
条形码 是由平行线或几何图案直观地表示的数据的机器可读表示形式。它们提供了一种快速准确的方法来存储和检索信息,例如产品详细信息、库存代码或跟踪号码。条形码有多种类型,包括 UPC、EAN、QR 码等。每种类型都有特定的结构和编码机制。在这篇博文中,我们将学习如何构建高性能的 Python 条形码阅读器。附有代码示例的 Python 教程将向您展示如何扫描条形码。
本文将涵盖以下主题:
Python 条码读取器 API
我们将使用Aspose.BarCode for Python来扫描和读取条形码。它是一个条形码生成和识别库,允许您向 Python 应用程序添加条形码功能。它提供简单直观的 API 来生成和识别各种类型的条形码,包括 QR 码、Code 128、EAN-13、UPC-A 等。使用 Aspose.BarCode for Python,您可以轻松生成条形码图像、自定义其外观以及从图像或扫描文档中读取条形码。它是将条形码功能集成到 Python 项目中的强大工具。
请下载Python Barcode库包或在控制台中使用以下pip命令从PyPI安装API:
pip install aspose-barcode-for-python-via-net
Python 从图像中读取条形码
我们可以按照以下步骤扫描并读取条形码:
- 创建 BarCodeReader 类的实例,并将图像路径作为参数。
- 调用readbarcodes()方法并获取识别结果。
- 最后,循环结果并显示识别的类型和代码文本。
以下代码示例展示了如何使用 Python 从图像中读取条形码。
# 此代码示例演示如何使用 Python 扫描和读取图像中的条形码。
# 图片路径
full_path = "C:\\Files\\barcode.jpg"
# 初始化条形码阅读器
reader = barcoderecognition.BarCodeReader(full_path)
# 读取条形码
recognized_results = reader.read_bar_codes()
# 显示结果
for x in recognized_results:
print("Code text: " + x.code_text)
print("Barcode type: " + x.code_type_name)

用 Python 读取条形码。
Code text: 1234567890
Barcode type: Code39Standard
在Python中读取多个条形码
同样,我们按照前面提到的步骤扫描并读取文档中可用的多个条形码。
以下代码示例展示了如何使用 Python 从图像中读取多个条形码。
# 此代码示例演示如何使用 Python 扫描和读取图像中的多个条形码。
# 图片路径
full_path = "C:\\Files\\barcodes_different_quality.png"
# 初始化条形码阅读器
reader = barcoderecognition.BarCodeReader(full_path)
# 读取条形码
recognized_results = reader.read_bar_codes()
# 显示结果
for x in recognized_results:
print(x.code_text)
print(x.code_type_name)
print("------------------------------")

用 Python 读取多个条形码。
Code text: Aspose Code 04
Barcode type: Code128
------------------------------
Code text: Aspose Regular
Barcode type: Aztec
------------------------------
Code text: /YYAD25HL
Barcode type: Code39Standard
------------------------------
Code text: 7894706
Barcode type: Matrix2of5
------------------------------
Code text: D19-WQ9-F91046-0811
Barcode type: DataMatrix
------------------------------
Code text: 0058
Barcode type: Code39Standard
------------------------------
Code text: 990000837284
Barcode type: Planet
------------------------------
在Python中读取特定的条形码类型
我们可以按照以下步骤扫描读取指定的条码类型:
- 创建 BarCodeReader 类的实例。
- 指定图像路径和条形码解码类型作为参数。
- 之后,调用readbarcodes()方法并获取识别结果。
- 最后,循环结果并显示识别的类型和代码文本。
以下代码示例展示了如何在Python中扫描和读取特定的条形码类型。
# 此代码示例演示如何使用 Python 扫描和读取图像中的特定条形码类型。
# 图片路径
full_path = "C:\\Files\\Code_128.png"
# 初始化条形码阅读器
# 指定解码类型以读取特定的条形码类型
reader = barcoderecognition.BarCodeReader(full_path, barcoderecognition.DecodeType.CODE128)
# 读取条形码
recognized_results = reader.read_bar_codes()
# 显示结果
for x in recognized_results:
print("Code text: " + x.code_text)
print("Barcode type: " + x.code_type_name)

