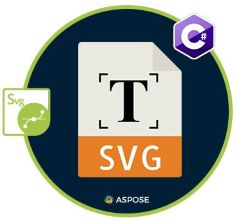
SVG 文本元素用於定義 SVG 中的文本。 SVG(可縮放矢量圖形)是一種網絡友好的矢量文件格式。它用於在網頁上顯示視覺信息。我們可以使用 SVG 文本元素輕鬆地在 SVG 中編寫任何文本。在本文中,我們將學習如何在 C# 中將文本轉換為 SVG。
本文應涵蓋以下主題:
將文本轉換為 SVG 的 C# API
要將文本轉換為 SVG,我們將使用 Aspose.SVG for .NET API。它允許加載、解析、渲染、創建 SVG 文件並將其轉換為 流行格式,而無需任何軟件依賴。
API 的 SVGDocument 類表示 SVG 層次結構的根並包含全部內容。我們在這個類中有 Save() 方法,它允許將 SVG 保存到指定的文件路徑。 SVGTextElement 類表示“文本”元素。 SVGTSpanElement 接口對應於“tspan”元素。 API 提供 SVGTextPathElement 類表示“textPath”元素和 SVGPathElement 表示“path”元素。我們可以使用 SetAttribute(string, string) 方法為 SVG 元素設置各種屬性。 API 的 AppendChild(Node) 方法將節點的新子節點添加到此 node 的子節點列表的末尾。
請 下載 API 的 DLL 或使用 NuGet 安裝它。
PM> Install-Package Aspose.SVG
什麼是 SVG 文本?
在 SVG 中,文本是使用 元素呈現的。默認情況下,文本呈現為黑色填充且沒有輪廓。我們可以定義以下屬性:
- x:水平設置點的位置。
- y:垂直設置點的位置。
- fill:定義呈現的文本顏色。
- transform :旋轉、平移、傾斜和縮放。
使用 C# 將文本轉換為 SVG
我們可以按照以下步驟將任何文本添加到 SVG:
- 首先,創建 SVGDocument 類的一個實例。
- 接下來,獲取文檔的根元素。
- 然後,創建 SVGTextElement 類對象。
- 接下來,使用 SetAttribute() 方法設置各種屬性。
- 之後,調用 AppendChild() 方法將其附加到根元素。
- 最後,使用 Save() 方法保存輸出的 SVG 圖像。
以下代碼示例顯示瞭如何在 C# 中將文本轉換為 SVG。
// 此代碼示例演示如何將文本添加到 SVG。
var document = new SVGDocument();
// 獲取文檔的根 svg 元素
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// 定義 SVG 文本元素
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
// 定義要顯示的文本
text.TextContent = "The is a simple SVG text!";
// 設置各種屬性
text.SetAttribute("fill", "blue");
text.SetAttribute("x", "10");
text.SetAttribute("y", "30");
// 將文本附加到根
svgElement.AppendChild(text);
// 另存為 SVG
document.Save(@"C:\Files\simple-text.svg");
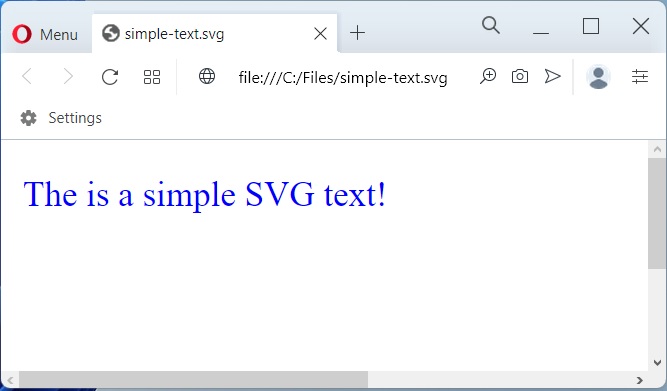
使用 C# 將文本轉換為 SVG。
請在下面找到 simple-text.svg 圖像的內容。
<svg xmlns="http://www.w3.org/2000/svg">
<text fill="blue" x="10" y="30">The is a simple SVG text!</text>
</svg>
在 C# 中使用 TSpan 將文本轉換為 SVG
SVG 元素定義了 元素中的子文本。我們可以按照以下步驟將帶有 tspan 元素的任何文本添加到 SVG:
- 首先,創建 SVGDocument 類的一個實例。
- 接下來,獲取文檔的根元素。
- 然後,創建 SVGTextElement 類對象。
- 或者,使用 SetAttribute() 方法設置各種屬性。
- 接下來,定義 SVGTSpanElement 對象。
- 然後,使用 SetAttribute() 方法設置其屬性。
- 現在,調用 AppendChild() 方法將其附加到 SVGTextElement 元素。
- 重複上述步驟以添加更多 SVGTSpanElement 對象。
- 之後,調用 AppendChild() 方法將 SVGTextElement 附加到根元素。
- 最後,使用 Save() 方法保存輸出的 SVG 圖像。
以下代碼示例顯示瞭如何在 C# 中將帶有 tspan 的文本轉換為 SVG。
// 此代碼示例演示如何使用 tspan 將文本添加到 SVG。
var document = new SVGDocument();
// 獲取文檔的根 svg 元素
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// SVG 文本元素
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
text.SetAttribute("style", "font-family:arial");
text.SetAttribute("x", "20");
text.SetAttribute("y", "60");
// SVG TSpan 元素
var tspan1 = (SVGTSpanElement)document.CreateElementNS(@namespace, "tspan");
