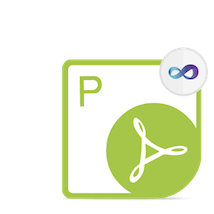
Byte Array 有助於存儲或傳輸數據。同樣,PDF 文件格式因其特性和兼容性而廣受歡迎。您可以使用 C# 語言將 PDF 文件轉換為字節數組,也可以將字節數組轉換為 PDF 文件。這可以幫助您更有效地在數據庫中存儲和存檔 PDF 文件。您還可以通過使用字節數組來序列化數據。讓我們探索這些格式的相互轉換。
PDF 文件到字節數組和字節數組到 PDF 文件的轉換 – API 安裝
Aspose.PDF for .NET API 提供了許多處理 PDF 文檔的功能。您可以通過簡單易行的 API 調用來創建、編輯、操作或轉換 PDF 文件。要將 PDF 文件轉換為字節數組或將 PDF 文件轉換為字節數組,您需要通過從 官方網站 下載或從 NuGet 庫下載它來安裝 API,在 Visual Studio IDE 中使用以下安裝命令。
PM> Install-Package Aspose.Pdf
使用 C# 將 PDF 文件轉換為字節數組
您可以將 PDF 轉換為字節數組,以便傳輸或存儲它以供進一步處理。例如,您可能需要序列化 PDF 文檔,然後將其轉換為字節數組會有所幫助。您需要按照以下步驟將 PDF 轉換為字節數組:
- 加載輸入 PDF 文件
- 初始化字節數組
- 初始化 FileStream 對象
- 加載字節數組中的文件內容
完成所有這些步驟後,現在您可以以字節數組的形式處理 PDF 文件。例如,您可以將它傳遞給另一個函數,如下例所示。
以下代碼顯示瞭如何使用 C# 將 PDF 文件轉換為字節數組,其中生成的 ByteArray 被傳遞給將輸入文件轉換為圖像的方法:
dataDir = @"D:\Test\";
// 加載輸入 PDF 文件
string inputFile = dataDir + @"testpdf.pdf";
// 初始化一個字節數組
byte[] buff = null;
// 初始化 FileStream 對象
FileStream fs = new FileStream(inputFile, FileMode.Open, FileAccess.Read);
BinaryReader br = new BinaryReader(fs);
long numBytes = new FileInfo(inputFile).Length;
// 加載字節數組中的文件內容
buff = br.ReadBytes((int) numBytes);
fs.Close();
// 使用字節數組中的 PDF 文件
ConvertPDFToJPEG(buff, 300, dataDir);
public static void ConvertPDFToJPEG(Byte[] PDFBlob, int resolution, string dataDir)
{
// 打開文檔
using (MemoryStream InputStream = new MemoryStream(PDFBlob))
{
Aspose.Pdf.Document pdfDocument = new Aspose.Pdf.Document(InputStream);
for (int pageCount = 1; pageCount <= pdfDocument.Pages.Count; pageCount++)
{
using (FileStream imageStream = new FileStream(dataDir + "image" + pageCount + "_out" + ".jpg", FileMode.Create))
{
// 創建具有指定屬性的 JPEG 設備
// 寬度、高度、分辨率、質量
// 質量 [0-100],100 是最大值
// 創建分辨率對象
Aspose.Pdf.Devices.Resolution res = new Aspose.Pdf.Devices.Resolution(resolution);
// JpegDevice jpegDevice = new JpegDevice(500, 700, 分辨率, 100);
// 添加以下內容以確定是否橫向
Int32 height, width = 0;
PdfFileInfo info = new PdfFileInfo(pdfDocument);
width = Convert.ToInt32(info.GetPageWidth(pdfDocument.Pages[pageCount].Number));
height = Convert.ToInt32(info.GetPageHeight(pdfDocument.Pages[pageCount].Number));
Aspose.Pdf.Devices.JpegDevice jpegDevice =
//新的 Aspose.Pdf.Devices.JpegDevice(Aspose.Pdf.PageSize.A4, res, 100);
new Aspose.Pdf.Devices.JpegDevice(width, height, res, 100);
// 轉換特定頁面並將圖像保存到流
//Aspose.Pdf.PageSize.A4.IsLandscape = true;
jpegDevice.Process(pdfDocument.Pages[pageCount], imageStream);
// 關閉流
imageStream.Close();
}
}
}
}
使用 C# 將字節數組轉換為 PDF 文件
讓我們更進一步,可以將字節數組轉換為 PDF 文件。讓我們通過將圖像作為字節數組轉換為 PDF 文件的示例來了解這一點。您需要按照以下步驟將字節數組轉換為 PDF 文件。
- 加載輸入文件
- 初始化字節數組
- 將輸入圖像加載到字節數組中
- 初始化 Document 類的一個實例
- 在 PDF 頁面上添加圖像
- 保存輸出 PDF 文件
以下代碼解釋瞭如何使用 C# 以編程方式將字節數組轉換為 PDF 文件:
// 加載輸入文件
string inputFile = dataDir + @"Test.PNG";
// 初始化字節數組
byte[] buff = null;
FileStream fs = new FileStream(inputFile, FileMode.Open, FileAccess.Read);
BinaryReader br = new BinaryReader(fs);
long numBytes = new FileInfo(inputFile).Length;
// 將輸入圖像加載到字節數組中
buff = br.ReadBytes((int)numBytes);
Document doc = new Document();
// 將頁面添加到文檔的頁面集合
Page page = doc.Pages.Add();
// 將源圖像文件加載到 Stream 對象
MemoryStream outstream = new MemoryStream();
MemoryStream mystream = new MemoryStream(buff);
// 使用加載的圖像流實例化 BitMap 對象
Bitmap b = new Bitmap(mystream);
// 設置邊距使圖像適合等。
page.PageInfo.Margin.Bottom = 0;
page.PageInfo.Margin.Top = 0;
page.PageInfo.Margin.Left = 0;
page.PageInfo.Margin.Right = 0;
page.CropBox = new Aspose.Pdf.Rectangle(0, 0, b.Width, b.Height);
// 創建圖像對象
Aspose.Pdf.Image image1 = new Aspose.Pdf.Image();
// 將圖像添加到該部分的段落集合中
page.Paragraphs.Add(image1);
// 設置圖像文件流
image1.ImageStream = mystream;
// 保存生成的 PDF 文件
doc.Save(outstream, SaveFormat.Pdf);
//doc.Save(dataDir + "outstream.pdf", SaveFormat.Pdf);
// 關閉內存流對象
mystream.Close();
結論
在本文中,我們探討瞭如何使用 C# 編程語言將 PDF 文件轉換為字節數組以及將字節數組轉換為 PDF 文件。如果您有興趣進一步使用 PDF 文件,請通過 免費支持論壇 與我們分享您的要求。此外,您還可以瀏覽 API 文檔 和 API 參考 以深入分析 API 提供的功能。我們期待與您取得聯繫!