
XPS(Open XML 紙張規格) 和 OXPS (OpenXPS) 表示固定頁面文件。 XPS 文件與 PDF 文件類似,都保留原始文件的佈局和格式。但是,XPS 文件不像 PDF 文件那樣得到廣泛支援。 PDF(便攜式文件格式)文件在各種平台和裝置上廣泛支援。在本文中,我們將向您展示如何使用 C# 將 XPS 或 OXPS 轉換為 PDF。
本文涵蓋以下主題:
- 將 XPS 轉換為 PDF 的 C# API
- 使用 C# 將 XPS 轉換為 PDF
- 將 XPS 的特定頁面轉換為 PDF
- C# 中的 OXPS 到 PDF 轉換器
- 將 OXPS 的特定頁面轉換為 PDF
- 線上將XPS檔轉換為PDF
- XPS 轉 PDF - 免費學習資源
將 XPS 轉換為 PDF 的 C# API
我們將使用 Aspose.Page for .NET API 將 XPS 或 OXPS 文件轉換為 PDF 格式。它是一個功能強大的 API,使開發人員能夠創建、編輯、操作和轉換 XPS 和 EPS/PS 文件。 Aspose.Page for .NET 是一個可靠且獨立的 API,易於使用並整合到您的 .NET 應用程式中。
請下載 API 的 DLL 或使用以下 NuGet 指令安裝它:
PM> Install-Package Aspose.Page
使用 C# 將 XPS 轉換為 PDF
我們可以按照以下步驟將整個 XPS 文件轉換為 PDF 格式:
- 載入輸入 XPS 檔案。
- 使用必要的參數初始化選項物件。
- 建立 PdfDevice 的實例以進行渲染。
- 將 XPS 匯出為 PDF 文件。
下面給出的程式碼範例展示如何使用 C# 將 XPS 檔案轉換為 PDF。
// 初始化 PDF 輸出流
using (System.IO.Stream pdfStream = System.IO.File.Open(dataDir + "XPStoPDF.pdf", System.IO.FileMode.OpenOrCreate, System.IO.FileAccess.Write))
// 初始化XPS輸入流
//使用 (System.IO.Stream xpsStream = System.IO.File.Open(dataDir + "input.xps", System.IO.FileMode.Open))
using (System.IO.Stream xpsStream = System.IO.File.Open(dataDir + "sample.xps", System.IO.FileMode.Open))
{
// 從流程載入 XPS 文檔
Aspose.Page.XPS.XpsDocument document = new Aspose.Page.XPS.XpsDocument(xpsStream, new Aspose.Page.XPS.XpsLoadOptions());
// 或直接從文件載入 XPS 文件。則不需要 xpsStream。
// XpsDocument 文件 = new XpsDocument(inputFileName, new XpsLoadOptions());
// 使用必要的參數初始化選項物件。
Aspose.Page.XPS.Presentation.Pdf.PdfSaveOptions options = new Aspose.Page.XPS.Presentation.Pdf.PdfSaveOptions()
{
JpegQualityLevel = 100,
ImageCompression = Aspose.Page.XPS.Presentation.Pdf.PdfImageCompression.Jpeg,
TextCompression = Aspose.Page.XPS.Presentation.Pdf.PdfTextCompression.Flate,
};
// 建立PDF格式的渲染設備
Aspose.Page.XPS.Presentation.Pdf.PdfDevice device = new Aspose.Page.XPS.Presentation.Pdf.PdfDevice(pdfStream);
document.Save(device, options);
}
在 C# 中將 XPS 的特定頁面轉換為 PDF
我們也可以按照以下步驟將 XPS 文件的選定頁面轉換為 PDF 格式:
- 初始化 XPS 輸入流。
- 從串流載入 XPS 文件。
- 初始化 PdfSaveOptions 物件。
- 指定轉換的頁碼。
- 將文件另存為 PDF 文件。
以下程式碼範例示範如何使用 C# 將 XPS 的特定頁面轉換為 PDF。
// 初始化 PDF 輸出流
