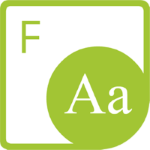
在數字 typography 中,字體定義了用於字符外觀的特定樣式。大多數情況下,字體在文檔和網頁中用於使文本風格化。每種字體都在一個文件中進行描述,該文件包含有關字符大小、粗細、樣式以及編碼的信息。由於字體是各種文件格式的重要組成部分,Aspose 提供了一個專用 API 來處理流行字體類型的操作和渲染,包括 TrueType、CFF、OpenType、和 Type1。在本文中,您將學習如何使用 C# 和 Aspose.Font for .NET 從字體中加載、保存和提取信息。
.NET 字體操作 API - 免費下載
Aspose.Font for .NET 是一種預置型 API,可讓您在 .NET 應用程序中使用 C# 實現字體管理功能。除了加載、保存和從字體中提取信息外,API 還允許您在需要時呈現字形或文本。您可以 下載 API 或使用 NuGet 在您的 .NET 應用程序中安裝它。
PM> Install-Package Aspose.Font
使用 C# 從文件加載或保存字體
您可以從存儲在數字存儲器上的文件中加載字體以檢索字體信息。 Aspose.Font for .NET 公開了單獨的類來處理特定類型的字體,即CFF、TrueType 等。以下是加載和保存字體的步驟。
- 使用 FontDefinition 類從文件加載字體(使用文件路徑或字節數組)。
- 使用 CffFont、TtfFont 或 Type1Font 類訪問字體信息。
- 保存文件(如果需要)。
加載和保存 CFF 字體
下面的代碼示例展示瞭如何使用 C# 加載和保存 CFF 字體。
// 如需完整示例和數據文件,請訪問 https://github.com/aspose-font/Aspose.Font-for-.NET
//從中加載字體的字節數組
string dataDir = RunExamples.GetDataDir_Data();
byte[] fontMemoryData = File.ReadAllBytes(dataDir + "OpenSans-Regular.cff");
FontDefinition fd = new FontDefinition(FontType.CFF, new FontFileDefinition("cff", new ByteContentStreamSource(fontMemoryData)));
CffFont cffFont = Aspose.Font.Font.Open(fd) as CffFont;
//使用剛剛加載的 CffFont 對像中的數據
//將 CffFont 保存到磁盤
//帶完整路徑的輸出字體文件名
string outputFile = RunExamples.GetDataDir_Data() + "OpenSans-Regular_out.cff";
cffFont.Save(outputFile);
加載和保存 TrueType 字體
下面的代碼示例顯示瞭如何使用 C# 加載和保存 TrueType 字體。
// 如需完整示例和數據文件,請訪問 https://github.com/aspose-font/Aspose.Font-for-.NET
//從中加載字體的字節數組
string dataDir = RunExamples.GetDataDir_Data();
byte[] fontMemoryData = File.ReadAllBytes(dataDir + "Montserrat-Regular.ttf");
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new ByteContentStreamSource(fontMemoryData)));
TtfFont ttfFont = Aspose.Font.Font.Open(fd) as TtfFont;
//使用剛剛加載的 TtfFont 對象的數據
//將 TtfFont 保存到磁盤
//帶完整路徑的輸出字體文件名
string outputFile = RunExamples.GetDataDir_Data() + "Montserrat-Regular_out.ttf";
ttfFont.Save(outputFile);
加載和保存 Type1 字體
下面的代碼示例顯示瞭如何使用 C# 加載和保存 Type1 字體。
// 如需完整示例和數據文件,請訪問 https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = Aspose.Font.Font.Open(fd) as Type1Font;
使用 C# 提取字體指標
Aspose.Font for .NET 還允許您檢索字體規格信息,例如 Ascender、Descender、TypoAscender、TypoDescender 和 UnitsPerEm。以下是執行此操作的步驟。
- 使用 FontDefinition 類從文件中加載 TrueType 或 Type1 字體。
- 使用相應的字體類型類打開字體,即 Type1Font、TtfFont 等。
- 訪問字體的規格信息。
以下代碼示例展示瞭如何使用 C# 從 TrueType 和 Type1 字體中提取字體指標。
從 TrueType 字體中提取指標
// 如需完整示例和數據文件,請訪問 https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont font = Aspose.Font.Font.Open(fd) as TtfFont;
string name = font.FontName;
Console.WriteLine("Font name: " + name);
Console.WriteLine("Glyph count: " + font.NumGlyphs);
string metrics = string.Format(
"Font metrics: ascender - {0}, descender - {1}, typo ascender = {2}, typo descender = {3}, UnitsPerEm = {4}",
font.Metrics.Ascender, font.Metrics.Descender,
font.Metrics.TypoAscender, font.Metrics.TypoDescender, font.Metrics.UnitsPerEM);
Console.WriteLine(metrics);
//從字體中獲取 cmap unicode 編碼表作為對象 TtfCMapFormatBaseTable 以訪問有關符號“A”的字體字形的信息。
//還要檢查字體是否具有對象 TtfGlyfTable(表“glyf”)以訪問字形。
Aspose.Font.TtfCMapFormats.TtfCMapFormatBaseTable cmapTable = null;
