
圖像編輯廣泛用於提高圖像質量。隨著移動相機和圖像編輯應用程序的出現,每個移動用戶都知道如何編輯圖像。在其他圖像編輯功能中,創建拼貼是一種流行的功能,其中將多個圖像組合成單個圖像。因此,在本文中,我們將演示如何在 Python 中合併多個圖像。如果您正在 Python 應用程序中處理圖像編輯,這可能對您很有用。
用於合併圖像的 Python 庫
要將多個圖像合併為單個圖像,我們將使用 Aspose.Imaging for Python。它是一個功能豐富的圖像處理庫,可以輕鬆執行多種圖像編輯操作。您可以下載該庫或使用以下命令安裝它。
> pip install aspose-imaging-python-net
在 Python 中合併多個圖像
合併圖像有兩種方式:垂直合併和水平合併。您可以選擇合適的方法。讓我們在下面的部分中看看如何以兩種方式組合圖像。
在Python中水平合併圖像
以下是使用 Aspose.Imaging for Python 水平合併圖像的步驟。
- 首先指定數組中圖像的路徑。
- 然後,計算所得圖像的高度和寬度。
- 創建 JpegOptions 類的對象並設置所需的選項。
- 創建 JpegImage 類的對象,並使用 JpegOptions 對像以及生成圖像的高度和寬度對其進行初始化。
- 循環遍歷圖像列表並使用 RasterImage 類加載每個圖像。
- 為每個圖像創建一個矩形,並使用 JpegImage.saveargb32pixels() 方法將其添加到結果圖像中。
- 增加每次迭代中的縫合寬度。
- 最後,使用 JpegImage.save(string) 方法保存生成的圖像。
下面是Python中水平合併圖像的代碼。
import aspose.pycore as aspycore
from aspose.imaging import Image, Rectangle, RasterImage
from aspose.imaging.imageoptions import JpegOptions
from aspose.imaging.sources import FileCreateSource
from aspose.imaging.fileformats.jpeg import JpegImage
import os
# 定義文件夾
if 'TEMPLATE_DIR' in os.environ:
templates_folder = os.environ['TEMPLATE_DIR']
else:
templates_folder = r"C:\Users\USER\Downloads\templates"
delete_output = 'SAVE_OUTPUT' not in os.environ
data_dir = templates_folder
image_paths = [os.path.join(data_dir, "template.jpg"),
os.path.join(data_dir, "template.jpeg")]
output_path = os.path.join(data_dir, "result.jpg")
temp_file_path = os.path.join(data_dir, "temp.jpg")
# 獲取結果圖像大小
image_sizes = []
for image_path in image_paths:
with Image.load(image_path) as image:
image_sizes.append(image.size)
# 計算新尺寸
new_width = 0
new_height = 0
for size in image_sizes:
new_width += size.width
new_height = max(new_height, size.height)
# 將圖像合併為新圖像
temp_file_source = FileCreateSource(temp_file_path, delete_output)
with JpegOptions() as options:
options.source = temp_file_source
options.quality = 100
with aspycore.as_of(Image.create(options, new_width, new_height), JpegImage) as new_image:
stitched_width = 0
for image_path in image_paths:
with aspycore.as_of(Image.load(image_path), RasterImage) as image:
bounds = Rectangle(stitched_width, 0, image.width, image.height)
new_image.save_argb_32_pixels(bounds, image.load_argb_32_pixels(image.bounds))
stitched_width += image.width
new_image.save(output_path)
# 刪除臨時文件
if delete_output:
os.remove(output_path)
if os.path.exists(temp_file_path):
