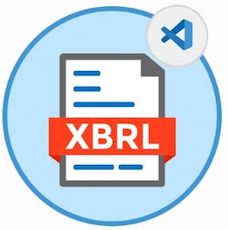
XBRL(可扩展业务报告语言)定义和交换财务信息,例如财务报表。 XBRL 实例文档是共同构成业务报告的事实集合。我们可以轻松地以编程方式创建 XBRL 实例文档并添加对象或元素,例如架构引用、上下文、单元、项等。在本文中,我们将学习如何使用 C# 将对象添加到 XBRL 实例文档。
本文将涵盖以下主题:
用于将对象添加到 XBRL 实例文档的 C# API
为了创建 XBRL 实例文档和添加对象,我们将使用 Aspose.Finance for .NET API。它允许创建 XBRL 实例、解析和验证 XBRL 或 iXBRL 文件。请下载 API 的 DLL 或使用 NuGet 安装它。
PM> Install-Package Aspose.Finance
使用 C# 添加对 XBRL 的架构引用
我们可以按照以下步骤在 XBRL 实例文档中添加模式引用:
- 首先,创建一个 XbrlDocument 类的实例。
- 接下来,将 XbrlDocument 对象的实例集合放入 XbrlInstanceCollection 对象中。
- 然后,使用 XbrlInstanceCollection.Add() 方法添加一个新的 XBRL 实例。
- 接下来,将 XbrlInstance 对象的架构引用集合放入 SchemaRefCollection 对象中。
- 之后,使用 SchemaRefCollection.Add() 方法添加一个新的模式引用。
- 最后,使用 XbrlDocument.Save() 方法保存 XBRL 文件。它将输出文件路径作为参数。
以下代码示例展示了如何使用 C# 在 XBRL 实例文档中添加架构引用。
// 此代码示例演示如何将 SchemaReference 对象添加到 XBRL。
// 创建 XbrlDocument 类的实例
XbrlDocument document = new XbrlDocument();
// 获取 XbrlInstances
XbrlInstanceCollection xbrlInstances = document.XbrlInstances;
// 添加 XbrlInstance
xbrlInstances.Add();
XbrlInstance xbrlInstance = xbrlInstances[0];
// 获取 Schema 引用集合
SchemaRefCollection schemaRefs = xbrlInstance.SchemaRefs;
// 添加架构参考
schemaRefs.Add(@"D:\Files\Finance\schema.xsd", "example", "http://example.com/xbrl/taxonomy");
// 保存输出文件
document.Save(@"D:\Files\Finance\output.xbrl");
使用 CSharp 在 XBRL 中添加上下文对象
我们可以按照以下步骤向 XBRL 实例文档添加上下文:
- 首先,创建一个 XbrlDocument 类的实例。
- 接下来,将 XbrlDocument 对象的实例集合放入 XbrlInstanceCollection 对象中。
- 然后,使用 XbrlInstanceCollection.Add() 方法添加一个新的 XBRL 实例。
- 接下来,创建具有开始日期和结束日期的 ContextPeriod 类的实例。
- 然后,创建一个 ContextEntity 并提供架构和标识符。
- 接下来,使用已定义的 ContextPeriod 和 ContextEntity 创建 Context 类的实例。
- 之后,将 Context 对象添加到 Context objects 集合中。
- 最后,使用 XbrlDocument.Save() 方法保存 XBRL 文件。它将输出文件路径作为参数。
以下代码示例展示了如何使用 C# 在 XBRL 实例文档中添加上下文对象。
// 此代码示例演示如何将 Context 对象添加到 XBRL。
// 创建 XbrlDocument 类的实例
XbrlDocument document = new XbrlDocument();
// 获取 XbrlInstances
XbrlInstanceCollection xbrlInstances = document.XbrlInstances;
// 添加 XbrlInstance
xbrlInstances.Add();
XbrlInstance xbrlInstance = xbrlInstances[0];
// 定义上下文期间
ContextPeriod contextPeriod = new ContextPeriod(DateTime.Parse("2020-01-01"), DateTime.Parse("2020-02-10"));
// 克里特语上下文条目
