
條形碼包含有關產品或公司的信息,以機器可讀的形式直觀地表示。條形碼廣泛用於跟踪貨運和庫存管理。我們可以在 WPF 應用程序中輕鬆生成各種類型的條形碼。在本文中,我們將學習如何在 WPF 應用程序中生成和顯示條碼圖像。完成上述步驟後,我們將在 C# 中擁有自己的 WPF 條碼生成器。讓我們開始吧。
文章應涵蓋以下主題:
WPF條碼生成器的特點
我們的 WPF 條碼生成器將具有以下功能。
C# 條碼生成器 API
我們將使用 Aspose.BarCode for .NET API 生成條碼圖像並在 WPF 應用程序中預覽它們。它是一個功能豐富的 API,可讓您生成、掃描和讀取各種條碼類型。此外,它允許操縱生成的條形碼的外觀,例如背景顏色、條形顏色、旋轉角度、x 維度、圖像質量、分辨率、標題、大小等等。
創建 WPF 條碼生成器的步驟
我們可以按照以下步驟在 WPF 應用程序中生成和顯示條碼圖像:
- 首先,創建一個新項目並選擇 WPF Application 項目模板。

接下來,輸入項目名稱,例如“BarcodeGen”。
然後,選擇.NET框架,然後選擇創建。
接下來,打開 NuGet 包管理器並安裝 Aspose.BarCode for .NET 包。
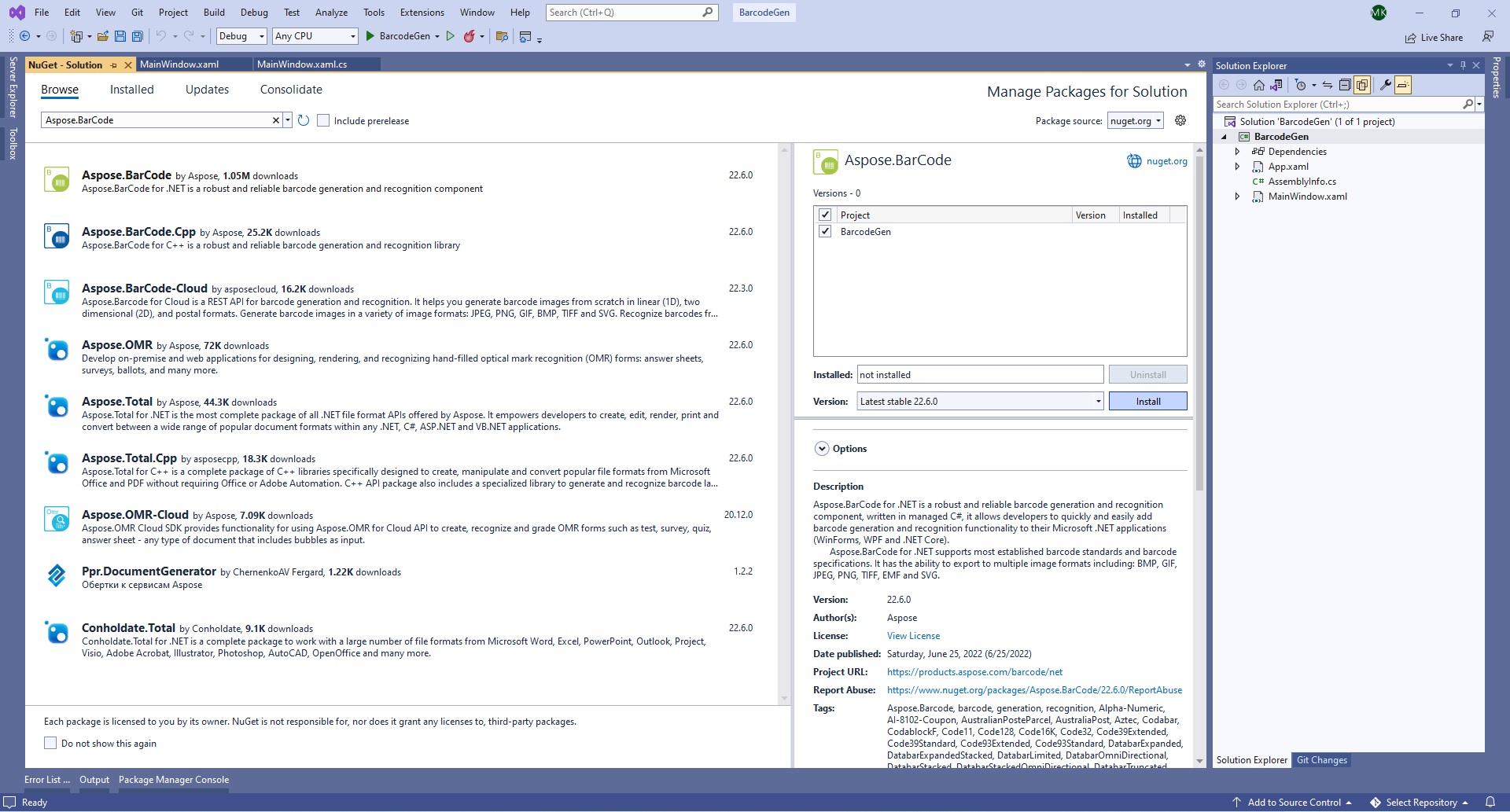
為 .NET 安裝 Aspose.BarCode
- 然後,添加一個新類 Barcode.cs 來定義條形碼。
public class Barcode
{
public string? Text { get; set; }
public BaseEncodeType? BarcodeType { get; set; }
public BarCodeImageFormat ImageType { get; set; }
}
- 接下來,打開 MainWindow.xaml 並添加所需的控件,如下所示:
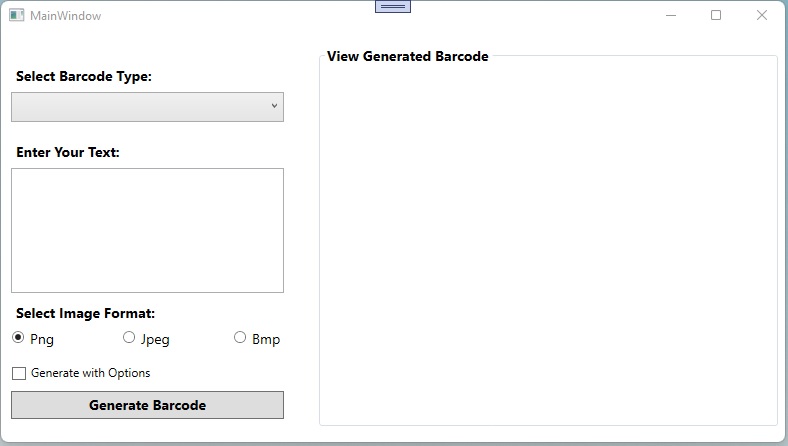
添加需要的控件
您還可以將 MainWindow.xaml 的內容替換為以下腳本。
<Window x:Class="BarcodeGen.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:BarcodeGen"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid Width="800" Height="384">
<Grid.RowDefinitions>
<RowDefinition Height="191*"/>
<RowDefinition/>
</Grid.RowDefinitions>
<Label Content="Select Barcode Type:" HorizontalAlignment="Left" Margin="10,16,0,0" VerticalAlignment="Top" FontSize="14" FontWeight="Bold"/>
<ComboBox x:Name="comboBarcodeType" HorizontalAlignment="Left" Margin="10,47,0,305" Width="273" Text="Select Barcode Type" IsReadOnly="True" SelectedIndex="-1" FontSize="14" Height="30">
<ComboBoxItem Content="Code128"></ComboBoxItem>
<ComboBoxItem Content="Code11"></ComboBoxItem>
<ComboBoxItem Content="Code32"></ComboBoxItem>
<ComboBoxItem Content="QR"></ComboBoxItem>
<ComboBoxItem Content="DataMatrix"></ComboBoxItem>
<ComboBoxItem Content="EAN13"></ComboBoxItem>
<ComboBoxItem Content="EAN8"></ComboBoxItem>
<ComboBoxItem Content="ITF14"></ComboBoxItem>
<ComboBoxItem Content="PDF417"></ComboBoxItem>
</ComboBox>
<Button Name="btnGenerate" Click="btnGenerate_Click" Content="Generate Barcode" HorizontalAlignment="Left" Margin="10,346,0,0" VerticalAlignment="Top" Height="28" Width="273" FontSize="14" FontWeight="Bold"/>
<Label Content="Enter Your Text:" HorizontalAlignment="Left" Margin="10,92,0,0" VerticalAlignment="Top" FontSize="14" FontWeight="Bold"/>