os.remove(temp_file_path)
下面是我們水平合併圖像後得到的輸出圖像。

在 Python 中垂直組合圖像
現在讓我們看看如何垂直合併多個圖像。垂直合併圖像的步驟與上一節中的相同。唯一的區別是,我們會交換高度和寬度屬性的角色。
以下代碼示例展示瞭如何在 Python 中合併垂直佈局中的圖像。
import aspose.pycore as aspycore
from aspose.imaging import Image, Rectangle, RasterImage
from aspose.imaging.imageoptions import JpegOptions
from aspose.imaging.sources import StreamSource
from aspose.imaging.fileformats.jpeg import JpegImage
from aspose.imaging.extensions import StreamExtensions
import os
import functools
# 定義文件夾
if 'TEMPLATE_DIR' in os.environ:
templates_folder = os.environ['TEMPLATE_DIR']
else:
templates_folder = r"C:\Users\USER\Downloads\templates"
delete_output = 'SAVE_OUTPUT' not in os.environ
data_dir = templates_folder
image_paths = [os.path.join(data_dir, "template.jpg"), os.path.join(data_dir, "template.jpeg")]
output_path = os.path.join(data_dir, "result.jpg")
temp_file_path = os.path.join(data_dir, "temp.jpg")
# 獲取結果圖像大小
image_sizes = []
for image_path in image_paths:
with Image.load(image_path) as image:
image_sizes.append(image.size)
# 計算新尺寸
new_width = 0
new_height = 0
for size in image_sizes:
new_height += size.height
new_width = max(new_width, size.width)
# 將圖像合併為新圖像
with StreamExtensions.create_memory_stream() as memory_stream:
output_stream_source = StreamSource(memory_stream)
with JpegOptions() as options:
options.source = output_stream_source
options.quality = 100
with aspycore.as_of(Image.create(options, new_width, new_height), JpegImage) as new_image:
stitched_height = 0
for image_path in image_paths:
with aspycore.as_of(Image.load(image_path), RasterImage) as image:
bounds = Rectangle(0, stitched_height, image.width, image.height)
new_image.save_argb_32_pixels(bounds, image.load_argb_32_pixels(image.bounds))
stitched_height += image.height
new_image.save(output_path)
# 刪除臨時文件
if delete_output:
os.remove(output_path)
下圖顯示了垂直合併兩個相似圖像的輸出。

在 Python 中合併 PNG 圖像
前面幾節中提供的步驟和代碼示例用於合併 JPG 圖像,但是,您可能還需要合併 PNG 圖像。不用擔心。相同的步驟和代碼示例對於 PNG 圖像有效,唯一的更改是分別使用 PngImage 和 PngOptions 類而不是 JpegImage 和 JpegOptions。
獲取免費的 Python 圖像合併庫
您可以獲取免費的臨時許可證並合併圖像,而不受評估限制。
在線合併圖像
您還可以使用我們的免費圖像合併工具在線合併您的圖像。該工具基於 Aspose.Imaging for Python,您無需為其創建帳戶。
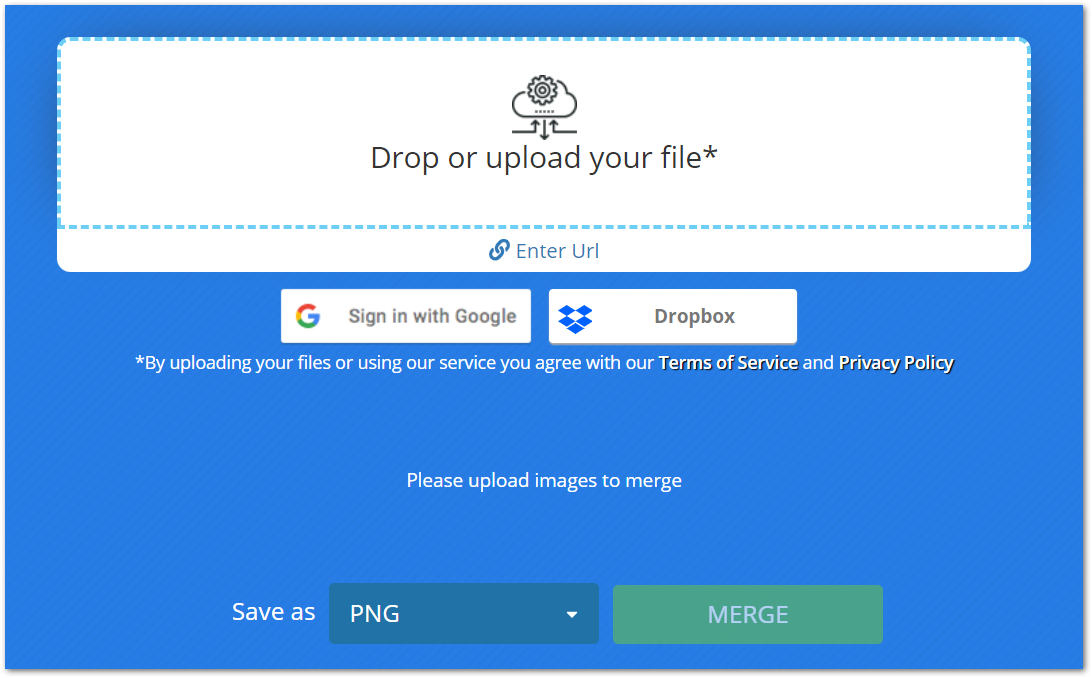
結論
本文為您提供了在 Python 中將多個圖像合併為單個圖像的最佳和最簡單的解決方案之一。借助代碼示例演示了水平和垂直圖像合併。此外,我們還向您介紹了一個免費合併圖像的在線工具。
您可以通過訪問文檔探索有關Python圖像處理庫的更多信息。此外,您還可以通過我們的論壇與我們分享您的疑問。