tspan1.TextContent = "ASPOSE";
tspan1.SetAttribute("style", "font-weight:bold; font-size:55px");
tspan1.SetAttribute("x", "20");
tspan1.SetAttribute("y", "60");
// 附加到 SVG 文本
text.AppendChild(tspan1);
// 另一個 TSpan 元素
var tspan2 = (SVGTSpanElement)document.CreateElementNS(@namespace, "tspan");
tspan2.TextContent = "Your File Format APIs";
tspan2.SetAttribute("style", "font-size:20px; fill:grey");
tspan2.SetAttribute("x", "37");
tspan2.SetAttribute("y", "90");
// 附加到 SVG 文本
text.AppendChild(tspan2);
// 將 SVG 文本附加到根
svgElement.AppendChild(text);
// 保存 SVG
document.Save(@"C:\Files\svg-tSpan.svg");

在 C# 中使用 tspan 將文本轉換為 SVG。
請在下面找到 svg-tspan.svg 圖像的內容。
<svg xmlns="http://www.w3.org/2000/svg">
<text style="font-family: arial;" x="20" y="60">
<tspan style="font-weight: bold; font-size: 55px;" x="20" y="60">ASPOSE</tspan>
<tspan style="font-size: 20px; fill: grey;" x="37" y="90">Your File Format APIs</tspan>
</text>
</svg>
在 C# 中使用 TextPath 的 SVG 文本
我們還可以沿著 元素的形狀呈現文本,並將文本包含在 元素中。它允許使用 href 屬性設置對 元素的引用。我們可以按照以下步驟轉換帶有文本路徑的文本:
- 首先,創建 SVGDocument 類的一個實例。
- 接下來,獲取文檔的根元素。
- 然後,創建 SVGPathElement 類對象並使用 SetAttribute() 方法設置各種屬性。
- 之後,調用 AppendChild() 方法將其附加到根元素。
- 接下來,創建 SVGTextElement 類對象。
- 然後,初始化 SVGTextPathElement 對象並設置文本內容。
- 接下來,使用 SetAttribute() 方法將 SVGPathElement 分配給它的 href 屬性。
- 然後,調用 AppendChild() 方法將 SVGTextPathElement 附加到 SVGTextElement 元素。
- 之後,使用 AppendChild() 方法將 SVGTextElement 附加到根元素。
- 最後,使用 Save() 方法保存輸出的 SVG 圖像。
以下代碼示例顯示瞭如何在 C# 中將帶有 textPath 的文本轉換為 SVG。
// 此代碼示例演示如何將帶有 textPath 的文本添加到 SVG。
var document = new SVGDocument();
// 獲取文檔的根 svg 元素
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// SVG 路徑元素
var path1 = (SVGPathElement)document.CreateElementNS(@namespace, "path");
path1.SetAttribute("id", "path_1");
path1.SetAttribute("d", "M 50 100 Q 25 10 180 100 T 350 100 T 520 100 T 690 100");
path1.SetAttribute("fill", "transparent");
// 將 SVG 路徑附加到根元素
svgElement.AppendChild(path1);
// Another SVG 路徑元素
var path2 = (SVGPathElement)document.CreateElementNS(@namespace, "path");
path2.SetAttribute("id", "path_2");
path2.SetAttribute("d", "M 50 100 Q 25 10 180 100 T 350 100");
path2.SetAttribute("transform", "translate(0,75)");
path2.SetAttribute("fill", "transparent");
// 將 SVG 路徑附加到根元素
svgElement.AppendChild(path2);
// SVG 文本元素
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
// 創建 SVG 文本路徑元素
var textPath1 = (SVGTextPathElement)document.CreateElementNS(@namespace, "textPath");
textPath1.TextContent = "Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.";
