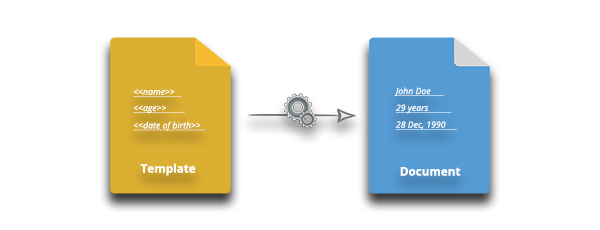
Word文檔的自動生成被企業廣泛用於創建大量報告。在某些情況下,文檔是從頭開始創建的。另一方面,預定義的模板用於通過填充佔位符來生成 Word 文檔。在本文中,我將演示如何在 C# 中以編程方式動態地從模板生成 Word 文檔。您將了解如何從不同類型的數據源填充 Word 模板。
本文將介紹以下場景以及代碼示例:
C# Word 自動化 API
我們將使用 Aspose.Words for .NET - 一個 Word 自動化 API,允許您從頭開始或通過填充預定義的 Word 模板生成 Word 文檔。您可以 下載 API 的二進製文件或使用以下方法之一安裝它。
使用 NuGet 包管理器

使用包管理器控制台
PM> Install-Package Aspose.Words
使用 C# 對像從模板生成 Word 文檔
首先,讓我們看看如何使用 C# 對象填充 Word 模板。為此,我們將創建一個 Word 文檔 (DOC/DOCX),並將以下佔位符作為文檔的內容:
<<[sender.Name]>> says: "<<[sender.Message]>>."
在這裡,發件人是我們將用於填充模板的以下類的對象。
public class Sender
{
public string Name { get; set; }
public string Message { get; set; }
public Sender (string _name, string _message)
{
Name = _name;
Message = _message;
}
}
現在,我們將使用 Aspose.Words 的報告引擎,按照以下步驟從模板和 Sender 類的對像生成 Word 文檔。
- 創建 Document 類的對象並使用 Word 模板的路徑對其進行初始化。
- 創建並初始化 Sender 類的對象。
- 實例化 ReportingEngine 類。
- 使用將文檔對象、數據源和數據源名稱作為參數的 ReportingEngine.BuildReport() 填充模板。
- 使用 Document.Save() 方法保存生成的 Word 文檔。
以下代碼示例顯示瞭如何在 C# 中從模板生成 Word 文檔。
Document doc = new Document("template.docx");
Sender sender = new Sender("LINQ Reporting Engine", "Hello World");
ReportingEngine engine = new ReportingEngine();
engine.BuildReport(doc, sender, "sender");
doc.Save("word.docx");
輸出

在 C# 中從 XML 數據源生成 Word 文檔
為了從 XML 數據源生成 Word 文檔,我們將使用帶有以下佔位符的更複雜的 Word 模板:
<<foreach [in persons]>>Name: <<[Name]>>, Age: <<[Age]>>, Date of Birth: <<[Birth]:"dd.MM.yyyy">>
<</foreach>>
Average age: <<[persons.Average(p => p.Age)]>>
下面給出了我在此示例中使用的 XML 數據源。
<Persons>
<Person>
<Name>John Doe</Name>
<Age>30</Age>
<Birth>1989-04-01 4:00:00 pm</Birth>
</Person>
<Person>
<Name>Jane Doe</Name>
<Age>27</Age>
<Birth>1992-01-31 07:00:00 am</Birth>
</Person>
<Person>
<Name>John Smith</Name>
<Age>51</Age>
<Birth>1968-03-08 1:00:00 pm</Birth>
</Person>
</Persons>
以下是從 XML 數據源生成 Word 文檔的步驟:
- 創建 Document 類的實例並使用 Word 模板的路徑對其進行初始化。
- 創建 XmlDataSource 類的實例並使用 XML 文件的路徑對其進行初始化。
- 實例化 ReportingEngine 類。
- 按照我們之前用來填充 Word 模板的相同方式使用 ReportingEngine.BuildReport() 方法。
- 使用 Document.Save() 方法保存生成的 Word 文檔。
下面的代碼示例顯示瞭如何在 C# 中從 XML 數據源生成 Word 文檔。
Document doc = new Document("template.docx");
XmlDataSource dataSource = new XmlDataSource("datasource.xml");
ReportingEngine engine = new ReportingEngine();
engine.BuildReport(doc, dataSource, "persons");
doc.Save("word.docx");
輸出

在 C# 中從 JSON 數據源生成 Word 文檔
現在讓我們看看如何使用 JSON 數據源生成 Word 文檔。在此示例中,我們將生成按經理分組的客戶列表。在這種情況下,以下是 Word 模板:
<<foreach [in managers]>>Manager: <<[Name]>>
Contracts:
<<foreach [in Contract]>>- <<[Client.Name]>> ($<<[Price]>>)
<</foreach>>
<</foreach>>
以下是我們將用於填充模板的 JSON 數據源:
[
{
Name: "John Smith",
Contract:
[
{
Client:
{
Name: "A Company"
},
Price: 1200000
},
{
Client:
{
Name: "B Ltd."
},
Price: 750000
},
{
Client:
{
Name: "C & D"
},
Price: 350000
}
]
},
{
Name: "Tony Anderson",
Contract:
[
{
Client:
{
Name: "E Corp."
},
Price: 650000
},
{
Client:
{
Name: "F & Partners"
},
Price: 550000
}
]
},
]
為了從 JSON 生成 Word 文檔,我們將使用 JsonDataSource 類來加載和使用 JSON 數據源,其餘步驟將保持不變。以下代碼示例顯示如何在 C# 中使用 JSON 從模板生成 Word 文檔。
Document doc = new Document("template.docx");
JsonDataSource dataSource = new JsonDataSource("datasource.json");
ReportingEngine engine = new ReportingEngine();
engine.BuildReport(doc, dataSource, "managers");
doc.Save("word.docx");
輸出

在 C# 中從 CSV 數據源生成 Word 文檔
為了從 CSV 生成 Word 文檔,我們將使用以下 Word 模板:
<<foreach [in persons]>>Name: <<[Column1]>>, Age: <<[Column2]>>, Date of Birth: <<[Column3]:"dd.MM.yyyy">>
<</foreach>>
Average age: <<[persons.Average(p => p.Column2)]>>
該模板將填充以下 CSV 數據:
John Doe,30,1989-04-01 4:00:00 pm
Jane Doe,27,1992-01-31 07:00:00 am
John Smith,51,1968-03-08 1:00:00 pm
現在,讓我們來看看 C# 代碼。除了我們將使用 CsvDataSource 類加載 CSV 數據這一變化外,所有步驟在這裡也將保持不變。以下代碼示例顯示如何從 CSV 數據源生成 Word 文檔。
Document doc = new Document("template.docx");
CsvDataSource dataSource = new CsvDataSource("datasource.csv");
ReportingEngine engine = new ReportingEngine();
engine.BuildReport(doc, dataSource, "persons");
doc.Save("word.docx");
輸出

免費試用 Aspose.Words for .NET
您可以使用免費的 臨時許可證 試用 Aspose.Words for .NET。
結論
在本文中,您了解瞭如何使用 C# 從模板生成 Word 文檔。此外,您還看到瞭如何使用對象、XML、JSON 和 CSV 數據源來生成 Word 文檔。您可以使用 文檔 探索有關 C# Word 自動化 API 的更多信息。此外,您可以通過我們的 論壇 聯繫我們。