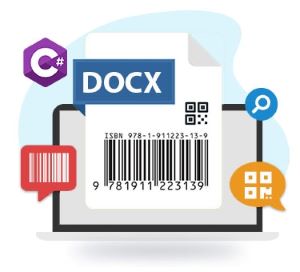
Barcodes are images in the form of parallel lines, dots, or rectangles with encoded data/information. The industry professionals embed and access product information, track product movement, and keep up with inventory using barcodes. In certain cases, we may need to generate and add barcodes in MS Word documents. MS Word is the most popular and widely used graphical word processing program. It is used to create new documents with text, images, or graphics, write professional-quality write-ups, edit, and format the existing documents, etc. The DOCX and DOC are the popular file formats supported by MS Word. In this article, we will learn how to create a barcode in Word documents programmatically using C#.
The following topics shall be covered in this article:
- C# API to Create Barcode in Word Documents
- Generate and Add Barcode to Word Document
- Add Barcode to Existing Word Document
- Add QR Code to Word Document
- Read Barcode from Word Document
C# API to Create Barcode in Word Documents
For generating a barcode and adding a barcode image to Word documents, we will follow a two-step procedure. Firstly, we will be using the Aspose.Words for .NET API to create or load a Word document, then we will generate and add the barcode image to the document using the Aspose.BarCode for .NET API. The Document class of the Aspose.Words for .NET API allows creating a new Word document or loading an existing Word file in the application. The Save() method of this class saves the document on the given file path. The DocumentBuilder class of the API provides methods for building a document. It provides various overloaded InsertImage() methods to insert an image in the document.
The Aspose.BarCode for .NET API allows generating various types of supported barcodes. For this purpose, it provides the BarcodeGenerator class to generate the barcode of the specified EncodeType. We can save the generated barcode image using the Save() method of this class. The API also provides the BarCodeImageFormat enumeration to specify the save formats. We can read the barcode from images using the BarCodeReader class of the API.
Please either download the DLLs of the APIs or install them using NuGet.
PM> Install-Package Aspose.BarCode
PM> Install-Package Aspose.Words
Generate and Add Barcode to Word Document in C#
We can create a new Word document and add a barcode image to the document by following the steps given below:
- Firstly, create an instance of the BarcodeGenerator class. It takes the EncodeType and text to encode as arguments.
- Next, create an instance of the memory stream object.
- Then, call the Save() method to save the barcode image to the memory stream.
- Next, create an instance of the Document class.
- Then, initialize an instance of the DocumentBuilder class with the Document object.
- After that, insert the barcode image using the InsertImage() method with the stream object as an argument.
- Finally, call the Save() method. It takes the output DOCX file path as an argument.
The following code example shows how to generate and add a barcode to a new Word document using C#.

Generate and add Barcode to a new Word document in C#.
Add Barcode to Existing Word Document in C#
We can generate and add a barcode image to any existing Word document by following the steps given below:
- Firstly, create an instance of the BarcodeGenerator class with the EncodeType and text to encode as arguments.
- Next, create an instance of the memory stream object.
- Then, call the Save() method to save the barcode image to the memory stream.
- Next, load an existing Word document using the Document class.
- Then, initialize an instance of the DocumentBuilder class with the Document object.
- After that, insert the barcode image using the InsertImage() method with the stream object and its position as arguments.
- Finally, call the Save() method. It takes the output DOCX file path as an argument.
The following code example shows how to generate and add a barcode to an existing Word document using C#.
