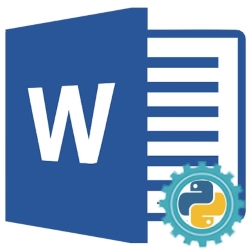
我們可以自動化 MS Word 創建新的 Word 文檔(DOC 或 DOCX),編輯或修改現有文檔,或將它們轉換為其他格式,而無需使用 Microsoft Office。 Python MS Word 自動化允許以編程方式執行我們可以通過 MS Word 的用戶界面執行的所有操作。在本文中,我們將學習如何使用 Python 自動化 MS Word 來創建、編輯或轉換 Word 文檔。
本文涵蓋了使用 Python 以編程方式生成和操作 Word 文檔所需的所有基本功能。本文包括以下主題:
- 用於創建、編輯或轉換 Word 文檔的 Python MS Word Automation API
- 創建 Word 文檔
- 編輯或修改 Word 文檔
- 在 Word 文檔中查找和替換文本
- 轉換 Word 文檔
- 解析 Word 文檔
用於創建、編輯或轉換 Word 文檔的 Python MS Word Automation API
為了自動化 Word,我們將使用 Aspose.Words for Python API。它是一個完整且功能豐富的 Word 自動化解決方案,用於以編程方式創建、編輯或分析 Word 文檔。 API 的 Document 類表示 Word 文檔。 API 提供了 DocumentBuilder 類,該類提供了在文檔中插入文本、圖像和其他內容的各種方法。此類還允許指定字體、段落和部分格式。 API 的 Run 類代表一系列具有相同字體格式的字符。請使用以下 pip 命令從 PyPI 在您的 Python 應用程序中安裝庫。
pip install aspose-words
使用 Python 創建 Word 文檔
我們可以按照以下步驟以編程方式創建 Word 文檔:
- 首先,創建一個 Document 類的實例。
- 接下來,使用 Document 對像作為參數創建 DocumentBuilder 類的實例。
- 之後,使用 DocumentBuilder 對象插入/寫入元素以添加一些文本、段落、表格或圖像。
- 最後,以輸出文件路徑作為參數調用 save() 方法來保存創建的文件。
以下代碼示例顯示瞭如何使用 Python 創建 Word 文檔 (DOCX)。
import aspose.words as aw
# 此代碼示例演示如何使用 Python 創建新的 Word 文檔。
# 創建文檔對象
doc = aw.Document()
# 創建文檔構建器對象
builder = aw.DocumentBuilder(doc)
# 指定字體格式 Font
font = builder.font
font.size = 32
font.bold = True
font.name = "Arial"
font.underline = aw.Underline.SINGLE
# 插入文字
builder.writeln("Welcome")
builder.writeln()
# 設置段落格式
font.size = 14
font.bold = False
font.name = "Arial"
font.underline = aw.Underline.NONE
paragraphFormat = builder.paragraph_format
paragraphFormat.first_line_indent = 8
paragraphFormat.alignment = aw.ParagraphAlignment.JUSTIFY
paragraphFormat.keep_together = True
# 插入段落
builder.writeln('''Aspose.Words for Python is a class library that enables your applications to perform a great range of document processing tasks.
It supports most of the popular document formats such as DOC, DOCX, RTF, HTML, Markdown, PDF, XPS, EPUB, and others.
With the API, you can generate, modify, convert, render, and print documents without third-party applications or Office Automation.
