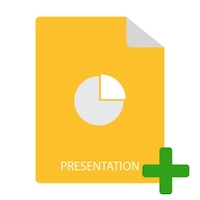
MS PowerPoint 演示文稿允許您創建包含文本、圖像、圖表、動畫和其他元素的幻燈片。各種額外的格式設置選項可讓您的演示文稿更具吸引力。在本文中,您將了解如何以編程方式創建此類演示文稿。您將學習如何使用 C# 創建包含文本、表格、圖像和圖表的 PPTX 演示文稿。
- C# PowerPoint API
- 創建 PowerPoint 演示文稿
- 打開現有的 PowerPoint 演示文稿
- 將幻燈片添加到演示文稿
- 將文本添加到演示文稿的幻燈片
- 在演示文稿中創建表格
- 在演示文稿中創建圖表
- 在演示文稿中添加圖像
C# PowerPoint API - 免費下載
Aspose.Slides for .NET 是一種演示文稿操作 API,可讓您在 .NET 應用程序中創建和操作 PowerPoint 文檔。 API 提供了實現基本和高級 PowerPoint 自動化功能所需的幾乎所有可能的功能。您可以 下載 API 或通過 NuGet 安裝它。
Install-Package Aspose.Slides.NET
在 C# 中創建 PowerPoint 演示文稿
讓我們首先使用 Aspose.Slides for .NET 創建一個空的 PowerPoint 演示文稿。以下是執行此操作的步驟。
- 創建 Presentation 類的實例。
- 使用 Presentation.Save(String, SaveFormat) 方法將其保存為 PPTX。
以下代碼示例顯示如何在 C# 中創建 PowerPoint 演示文稿。
// 實例化表示演示文稿文件的 Presentation 對象
using (Presentation presentation = new Presentation())
{
// 獲取第一張幻燈片
ISlide slide = presentation.Slides[0];
// 添加內容到幻燈片...
// 保存演示文稿
presentation.Save("NewPresentation.pptx", SaveFormat.Pptx);
}
在 C# 中打開現有的 PowerPoint 演示文稿
您無需額外努力即可打開現有的 PowerPoint 演示文稿。只需將 PPTX 文件的路徑提供給 Presentation 類的構造函數即可。下面的代碼示例顯示瞭如何打開現有的 PPTX 演示文稿。
// 通過將文件路徑傳遞給 Presentation 類的構造函數來打開演示文稿文件
Presentation pres = new Presentation("OpenPresentation.pptx");
// 打印演示文稿中的幻燈片總數
System.Console.WriteLine(pres.Slides.Count.ToString());
在 C# 中向演示文稿添加幻燈片
創建演示文稿後,您可以開始向其中添加幻燈片。以下是使用 Aspose.Slides for .NET 在演示文稿中添加幻燈片的步驟。
- 創建 Presentation 類的實例。
- 通過設置對 Presentations.Slides 屬性的引用來實例化 ISlideCollection 類。
- 使用 ISlideCollection 對象公開的 Slide.AddEmptySlide(ILayoutSlide) 方法向演示文稿添加一張空幻燈片
- 使用 Presentation.Save(String, SaveFormat) 方法保存演示文稿文件。
以下代碼示例演示如何使用 C# 在 PowerPoint 演示文稿中添加幻燈片。
// 實例化表示演示文稿文件的 Presentation 類
using (Presentation pres = new Presentation())
{
// 實例化 SlideCollection 類
ISlideCollection slds = pres.Slides;
for (int i = 0; i < pres.LayoutSlides.Count; i++)
{
// 將空幻燈片添加到幻燈片集合
slds.AddEmptySlide(pres.LayoutSlides[i]);
}
// 將 PPTX 文件保存到磁盤
pres.Save("EmptySlide_out.pptx", SaveFormat.Pptx);
}
使用 C# 在幻燈片中插入文本
現在我們可以向 PowerPoint 演示文稿中的幻燈片添加內容。讓我們首先使用以下步驟向幻燈片添加一段文本。
- 使用 Presentation 類創建一個新的演示文稿。
- 獲取演示文稿中幻燈片的引用。
- 在幻燈片的指定位置添加一個 IAutoShape,ShapeType 為 Rectangle。
- 獲取新添加的 IAutoShape 對象的引用。
- 將 TextFrame 添加到包含默認文本的自選圖形。
- 將演示文稿另存為 PPTX 文件。
以下代碼示例顯示如何使用 C# 在演示文稿中添加文本以幻燈片。
// Instantiate PresentationEx//實例化PresentationEx
using (Presentation pres = new Presentation())
{
// 獲取第一張幻燈片
ISlide sld = pres.Slides[0];
// 添加矩形類型的自選圖形
IAutoShape ashp = sld.Shapes.AddAutoShape(ShapeType.Rectangle, 150, 75, 150, 50);
// 將 TextFrame 添加到矩形
ashp.AddTextFrame(" ");
// 訪問文本框
ITextFrame txtFrame = ashp.TextFrame;
// 為文本框創建段落對象
IParagraph para = txtFrame.Paragraphs[0];
// 為段落創建部分對象
IPortion portion = para.Portions[0];
// 設置文本
portion.Text = "Aspose TextBox";
// 將演示文稿保存到磁盤
pres.Save("presentation.pptx", Aspose.Slides.Export.SaveFormat.Pptx);
}
使用 C# 在演示文稿中創建表
Aspose.Slides for .NET 提供了一種在演示文稿中創建表格的簡單方法。以下是它的步驟。
- 創建 Presentation 類的實例。
- 使用索引獲取幻燈片的引用。
- 定義具有寬度的列數組和具有高度的行。