<TextBox Name="tbCodeText" TextWrapping="Wrap" Margin="10,123,517,134" Width="273" Height="125"/>
<Label Content="Select Image Format:" HorizontalAlignment="Left" Margin="10,253,0,0" VerticalAlignment="Top" FontSize="14" FontWeight="Bold"/>
<RadioButton Name="rbPng" Content="Png" GroupName="rbImageType" Margin="10,285,739,77" Width="51" Height="20" FontSize="14" IsChecked="True"/>
<RadioButton Name="rbJpg" Content="Jpeg" GroupName="rbImageType" Margin="121,285,628,77" Width="51" Height="20" FontSize="14"/>
<RadioButton Name="rbBmp" Content="Bmp" GroupName="rbImageType" Margin="232,285,517,77" Width="51" Height="20" FontSize="14"/>
<CheckBox Name="cbGenerateWithOptions" Height="20" Margin="10,321,517,41" Content="Generate with Options" />
<GroupBox Header="View Generated Barcode" Margin="317,0,22,0" FontSize="14" FontWeight="Bold">
<Image Name="imgDynamic" Margin="6,-6,7,6" Stretch="None" />
</GroupBox>
</Grid>
</Window>
- 然後,打開 MainWindow.xaml.cs 類並添加 btnGenerateClick 事件來處理生成條碼按鈕的單擊操作。
private void btnGenerate_Click(object sender, RoutedEventArgs e)
{
// 將默認設置為 Png
var imageType = "Png";
// 獲取用戶選擇的圖像格式
if(rbPng.IsChecked == true)
{
imageType = rbPng.Content.ToString();
}
else if(rbBmp.IsChecked == true)
{
imageType = rbBmp.Content.ToString();
}
else if(rbJpg.IsChecked == true)
{
imageType = rbJpg.Content.ToString();
}
// 從枚舉中獲取圖像格式
var imageFormat = (BarCodeImageFormat)Enum.Parse(typeof(BarCodeImageFormat), imageType.ToString());
// 設置默認為 Code128
var encodeType = EncodeTypes.Code128;
// 獲取用戶選擇的條碼類型
if (!string.IsNullOrEmpty(comboBarcodeType.Text))
{
switch (comboBarcodeType.Text)
{
case "Code128":
encodeType = EncodeTypes.Code128;
break;
case "ITF14":
encodeType = EncodeTypes.ITF14;
break;
case "EAN13":
encodeType = EncodeTypes.EAN13;
break;
case "Datamatrix":
encodeType = EncodeTypes.DataMatrix;
break;
case "Code32":
encodeType = EncodeTypes.Code32;
break;
case "Code11":
encodeType = EncodeTypes.Code11;
break;
case "PDF417":
encodeType = EncodeTypes.Pdf417;
break;
case "EAN8":
encodeType = EncodeTypes.EAN8;
break;
case "QR":
encodeType = EncodeTypes.QR;
break;
}
}
// 初始化條形碼對象
Barcode barcode = new Barcode();
barcode.Text = tbCodeText.Text;
barcode.BarcodeType = encodeType;
