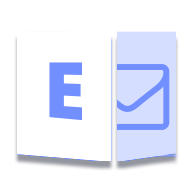
Microsoft Exchange Server 上的對話被稱為線程中的一組電子郵件。簡而言之,一封電子郵件及其所有回复都被認為是一次對話。在本文中,我們將向您展示如何以編程方式處理 MS Exchange Server 中的對話。特別是,您將學習如何在 C# .NET 中查找、複製、移動和刪除 MS Exchange Server 上的對話。
- 用於在 MS Exchange Server 上進行對話的 C# .NET API
- 在 C# 中查找 MS Exchange Server 上的對話
- 在 C# 中復制 MS Exchange 服務器上的對話
- 在 C# 中移動 MS Exchange 服務器上的對話
- 在 C# 中刪除 MS Exchange Server 上的對話
用於在 MS Exchange Server 上進行對話的 C# .NET API
為了管理 Microsoft Exchange Server 上的對話,我們將使用 Aspose.Email for .NET。它是一個強大的 API,提供了一系列功能來實現電子郵件客戶端應用程序。此外,它還允許您無縫訪問 MS Exchange Server 的各種服務。您可以 下載 API 的 DLL 或使用以下命令從 NuGet 安裝它。
PM> Install-Package Aspose.Email
在 C# 中查找 MS Exchange Server 上的對話
以下是在 C# 中從 MS Exchange Server 中的文件夾中查找對話的步驟。
- 首先,使用用戶名、密碼和域創建並初始化 NetworkCredential 對象。
- 然後,用郵箱 URI 和 NetworkCredential 對像初始化 IEWSClient。
- 調用 IEWSClient.FindConversations(IEWSClient.MailboxInfo.InboxUri) 方法並在 ExchangeConversation 數組中獲取對話。
- 最後,遍歷數組中的每個 ExchangeConversation 對象並檢索其詳細信息。
以下代碼示例顯示如何使用 C# 從 MS Exchange Server 中的文件夾中查找對話。
string mailboxUri = "https://ex2010/ews/exchange.asmx";
string username = "test.exchange";
string password = "pwd";
string domain = "ex2010.local";
NetworkCredential credentials = new NetworkCredential(username, password, domain);
// 連接到 MS Exchange 服務器
IEWSClient client = EWSClient.GetEWSClient(mailboxUri, credentials);
Console.WriteLine("Connected to Exchange");
// 從收件箱獲取對話
ExchangeConversation[] conversations = client.FindConversations(client.MailboxInfo.InboxUri);
// 顯示所有對話
foreach (ExchangeConversation conversation in conversations)
{
// 顯示會話屬性,如 Id 和 Topic
Console.WriteLine("Topic: " + conversation.ConversationTopic);
Console.WriteLine("Flag Status: " + conversation.FlagStatus.ToString());
Console.WriteLine();
}
在 C# 中復制 MS Exchange 服務器上的對話
您還可以將對話從一個文件夾複製到另一個文件夾,而無需編寫複雜的代碼。為了演示,我們來看看如何在 C# .NET 中將收件箱中的對話複製到 Exchange Server 的已刪除項目文件夾中。
- 首先,使用用戶名、密碼和域創建並初始化 NetworkCredential 對象。
- 然後,用郵箱 URI 和 NetworkCredential 對像初始化 IEWSClient。
- 調用 IEWSClient.FindConversations(IEWSClient.MailboxInfo.InboxUri) 方法並在 ExchangeConversation 數組中獲取對話。
- 遍歷數組中的每個 ExchangeConversation 並過濾所需的一個。
- 最後,使用 IEWSClient.CopyConversationItems(ExchangeConversation.ConversationId, client.MailboxInfo.DeletedItemsUri) 方法複製對話。
以下代碼示例顯示瞭如何使用 C# .NET 在 MS Exchange Server 中復制對話。
string mailboxUri = "https://ex2010/ews/exchange.asmx";
string username = "test.exchange";
string password = "pwd";
string domain = "ex2010.local";
NetworkCredential credentials = new NetworkCredential(username, password, domain);
// 連接到 MS Exchange 服務器
IEWSClient client = EWSClient.GetEWSClient(mailboxUri, credentials);
Console.WriteLine("Connected to Exchange");
// 獲取對話
ExchangeConversation[] conversations = client.FindConversations(client.MailboxInfo.InboxUri);
foreach (ExchangeConversation conversation in conversations)
{
Console.WriteLine("Topic: " + conversation.ConversationTopic);
// 根據某些條件複製對話項目
if (conversation.ConversationTopic.Contains("test email") == true)
{
client.CopyConversationItems(conversation.ConversationId, client.MailboxInfo.DeletedItemsUri);