if (font.TtfTables.CMapTable != null)
{
cmapTable = font.TtfTables.CMapTable.FindUnicodeTable();
}
if (cmapTable != null && font.TtfTables.GlyfTable != null)
{
Console.WriteLine("Font cmap unicode table: PlatformID = " + cmapTable.PlatformId + ", PlatformSpecificID = " + cmapTable.PlatformSpecificId);
//“A”符號的代碼
char unicode = (char)65;
//“A”的字形索引
uint glIndex = cmapTable.GetGlyphIndex(unicode);
if (glIndex != 0)
{
//“A”的字形
Glyph glyph = font.GetGlyphById(glIndex);
if (glyph != null)
{
//打印字形指標
Console.WriteLine("Glyph metrics for 'A' symbol:");
string bbox = string.Format(
"Glyph BBox: Xmin = {0}, Xmax = {1}" + ", Ymin = {2}, Ymax = {3}",
glyph.GlyphBBox.XMin, glyph.GlyphBBox.XMax,
glyph.GlyphBBox.YMin, glyph.GlyphBBox.YMax);
Console.WriteLine(bbox);
Console.WriteLine("Width:" + font.Metrics.GetGlyphWidth(new GlyphUInt32Id(glIndex)));
}
}
}
從 Type1 字體中提取指標
// 如需完整示例和數據文件,請訪問 https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = Aspose.Font.Font.Open(fd) as Type1Font;
string name = font.FontName;
Console.WriteLine("Font name: " + name);
Console.WriteLine("Glyph count: " + font.NumGlyphs);
string metrics = string.Format(
"Font metrics: ascender - {0}, descender - {1}, typo ascender = {2}, typo descender = {3}, UnitsPerEm = {4}",
font.Metrics.Ascender, font.Metrics.Descender,
font.Metrics.TypoAscender, font.Metrics.TypoDescender, font.Metrics.UnitsPerEM);
Console.WriteLine(metrics);
使用 C# 檢測字體中的拉丁符號
您還可以通過使用 Aspose.Font for .NET 解碼字形代碼來檢測字體中的拉丁文本。以下是執行此操作的步驟。
- 使用 FontDefinition 類從文件加載字體。
- 使用相應字體類的 DecodeToGid() 方法解碼 GlyphId。
以下代碼示例顯示如何使用 C# 解碼 TrueType 和 Type1 字體中的拉丁符號。
檢測 TrueType 字體中的拉丁符號
// 如需完整示例和數據文件,請訪問 https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont ttfFont = Aspose.Font.Font.Open(fd) as TtfFont;
bool latinText = true;
for (uint code = 65; code < 123; code++)
{
GlyphId gid = ttfFont.Encoding.DecodeToGid(code);
if (gid == null || gid == GlyphUInt32Id.NotDefId)
{
latinText = false;
}
}
if (latinText)
{
Console.WriteLine(string.Format("Font {0} supports latin symbols.", ttfFont.FontName));
}
else
{
Console.WriteLine(string.Format("Latin symbols are not supported by font {0}.", ttfFont.FontName));
}
檢測 Type1 字體中的拉丁符號
// 如需完整示例和數據文件,請訪問 https://github.com/aspose-font/Aspose.Font-for-.NET
string fileName = dataDir + "courier.pfb"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new FileSystemStreamSource(fileName)));
Type1Font font = Aspose.Font.Font.Open(fd) as Type1Font;
bool latinText = true;
for (uint code = 65; code < 123; code++)
{
GlyphId gid = font.Encoding.DecodeToGid(code);
if (gid == null || gid == GlyphUInt32Id.NotDefId)
{
latinText = false;
}
}
if (latinText)
{
Console.WriteLine(string.Format("Font {0} supports latin symbols.", font.FontName));
}
else
{
Console.WriteLine(string.Format("Latin symbols are not supported by font {0}.", font.FontName));
}
結論
在本文中,您了解瞭如何使用 C# 以編程方式加載和保存 CFF、TrueType 和 Type1 字體。此外,您還學習瞭如何從 .NET 應用程序中的 TrueType 和 Type1 字體中提取字體規格信息。您可以在 文檔 中探索 Aspose.Font for .NET 提供的更多有趣功能。如需更多更新,請繼續訪問 Aspose.Font 博客。