- 使用 IShapes 對象公開的 Slide.Shapes.AddTable() 方法向幻燈片添加表格,並在 ITable 實例中獲取對錶格的引用。
- 遍歷每個單元格以應用格式。
- 使用 Table.Rows[][].TextFrame.Text 屬性向單元格添加文本。
- 將演示文稿另存為 PPTX 文件。
以下代碼示例顯示如何在 PowerPoint 演示文稿的幻燈片中創建表格。
// 實例化表示 PPTX 文件的 Presentation 類
Presentation pres = new Presentation();
// 訪問第一張幻燈片
ISlide sld = pres.Slides[0];
// 定義具有寬度的列和具有高度的行
double[] dblCols = { 50, 50, 50 };
double[] dblRows = { 50, 30, 30, 30, 30 };
// 將表格形狀添加到幻燈片
ITable tbl = sld.Shapes.AddTable(100, 50, dblCols, dblRows);
// 為每個單元格設置邊框格式
for (int row = 0; row < tbl.Rows.Count; row++)
{
for (int cell = 0; cell < tbl.Rows[row].Count; cell++)
{
tbl.Rows[row][cell].CellFormat.BorderTop.FillFormat.FillType = FillType.Solid;
tbl.Rows[row][cell].CellFormat.BorderTop.FillFormat.SolidFillColor.Color = Color.Red;
tbl.Rows[row][cell].CellFormat.BorderTop.Width = 5;
tbl.Rows[row][cell].CellFormat.BorderBottom.FillFormat.FillType = (FillType.Solid);
tbl.Rows[row][cell].CellFormat.BorderBottom.FillFormat.SolidFillColor.Color= Color.Red;
tbl.Rows[row][cell].CellFormat.BorderBottom.Width =5;
tbl.Rows[row][cell].CellFormat.BorderLeft.FillFormat.FillType = FillType.Solid;
tbl.Rows[row][cell].CellFormat.BorderLeft.FillFormat.SolidFillColor.Color =Color.Red;
tbl.Rows[row][cell].CellFormat.BorderLeft.Width = 5;
tbl.Rows[row][cell].CellFormat.BorderRight.FillFormat.FillType = FillType.Solid;
tbl.Rows[row][cell].CellFormat.BorderRight.FillFormat.SolidFillColor.Color = Color.Red;
tbl.Rows[row][cell].CellFormat.BorderRight.Width = 5;
}
}
// 合併第 1 行的單元格 1 和 2
tbl.MergeCells(tbl.Rows[0][0], tbl.Rows[1][1], false);
// 將文本添加到合併的單元格
tbl.Rows[0][0].TextFrame.Text = "Merged Cells";
// 將 PPTX 保存到磁盤
pres.Save("table.pptx", SaveFormat.Pptx);
使用 C# 在演示文稿中創建圖表
以下是使用 C# 在 PowerPoint 演示文稿中添加圖表的步驟。
- 創建 Presentation 類的實例。
- 通過索引獲取幻燈片的引用。
- 使用 ISlide.Shapes.AddChart(ChartType, Single, Single, Single, Single) 方法添加所需類型的圖表。
- 添加圖表標題。
- 訪問圖表數據工作表。
- 清除所有默認系列和類別。
- 添加新系列和類別。
- 為圖表系列添加新的圖表數據。
- 設置圖表系列的填充顏色。
- 添加圖表系列標籤。
- 將演示文稿另存為 PPTX 文件。
以下代碼示例顯示瞭如何使用 C# 在演示文稿中添加圖表。
// 實例化表示 PPTX 文件的 Presentation 類
Presentation pres = new Presentation();
// 訪問第一張幻燈片
ISlide sld = pres.Slides[0];
// 添加具有默認數據的圖表
IChart chart = sld.Shapes.AddChart(ChartType.ClusteredColumn, 0, 0, 500, 500);
// 設置圖表標題
// Chart.ChartTitle.TextFrameForOverriding.Text = "示例標題";
chart.ChartTitle.AddTextFrameForOverriding("Sample Title");
chart.ChartTitle.TextFrameForOverriding.TextFrameFormat.CenterText = NullableBool.True;
chart.ChartTitle.Height = 20;
chart.HasTitle = true;
// 將第一個系列設置為顯示值
chart.ChartData.Series[0].Labels.DefaultDataLabelFormat.ShowValue = true;
// 設置圖表數據表索引
int defaultWorksheetIndex = 0;
// 獲取圖表數據工作表
IChartDataWorkbook fact = chart.ChartData.ChartDataWorkbook;
// 刪除默認生成的系列和類別
chart.ChartData.Series.Clear();
chart.ChartData.Categories.Clear();
int s = chart.ChartData.Series.Count;
s = chart.ChartData.Categories.Count;
// 添加新系列
