This post covers how to perform Mail Merge operations in MS Word documents using Java. By the end of this article, you will learn how to create Mail Merge templates and execute Mail Merge programmatically.
- About Mail Merge
- Java Mail Merge API
- Data Sources for Mail Merge
- Create a Mail Merge Template in MS Word
- Create a Mail Merge Template using Java
- Perform Mail Merge in Word Document using Java
- Mail Merge using XML Data Source
- Perform Mail Merge with Regions
- Create Nested Mail Merge Regions
- Custom Formatting of Merge Fields
About Mail Merge
Mail Merge is a convenient way of generating letters, envelopes, invoices, reports, and other types of documents dynamically. Using Mail Merge, you create a template file containing the merge fields and then populate those fields using the data in a data source. Let’s suppose you have to send a letter to 20 different people and you only need to change the name and the address of the receivers on each copy. In this case, you can create a Mail Merge template for the letter and then generate 20 letters by populating the name and address fields dynamically.
Java Mail Merge API - Free Download
Aspose.Words for Java is a well-known word processing API that lets you create various types of documents from scratch. The API provides built-in Mail Merge features that allow you to dynamically generate documents using templates and data sources. Aspose.Words for Java can be downloaded as JAR or installed within the Maven-based applications.
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-words</artifactId>
<version>20.11</version>
<classifier>jdk17</classifier>
</dependency>
Data Sources for Mail Merge
The data in the Mail Merge could be fetched from any data source such as JSON, XML, spreadsheet, or a database.
Create Template for Mail Merge in MS Word
The template being used in Mail Merge can be a simple Word document (i.e DOCX) and it doesn’t need to be in a template format. The template document contains the merge fields that are populated with data when Mail Merge is executed. The following are the steps of how to prepare a Mail Merge template using MS Word.
- Create a new document in MS Word.
- Place the cursor where you want to add a merge field.
- From the Insert menu select the Field option.
- From the Field names list, select MergeField.
- Enter a name for the merge field in the Field name and press OK.
- Save the document as DOCX.
The following is the screenshot of the sample template document.

Create Mail Merge Template using Java
You can also generate the Mail Merge template programmatically. The following are the steps for it.
- Create an instance of DocumentBuilder class.
- Insert merge fields using methods provided by DocumentBuilder such as insertTextInput, insertField, InsertParagraph, and etc.
- Save the document using DocumentBuilder.getDocument().save(String fileName) method.
The following code sample shows how to create a Mail Merge template using Java.
Perform Mail Merge in Word Document using Java
Once the template is ready, you can populate the merge fields with data. The following are the steps of performing Mail Merge on a Word template.
- Create a new template using Document class (or load the existing template).
- Create an instance of DocumentBuilder class and pass the Document object to its constructor.
- Execute a Mail Merge using Document.getMailMerge().execute() method and pass the data source as the parameter.
- Save the generated Word document using DocumentBuilder.getDocument().save(String) method.
The following code sample shows how to perform mail merge in Word documents using Java.
Template