''')
builder.writeln()
# 插入表格
font.bold = True
builder.writeln("This is a sample table")
font.bold = False
# 啟動表
table = builder.start_table()
# 插入單元格
builder.insert_cell()
table.auto_fit(aw.tables.AutoFitBehavior.AUTO_FIT_TO_CONTENTS)
# 設置格式並添加文本
builder.cell_format.vertical_alignment = aw.tables.CellVerticalAlignment.CENTER
builder.write("Row 1 cell 1")
builder.insert_cell()
builder.write("Row 1 cell 2")
builder.end_row()
builder.insert_cell()
builder.write("Row 2 cell 1")
builder.insert_cell()
builder.write("Row 2 cell 2")
builder.end_row()
# 茶几
builder.end_table()
builder.writeln()
# 插入圖片
builder.insert_image("C:\\Files\\aspose-icon.png")
# 保存文檔
doc.save("C:\\Files\\sample_output.docx")
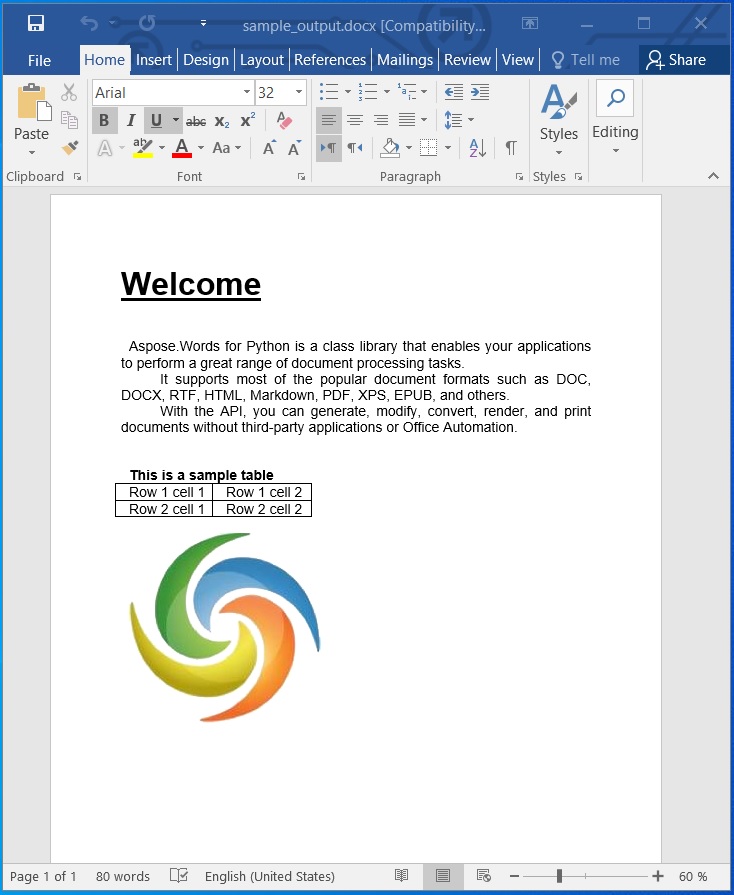
使用 Python 創建 Word 文檔。
使用 Python 編輯或修改 Word 文檔
在上一節中,我們創建了一個 Word 文檔。現在,讓我們編輯它並更改文檔的內容。我們可以按照以下步驟編輯Word文檔:
- 首先,使用 Document 類加載現有的 Word 文檔。
- 接下來,通過索引訪問特定部分。
- 然後,將第一段內容作為 Run 類的對象進行訪問。
- 之後,為訪問的段落設置要更新的文本。
- 最後,使用輸出文件路徑調用 save() 方法以保存更新的文件。
以下代碼示例顯示瞭如何使用 Python 編輯 Word 文檔 (DOCX)。
import aspose.words as aw
# 此代碼示例演示如何編輯現有的 Word 文檔。
# 裝入文檔
doc = aw.Document("C:\\Files\\sample_output.docx")
# 初始化文檔生成器
builder = aw.DocumentBuilder(doc)
# 訪問段落
paragraph = doc.sections[0].body.paragraphs[0].runs[0]
paragraph.text = "This is an updated text!"
