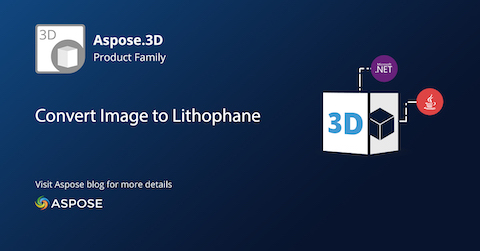
JPG 圖像廣泛用於保存圖片、繪圖和其他視覺信息。然而,litophane 表示三維圖像,其特性會根據其背後的光源而改變。本文介紹如何在 C# 中將 JPG 圖像轉換為 lithohane。
JPG 圖像到 Lithophane 轉換器 – C# API 安裝
Aspose.3D for .NET API 可用於操作不同的三維模型。通過從 下載 部分或使用以下 NuGet 安裝命令下載 DLL 文件來簡單地配置 API:
PM> Install-Package Aspose.3D
在 C# 中將 JPG 圖像轉換為 Lithophane
您可以按照以下步驟將 JPG 圖像轉換為 Lithophane:
- 加載輸入的 JPG 圖像。
- 對網格對象執行計算操作。
- 使用 Save 方法生成 3d 場景並保存對象。
以下示例代碼演示瞭如何在 C# 中以編程方式將 JPG 圖像轉換為 Lithophane:
string file = "template.jpg";
string output = "file.fbx";
// 創建一些新參數
Aspose.ThreeD.Render.TextureData td = Aspose.ThreeD.Render.TextureData.FromFile(file);
const float nozzleSize = 0.9f;
const float layerHeight = 0.2f;
var grayscale = ToGrayscale(td);
const float width = 120.0f;
float height = width / td.Width * td.Height;
float thickness = 10.0f;
float layers = thickness / layerHeight;
int widthSegs = (int)Math.Floor(width / nozzleSize);
int heightSegs = (int)Math.Floor(height / nozzleSize);
// 對 Mesh 對象執行計算操作
Aspose.ThreeD.Entities.Mesh mesh = new Aspose.ThreeD.Entities.Mesh();
for (int y = 0; y < heightSegs; y++)
{
float dy = (float)y / heightSegs;
for (int x = 0; x < widthSegs; x++)
{
float dx = (float)x / widthSegs;
float gray = Sample(grayscale, td.Width, td.Height, dx, dy);
float v = (1 - gray) * thickness;
mesh.ControlPoints.Add(new Aspose.ThreeD.Utilities.Vector4(dx * width, dy * height, v));
}
}
for (int y = 0; y < heightSegs - 1; y++)
{
int row = (y * heightSegs);
int ptr = row;
for (int x = 0; x < widthSegs - 1; x++)
{
mesh.CreatePolygon(ptr, ptr + widthSegs, ptr + 1);
mesh.CreatePolygon(ptr + 1, ptr + widthSegs, ptr + widthSegs + 1);
ptr++;
}
}
// 生成 3d 場景並保存對象
Aspose.ThreeD.Scene scene = new Aspose.ThreeD.Scene(mesh);
scene.Save(output, Aspose.ThreeD.FileFormat.FBX7400ASCII);
// 要調用的示例方法
static float Sample(float[,] data, int w, int h, float x, float y)
{
return data[(int)(x * w), (int)(y * h)];
}
// ToGrayscale 方法調用
static float[,] ToGrayscale(Aspose.ThreeD.Render.TextureData td)
{
var ret = new float[td.Width, td.Height];
var stride = td.Stride;
var data = td.Data;
var bytesPerPixel = td.BytesPerPixel;
for (int y = 0; y < td.Height; y++)
{
int ptr = y * stride;
for (int x = 0; x < td.Width; x++)
{
var v = (data[ptr] * 0.21f + data[ptr + 1] * 0.72f + data[ptr + 2] * 0.07f) / 255.0f;
ret[x, y] = v;
ptr += bytesPerPixel;
}
}
return ret;
}
獲得免費的臨時許可證
您可以通過申請 免費臨時許可證 來全面評估 API。
在線演示
請嘗試使用此 API 開發的 JPG Image to Lithophane Converter 網絡應用程序。
結論
在本文中,您探索了 JPG 圖像到 lithophane 的轉換。您可以使用 lithophane 創建 3D 模型,它使用厚度來反映圖像的暗度,當您將光源放在打印模型後面時,您可以看到圖像。此外,您可以訪問 文檔 部分以查看 API 提供的其他功能。如果您發現任何歧義,請通過 論壇 與我們聯繫。