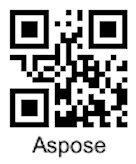
二维码是一种可以被机器读取的条形码。它是一种用于识别物体或传播某些信息的光学标签。在某些用例中,您可能需要扫描 QR 码。针对这样的场景,本文解释了如何在 Java 中以编程方式扫描二维码。
Java API 配置扫描二维码
您可以轻松访问 Aspose.BarCode for Java API 来处理不同类型的 barcodes,包括 QR 码。通过在 New Releases 部分下载其 JAR 文件或在应用程序的 pom.xml 文件中使用以下 Maven 配置来快速安装 API。
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-barcode</artifactId>
<version>22.8</version>
</dependency>
用Java扫描二维码
您需要按照以下步骤扫描二维码:
- 创建 BarCodeReader 类的实例并指定 DecodeType 值。
- 实例化 BarCodeResult 类的对象。
- 扫描二维码并获取输出文本。
下面的代码片段解释了如何在 Java 中扫描二维码:
// 创建 BarCodeReader 类的实例
BarCodeReader reader = new BarCodeReader("input.png", DecodeType.QR);
for (BarCodeResult result : reader.readBarCodes()) {
System.out.println("BarCode CodeText: " + result.getCodeText());
System.out.println("BarCode CodeType: " + result.getCodeTypeName());
}
从 Java 流中扫描 QR 码
以下是从 Java 流中读取二维码的步骤:
- 从 Stream 加载输入文件并启动 BarcodeReader 类的对象。
- 将 DecodeType 属性设置为 QR 并创建 BarCodeResult 类的实例。
- 扫描二维码并打印扫描结果。
以下代码片段详细说明了如何在 Java 中以编程方式从流中扫描 QR 码:
// 使用流加载输入的二维码
InputStream stream = new FileInputStream("input.png");
// 初始化 BarCodeReader 类的对象
BarCodeReader reader = new BarCodeReader(stream, DecodeType.QR);
for (BarCodeResult result : reader.readBarCodes()) {
System.out.println("BarCode CodeText: " + result.getCodeText());
System.out.println("BarCode CodeType: " + result.getCodeTypeName());
}
获得免费许可证
您可以申请 免费临时许可证 以不受任何限制地评估 API。
结论
在本文中,您学习了如何在 Java 中以编程方式扫描 QR 码。此外,如果您有兴趣探索 API 的各种其他功能和特性,请访问 文档 部分。如果您需要讨论您的任何疑虑,请随时在 论坛 上给我们写信。