# 保存文檔
doc.save("C:\\Files\\sample_updated.docx")
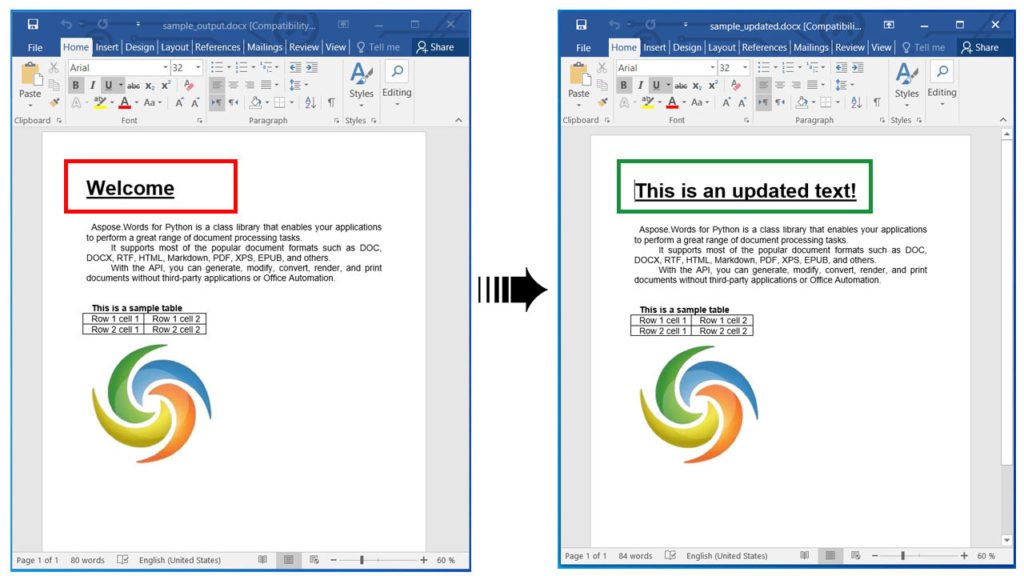
使用 Python 編輯或修改 Word 文檔。
使用 Python 查找和替換 Word 文檔中的文本
我們還可以按照以下步驟找到任何文本並將其替換為新文本:
- 首先,使用 Document 類加載 Word 文檔。
- 接下來,創建 FindReplaceOptions 類的一個實例。
- 之後,調用 replace() 方法。它以搜索字符串、替換字符串和 FindReplaceOptions 對像作為參數。
- 最後,使用輸出文件路徑調用 save() 方法以保存更新的文件。
以下代碼示例展示瞭如何使用 Python 在 Word 文檔 (DOCX) 中查找和替換特定文本。
import aspose.words as aw
# 此代碼示例演示如何在 Word 文檔中查找和替換文本。
# 裝入文檔
doc = aw.Document("C:\\Files\\sample_output.docx")
# 使用查找和替換更新
# 使用 Replace 方法指定搜索字符串和替換字符串。
doc.range.replace("Aspose.Words", "Hello",
aw.replacing.FindReplaceOptions(aw.replacing.FindReplaceDirection.FORWARD))
# 保存文件
doc.save("C:\\Files\\find_and_replace.docx")
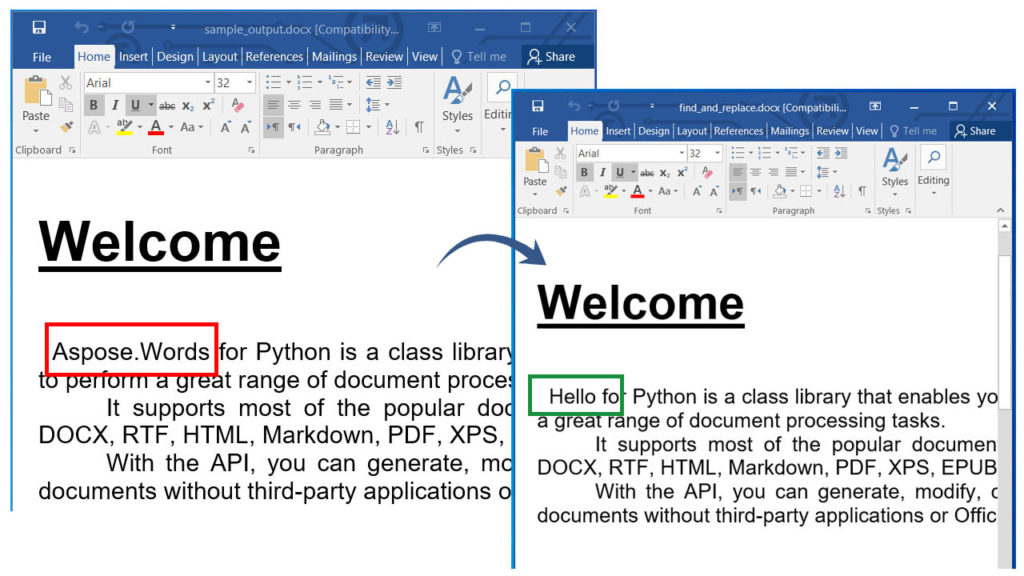
在 Word 文檔中查找和替換文本。
使用 Python 轉換 Word 文檔
我們可以將 Word 文檔轉換為其他格式,如 PDF、XPS、EPUB、HTML、JPG、PNG 等。請按照以下步驟將 Word 文檔轉換為 HTML 網頁:
- 首先,使用 Document 類加載 Word 文檔。
- 接下來,使用 Document 對像作為參數創建 HtmlSaveOptions 類的實例。
- 之後,指定 cssstylesheettype、exportfontresources、resourcefolder 和別名屬性。
- 最後,以輸出文件路徑和 HtmlSaveOptions 對像作為參數調用 save() 方法以保存轉換後的 HTML 文件。
以下代碼示例顯示瞭如何使用 Python 將 Word 文檔 (DOCX) 轉換為 HTML。
