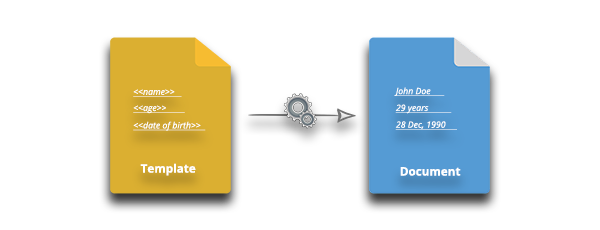
The automated generation of Word documents is widely used by enterprises for creating a multitude of reports. In some cases, the documents are created from scratch. On the other hand, predefined templates are used to generate Word documents by populating the placeholders. In this article, I’ll demonstrate how to generate Word documents from templates dynamically and programmatically in C#. You’ll come to know how to populate the Word templates from different types of data sources.
The following scenarios along with the code samples will be covered in this article:
- C# Word Automation API
- Generate Word document from a template using C# object’s values
- Generate Word document using an XML data source
- Create Word document using a JSON data source
- Generate Word document using a CSV data source
C# Word Automation API
We’ll use Aspose.Words for .NET - A Word automation API that allows you to generate Word document from scratch or by populating the predefined Word templates. You can either download API’s binaries or install it using one of the following methods.
Using NuGet Package Manager
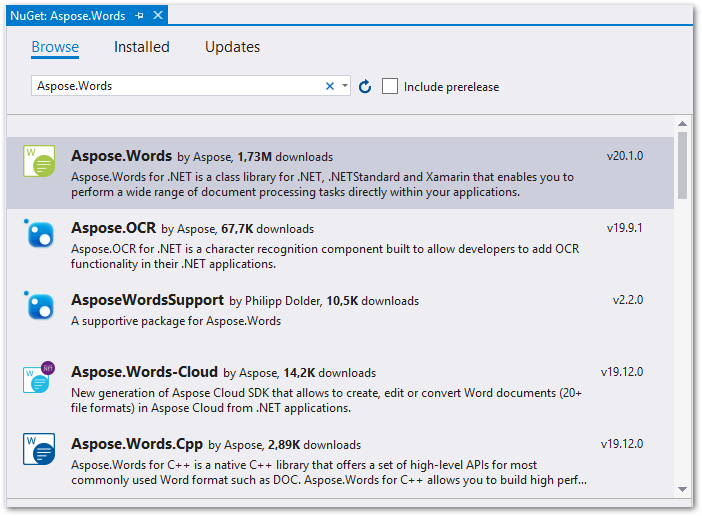
Using the Package Manager Console
PM> Install-Package Aspose.Words
Generate Word Document from Template using C# Objects
First of all, let’s see how to populate the Word template using the C# objects. For this, we’ll create a Word document (DOC/DOCX) with the following placeholders as the content of the document:
<<[sender.Name]>> says: "<<[sender.Message]>>."
Here, the sender is the object of the following class that we’ll use to populate the template.
Now, we’ll use the reporting engine of Aspose.Words to generate the Word document from the template and the object of Sender class by following the below steps.
- Create an object of the Document class and initialize it with the Word template’s path.
- Create and initialize an object of Sender class.
- Instantiate ReportingEngine class.
- Populate the templates using ReportingEngine.BuildReport() that takes the Document’s object, data source and the name of the data source as parameters.
- Save generated Word document using Document.Save() method.
The following code sample shows how to generate a Word document from template in C#.
Output

Generate Word Document from an XML Data Source in C#
For generating the Word document from an XML data source, we’ll use a bit more complex Word template with the following placeholders:
<<foreach [in persons]>>Name: <<[Name]>>, Age: <<[Age]>>, Date of Birth: <<[Birth]:"dd.MM.yyyy">>
<</foreach>>
Average age: <<[persons.Average(p => p.Age)]>>
The XML data source I have used in this example is given below.
The following are the steps to generate a Word document from an XML data source:
- Create an instance of the Document class and initialize it with the Word template’s path.
- Create an instance of the XmlDataSource class and initialize it with the XML file’s path.
- Instantiate ReportingEngine class.
- Use ReportingEngine.BuildReport() method in the same way we have used before to populate the Word template.
- Save the generated Word document using Document.Save() method.
The following code sample shows how to generate a Word document from an XML data source in C#.
Output

Generate Word Document from a JSON Data Source in C#
Let’s now see how to generate a Word document using a JSON data source. In this example, we’ll generate a list of the clients that are grouped by their managers. The following would be the Word template in this case:
<<foreach [in managers]>>Manager: <<[Name]>>
Contracts:
<<foreach [in Contract]>>- <<[Client.Name]>> ($<<[Price]>>)
<</foreach>>
<</foreach>>
The following would be the JSON data source that we’ll use to populate the template:
For generating the Word document from JSON, we’ll use the JsonDataSource class for loading and using the JSON data source and the rest of the steps will remain the same. The following code sample shows how to generate a Word document from the template using JSON in C#.
Output

Generate Word Document from CSV Data Source in C#
For generating the Word document from CSV, we’ll use the following Word template:
<<foreach [in persons]>>Name: <<[Column1]>>, Age: <<[Column2]>>, Date of Birth: <<[Column3]:"dd.MM.yyyy">>
<</foreach>>
Average age: <<[persons.Average(p => p.Column2)]>>
The template will be populated with the following CSV data:
Now, lets come to the C# code. All the steps will remain the same here as well except for the one change that is we’ll use the CsvDataSource class to load the CSV data. The following code sample shows how to generate the Word document from a CSV data source.
Output

Try Aspose.Words for .NET for Free
You can try Aspose.Words for .NET using a free temporary license.
Conclusion
In this article, you have learned how to generate Word documents from templates using C#. Furthermore, you have seen how to use objects, XML, JSON and CSV data sources to generate Word documents. You can explore more about the C# Word automation API using documentation. In addition, you can contact us via our forum.