
在 PowerPoint 演示文稿中,評論用於編寫有關幻燈片內容的反饋。在處理 PowerPoint PPT/PPTX 演示文稿時,您可能需要以編程方式添加評論。在本文中,您將學習如何使用 Java 為 PowerPoint PPT 幻燈片添加評論。此外,我們將介紹如何閱讀或刪除幻燈片評論並添加他們的回复。
用於在 PowerPoint 中處理評論的 Java API
Aspose.Slides for Java 是一種流行的演示文稿操作 API,可讓您創建和修改 PowerPoint PPT/PPTX 文件。我們將使用此 API 來操作 PowerPoint 演示文稿中的評論。您可以 下載 API 的 JAR 或使用以下 Maven 配置安裝它。
存儲庫:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
依賴:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-slides</artifactId>
<version>22.2</version>
<classifier>jdk16</classifier>
</dependency>
在 Java 中向 PowerPoint PPT 幻燈片添加評論
在 PowerPoint 演示文稿中,每條評論都附有特定的作者。然而,每條評論都包含一些附加信息,例如創建時間、添加幻燈片的位置及其位置。以下是在 Java 中為 PPT 幻燈片添加評論的步驟。
- 首先,加載演示文稿文件或使用 Presentation 類創建一個新文件。
- 使用 Presentation.getCommentAuthors().addAuthor(String, String) 方法添加新作者。
- 獲取對像中新創建作者的引用。
- 定義註釋的位置。
- 使用 ICommentAuthor.getComments().addComment(String, ISlide, Point2D.Float, Date) 方法添加評論。
- 最後,使用 Presentation.save(String, SaveFormat) 方法保存演示文稿。
下面的代碼示例展示瞭如何在 Java 中為 PPT 幻燈片添加評論。
// 創建或加載演示文稿
Presentation presentation = new Presentation("presentation.pptx");
try {
// 添加空幻燈片或獲取現有幻燈片的引用
presentation.getSlides().addEmptySlide(presentation.getLayoutSlides().get_Item(0));
// 添加作者
ICommentAuthor author = presentation.getCommentAuthors().addAuthor("Usman", "UA");
// 設置評論的位置
Point2D.Float point = new Point2D.Float(0.2f, 0.2f);
// 在第一張幻燈片上添加幻燈片評論
author.getComments().addComment("Hello, this is slide comment", presentation.getSlides().get_Item(0), point, new Date());
// 保存演示文稿
presentation.save("add-comment.pptx", SaveFormat.Pptx);
} finally {
if (presentation != null)
presentation.dispose();
}
以下是我們使用上述代碼示例添加的評論的屏幕截圖。

用Java在PPT幻燈片中添加評論回复
Aspose.Slides 還允許您添加對評論的回复。回複本身是一條評論,它作為現有評論的子項出現。那麼讓我們看看如何用Java在PowerPoint PPT幻燈片中添加評論回复。
- 首先,加載演示文稿文件或使用 Presentation 類創建一個新文件。
- 使用 Presentation.getCommentAuthors().addAuthor(String, String) 方法添加新作者。
- 使用 ICommentAuthor.getComments().addComment(String, ISlide, Point2D.Float, Date) 方法添加評論並獲取返回的對象。
- 以同樣的方式插入另一個評論並在對像中獲取其引用。
- 使用 IComment.setParentComment(IComment) 方法設置第二條評論的父級。
- 最後,使用 Presentation.save(String, SaveFormat) 方法保存演示文稿。
以下代碼示例顯示瞭如何在 Java 中的 PPTX 演示文稿中添加對評論的回复。
// 創建或加載演示文稿
Presentation presentation = new Presentation("presentation.pptx");
try {
// 添加空幻燈片或獲取現有幻燈片的引用
presentation.getSlides().addEmptySlide(presentation.getLayoutSlides().get_Item(0));
// 添加作者
ICommentAuthor author = presentation.getCommentAuthors().addAuthor("Usman", "UA");