import aspose.words as aw
# 此代碼示例演示如何將 Word 文檔轉換為 PDF。
# 加載現有的 Word 文檔
doc = aw.Document("C:\\Files\\sample_output.docx")
# 指定保存選項
saveOptions = aw.saving.HtmlSaveOptions()
saveOptions.css_style_sheet_type = aw.saving.CssStyleSheetType.EXTERNAL
saveOptions.export_font_resources = True
saveOptions.resource_folder = "C:\\Files\\Resources"
saveOptions.resource_folder_alias = "C:/Files/resources"
# 保存轉換後的文檔
doc.save("C:\\Files\\Converted.html", saveOptions)

同樣,我們也可以將Word文檔轉換成其他支持的格式。請在文檔中詳細了解如何將Word 轉 EPUB、Word 轉 PDF、Word 文檔轉 Markdown、Word 轉 JPG 或 PNG 圖片 .
使用 Python 解析 Word 文檔
我們可以按照下面給出的步驟解析Word文檔並將內容提取為純文本:
- 首先,使用 Document 類加載 Word 文檔。
- 接下來,提取並打印文本。
- 最後調用save()方法將Word文檔保存為文本文件。此方法將輸出文件的路徑作為參數。
以下代碼示例展示瞭如何使用 Python 解析 Word 文檔 (DOCX)。
import aspose.words as aw
# 此代碼示例演示如何解析 Word 文檔。
# 裝入文檔
doc = aw.Document("C:\\Files\\Sample.docx")
# 提取文本
print(doc.range.text)
# 另存為純文本
doc.save("C:\\Files\\output.txt")
獲得免費許可證
您可以 獲得免費的臨時許可證 來試用該庫,而沒有評估限制。
結論
在本文中,我們學習瞭如何:
- 使用 Python 自動化 MS Word;
- 以編程方式創建和編輯 Word 文檔;
- 解析或轉換 DOCX 文件;
- 使用 Python 查找和替換 Word 文檔中的文本。
此外,您可以使用 文檔 了解更多關於 Aspose.Words for Python API 的信息。如有任何歧義,請隨時在論壇上與我們聯繫。