數據透視表重新排列數據以有意義的方式表示它。它們提供不同的排序選項,並通過將數據分組在一起來提供總和、平均值或其他統計數據。它是數據分析的重要工具,是 MS Excel 的基本組成部分。您可能會發現自己處於需要以編程方式創建和操作數據透視表的場景中。為此,本文將教您如何使用 C++ 在 Excel 文件中處理數據透視表。
- 用於處理 Excel 文件中的數據透視表的 C++ API
- 使用 C++ 在 Excel 文件中創建數據透視表
- 使用 C++ 對 Excel 文件中的數據透視表進行排序
- 使用 C++ 隱藏數據透視表中的行
- 使用 C++ 操作數據透視表數據
用於處理 Excel 文件中的數據透視表的 C++ API
Aspose.Cells for C++ 是一個本地 C++ 庫,允許您創建、讀取和更新 Excel 文件,而無需安裝 Microsoft Excel。 API 還支持使用 Excel 文件中的數據透視表。您可以通過 NuGet 安裝 API 或直接從 下載 部分下載。
PM> Install-Package Aspose.Cells.Cpp
使用 C++ 在 Excel 文件中創建數據透視表
在下面的示例中,我們將創建一個新的 Excel 文件,將示例數據插入其中並創建一個數據透視表。本示例中生成的文件將用作其他示例的源文件。以下是在 Excel 文件中創建數據透視表的步驟。
- 首先,創建一個 IWorkbook 類的實例來表示新的 Excel 文件。
- 使用 IWorkbook->GetIWorksheets()->GetObjectByIndex (Aspose::Cells::Systems::Int32 index) 方法訪問要插入數據透視表的工作表。
- 為數據透視表添加示例數據。
- 使用 IWorksheet->GetIPivotTables()->Add(intrusiveptrAspose::Cells::Systems::String sourceData,侵入式Aspose::Cells::Systems::StringdestCellName,侵入式Aspose::Cells::Systems::String表名) 方法。
- 要訪問數據透視表,請使用 IWorksheet->GetIPivotTables()->GetObjectByIndex(Aspose::Cells::Systems::Int32 index) 方法。
- 操作字段並設置數據透視表的樣式。
- 最後,使用 IWorkbook->Save (intrusiveptrAspose::Cells::Systems::String文件名) 方法。
以下示例代碼顯示瞭如何使用 C++ 在 Excel 文件中創建數據透視表。
// 源目錄路徑。
StringPtr srcDir = new String("SourceDirectory\\");
// 輸出目錄路徑。
StringPtr outDir = new String("OutputDirectory\\");
// 創建 IWorkbook 類的實例
intrusive_ptr<IWorkbook> workbook = Factory::CreateIWorkbook();
// 訪問第一個工作表
intrusive_ptr<IWorksheet> worksheet = workbook->GetIWorksheets()->GetObjectByIndex(0);
// 為數據透視表添加源數據
intrusive_ptr<String> str = new String("Fruit");
worksheet->GetICells()->GetObjectByIndex(new String("A1"))->PutValue(str);
str = new String("Quantity");
worksheet->GetICells()->GetObjectByIndex(new String("B1"))->PutValue(str);
str = new String("Price");
worksheet->GetICells()->GetObjectByIndex(new String("C1"))->PutValue(str);
str = new String("Apple");
worksheet->GetICells()->GetObjectByIndex(new String("A2"))->PutValue(str);
str = new String("Orange");
worksheet->GetICells()->GetObjectByIndex(new String("A3"))->PutValue(str);
str = new String("Mango");
worksheet->GetICells()->GetObjectByIndex(new String("A4"))->PutValue(str);
worksheet->GetICells()->GetObjectByIndex(new String("B2"))->PutValue(3);
worksheet->GetICells()->GetObjectByIndex(new String("B3"))->PutValue(4);
worksheet->GetICells()->GetObjectByIndex(new String("B4"))->PutValue(4);
worksheet->GetICells()->GetObjectByIndex(new String("C2"))->PutValue(2);
worksheet->GetICells()->GetObjectByIndex(new String("C3"))->PutValue(1);
worksheet->GetICells()->GetObjectByIndex(new String("C4"))->PutValue(4);
// 添加數據透視表
int idx = worksheet->GetIPivotTables()->Add(new String("A1:C4"), new String("E5"), new String("MyPivotTable"));
// 訪問創建的數據透視表
intrusive_ptr<IPivotTable> pivotTable = worksheet->GetIPivotTables()->GetObjectByIndex(idx);
// 操縱數據透視表的行、列和數據字段
pivotTable->AddFieldToArea(PivotFieldType_Row, pivotTable->GetIBaseFields()->GetObjectByIndex(0));
