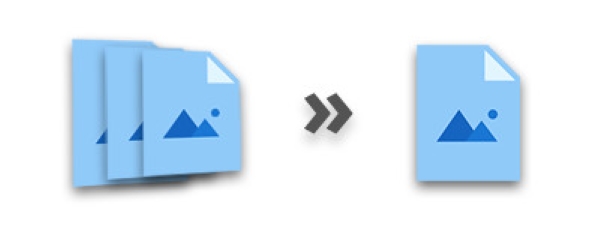
在許多場景中,圖像編輯和操作都是以編程方式執行的。在圖像編輯中,合併圖像是一個重要的功能,用於組合兩個或多個圖像,例如製作拼貼畫。在本文中,您將學習如何在 Java 中將多個圖像合併為一個圖像。我們將明確演示如何水平和垂直合併圖像。
用於合併圖像的 Java API - 免費下載
Aspose.Imaging for Java 是一個功能強大的圖像處理 API,可讓您處理各種圖像格式。它提供了圖像編輯所需的各種功能。在這篇博文中,我們將使用此 API 來合併我們的圖像。您可以 下載 API 或使用以下 Maven 配置安裝它。
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-imaging</artifactId>
<version>22.7</version>
</dependency>
在 Java 中合併多個圖像
您可以通過兩種方式之一合併圖像:垂直和水平。在垂直合併中,圖像在垂直方向上一張一張地合併。而在水平合併中,圖像在水平方向上相互附加。那麼讓我們看看如何以兩種方式合併圖像。
在 Java 中水平合併圖像
以下是使用 Java 水平合併圖像的步驟。
- 首先,在字符串數組中指定圖像的路徑。
- 計算結果圖像的高度和寬度。
- 創建一個 JpegOptions 類的對象並設置所需的選項。
- 創建一個 JpegImage 類的對象,並使用 JpegOptions 對象和結果圖像的高度和寬度對其進行初始化。
- 遍歷圖像列表並使用 RasterImage 類加載每個圖像。
- 為每個圖像創建一個 Rectangle 並使用 JpegImage.saveArgb32Pixels() 方法將其添加到結果圖像中。
- 在每次迭代中增加縫合寬度。
- 完成後,使用 JpegImage.save(string) 方法保存生成的圖像。
以下代碼示例顯示瞭如何在 Java 中水平合併圖像。
// 圖片一覽
String[] imagePaths = { "image.jpg", "image.jpg" };
// 輸出圖片路徑
String outputPath = "output-horizontal.jpg";
String tempFilePath = "temp.jpg";
// 獲取生成的圖像大小
int newWidth = 0;
int newHeight = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (com.aspose.imaging.RasterImage) com.aspose.imaging.Image.load(imagePath)) {
Size size = image.getSize();
newWidth += size.getWidth();
newHeight = Math.max(newHeight, size.getHeight());
}
}
// 將圖像組合成新圖像
try (JpegOptions options = new JpegOptions()) {
Source tempFileSource = new FileCreateSource(tempFilePath, true);
options.setSource(tempFileSource);
options.setQuality(100);
// 創建結果圖像
try (JpegImage newImage = (JpegImage) Image.create(options, newWidth, newHeight)) {
int stitchedWidth = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (RasterImage) Image.load(imagePath)) {
Rectangle bounds = new Rectangle(stitchedWidth, 0, image.getWidth(), image.getHeight());
newImage.saveArgb32Pixels(bounds, image.loadArgb32Pixels(image.getBounds()));
stitchedWidth += image.getWidth();
}
}
// 保存輸出圖像
newImage.save(outputPath);
}
}
下圖顯示了水平合併兩個相似圖像的輸出。
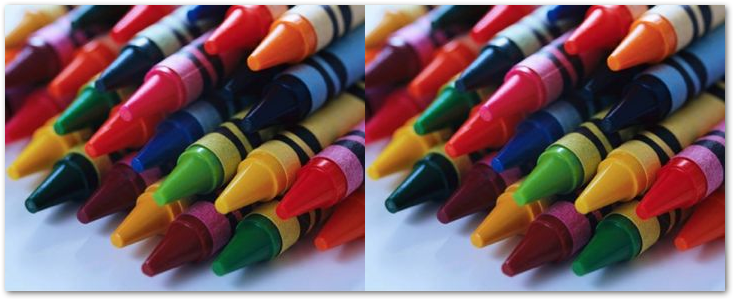
在 Java 中垂直合併圖像
要垂直合併圖像,只需要切換高度和寬度屬性的作用即可。其餘代碼將相同。下面的代碼示例演示瞭如何在 Java 中垂直合併多個圖像。
// 圖片一覽
String[] imagePaths = { "image.jpg", "image.jpg" };
// 輸出圖像的路徑
String outputPath = "output-vertical.jpg";
// 獲取生成的圖像大小
int newWidth = 0;
int newHeight = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (RasterImage) Image.load(imagePath)) {
