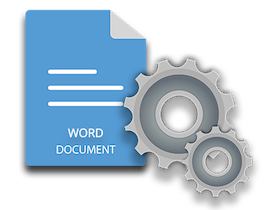
MS Word files are immensely used to create various types of documents such as invoices, reports, technical articles, etc. Document automation has facilitated users to generate Word documents dynamically from within their web or desktop portals. Therefore, in this article, we will cover how to generate Word documents in Python without MS Office. Moreover, you will learn how to create a DOCX or DOC file and add text or other elements into it dynamically using Python.
- Python Library to Create Word Documents
- Create a Word DOCX/DOC File in Python
- Load an Existing Word Document
- Insert Paragraph in a Word Document
- Add Table in a Word Document
- Add List in a Word DOCX/DOC File
- Insert Image in a Word Document
- Add Table of Contents in a Word File
Python Library to Create Word Documents
To create Word documents dynamically, we will use Aspose.Words for Python. It is a powerful Python library that lets you create and manipulate MS Word documents seamlessly. You can install the library in your Python application from PyPI using the following pip command.
pip install aspose-words
Create a Word DOCX or DOC File in Python
The following are the steps to create a Word document from scratch in Python.
- Create an object of Document class.
- Create an object of DocumentBuilder class.
- Insert text into document using DocumentBuilder.write() method.
- Save the Word document using Document.save() method.
Python Code to Create a Word Document
The following code sample shows how to create a Word DOCX document.
Output
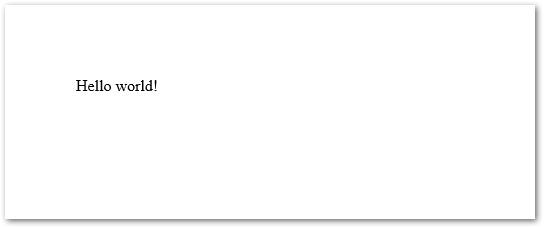
How to Load a Word Document in Python
You can also load an existing Word document by providing its path to the Document constructor. The following code sample shows how to load a Word document.
Python Create a Word Document - Insert Paragraph
Word documents contain text in the form of paragraphs. Therefore, in this section, we will demonstrate how to insert a paragraph into a Word document using Python.
- Create an object of Document class.
- Create an object of DocumentBuilder class.
- Get a reference of font from the Documentbuilder object and set font.
- Get the reference of the paragraph format from Documentbuilder object and set indentation, alignment, etc.
- Insert text into paragraph using DocumentBuilder.write() method.
- Save the Word document using Document.save() method.
The following code sample shows how to insert a paragraph in a Word document using Python.
Output
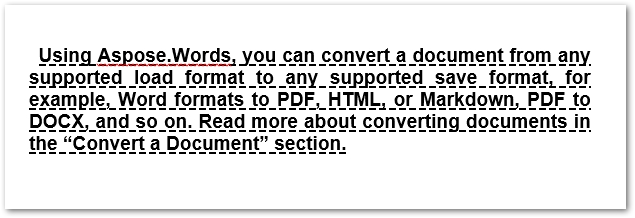
Learn more about working with paragraphs in Word documents using Python.
Generate Table in a Word Document in Python
Tables are an integral part of documents to display information in the form of rows and columns. Aspose.Words for Python makes it quite easier to work with tables. The following are the steps to add a table in a Word document using Python.
- Create an object of Document class.
- Create an object of DocumentBuilder class.
- Start a table using DocumentBuilder.start_table() method and get reference of the table in an object.
- Insert a cell using DocumentBuilder.insert_cell() method.
- Set auto fit using auto_fit(aw.tables.AutoFitBehavior.FIXED_COLUMN_WIDTHS) method.
- Set alignment of the cell.
- Insert text into cell using DocumentBuilder.write() method.
- Repeat inserting cells and text into cells as required.
- End a row when you complete inserting cells.
- End table when you have inserted all the rows.
- Save the Word document using Document.save() method.
The following code sample shows how to insert a table in a Word document using Python.
