
在圖像中添加文本是添加上下文、品牌甚至只是一點點樂趣的好方法。它可用於創建模因、社交媒體帖子、營銷材料等。在這篇博文中,我們將學習如何在 C# 中向圖像添加文本。我們將逐步指導您如何在 JPG 或 PNG 格式的照片或其他圖像上書寫。那麼讓我們開始吧!
本文涵蓋以下主題:
用於向圖像添加文本的 C# API
我們將使用 Aspose.Drawing for .NET 向圖像添加文本。它是一個功能強大且多功能的 2D 圖形庫,允許開發人員在各種應用程序中創建和操作圖形。 Aspose.Drawing for .NET 支持廣泛的圖像處理操作,例如裁剪、調整大小、旋轉、翻轉和水印。對於需要為其 .NET 應用程序提供跨平台、高性能圖形庫的開發人員來說,它是一個不錯的選擇。
請下載 API 的 DLL 或使用 NuGet 安裝它。
PM> Install-Package Aspose.Drawing
在 C# 中向 JPG 圖像添加文本
我們可以按照以下步驟向 JPG 圖像添加文本:
- 使用 Bitmap 類加載 JPG 圖像。
- 使用 FromImage() 方法從 Bitmap 對象創建一個新的 Graphics 對象。
- 使用指定的文本顏色初始化 SolidBrush 類對象。
- 使用所需的文本字體系列、樣式和大小定義 Font 類對象。
- (可選)初始化一個 Rectangle 對象。
- 之後,使用要顯示的文本、Font、Brush 和 Rectangle 類對像作為參數調用 DrawString() 方法。
- 最後,使用 Save() 方法保存輸出圖像。
以下代碼示例演示如何使用 C# 將文本添加到 JPG 圖像。
// 加載圖像
Bitmap bitmap = new Bitmap("C:\\Files\\Sample_JPG.jpg");
Graphics graphics = Graphics.FromImage(bitmap);
// 定義文本顏色
Brush brush = new SolidBrush(Color.DarkBlue);
// 定義文本字體
Font arial = new Font("Arial", 25, FontStyle.Regular);
// 要顯示的文本
string text = "Hello, this is a sample text!";
// 定義矩形
Rectangle rectangle = new Rectangle(100, 100, 450, 100);
// 在圖像上繪製文本
graphics.DrawString(text, arial, brush, rectangle);
// 保存輸出文件
bitmap.Save("C:\\Files\\DrawTextOnJpg.jpg");

在 C# 中向 JPG 圖像添加文本
在 C# 中將文本添加到 PNG 圖像
同樣,我們可以按照前面提到的步驟向 PNG 圖像添加文本。但是,我們需要在第一步加載 PNG 圖像。
以下代碼示例演示如何使用 C# 將文本添加到 PNG 圖像。
// 加載圖像
Bitmap bitmap = new Bitmap("C:\\Files\\Sample_PNG.png");
Graphics graphics = Graphics.FromImage(bitmap);
// 定義文本顏色
Brush brush = new SolidBrush(Color.Red);
// 定義文本字體
Font arial = new Font("Arial", 30, FontStyle.Regular);
// 要顯示的文本
string text = "Hello, this is a sample text!";
// 定義矩形
Rectangle rectangle = new Rectangle(400, 1500, 1600, 150);
// 指定矩形邊框
Pen pen = new Pen(Color.White, 2);
// 繪製矩形
graphics.DrawRectangle(pen, rectangle);
// 在圖像上繪製文本
graphics.DrawString(text, arial, brush, rectangle);
// 保存輸出文件
bitmap.Save("C:\\Files\\DrawText.png");

