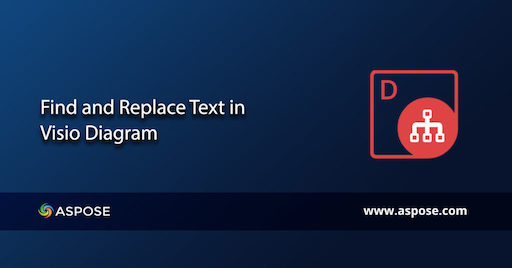
Visio 文件可以包含形状、连接器、图像或文本来描述用于创建组织图、流程图等图表的不同图表。在某些情况下,您可能希望查找和替换 VSD 或 VSDX 格式的某些文本视觉图。因此,本文解释了如何在 Java 中以编程方式查找和替换 Visio 绘图中的文本。
在 Visio 图表中搜索和替换文本 - Java API 安装
Aspose.Diagram for Java API 支持使用不同的 Visio 文件格式,包括 VSD、VSDX、VSDM、VSSX 等。您可以从 下载 部分下载 JAR 文件,或使用以下项目的 pom.xml 文件中的配置,以便从 Aspose Repository 访问 API:
存储库:
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
</repositories>
依赖:
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-diagram</artifactId>
<version>22.4</version>
<classifier>jdk16</classifier>
</dependency>
</dependencies>
在 Java 中以编程方式查找和替换 Visio 图表中的文本
您可以使用以下步骤在 VSD VSDX 格式的 Visio 文件中查找和替换文本:
- 创建字符串集合以搜索和替换文本。
- 加载源 Visio 图表文件并循环浏览每个形状中的文本。
- 编写输出 Visio 图表文件。
下面的代码示例显示了如何使用 Java 以编程方式查找和替换 Visio 文件中的文本:
// 加载输入图
Diagram diagram = new Diagram("FindReplaceText.vsdx");
DateFormat dateFormat = new SimpleDateFormat("dd/MMMM/yyyy");
Date myDate = new Date(System.currentTimeMillis());
Calendar cal = Calendar.getInstance();
// 准备集合新旧文本
Hashtable<String, String> replacements = new Hashtable<String, String>();
replacements.put("[[CompanyName]]", "Research Society of XYZ");
replacements.put("[[CompanyName]]", "Research Society of XYZ");
replacements.put("[[EmplyeeName]]", "James Bond");
replacements.put("[[SubjectTitle]]", "The affect of the internet on social behavior in the industrialize world");
cal.setTime(myDate);
cal.add(Calendar.YEAR, -1);
System.out.println(dateFormat.format(cal.getTime()));
replacements.put("[[TimePeriod]]", dateFormat.format(cal.getTime()) + " -- " + dateFormat.format(myDate));
cal.setTime(myDate);
cal.add(Calendar.DAY_OF_MONTH, -7);
System.out.println(dateFormat.format(cal.getTime()));
replacements.put("[[SubmissionDate]]", dateFormat.format(cal.getTime()));
replacements.put("[[AmountReq]]", "$100,000");
cal.setTime(myDate);
cal.add(Calendar.DAY_OF_MONTH, 1);
System.out.println(dateFormat.format(cal.getTime()));
replacements.put("[[DateApproved]]", dateFormat.format(cal.getTime()));
// 遍历页面的形状
for (Shape shape : (Iterable<Shape>) diagram.getPages().getPage("Page-1").getShapes())
{
Set<String> keys = replacements.keySet();
for(String key: keys)
{
for (FormatTxt txt : (Iterable<FormatTxt>) shape.getText().getValue())
{
Txt tx = (Txt)((txt instanceof Txt) ? txt : null);
if (tx != null && tx.getText().contains(key))
{
// 查找和替换形状的文本
tx.setText(tx.getText().replace(key, replacements.get(key)));
}
}
}
}
// 保存图表
diagram.save("FindReplaceText_Out.vsdx", SaveFileFormat.VSDX);
结论
总之,您已了解如何在 Visio 图表中查找和替换文本。您还可以即兴编写代码片段以使用替换方法的其他重载。例如,替换匹配文本的所有实例或仅替换 Visio 文件中搜索词的第一次出现。您可以访问 documentation 空间来了解其他几个操作或转换 MS Visio 文件的功能。如果您需要讨论您的任何疑虑或要求,请通过 论坛 与我们联系。