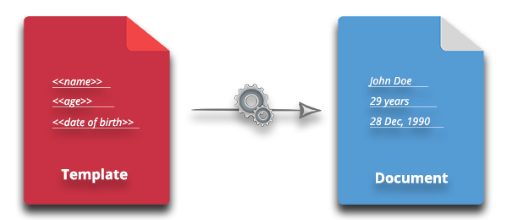
The report generation process often consists of populating the predefined document templates containing the placeholders for desired fields. The reporting engines take the template document as input, populate the placeholders with the data dynamically and generate the resultant reports. If you are searching for a Java program to generate Word documents from templates, this article is going to give you the best solution. So let’s see how to create Word documents by populating the templates programmatically in Java.
Java Library to Generate Word Documents from Templates
To generate Word documents from DOCX templates, we’ll use the LINQ Reporting Engine offered by Aspose.Words for Java. LINQ Reporting Engine supports a variety of tags for text, images, lists, tables, hyperlinks, and bookmarks for Word templates. The template documents containing these tags are populated by the engine with data coming from Java objects as well as XML, JSON, or CSV data sources. So let’s begin generating the Word documents from the templates using Java.
This article will cover how to generate a Word document from a template using:
- values from the Java objects,
- XML data source,
- JSON data source,
- and CSV data source.
Installing Aspose.Words for Java
You can download Aspose.Words for Java JAR or add it to your Maven-based application using the following configurations.
Repository:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
Dependency:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-words</artifactId>
<version>22.11</version>
<type>pom</type>
</dependency>
Generate Word Documents from Template using Java Objects
To begin the report generation, let’s first create a Word document by populating the template with values from the Java objects. To define a document template, create a new Word document, insert the following tags and save it as a DOCX document.
<<[s.getName()]>> says: "<<[s.getMessage()]>>."
In the above template, “s” will be treated as an object of Java class that will be used to populate the tags. So let’s create a class named Sender with two data members.
Now, it is time to pass the Word template to the LINQ Reporting Engine and generate the Word document based on the values of Sender’s object. The following are the steps for generating the Word document:
- Create the Document object and initialize it with the template’s path.
- Create and initialize an object of Sender class.
- Create an object of ReportingEngine class.
- Build the report using ReportingEngine.buildReport() method that accepts the document template, the data source, and the name of the data source.
- Save generated Word document using Document.save() method.
The following code sample shows how to generate a Word document from a Word template using the values of the Java object.
Output
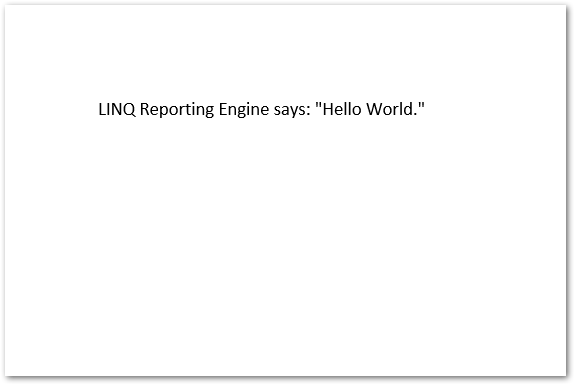
Java: Create Word Documents from Template using XML Data
Let’s now take one step further and see how to populate the Word templates using an XML data source in a bit complex scenario. The following is the XML data source that we’ll use to populate the Word template.
In this scenario, we’ll use the following tags in the template document for multiple records in the XML data source.
<<foreach [in persons]>>Name: <<[Name]>>, Age: <<[Age]>>, Date of Birth: <<[Birth]:"dd.MM.yyyy">>
<</foreach>>
Average age: <<[persons.average(p => p.Age)]>>
The Java code for generating Word document, in this case, would be the same and except for passing Java object as the data source, we’ll pass the XmlDataSource object in ReportingEngine.buildReport() method. The following code sample shows how to create a Word document by populating the document template using XML data source in Java.
Output
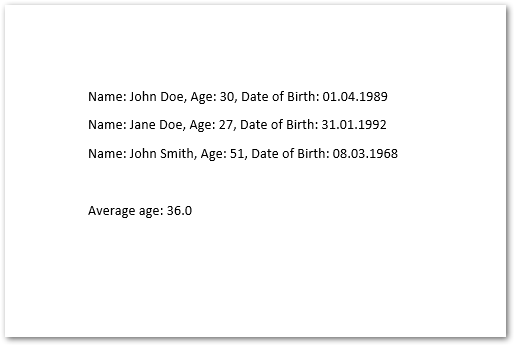
Java: Generate Word Documents from Template using JSON Data
Let’s now have a look at how to generate Word document from the DOCX template using a JSON data source. Following is the JSON data that we’ll use in this example.
In this example, we’ll generate the Word document containing a list of the clients grouped by their managers. Following this scenario, the DOCX template will look like the following:
<<foreach [in managers]>>Manager: <<[Name]>>
Contracts:
<<foreach [in Contract]>>- <<[Client.Name]>> ($<<[Price]>>)
<</foreach>>
<</foreach>>
For loading JSON data source, Aspose.Words provides JsonDataSource class. The following code sample shows how to create a Word document from the template using a JSON data source in Java.
Output
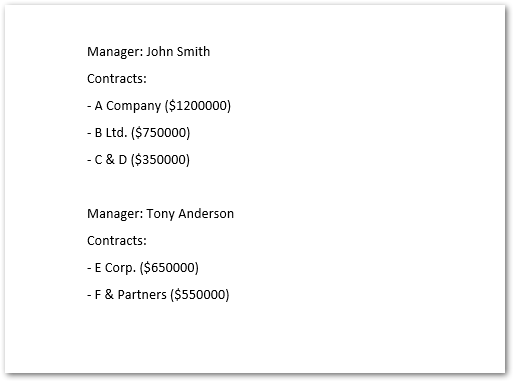
Generate Word Documents with CSV Data in Java
Last but not least, let’s check out how to generate the Word document by populating the Word template using the following CSV data source.
The following Word template will be used in this example:
<<foreach [in persons]>>Name: <<[Column1]>>, Age: <<[Column2]>>, Date of Birth: <<[Column3]:"dd.MM.yyyy">>
<</foreach>>
Average age: <<[persons.average(p => p.Column2)]>>
For dealing with CSV data sources, Aspose.Words offers CsvDataSource class. The following code sample shows how to generate a Word document using a CSV data source in Java.
Output

Generate Word Documents with a Free License
You can get a free temporary license and generate Word documents without evaluation limitations.
Java LINQ Reporting Engine - Read More
LINQ Reporting Engine supports a wide range of tags for generating fully-featured Word documents dynamically in Java. You can explore more about these tags along with their syntax in this article.
In case you would have any questions or queries, you can post to Aspose.Words Forum.