读取 Python 中的特定条形码类型。
Code text: 1234567890
Barcode type: Code128
Python 条形码扫描仪 – 指定质量设置
我们可以按照以下步骤指定各种质量设置来读取扭曲、损坏或低质量的条形码图像:
- 创建 BarCodeReader 类的实例,并将图像路径作为参数。
- 使用qualitysettings类指定各种质量设置。
- 之后,调用readbarcodes()方法并获取识别结果。
- 最后,循环结果并显示识别的类型和代码文本。
以下代码示例显示如何指定 Python 条形码扫描仪的质量设置。
# 此代码示例演示了如何在 Python 中扫描和读取条形码时指定质量设置。
# 图片路径
full_path = "C:\\Files\\barcodes_different_quality.png"
# 初始化条形码阅读器
reader = barcoderecognition.BarCodeReader(full_path)
# 指定质量设置
reader.quality_settings = barcoderecognition.QualitySettings.high_performance
reader.quality_settings.allow_median_smoothing = True
reader.quality_settings.median_smoothing_window_size = 5
# 读取条形码
recognized_results = reader.read_bar_codes()
# 显示结果
for x in recognized_results:
print(x.code_text)
print(x.code_type_name)
print("------------------------------")
Python 条形码阅读器 – 获取免费许可证
Aspose 提供免费评估版本来测试该库的特性和功能。您可以获取免费的临时许可证免费试用该库,没有任何限制。
Python 在线条码扫描器
您可以使用相机和使用此 API 开发的免费在线条形码扫描仪 网络应用程序在线扫描条形码。
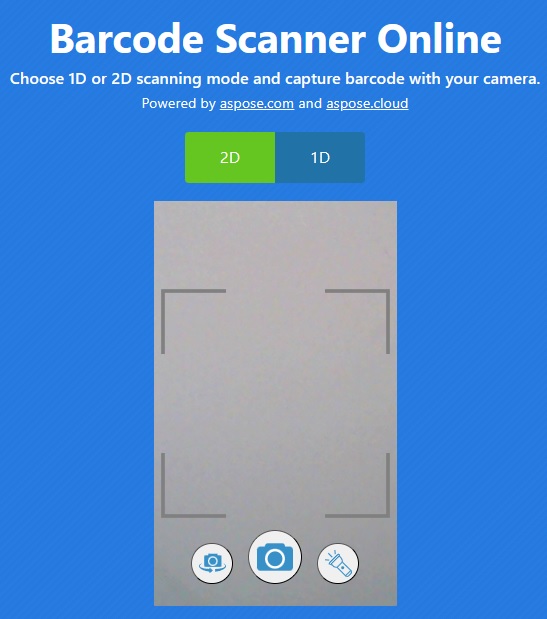
在线阅读条形码
您还可以使用免费在线条形码阅读器网络应用程序上传图像来在线读取条形码。
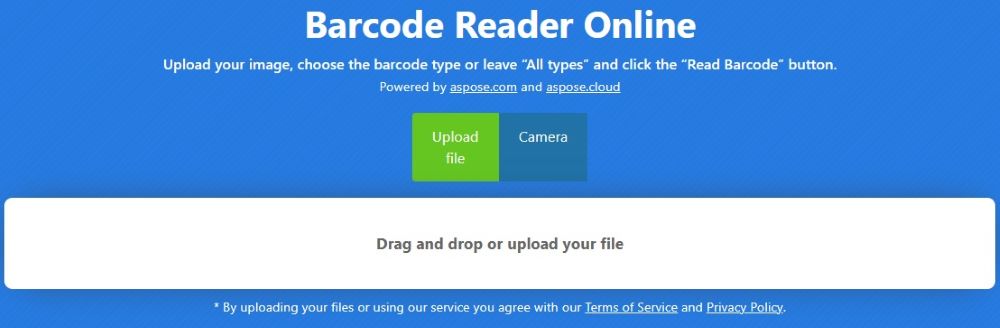
Python 条形码阅读器 – 免费学习资源
您可以使用以下资源了解有关生成和读取条形码的更多信息,并探索 Aspose.BarCode for Python 的其他功能:
结论
总之,Python 为在应用程序中实现条形码扫描功能提供了一个出色的平台。 Aspose.BarCode for Python 允许您轻松扫描、解码条形码并提取信息。开始将条形码扫描集成到您的 Python 项目中,并释放数据捕获和自动化的新可能性。如有任何疑问,请随时通过我们的免费支持论坛与我们联系。