chart.ChartData.Series.Add(fact.GetCell(defaultWorksheetIndex, 0, 1, "Series 1"), chart.Type);
chart.ChartData.Series.Add(fact.GetCell(defaultWorksheetIndex, 0, 2, "Series 2"), chart.Type);
// 添加新類別
chart.ChartData.Categories.Add(fact.GetCell(defaultWorksheetIndex, 1, 0, "Caetegoty 1"));
chart.ChartData.Categories.Add(fact.GetCell(defaultWorksheetIndex, 2, 0, "Caetegoty 2"));
chart.ChartData.Categories.Add(fact.GetCell(defaultWorksheetIndex, 3, 0, "Caetegoty 3"));
// 獲取第一個圖表系列
IChartSeries series = chart.ChartData.Series[0];
// 現在填充系列數據
series.DataPoints.AddDataPointForBarSeries(fact.GetCell(defaultWorksheetIndex, 1, 1, 20));
series.DataPoints.AddDataPointForBarSeries(fact.GetCell(defaultWorksheetIndex, 2, 1, 50));
series.DataPoints.AddDataPointForBarSeries(fact.GetCell(defaultWorksheetIndex, 3, 1, 30));
// 設置系列的填充顏色
series.Format.Fill.FillType = FillType.Solid;
series.Format.Fill.SolidFillColor.Color = Color.Red;
// 採取第二個圖表系列
series = chart.ChartData.Series[1];
// 現在填充系列數據
series.DataPoints.AddDataPointForBarSeries(fact.GetCell(defaultWorksheetIndex, 1, 2, 30));
series.DataPoints.AddDataPointForBarSeries(fact.GetCell(defaultWorksheetIndex, 2, 2, 10));
series.DataPoints.AddDataPointForBarSeries(fact.GetCell(defaultWorksheetIndex, 3, 2, 60));
// 設置系列的填充顏色
series.Format.Fill.FillType = FillType.Solid;
series.Format.Fill.SolidFillColor.Color = Color.Green;
// 第一個標籤將顯示類別名稱
IDataLabel lbl = series.DataPoints[0].Label;
lbl.DataLabelFormat.ShowCategoryName = true;
lbl = series.DataPoints[1].Label;
lbl.DataLabelFormat.ShowSeriesName = true;
// 顯示第三個標籤的值
lbl = series.DataPoints[2].Label;
lbl.DataLabelFormat.ShowValue = true;
lbl.DataLabelFormat.ShowSeriesName = true;
lbl.DataLabelFormat.Separator = "/";
// 用圖表保存演示文稿
pres.Save("AsposeChart_out.pptx", SaveFormat.Pptx);
在 此處 了解有關演示圖表的更多信息。
在演示文稿中添加圖像
以下是在演示幻燈片中添加圖像的步驟。
- 使用 Presentation 類創建一個新的演示文稿。
- 使用 File.ReadAllText(String path) 方法讀取 SVG 圖像。
- 使用 Presentation.Slides[0].Shapes.AddPictureFrame(ShapeType shapeType, float x, float y,float width, float height, IPPImage image) 方法將圖像添加到幻燈片。
- 保存演示文稿。
以下代碼示例顯示瞭如何使用 C# 將圖像添加到演示文稿。
// 創建演示文稿
using (var p = new Presentation())
{
// 讀取圖片
var svgContent = File.ReadAllText("image.svg");
// 將圖像添加到圖像集合
var emfImage = p.Images.AddFromSvg(svgContent);
// 將圖像添加到幻燈片
p.Slides[0].Shapes.AddPictureFrame(ShapeType.Rectangle, 0, 0, emfImage.Width, emfImage.Height, emfImage);
// 保存演示文稿
p.Save("presentation.pptx", SaveFormat.Pptx);
}
結論
在本文中,您學習瞭如何使用 C# 從頭開始創建 PowerPoint 演示文稿。此外,您還了解瞭如何在新的或現有的 PPTX 演示文稿中添加幻燈片、文本、表格、圖像和圖表。您可以使用 文檔 了解有關 API 的更多信息。
也可以看看
- 使用 C# 保護 PowerPoint PPTX 演示文稿
- 在 ASP.NET 中創建 MS PowerPoint 演示文稿
- 在 C# 中將 PowerPoint PPTX/PPT 轉換為 PNG 圖像
- 使用 C# 在 PowerPoint 演示文稿中設置幻燈片背景
- 使用 C# 為 PowerPoint PPTX 或 PPT 生成縮略圖
- 使用 C# 將動畫應用於 PowerPoint 中的文本
- 使用 C# 拆分 PowerPoint 演示文稿
提示:除了創建幻燈片或演示文稿外,Aspose.Slides 還提供許多功能,允許您處理演示文稿。例如,Aspose 使用自己的 API 開發了 Microsoft PowerPoint 演示文稿的免費在線查看器。