Size size = image.getSize();
newWidth = Math.max(newWidth, size.getWidth());
newHeight += size.getHeight();
}
}
// 將圖像組合成新圖像
try (JpegOptions options = new JpegOptions()) {
options.setSource(new StreamSource()); // empty
options.setQuality(100);
// 創建結果圖像
try (JpegImage newImage = (JpegImage) Image.create(options, newWidth, newHeight)) {
int stitchedHeight = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (RasterImage) Image.load(imagePath)) {
Rectangle bounds = new Rectangle(0, stitchedHeight, image.getWidth(), image.getHeight());
newImage.saveArgb32Pixels(bounds, image.loadArgb32Pixels(image.getBounds()));
stitchedHeight += image.getHeight();
}
}
// 保存生成的圖像
newImage.save(outputPath);
}
}
下圖顯示了垂直合併兩個相似圖像的輸出。
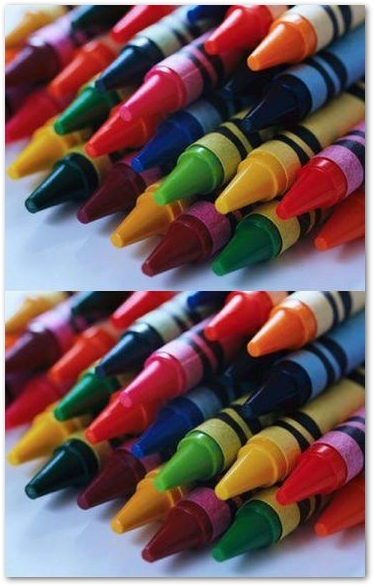
在 Java 中合併 PNG 圖像
在前面的部分中,我們演示瞭如何合併 JPG 格式的圖像。但是,您可能還需要合併 PNG 格式的圖像。要合併 PNG 圖像,只需將 JpegImage 和 JpegOptions 類分別替換為 PngImage 和 PngOptions 類,其餘代碼將保持不變。
以下代碼示例顯示瞭如何在 Java 中合併多個 PNG 格式的圖像。
// 圖片一覽
String[] imagePaths = { "image.png", "image.png" };
// 輸出圖片路徑
String outputPath = "output-horizontal.png";
String tempFilePath = "temp.png";
// 獲取生成的圖像大小
int newWidth = 0;
int newHeight = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (com.aspose.imaging.RasterImage) com.aspose.imaging.Image.load(imagePath)) {
Size size = image.getSize();
newWidth += size.getWidth();
newHeight = Math.max(newHeight, size.getHeight());
}
}
// 將圖像組合成新圖像
try (PngOptions options = new PngOptions()) {
Source tempFileSource = new FileCreateSource(tempFilePath, true);
options.setSource(tempFileSource);
// 創建結果圖像
try (PngImage newImage = (PngImage) Image.create(options, newWidth, newHeight)) {
int stitchedWidth = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (RasterImage) Image.load(imagePath)) {
Rectangle bounds = new Rectangle(stitchedWidth, 0, image.getWidth(), image.getHeight());
newImage.saveArgb32Pixels(bounds, image.loadArgb32Pixels(image.getBounds()));
stitchedWidth += image.getWidth();
}
}
// 保存圖片
newImage.save(outputPath);
}
}
Java 圖像合併 API - 獲得免費許可證
您可以 獲得免費的臨時許可證 並在沒有評估限制的情況下合併圖像。
結論
在本文中,您學習瞭如何使用 Java 將多個圖像合併為一個圖像。 Java 代碼示例演示瞭如何垂直和水平組合圖像。此外,您可以使用 文檔 探索有關 Java 圖像處理 API 的更多信息。此外,您可以通過我們的 論壇 與我們分享您的疑問。
也可以看看
信息:Aspose 提供了一個免費的拼貼網絡應用程序。使用此在線服務,您可以合併 JPG 到 JPG 或 PNG 到 PNG 圖像,創建照片網格,等等。