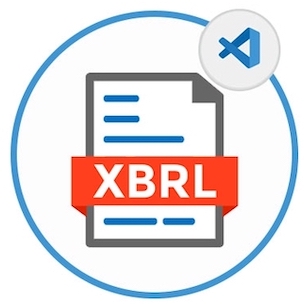
在 上一篇文章 中,我們學習瞭如何使用 C# 將模式引用、上下文、單位和事實對象添加到 XBRL 實例文檔。在本文中,我們將學習如何使用 C# 向 XBRL 添加腳註鏈接和角色引用對象。
本文應涵蓋以下主題:
用於向 XBRL 添加腳註鏈接和角色參考對象的 C# API
為了向 XBRL 實例文檔添加角色引用和弧形角色引用對象,我們將使用 Aspose.Finance for .NET API。它允許創建 XBRL 實例、解析和驗證 XBRL 或 iXBRL 文件。 API 的 XbrlDocument 類表示包含一個或多個 XBRL 實例的 XBRL 文檔。 XBRL 實例是一個 XML 片段,根元素具有 XBRL 標記。 XbrlInstance 類提供各種方法和屬性來處理 XBRL 實例。 FootnoteLink 類包含定位器、資源和弧,用於描述 XBRL 實例中事實之間的關係。 API 提供 RoleReference 類,允許引用 XBRL 實例中腳註鏈接中使用的任何自定義角色屬性值的定義。同樣,ArcRoleReference 類允許解析在鏈接基礎或 XBRL 實例中使用的自定義弧角色值。
PM> Install-Package Aspose.Finance
使用 C# 將腳註鏈接添加到 XBRL
我們可以按照以下步驟在 XBRL 實例文檔中添加腳註鏈接:
- 首先,創建 XbrlDocument 類的一個實例。
- 將新的 XBRL 實例添加到 XbrlDocument 對象的實例。
- 將新的架構引用添加到 XbrlInstance 對象的架構引用。
- 通過 SchemaRefCollection 的索引獲取 SchemaRef。
- 初始化 Context 實例並將其添加到 Context objects 集合中。
- 定義一個 Footnote 實例並設置其標籤和文本。
- 使用 Loc 類實例初始化定位器類型。
- 以定位器標籤和腳註標籤作為參數定義 FootnoteArc。
- 創建 FootnoteLink 類的實例。
- 將 Footnote、Locator 和 FootnoteArc 添加到相關的 FootnoteLink 集合中。
- 之後,將 FootnoteLink 添加到 FootnoteLinks 集合。
- 最後,使用 XbrlDocument.Save() 方法保存 XBRL 文件。它以輸出文件路徑作為參數。
以下代碼示例顯示如何使用 C# 在 XBRL 實例文檔中添加腳註鏈接。
// 創建 XbrlDocument 類的實例
XbrlDocument document = new XbrlDocument();
// 獲取 XbrlInstances
XbrlInstanceCollection xbrlInstances = document.XbrlInstances;
// 添加 XbrlInstance
xbrlInstances.Add();
XbrlInstance xbrlInstance = xbrlInstances[0];
// 定義架構參考
SchemaRefCollection schemaRefs = xbrlInstance.SchemaRefs;
schemaRefs.Add(@"C:\Files\Finance\schema.xsd", "example", "http://example.com/xbrl/taxonomy");
SchemaRef schema = schemaRefs[0];
// 定義上下文
ContextPeriod contextPeriod = new ContextPeriod(DateTime.Parse("2020-01-01"), DateTime.Parse("2020-02-10"));
ContextEntity contextEntity = new ContextEntity("exampleIdentifierScheme", "exampleIdentifier");
Context context = new Context(contextPeriod, contextEntity);
context.Id = "cd1";
xbrlInstance.Contexts.Add(context);
// 定義腳註
Footnote footnote = new Footnote("footnote1");
footnote.Text = "Including the effects of the merger.";
// 定義定位器
Loc loc = new Loc("#cd1", "fact1");
// 定義腳註Arc
FootnoteArc footnoteArc = new FootnoteArc(loc.Label, footnote.Label);
// 定義腳註 link
FootnoteLink footnoteLink = new FootnoteLink();
footnoteLink.Footnotes.Add(footnote);
footnoteLink.Locators.Add(loc);
footnoteLink.FootnoteArcs.Add(footnoteArc);
xbrlInstance.FootnoteLinks.Add(footnoteLink);
// 保存文檔
document.Save(@"C:\Files\Finance\document6.xbrl");
使用 C# 將角色引用對象添加到 XBRL
我們可以按照以下步驟在 XBRL 實例文檔中添加角色引用:
- 首先,創建 XbrlDocument 類的一個實例。