using (System.IO.Stream pdfStream = System.IO.File.Open(dataDir + "XPStoPDF.pdf", System.IO.FileMode.OpenOrCreate, System.IO.FileAccess.Write))
// 初始化XPS輸入流
//使用 (System.IO.Stream xpsStream = System.IO.File.Open(dataDir + "input.xps", System.IO.FileMode.Open))
using (System.IO.Stream xpsStream = System.IO.File.Open(dataDir + "sample.xps", System.IO.FileMode.Open))
{
// 從流程載入 XPS 文檔
Aspose.Page.XPS.XpsDocument document = new Aspose.Page.XPS.XpsDocument(xpsStream, new Aspose.Page.XPS.XpsLoadOptions());
// 或直接從文件載入 XPS 文件。則不需要 xpsStream。
// XpsDocument 文件 = new XpsDocument(inputFileName, new XpsLoadOptions());
// 使用必要的參數初始化選項物件。
Aspose.Page.XPS.Presentation.Pdf.PdfSaveOptions options = new Aspose.Page.XPS.Presentation.Pdf.PdfSaveOptions()
{
JpegQualityLevel = 100,
ImageCompression = Aspose.Page.XPS.Presentation.Pdf.PdfImageCompression.Jpeg,
TextCompression = Aspose.Page.XPS.Presentation.Pdf.PdfTextCompression.Flate,
PageNumbers = new int[] {1, 3}
};
// 建立PDF格式的渲染設備
Aspose.Page.XPS.Presentation.Pdf.PdfDevice device = new Aspose.Page.XPS.Presentation.Pdf.PdfDevice(pdfStream);
document.Save(device, options);
}
此程式碼片段使用包含多個頁面的 XPS 文件作為輸入檔。然而,只有頁碼 1 和 3 會依照程式碼片段中的指定轉換為 PDF。以下螢幕截圖顯示了呈現為 PDF 文件的兩個頁面:
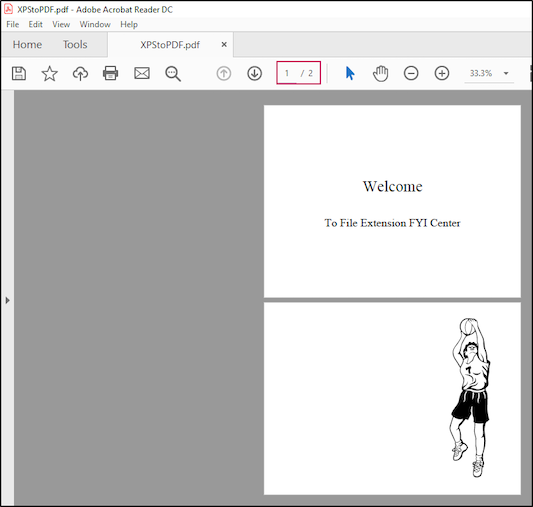
C# 中的 OXPS 到 PDF 轉換器
OXPS 格式是 XPS 檔案格式的更新和進階形式。但是,某些舊作業系統不支援此類檔案。我們可以按照以下步驟輕鬆將 OXPS 文件轉換為 PDF 格式:
- 初始化 OXPS 輸入流。
- 從流程載入 OXPS 檔案。
- 實例化 PdfSaveOptions 類別的對象
- 將 OXPS 匯出為 PDF 文件
以下程式碼範例展示如何使用 C# 將 OXPS 轉換為 PDF。
// 初始化 PDF 輸出流
using (System.IO.Stream pdfStream = System.IO.File.Open(dataDir + "OXPStoPDF.pdf", System.IO.FileMode.OpenOrCreate, System.IO.FileAccess.Write))
// 初始化OXPS輸入流
//使用 (System.IO.Stream xpsStream = System.IO.File.Open(dataDir + "input.oxps", System.IO.FileMode.Open))
using (System.IO.Stream xpsStream = System.IO.File.Open(dataDir + "sample.oxps", System.IO.FileMode.Open))
{
// 從流程載入 OXPS 文檔
Aspose.Page.XPS.XpsDocument document = new Aspose.Page.XPS.XpsDocument(xpsStream, new Aspose.Page.XPS.XpsLoadOptions());
// 或直接從檔案載入 OXPS 文件。則不需要 xpsStream。
// XpsDocument 文件 = new XpsDocument(inputFileName, new XpsLoadOptions());
// 使用必要的參數初始化選項物件。
Aspose.Page.XPS.Presentation.Pdf.PdfSaveOptions options = new Aspose.Page.XPS.Presentation.Pdf.PdfSaveOptions()