Output
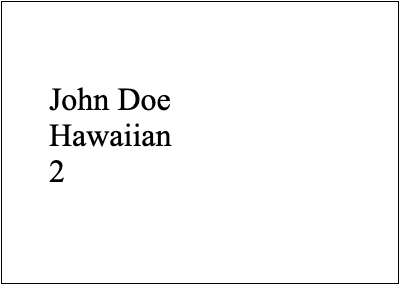
Perform Mail Merge using XML Data Source
In the previous example, we performed Mail Merge using the Java objects. However, in most of the cases, a data source is used to populate the merge fields. For demonstration, let’s check out how to use an XML data source in Mail Merge. The following are the steps for it.
- Load the XML data source using the DataSet class.
- Load the Mail Merge template using Document class.
- Use the execute function to populate the merge fields using the desired data table in the data source.
- Save the generated Word document using Document.save(String) method.
The following is the XML data source being used in this example.
The following code sample shows how to populate the Mail Merge template using the Customer data table in the provided XML data source.
Template
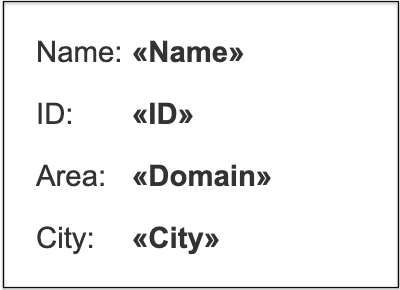
Output
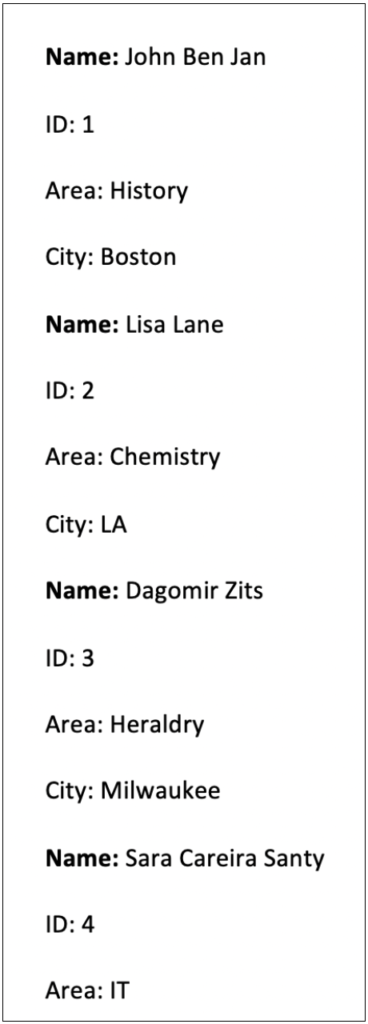
Mail Merge with Regions in Java
In certain cases, you may need to repeat a particular region in the document. For example, you want to display orders placed by each customer in a separate table. In such cases, you can take advantage of the Mail Merge regions. In order to create a region, you can specify the start and the end of the region. As a result, the region will be repeated for each instance of the data during the Mail Merge execution.
The following screenshot shows a template in which the region consists of a table. It starts with «TableStart:Customers» and ends at «TableEnd:Customers».

The following code sample shows how to create a template with regions and populate it with the data.
Output
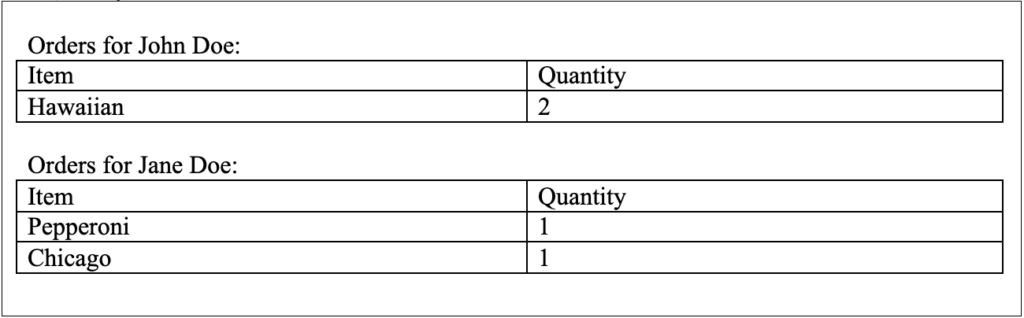
Create Nested Mail Merge Regions using Java
Another popular scenario in Mail Merge is when you have nested regions. For example, when you have to list the orders and the items in each order then you can use the nested regions. The following image makes the picture more clear about the nested regions.

In the image above, we have the Orders table and the Items table where each record in Items is linked to a record in Orders. Hence, there exists a one-to-many relationship between these two tables. In such cases, Aspose.Words executes the Mail Merge taking care of the relationships defined in the DataSet. For instance, if we have an XML data source then Aspose.Words will either use the schema information or the structure of XML to find out the relationships. Thus, you will not have to handle it manually by yourself and the Document.getMailMerge().executeWithRegions(DataSet) method will work for you (as the previous example).
Apply Custom Formatting on Merge Fields
In order to give you more control over the Mail Merge, Aspose.Words for Java allows you to customize the merge fields during the Mail Merge execution. The setFieldMergingCallback(IFieldMergingCallback) method accepts a class which implements fieldMerging(FieldMergingArgs) and imageFieldMerging(ImageFieldMergingArgs) methods for custom control over the Mail Merge process. The fieldMerging(FieldMergingArgs) event occurs when a merge field is encountered during the Mail Merge execution.
The following is the complete code sample of how to customize the Mail Merge operation and apply formatting to the cells.
Conclusion
Mail Merge provides you with a wide range of features to generate MS Word documents dynamically. You can generate simple as well as complex reports based on the data in databases or other data sources. In this article, you have seen how to implement the Mail Merge features programmatically using Java. You can learn more about the Java Mail Merge API using the documentation.