 30);
shape.getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Flat);
shape.getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
shape.getThreeDFormat().setExtrusionHeight(150);
shape.getThreeDFormat().getExtrusionColor().setColor(Color.GRAY);
// Lưu bản trình bày
pres.save("3D-Image.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
Sau đây là hình ảnh kết quả mà chúng tôi nhận được sau khi áp dụng hiệu ứng 3D.
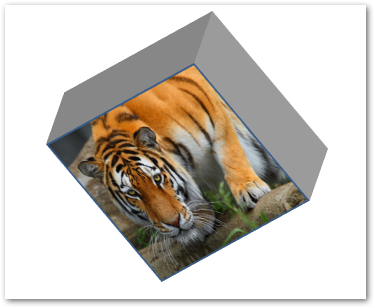
Nhận giấy phép miễn phí
Bạn có thể nhận giấy phép tạm thời miễn phí để sử dụng Aspose.Slides for Java mà không có giới hạn đánh giá.
Sự kết luận
Trong bài này, bạn đã học cách áp dụng hiệu ứng 3D trong PowerPoint PPT / PPTX bằng Java. Với sự trợ giúp của các mẫu mã, chúng tôi đã trình bày cách tạo văn bản hoặc hình dạng 3D và áp dụng hiệu ứng 3D cho hình ảnh trong bản trình bày PPT hoặc PPTX. Bạn có thể truy cập tài liệu để khám phá thêm về Aspose.Slides for Java. Ngoài ra, bạn có thể đăng câu hỏi hoặc thắc mắc của mình lên diễn đàn của chúng tôi.