Output
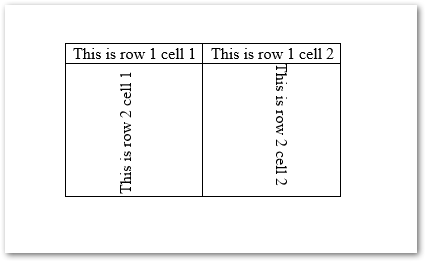
Learn more about how to work with tables in Word documents using Python.
Python: Generate a List in Word Document
The following are the steps to create a list in a Word document using Python.
- Create an object of Document class.
- Create an object of DocumentBuilder class.
- Set formatting using DocumentBuilder.list_format.apply_number_default() method.
- Insert item using DocumentBuilder.writeln(“Item 1”) method.
- Insert the second item using DocumentBuilder.writeln(“Item 2”) method.
- To insert items into next level of the list, call DocumentBuilder.list_format.list_indent() method and insert items.
- Remove numbers from the list using DocumentBuilder.list_format.remove_numbers() method.
- Save the Word document using Document.save() method.
The following code sample shows how to create a list in Word documents using Python.
Output

Read more about working with lists in Word documents using Python.
Insert Images in a Word Document in Python
While working with Word documents, you cannot ignore graphical objects such as images. So let’s have a look at how to insert images in a Word document dynamically using Python.
- Create an object of Document class.
- Create an object of DocumentBuilder class.
- Insert image using DocumentBuilder.insert_image() method and pass image file’s path as parameter.
- Save the Word document using Document.save() method.
The following code sample shows how to insert an image in a Word document using Python.
Output
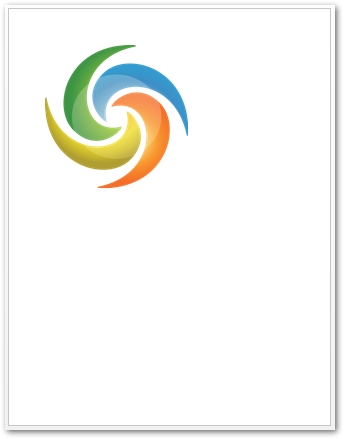
Create Table of Contents in Word Documents in Python
In various cases, Word documents contain a table of content (TOC). The TOC gives you an overview of the content of the Word document. The following steps demonstrate how to add a TOC in Word documents using Python.
- Create an object of Document class.
- Create an object of DocumentBuilder class.
- Insert table of contents using DocumentBuilder.insert_table_of_contents() method.
- Insert a page break after TOC using DocumentBuilder.insert_break(aw.BreakType.PAGE_BREAK) method.
- After adding/updating content of the document, update TOC using Document.update_fields() method.
- Save the Word document using Document.save() method.
The following code sample shows how to insert a table of contents in a Word document using Python.
Output
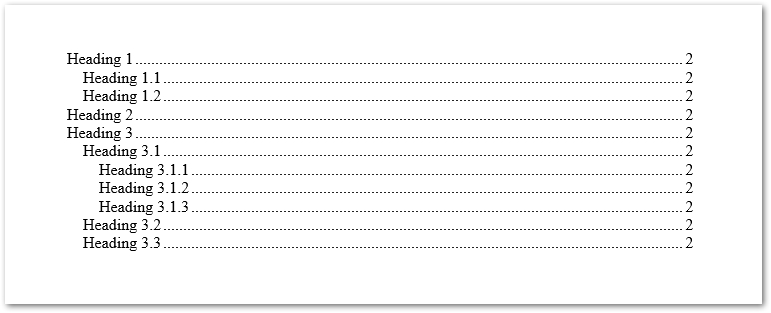
Read more about working with the table of contents using Python.
Create Word Documents with a Free License
You can get a free temporary license to generate MS Word documents without evaluation limitations.
Python Word Automation Library - Read More
This article covered some basic operations of creating Word documents and inserting different elements. Aspose.Words for Python provides a bunch of other features as well that you can explore using the documentation.
Conclusion
In this article, you have learned how to create Word DOCX or DOC files in Python. Moreover, you have seen how to insert text, images, tables, lists, and table of contents in Word documents dynamically. You can try the API and share your feedback or queries via our forum.