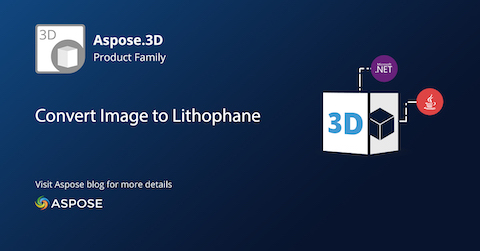
PNG 格式很受欢迎,因为它们可以包含透明图形。然而,lithophane 是一种蚀刻或模制的非常薄材料的艺术品,您可以通过将光源放在此类模型后面来看到它。本文介绍如何在 C# 中将 PNG 图像转换为 lithophane。
PNG 图像到 Lithophane 转换器 – C# API 安装
Aspose.3D for .NET API 可用于处理不同的 3D 模型和场景。您可以通过从 New Releases 页面下载其参考 DLL 文件或使用以下 NuGet 安装命令轻松配置 API:
PM> Install-Package Aspose.3D
在C#中将PNG图像转换为Lithophane
您可以按照以下步骤将 PNG 图像文件转换为 Lithophane 格式:
- 加载输入的 PNG 图像。
- 对 Mesh 对象执行计算操作。
- 使用 Save 方法生成 3d 场景并保存对象。
下面的代码片段解释了如何在 C# 中以编程方式将 PNG 图像转换为 Lithophane:
string file = "template.png";
string output = "file.fbx";
// 创建一些新参数
Aspose.ThreeD.Render.TextureData td = Aspose.ThreeD.Render.TextureData.FromFile(file);
const float nozzleSize = 0.9f;
const float layerHeight = 0.2f;
var grayscale = ToGrayscale(td);
const float width = 120.0f;
float height = width / td.Width * td.Height;
float thickness = 10.0f;
float layers = thickness / layerHeight;
int widthSegs = (int)Math.Floor(width / nozzleSize);
int heightSegs = (int)Math.Floor(height / nozzleSize);
// 对 Mesh 对象执行计算操作
Aspose.ThreeD.Entities.Mesh mesh = new Aspose.ThreeD.Entities.Mesh();
for (int y = 0; y < heightSegs; y++)
{
float dy = (float)y / heightSegs;
for (int x = 0; x < widthSegs; x++)
{
float dx = (float)x / widthSegs;
float gray = Sample(grayscale, td.Width, td.Height, dx, dy);
float v = (1 - gray) * thickness;
mesh.ControlPoints.Add(new Aspose.ThreeD.Utilities.Vector4(dx * width, dy * height, v));
}
}
for (int y = 0; y < heightSegs - 1; y++)
{
int row = (y * heightSegs);
int ptr = row;
for (int x = 0; x < widthSegs - 1; x++)
{
mesh.CreatePolygon(ptr, ptr + widthSegs, ptr + 1);
mesh.CreatePolygon(ptr + 1, ptr + widthSegs, ptr + widthSegs + 1);
ptr++;
}
}
// 生成 3d 场景并保存对象
Aspose.ThreeD.Scene scene = new Aspose.ThreeD.Scene(mesh);
scene.Save(output, Aspose.ThreeD.FileFormat.FBX7400ASCII);
// 调用的示例方法
static float Sample(float[,] data, int w, int h, float x, float y)
{
return data[(int)(x * w), (int)(y * h)];
}
// ToGrayscale 方法调用
static float[,] ToGrayscale(Aspose.ThreeD.Render.TextureData td)
{
var ret = new float[td.Width, td.Height];
var stride = td.Stride;
var data = td.Data;
var bytesPerPixel = td.BytesPerPixel;
for (int y = 0; y < td.Height; y++)
{
int ptr = y * stride;
for (int x = 0; x < td.Width; x++)
{
var v = (data[ptr] * 0.21f + data[ptr + 1] * 0.72f + data[ptr + 2] * 0.07f) / 255.0f;
ret[x, y] = v;
ptr += bytesPerPixel;
}
}
return ret;
}
获得免费临时许可证
您可以申请 免费临时许可证 来评估 API,不受任何限制。
在线演示
请尝试使用此 API 开发的 PNG Image to Lithophane Converter 网络应用程序。
结论
在本文中,您探索了 PNG 图像到 lithophane 的转换。此外,您可以查看 documentation 空间以了解 API 的其他功能。如果您有任何问题或疑问,请在 论坛 上给我们写信。