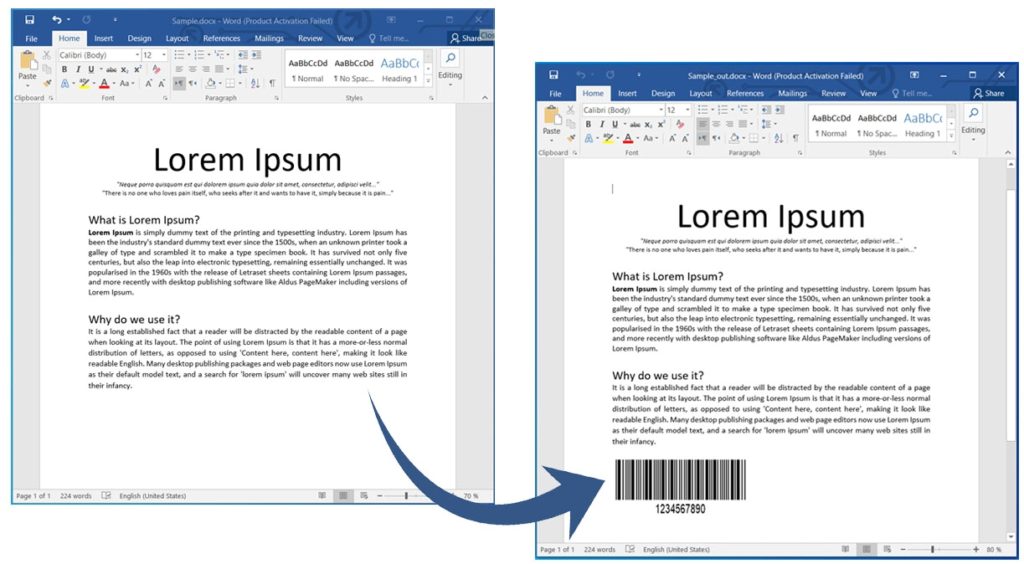
Add Barcode to Existing Word Document in C#.
Add QR Code to Word Document using C#
Similarly, we can also generate a QR code image and add it to the Word document by following the steps mentioned earlier. However, we just need to set the EncodeType as QR or GS1QR in the first step. We may also need to adjust the image position in step 6.
The following code example demonstrates how to add a QR code to a Word document using C#.
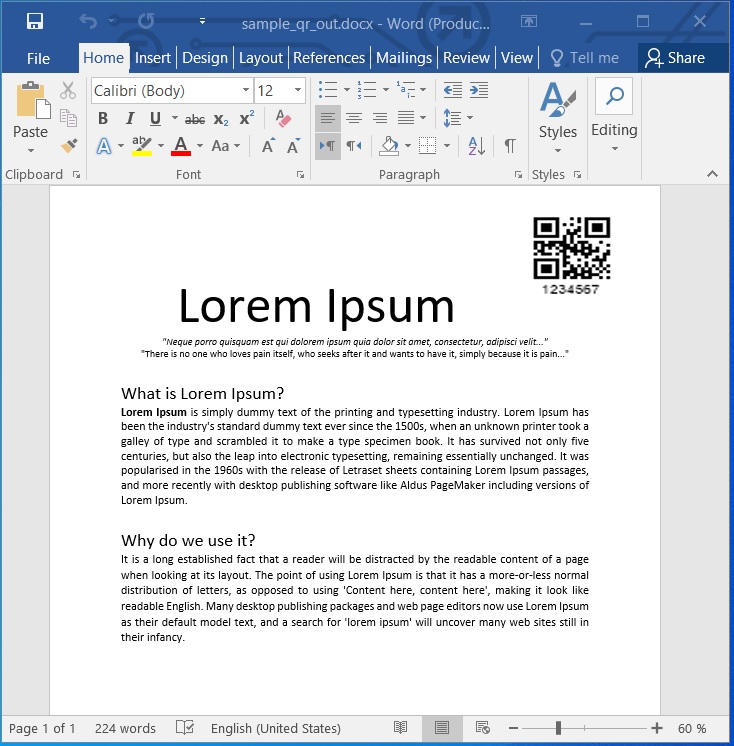
Add QR Code to Word Document using C#.
Read Barcode from Word Document using C#
We can recognize any barcode image available on any page of the Word document by following the steps given below:
- Firstly, load an existing Word document using the Document class.
- Next, access the NodeCollection of Shape types using the GetChildNodes() method.
- Then, loop through all the shapes and check if the shape is an image.
- Next, save the image to the stream.
- Then, create an instance of the BarCodeReader class with image stream and DecodeType as arguments.
- After that, call the ReadBarCodes() method to get the BarCodeResult object.
- Finally, show the barcode information.
The following code example shows how to read a barcode image from a Word document using C#.
Codetext found: 1234567890, Symbology: Code39Standard
Get a Free License
You can get a free temporary license to try the library without evaluation limitations.
Conclusion
In this article, we have learned how to:
- create a Word document programmatically;
- generate a barcode image and add it to the Word document;
- create a QR code and insert it into a Word document;
- read a barcode image from a Word document in C#.
Besides, you can learn more about Aspose.BarCode for .NET API using the documentation. In case of any ambiguity, please feel free to contact us on the forum.