pivotTable->AddFieldToArea(PivotFieldType_Data, pivotTable->GetIBaseFields()->GetObjectByIndex(1));
pivotTable->AddFieldToArea(PivotFieldType_Data, pivotTable->GetIBaseFields()->GetObjectByIndex(2));
pivotTable->AddFieldToArea(PivotFieldType_Column, pivotTable->GetIDataField());
// 設置數據透視表樣式
pivotTable->SetPivotTableStyleType(PivotTableStyleType_PivotTableStyleMedium9);
// 保存輸出的excel文件
workbook->Save(outDir->StringAppend(new String("outputCreatePivotTable.xlsx")));
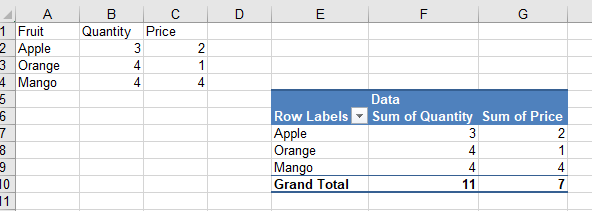
示例代碼創建的數據透視表的圖像
使用 C++ 對 Excel 文件中的數據透視表進行排序
在下面的示例中,我們將按降序對數據透視表的第一列進行排序。以下是對數據透視表中的數據進行排序的步驟。
- 首先,使用 IWorkbook 類加載示例 Excel 文件。
- 使用 IWorkbook->GetIWorksheets()->GetObjectByIndex (Aspose::Cells::Systems::Int32 index) 方法檢索包含數據透視表的工作表。
- 使用 IWorksheet->GetIPivotTables()->GetObjectByIndex(Aspose::Cells::Systems::Int32 index) 方法訪問數據透視表。
- 使用 IPivotField->SetAutoSort(bool value) 和 IPivotField->SetAscendSort(bool value) 方法獲取行字段並對數據透視表進行排序。
- 刷新數據透視表的內容並分別使用 IPivotTable->RefreshData() 和 IPivotTable->CalculateData() 方法計算數據。
- 最後,使用 IWorkbook->Save (intrusiveptrAspose::Cells::Systems::String文件名) 方法。
以下示例代碼演示瞭如何使用 C++ 對 Excel 文件中的數據透視表進行排序。
// 源目錄路徑。
StringPtr srcDir = new String("SourceDirectory\\");
// 輸出目錄路徑。
StringPtr outDir = new String("OutputDirectory\\");
// 輸入excel文件的路徑
StringPtr samplePivotTable = srcDir->StringAppend(new String("SamplePivotTable.xlsx"));
// 輸出excel文件的路徑
StringPtr outputSortedPivotTable = outDir->StringAppend(new String("outputSortedPivotTable.xlsx"));
// 加載示例 excel 文件
intrusive_ptr<IWorkbook> workbook = Factory::CreateIWorkbook(samplePivotTable);
// 訪問第一個工作表
intrusive_ptr<IWorksheet> worksheet = workbook->GetIWorksheets()->GetObjectByIndex(0);
// 訪問數據透視表
intrusive_ptr<IPivotTable> pivotTable = worksheet->GetIPivotTables()->GetObjectByIndex(0);
// 設置數據透視表排序
pivotTable->AddFieldToArea(PivotFieldType_Row, 0);
intrusive_ptr<IPivotField> pivotField = pivotTable->GetIRowFields()->GetObjectByIndex(0);
pivotField->SetAutoSort(true);
pivotField->SetAscendSort(false);
// 刷新併計算數據透視表中的數據。
pivotTable->RefreshData();
pivotTable->CalculateData();
// 保存輸出的excel文件
workbook->Save(outputSortedPivotTable);

示例代碼生成的排序數據透視表的圖像
使用 C++ 隱藏數據透視表中的行
使用 Aspose.Cells for C++ API,您還可以隱藏數據透視表中的行。在下面的示例中,我們將隱藏帶有“Orange”行標籤的行。以下是隱藏數據透視表中行的步驟。
- 首先,使用 IWorkbook 類加載示例 Excel 文件。
- 使用 IWorkbook->GetIWorksheets()->GetObjectByIndex (Aspose::Cells::Systems::Int32 index) 方法檢索包含數據透視表的工作表。
- 使用 IWorksheet->GetIPivotTables()->GetObjectByIndex(Aspose::Cells::Systems::Int32 index) 方法訪問數據透視表。
- 使用 IPivotTable->GetIDataBodyRange() 方法獲取數據透視表數據主體範圍。
- 遍歷數據透視表的行並隱藏符合條件的行。
- 刷新數據透視表的內容並分別使用 IPivotTable->RefreshData() 和 IPivotTable->CalculateData() 方法計算數據。
- 最後,使用 IWorkbook->Save (intrusiveptrAspose::Cells::Systems::String文件名) 方法。
以下示例代碼顯示瞭如何使用 C++ 隱藏數據透視表中的行。
// 源目錄路徑。
StringPtr srcDir = new String("SourceDirectory\\");
// 輸出目錄路徑。
StringPtr outDir = new String("OutputDirectory\\");