Console.WriteLine("Copied the conversation item to another folder");
}
}
在 C# 中移動 MS Exchange 服務器上的對話
在上一節中,我們只是將對話從一個文件夾複製到另一個文件夾。但是,在某些情況下,您可能需要將對話移動到特定文件夾。以下是在 C# .NET 中移動 MS Exchange Server 中的對話的步驟。
- 首先,使用用戶名、密碼和域創建並初始化 NetworkCredential 對象。
- 然後,用郵箱 URI 和 NetworkCredential 對像初始化 IEWSClient。
- 調用 IEWSClient.FindConversations(IEWSClient.MailboxInfo.InboxUri) 方法並在 ExchangeConversation 數組中獲取對話。
- 遍歷數組中的每個 ExchangeConversation 並過濾所需的一個。
- 最後,使用 IEWSClient.MoveConversationItems(ExchangeConversation.ConversationId, client.MailboxInfo.DeletedItemsUri) 方法移動對話。
以下代碼示例顯示如何使用 C# .NET 在 MS Exchange Server 中移動對話。
string mailboxUri = "https://ex2010/ews/exchange.asmx";
string username = "test.exchange";
string password = "pwd";
string domain = "ex2010.local";
NetworkCredential credentials = new NetworkCredential(username, password, domain);
// 連接到 MS Exchange 服務器
IEWSClient client = EWSClient.GetEWSClient(mailboxUri, credentials);
Console.WriteLine("Connected to Exchange");
// 獲取對話
ExchangeConversation[] conversations = client.FindConversations(client.MailboxInfo.InboxUri);
foreach (ExchangeConversation conversation in conversations)
{
Console.WriteLine("Topic: " + conversation.ConversationTopic);
// 根據某種條件移動對話項目
if (conversation.ConversationTopic.Contains("test email") == true)
{
client.MoveConversationItems(conversation.ConversationId, client.MailboxInfo.DeletedItemsUri);
Console.WriteLine("Moved the conversation item to another folder");
}
}
在 C# 中刪除 MS Exchange Server 上的對話
最後但同樣重要的是,讓我們看一下如何使用 C# 從 MS Exchange Server 中刪除對話。
- 首先,使用用戶名、密碼和域創建並初始化 NetworkCredential 對象。
- 然後,用郵箱 URI 和 NetworkCredential 對像初始化 IEWSClient。
- 之後,調用 IEWSClient.FindConversations(IEWSClient.MailboxInfo.InboxUri) 方法並在 ExchangeConversation 數組中獲取對話。
- 遍歷數組中的每個 ExchangeConversation 並過濾所需的一個。
- 最後,使用 IEWSClient.DeleteConversationItems(ExchangeConversation.ConversationId) 方法刪除對話。
以下代碼示例顯示瞭如何在 C# .NET 中從 MS Exchange Server 中刪除對話。
string mailboxUri = "https://ex2010/ews/exchange.asmx";
string username = "test.exchange";
string password = "pwd";
string domain = "ex2010.local";
NetworkCredential credentials = new NetworkCredential(username, password, domain);
// 連接到 MS Exchange 服務器
IEWSClient client = EWSClient.GetEWSClient(mailboxUri, credentials);
Console.WriteLine("Connected to Exchange");
// 獲取對話
ExchangeConversation[] conversations = client.FindConversations(client.MailboxInfo.InboxUri);
foreach (ExchangeConversation conversation in conversations)
{
Console.WriteLine("Topic: " + conversation.ConversationTopic);
// 根據某種條件刪除對話項
if (conversation.ConversationTopic.Contains("test email") == true)
{
client.DeleteConversationItems(conversation.ConversationId);
Console.WriteLine("Deleted the conversation item");
}
}
獲取免費的 API 許可證
您可以獲得免費的臨時許可證,以在沒有評估限制的情況下使用 Aspose.Email for .NET。
結論
在本文中,您了解瞭如何使用 C# 管理 Microsoft Exchange Server 中的對話。您已經了解瞭如何在 C# 中以編程方式查找、複製、移動和刪除 MS Exchange Server 上的對話。此外,您可以瀏覽 文檔 以閱讀有關 Aspose.Email for .NET 的更多信息。此外,您可以通過我們的 論壇 提問。