- 接下來,將一個新的 XBRL 實例添加到 XbrlDocument 對象的實例中。
- 然後,將新的架構引用添加到 XbrlInstance 對象的架構引用。
- 通過 SchemaRefCollection 的索引獲取 SchemaRef。
- 接下來,從 GetRoleTypeByURI() 方法獲取 RoleType。
- 然後,使用 RoleType 對像作為參數創建 RoleReference 類的實例。
- 之後,將 RoleReference 添加到 RoleReference 對象集合中。
- 最後,使用 XbrlDocument.Save() 方法保存 XBRL 文件。它以輸出文件路徑作為參數。
以下代碼示例顯示如何使用 C# 在 XBRL 實例文檔中添加角色引用。
// 創建 XbrlDocument 類的實例
XbrlDocument document = new XbrlDocument();
// 獲取 XbrlInstances
XbrlInstanceCollection xbrlInstances = document.XbrlInstances;
// 添加 XbrlInstance
xbrlInstances.Add();
XbrlInstance xbrlInstance = xbrlInstances[0];
// 定義架構參考
SchemaRefCollection schemaRefs = xbrlInstance.SchemaRefs;
schemaRefs.Add(@"C:\Files\Finance\schema.xsd", "example", "http://example.com/xbrl/taxonomy");
SchemaRef schema = schemaRefs[0];
// 添加角色參考
RoleType roleType = schema.GetRoleTypeByURI("http://abc.com/role/link1");
if (roleType != null)
{
RoleReference roleReference = new RoleReference(roleType);
xbrlInstance.RoleReferences.Add(roleReference);
}
// 保存文件
document.Save(@"C:\Files\Finance\document7.xbrl");
使用 C# 將 Arc 角色參考對象添加到 XBRL
我們可以按照以下步驟在 XBRL 實例文檔中添加 Arc 角色引用:
- 首先,創建 XbrlDocument 類的一個實例。
- 接下來,將一個新的 XBRL 實例添加到 XbrlDocument 對象的實例中。
- 然後,將新的架構引用添加到 XbrlInstance 對象的架構引用。
- 接下來,通過 SchemaRefCollection 中的索引獲取 SchemaRef。
- 然後,從 GetArcroleTypeByURI() 方法獲取 ArcRoleType。
- 接下來,使用 ArcRoleType 對像作為參數創建 ArcRoleReference 類的實例。
- 之後,將 ArcRoleReference 添加到 ArcRoleReference 對象集合。
- 最後,使用 XbrlDocument.Save() 方法保存 XBRL 文件。它以輸出文件路徑作為參數。
以下代碼示例顯示如何使用 C# 在 XBRL 實例文檔中添加弧角色引用。
// 創建 XbrlDocument 類的實例
XbrlDocument document = new XbrlDocument();
// 獲取 XbrlInstances
XbrlInstanceCollection xbrlInstances = document.XbrlInstances;
// 添加 XbrlInstance
xbrlInstances.Add();
XbrlInstance xbrlInstance = xbrlInstances[0];
// 定義架構參考
SchemaRefCollection schemaRefs = xbrlInstance.SchemaRefs;
schemaRefs.Add(@"C:\Files\Finance\schema.xsd", "example", "http://example.com/xbrl/taxonomy");
SchemaRef schema = schemaRefs[0];
// 添加 Arc 角色參考
ArcroleType arcroleType = schema.GetArcroleTypeByURI("http://abc.com/arcrole/footnote-test");
if (arcroleType != null)
{
ArcroleReference arcroleReference = new ArcroleReference(arcroleType);
xbrlInstance.ArcroleReferences.Add(arcroleReference);
}
// 保存文件
document.Save(@"C:\Files\Finance\document8.xbrl");
獲得免費許可證
您可以 獲得免費的臨時許可證 來試用該庫,而沒有評估限制。
結論
在本文中,我們學習瞭如何:
- 使用 C# 創建 XBRL 文檔;
- 使用腳註鏈接以編程方式向 XBRL 對象添加腳註和腳註弧;
- 在 C# 中的 XBRL 中添加角色和 arc 角色引用。
此外,您可以使用 文檔 了解有關 Aspose.Finance for .NET API 的更多信息。如有任何歧義,請隨時在論壇上與我們聯繫。