textPath1.SetAttribute("href", "#path_1");
// 將 SVG 文本路徑附加到 SVG 文本
text.AppendChild(textPath1);
// 另一個 SVG 文本路徑元素
var textPath2 = (SVGTextPathElement)document.CreateElementNS(@namespace, "textPath");
textPath2.TextContent = "Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.";
textPath2.SetAttribute("href", "#path_2");
// 將 SVG 文本路徑附加到 SVG 文本
text.AppendChild(textPath2);
// 將 SVG 文本附加到根
svgElement.AppendChild(text);
// 保存 SVG
document.Save(@"C:\Files\svg-textPath.svg");
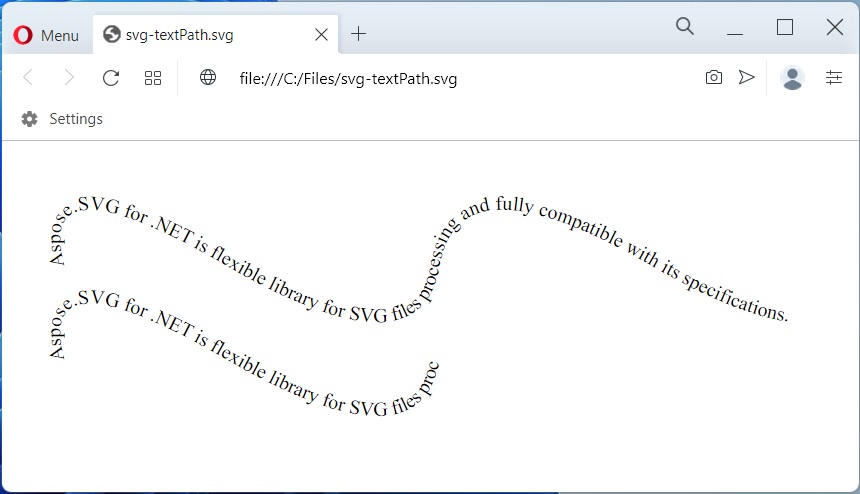
在 C# 中將帶有 textPath 的文本轉換為 SVG。
請在下面找到 svg-textPath.svg 圖像的內容。
<svg xmlns="http://www.w3.org/2000/svg">
<path id="path_1" d="M 50 100 Q 25 10 180 100 T 350 100 T 520 100 T 690 100" fill="transparent"/>
<path id="path_2" d="M 50 100 Q 25 10 180 100 T 350 100" transform="translate(0,75)" fill="transparent"/>
<text>
<textPath href="#path_1">Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.</textPath>
<textPath href="#path_2">Aspose.SVG for .NET is flexible library for SVG files processing and fully compatible with its specifications.</textPath>
</text>
</svg>
在 C# 中應用 SVG 文本樣式
我們可以按照以下步驟將任何文本添加到 SVG:
- 首先,創建 SVGDocument 類的一個實例。
- 接下來,獲取文檔的根元素。
- 然後,創建 SVGTextElement 類對象。
- 接下來,使用 SetAttribute() 方法設置樣式屬性。
- 之後,調用 AppendChild() 方法將其附加到根元素。
- 最後,使用 Save() 方法保存輸出的 SVG 圖像。
以下代碼示例顯示瞭如何在 C# 中將 CSS 樣式應用於 SVG 文本。
// 此代碼示例演示如何將文本添加到 SVG 並應用 CSS 樣式屬性。
var document = new SVGDocument();
// 獲取文檔的根 svg 元素
var svgElement = document.RootElement;
const string @namespace = "http://www.w3.org/2000/svg";
// 定義 SVG 文本元素
var text = (SVGTextElement)document.CreateElementNS(@namespace, "text");
// 定義要顯示的文本
text.TextContent = "The is a simple SVG text!";
// 設置各種屬性
text.SetAttribute("fill", "blue");
text.SetAttribute("x", "10");
text.SetAttribute("y", "30");
// 應用樣式
text.SetAttribute("style", "font-weight:bold; font-style: italic; text-decoration: line-through; text-transform: capitalize;");
// 將文本附加到根
svgElement.AppendChild(text);
// 另存為 SVG
document.Save(@"C:\Files\text-style.svg");

在 C# 中應用 SVG 文本樣式。
請在下面找到 text-style.svg 圖像的內容。
<svg xmlns="http://www.w3.org/2000/svg">
<text fill="blue" x="10" y="30" style="font-weight: bold; font-style: italic; text-decoration-line: line-through; text-transform: capitalize;">The is a simple SVG text!</text>
</svg>
獲得免費的臨時許可證
您可以獲得免費的臨時許可證 試用 Aspose.SVG for .NET,沒有評估限制。
結論
在本文中,我們學習瞭如何:
- 創建一個新的 SVG 圖像;
- 使用 SVG 文本元素;
- 將 SVG 文本渲染到路徑;
- 設置 SVG 文本的位置和填充屬性;
- 在 C# 中設置 SVG 文本的樣式屬性。
除了在 C# 中將文本轉換為 SVG 之外,您還可以在 文檔 中了解有關 Aspose.SVG for .NET 的更多信息,並探索 API 支持的不同功能。如有任何疑問,請隨時通過我們的免費支持論壇與我們聯繫。