// 設置評論的位置
Point2D.Float point = new Point2D.Float(0.2f, 0.2f);
// 在第一張幻燈片上添加幻燈片評論
IComment comment = author.getComments().addComment("Hello, this is slide comment", presentation.getSlides().get_Item(0), point, new Date());
// 添加回複評論
IComment subReply = author.getComments().addComment("This is the reply to the comment.", presentation.getSlides().get_Item(0), new Point2D.Float(10, 10), new Date());
subReply.setParentComment(comment);
// 添加回複評論
IComment reply2 = author.getComments().addComment("This is second reply.", presentation.getSlides().get_Item(0), new Point2D.Float(10, 10), new Date());
reply2.setParentComment(comment);
// 保存演示文稿
presentation.save("add-comment-reply.pptx", SaveFormat.Pptx);
} finally {
if (presentation != null)
presentation.dispose();
}
以下屏幕截圖顯示了上述代碼示例的輸出。
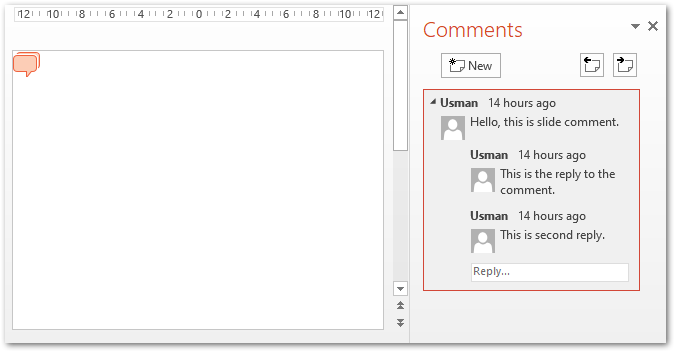
用 Java 閱讀 PPT 幻燈片中的評論
使用Aspose.Slides,您還可以閱讀特定作者或所有作者的評論。下面是用Java閱讀PPT幻燈片中的評論的步驟。
- 使用 Presentation 類加載演示文稿文件。
- 使用 Presentation.getCommentAuthors() 集合遍歷作者列表。
- 對於每個作者,使用 ICommentAuthor.getComments() 方法循環瀏覽其評論。
- 閱讀和打印評論詳細信息。
以下代碼示例顯示瞭如何使用 Java 讀取 PPT 幻燈片中的註釋。
// 負載演示
Presentation presentation = new Presentation("add-comment.pptx");
try {
// 遍歷作者
for (ICommentAuthor commentAuthor : presentation.getCommentAuthors())
{
// 訪問每個作者
CommentAuthor author = (CommentAuthor) commentAuthor;
// 循環瀏覽作者的評論
for (IComment comment1 : author.getComments())
{
// 閱讀評論
Comment comment = (Comment) comment1;
System.out.println("ISlide :" + comment.getSlide().getSlideNumber() + " has comment: " + comment.getText() +
" with Author: " + comment.getAuthor().getName() + " posted on time :" + comment.getCreatedTime() + "\n");
}
}
} finally {
if (presentation != null)
presentation.dispose();
}
在 Java 中從 PowerPoint PPT 中刪除評論
在上一節中,您了解瞭如何通過訪問評論集合來閱讀評論。同樣,您可以在獲得引用後刪除評論。以下代碼示例顯示瞭如何使用 Java 刪除 PowerPoint 演示文稿中的註釋。
// 負載演示
Presentation presentation = new Presentation("add-comment.pptx");
try {
// 獲取第一張幻燈片
ISlide slide = presentation.getSlides().get_Item(0);
// 獲取評論
IComment[] comments = slide.getSlideComments(null);
// 使用索引刪除所需的評論
comments[0].remove();
// 保存演示文稿
presentation.save("remove-comments.pptx", SaveFormat.Pptx);
} finally {
if (presentation != null)
presentation.dispose();
}
獲得免費許可證
通過申請臨時許可,您可以在沒有評估限制的情況下使用 Aspose.Slides for Java。
結論
在本文中,您了解瞭如何使用 Java 在 PowerPoint PPT 幻燈片中添加評論。此外,我們還介紹瞭如何以編程方式添加對評論的回复。最後,我們演示瞭如何閱讀或刪除 PPT 幻燈片中的註釋。您可以訪問 文檔 來探索更多關於 Aspose.Slides for Java 的信息。此外,您可以將您的查詢發佈到我們的論壇。