barcode.ImageType = imageFormat;
try
{
string imagePath = "";
if (cbGenerateWithOptions.IsChecked == true)
{
// 使用附加選項生成條形碼並獲取圖像路徑
imagePath = GenerateBarcodeWithOptions(barcode);
}
else
{
// 生成條形碼並獲取圖片路徑
imagePath = GenerateBarcode(barcode);
}
// 顯示圖像
Uri fileUri = new Uri(Path.GetFullPath(imagePath));
imgDynamic.Source = new BitmapImage(fileUri);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
- 之後,添加一個生成條形碼的函數。
private string GenerateBarcode(Barcode barcode)
{
// 圖片路徑
string imagePath = comboBarcodeType.Text + "." + barcode.ImageType;
// 初始化條碼生成器
BarcodeGenerator generator = new BarcodeGenerator(barcode.BarcodeType, barcode.Text);
// 保存圖像
generator.Save(imagePath, barcode.ImageType);
return imagePath;
}
- 最後,運行應用程序。
使用其他選項生成條形碼
我們還可以生成帶有特定於條形碼類型的附加選項的條形碼。在 WPF 條碼生成器中,我們添加了一個複選框來生成帶選項的條碼。它將調用以下函數,為不同的條形碼類型指定附加選項。
private string GenerateBarcodeWithOptions(Barcode barcode)
{
// 圖片路徑
string imagePath = comboBarcodeType.Text + "." + barcode.ImageType;
// 初始化條碼生成器
BarcodeGenerator generator = new BarcodeGenerator(barcode.BarcodeType, barcode.Text);
if(barcode.BarcodeType == EncodeTypes.QR)
{
generator.Parameters.Barcode.XDimension.Pixels = 4;
//設置自動版本
generator.Parameters.Barcode.QR.QrVersion = QRVersion.Auto;
//設置自動 QR 編碼類型
generator.Parameters.Barcode.QR.QrEncodeType = QREncodeType.Auto;
}
else if(barcode.BarcodeType == EncodeTypes.Pdf417)
{
generator.Parameters.Barcode.XDimension.Pixels = 2;
generator.Parameters.Barcode.Pdf417.Columns = 3;
}
else if(barcode.BarcodeType == EncodeTypes.DataMatrix)
{
//將 DataMatrix ECC 設置為 140
generator.Parameters.Barcode.DataMatrix.DataMatrixEcc = DataMatrixEccType.Ecc200;
}
else if(barcode.BarcodeType == EncodeTypes.Code32)
{
generator.Parameters.Barcode.XDimension.Millimeters = 1f;
}
else
{
generator.Parameters.Barcode.XDimension.Pixels = 2;
//設置 BarHeight 40
generator.Parameters.Barcode.BarHeight.Pixels = 40;
}
// 保存圖像
generator.Save(imagePath, barcode.ImageType);
return imagePath;
}
您可以在文檔中閱讀有關 條碼類型的生成規範 的更多信息。
演示 WPF 條碼生成器
以下是我們剛剛創建的 WPF 條碼生成器應用程序的演示。

演示 WPF 條碼生成器
下載源代碼
您可以從 GitHub 下載 WPF 條碼生成器應用程序的完整源代碼。
獲得免費許可證
您可以 獲得免費的臨時許可證 來試用該庫,而沒有評估限制。
結論
在本文中,我們了解瞭如何在 WPF 應用程序中生成各種類型的條形碼。我們還了解瞭如何以編程方式預覽生成的條形碼圖像。此外,您可以使用文檔了解更多關於 Aspose.BarCode for .NET API 的信息。如有任何疑問,請隨時在我們的 論壇 上與我們聯繫。