// 輸入excel文件的路徑
StringPtr samplePivotTable = srcDir->StringAppend(new String("SamplePivotTable.xlsx"));
// 輸出excel文件的路徑
StringPtr outputHiddenRowPivotTable = outDir->StringAppend(new String("outputHiddenRowPivotTable.xlsx"));
// 加載示例 excel 文件
intrusive_ptr<IWorkbook> workbook = Factory::CreateIWorkbook(samplePivotTable);
// 訪問第一個工作表
intrusive_ptr<IWorksheet> worksheet = workbook->GetIWorksheets()->GetObjectByIndex(0);
// 訪問數據透視表
intrusive_ptr<IPivotTable> pivotTable = worksheet->GetIPivotTables()->GetObjectByIndex(0);
// 獲取數據透視表主體範圍
intrusive_ptr<ICellArea> dataBodyRange = pivotTable->GetIDataBodyRange();
// 數據透視表起始行
int currentRow = 5;
// 數據透視表結束行
int rowsUsed = dataBodyRange->GetendRow();
// 遍歷行,比較單元格值並隱藏行。
for (int i = currentRow; i < rowsUsed; i++) {
intrusive_ptr<ICell> cell = worksheet->GetICells()->GetICell(i, 4);
if (strcmp(cell->GetStringValue()->charValue(), "Orange") == 0) {
worksheet->GetICells()->HideRow(i);
}
}
// 刷新併計算數據透視表中的數據。
pivotTable->RefreshData();
pivotTable->CalculateData();
// 保存輸出的excel文件
workbook->Save(outputHiddenRowPivotTable);
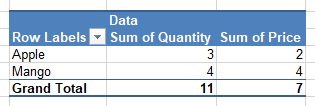
帶有隱藏行的數據透視表的圖像
使用 C++ 操作數據透視表數據
您還可以使用 Aspose.Cells for C++ API 操作現有數據透視表的數據。在以下示例中,我們將單元格“A2”中的文本“Apple”替換為“Orange”,並將更改反映在數據透視表中。以下是操作數據透視表數據的步驟。
- 首先,使用 IWorkbook 類加載示例 Excel 文件。
- 使用 IWorkbook->GetIWorksheets()->GetObjectByIndex (Aspose::Cells::Systems::Int32 index) 方法檢索包含數據透視表數據的工作表。
- 根據您的要求更新數據透視表的數據。
- 為了訪問數據透視表,使用 IWorksheet->GetIPivotTables()->GetObjectByIndex(Aspose::Cells::Systems::Int32 index) 方法。
- 刷新數據透視表的內容並分別使用 IPivotTable->RefreshData() 和 IPivotTable->CalculateData() 方法計算數據。
- 最後,使用 IWorkbook->Save (intrusiveptrAspose::Cells::Systems::String文件名) 方法。
以下示例代碼顯示瞭如何使用 C++ 更新數據透視表的數據。
// 源目錄路徑。
StringPtr srcDir = new String("SourceDirectory\\");
// 輸出目錄路徑。
StringPtr outDir = new String("OutputDirectory\\");
// 輸入excel文件的路徑
StringPtr samplePivotTable = srcDir->StringAppend(new String("SamplePivotTable.xlsx"));
// 輸出excel文件的路徑
StringPtr outputManipulatePivotTable = outDir->StringAppend(new String("outputManipulatePivotTable.xlsx"));
// 加載示例 excel 文件
intrusive_ptr<IWorkbook> workbook = Factory::CreateIWorkbook(samplePivotTable);
// 訪問第一個工作表
intrusive_ptr<IWorksheet> worksheet = workbook->GetIWorksheets()->GetObjectByIndex(0);
// 更改數據透視表源數據內的單元格 A2 的值
intrusive_ptr<String> str = new String("Orange");
worksheet->GetICells()->GetObjectByIndex(new String("A2"))->PutValue(str);
// 訪問數據透視表,刷新併計算它
intrusive_ptr<IPivotTable> pivotTable = worksheet->GetIPivotTables()->GetObjectByIndex(0);
pivotTable->RefreshData();
pivotTable->CalculateData();
// 保存輸出的excel文件
workbook->Save(outputManipulatePivotTable);
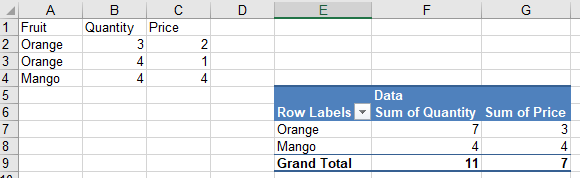
顯示更新數據的數據透視表
獲得免費許可證
為了在沒有評估限制的情況下試用 API,您可以申請免費的臨時許可證。
結論
在本文中,您學習瞭如何使用 C++ 在 Excel 文件中處理數據透視表。具體來說,您學習瞭如何使用 C++ 創建數據透視表以及排序、隱藏和更新數據透視表中的數據。 Aspose.Cells for C++ 是一個龐大的 API,它提供了一系列用於處理 Excel 文件的附加功能。您可以通過訪問 官方文檔 來詳細探索 API。如有任何疑問,請隨時通過我們的免費支持論壇與我們聯繫。