在 C# 中向 PNG 圖像添加文本
為照片添加標題 - 為照片添加文字
我們還可以按照以下步驟為照片添加標題:
- 使用 Bitmap 類加載照片圖像。
- 使用加載圖像的大小創建一個新位圖,並添加標題的矩形大小。
- 使用 FromImage() 方法從 Bitmap 對象創建一個新的 Graphics 對象。
- 使用 DrawImage() 方法在新創建的圖像上繪製加載的圖像。
- 為標題框繪製一個填充矩形。
- 使用 StringFormat 類指定文本字符串格式。
- 定義文本、顏色和字體
- 之後,使用要顯示的文本、Font、Brush 和 Rectangle 類對像作為參數調用 DrawString() 方法。
- 最後,使用 Save() 方法保存輸出圖像。
以下代碼示例展示瞭如何使用 C# 為照片添加標題。
// 加載圖像
Bitmap bitmap = new Bitmap("C:\\Files\\tower.jpg");
var imageHeight = bitmap.Height;
var imageWidth = bitmap.Width;
var textHeight = 50;
// 創建一個新位圖,其大小為加載圖像+標題矩形
Bitmap img = new Bitmap(imageWidth, imageHeight + textHeight, System.Drawing.Imaging.PixelFormat.Format32bppPArgb);
Graphics graphics = Graphics.FromImage(img);
// 在新創建的圖像上繪製加載的圖像
graphics.DrawImage(bitmap, 0, 0);
// 畫一個矩形作為標題框
Rectangle rectangle = new Rectangle(0, imageHeight, imageWidth, textHeight);
Brush fillColor = new SolidBrush(Color.White);
Pen pen = new Pen(Color.White, 2);
graphics.DrawRectangle(pen, rectangle);
graphics.FillRectangle(fillColor, rectangle);
// 指定文本字符串格式
StringFormat stringFormat = new StringFormat();
stringFormat.Alignment = StringAlignment.Center;
stringFormat.LineAlignment = StringAlignment.Center;
// 文字顏色
Brush textColor = new SolidBrush(Color.Black);
// 文字字體
Font arial = new Font("Arial", 18, FontStyle.Regular);
// 要顯示的文本
string text = "Hello, this is a sample text!";
// 繪製文字
graphics.TextRenderingHint = TextRenderingHint.AntiAliasGridFit;
graphics.DrawString(text, arial, textColor, rectangle, stringFormat);
// 保存輸出
img.Save("C:\\Files\\DrawTextOnPhoto.jpg");

在 C# 中為照片添加標題
獲取免費許可證
您可以獲得免費的臨時許可證來嘗試 Aspose.Drawing for .NET,而不受評估限制。
在線在圖像上寫入文字
您還可以嘗試免費的在線添加文本到圖像網絡應用程序。其用戶友好的界面使您可以輕鬆簡單地在圖像上寫入文本,而無需安裝任何額外的軟件、創建帳戶或訂閱任何內容。
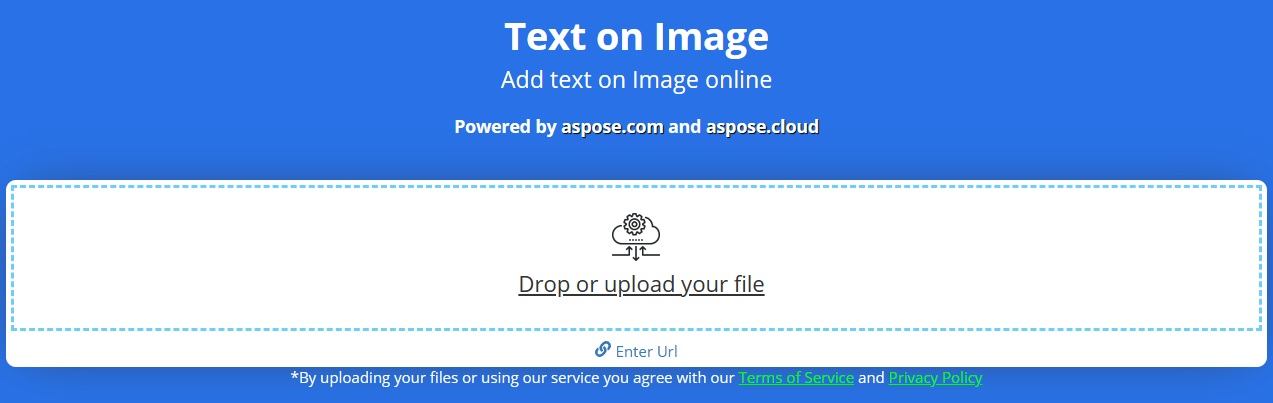
將文本添加到圖像 – 免費學習資源
您可以使用以下資源了解有關向圖像添加文本和在光柵圖像上顯示矢量圖形以及該庫的各種其他功能的更多信息:
結論
在這篇博文中,我們向您展示瞭如何在 C# 中向圖像添加文本。我們介紹了以編程方式在照片和圖像上編寫文本的基礎知識以及一些更高級的技術。此外,我們還推出了一個免費的在線工具,可以隨時隨地向圖像添加文本。如有任何疑問,請隨時通過我們的免費支持論壇與我們聯繫。