{
JpegQualityLevel = 100,
ImageCompression = Aspose.Page.XPS.Presentation.Pdf.PdfImageCompression.Jpeg,
TextCompression = Aspose.Page.XPS.Presentation.Pdf.PdfTextCompression.Flate,
};
// 建立PDF格式的渲染設備
Aspose.Page.XPS.Presentation.Pdf.PdfDevice device = new Aspose.Page.XPS.Presentation.Pdf.PdfDevice(pdfStream);
document.Save(device, options);
}
在 C# 中將 OXPS 的特定頁面轉換為 PDF
同樣,我們也可以按照以下步驟將特定頁面從 OXPS 文件轉換為 PDF 格式:
- 載入 OXPS 文件
- 聲明 PdfSaveOptions 物件。
- 設定要轉換的頁碼。
- 將 OXPS 渲染為 PDF。
以下程式碼片段展示如何使用 C# 將 OXPS 的特定頁面轉換為 PDF。它將 OXPS 檔案的第一頁轉換為 PDF,如程式碼片段中所述。
// 初始化 PDF 輸出流
using (System.IO.Stream pdfStream = System.IO.File.Open(dataDir + "OXPStoPDF.pdf", System.IO.FileMode.OpenOrCreate, System.IO.FileAccess.Write))
// 初始化OXPS輸入流
//使用 (System.IO.Stream xpsStream = System.IO.File.Open(dataDir + "input.oxps", System.IO.FileMode.Open))
using (System.IO.Stream xpsStream = System.IO.File.Open(dataDir + "sample.oxps", System.IO.FileMode.Open))
{
// 從流程載入 OXPS 文檔
Aspose.Page.XPS.XpsDocument document = new Aspose.Page.XPS.XpsDocument(xpsStream, new Aspose.Page.XPS.XpsLoadOptions());
// 或直接從文件載入 XPS 文件。則不需要 xpsStream。
// XpsDocument 文件 = new XpsDocument(inputFileName, new XpsLoadOptions());
// 使用必要的參數初始化選項物件。
Aspose.Page.XPS.Presentation.Pdf.PdfSaveOptions options = new Aspose.Page.XPS.Presentation.Pdf.PdfSaveOptions()
{
JpegQualityLevel = 100,
ImageCompression = Aspose.Page.XPS.Presentation.Pdf.PdfImageCompression.Jpeg,
TextCompression = Aspose.Page.XPS.Presentation.Pdf.PdfTextCompression.Flate,
PageNumbers = new int[] {1}
};
// 建立PDF格式的渲染設備
Aspose.Page.XPS.Presentation.Pdf.PdfDevice device = new Aspose.Page.XPS.Presentation.Pdf.PdfDevice(pdfStream);
document.Save(device, options);
}
取得免費許可證
您可以獲得免費的臨時許可證來測試該庫,而沒有任何評估限制。
在線將 XPS 檔案轉換為 PDF
您也可以使用此免費 XPS 到 PDF 轉換器 應用程式在線上將 XPS 檔案轉換為 PDF,而無需安裝任何軟體或外掛程式。
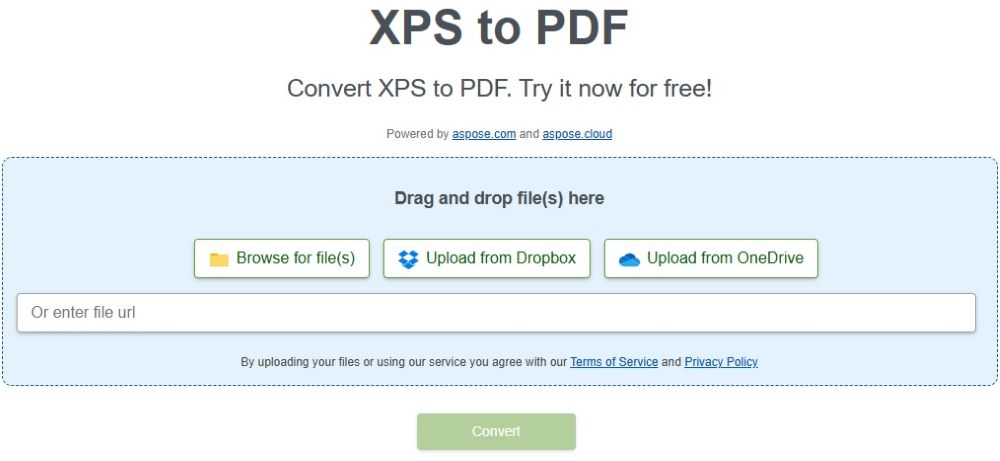
將 XPS 文件轉換為 PDF - 學習資源
除了將 XPS 或 OXPS 文件轉換為 PDF 格式之外,您還可以使用以下資源了解有關該程式庫的更多資訊並探索各種其他功能:
結論
在本文中,我們學習如何使用 C# 以程式設計方式將 XPS 轉換為 PDF 和 OXPS 轉換為 PDF。我們也了解如何將 XPS 或 OXPS 文件的特定頁面匯出為 PDF 格式。您可以進一步探索 API 並優化您的文件處理工作流程。如有任何疑問,請隨時透過我們的免費支援論壇與我們聯繫。