ContextEntity contextEntity = new ContextEntity("exampleIdentifierScheme", "exampleIdentifier");
// 创建上下文
Context context = new Context(contextPeriod, contextEntity);
// 添加上下文
xbrlInstance.Contexts.Add(context);
// 保存输出文件
document.Save(@"D:\Files\Finance\Output.xbrl");
使用 C# 在 XBRL 中创建单元
XBRL 中的单位测量数字项目。我们可以按照以下步骤在 XBRL 实例文档中添加一个单元:
- 首先,创建一个 XbrlDocument 类的实例。
- 接下来,将 XbrlDocument 对象的实例集合放入 XbrlInstanceCollection 对象中。
- 然后,使用 XbrlInstanceCollection.Add() 方法添加一个新的 XBRL 实例。
- 接下来,使用 UnitType 作为 Measure 创建 Unit 类的实例。
- 然后,将 QualifiedName 添加到 MeasureQualifiedNames 集合。
- 之后,将 Unit 添加到 Unit objects 集合中。
- 最后,使用 XbrlDocument.Save() 方法保存 XBRL 文件。它将输出文件路径作为参数。
以下代码示例展示了如何使用 C# 在 XBRL 实例文档中添加单位对象。
// 此代码示例演示如何将 Unit 对象添加到 XBRL。
// 创建 XbrlDocument 类的实例
XbrlDocument document = new XbrlDocument();
// 获取 XbrlInstances
XbrlInstanceCollection xbrlInstances = document.XbrlInstances;
// 添加 XbrlInstance
xbrlInstances.Add();
XbrlInstance xbrlInstance = xbrlInstances[0];
// 定义单位
Unit unit = new Unit(UnitType.Measure);
// 添加限定符名称
unit.MeasureQualifiedNames.Add(new QualifiedName("USD", "iso4217", "http://www.xbrl.org/2003/iso4217"));
// 添加单位
xbrlInstance.Units.Add(unit);
// 保存输出文件
document.Save(@"D:\Files\Finance\Output.xbrl");
使用 C# 在 XBRL 中添加事实对象
XBRL 中的事实是使用项元素定义的。 XBRL 中的一个项目包含简单事实的值和对正确解释该事实的上下文的引用。我们可以按照以下步骤在 XBRL 实例文档中添加项目:
- 首先,创建一个 XbrlDocument 类的实例。
- 将新的 XBRL 实例添加到 XbrlDocument 对象的实例。
- 向 XbrlInstance 对象的架构引用添加新架构引用。
- 通过 SchemaRefCollection 中的索引获取 SchemaRef。
- 初始化 Context 实例并将其添加到 Context objects 集合中。
- 定义一个 Unit 实例并将其添加到 Unit objects 集合中。
- 使用 SchemaRef.GetConceptByName() 方法创建一个 Concept 类实例。
- 使用 Concept 对象作为参数创建 Item 类的实例。
- 设置 ContextRef、UnitRef、Precision、Value 等项目属性。
- 之后,将 Item 添加到 Fact objects 集合中。
- 最后,使用 XbrlDocument.Save() 方法保存 XBRL 文件。它将输出文件路径作为参数。
以下代码示例展示了如何使用 C# 在 XBRL 实例文档中添加事实作为项目元素。
// 此代码示例演示如何将事实项对象添加到 XBRL。
// 创建 XbrlDocument
XbrlDocument document = new XbrlDocument();
XbrlInstanceCollection xbrlInstances = document.XbrlInstances;
XbrlInstance xbrlInstance = xbrlInstances[xbrlInstances.Add()];
// 定义架构参考
SchemaRefCollection schemaRefs = xbrlInstance.SchemaRefs;
schemaRefs.Add(@"D:\Files\Finance\schema.xsd", "example", "http://example.com/xbrl/taxonomy");
SchemaRef schema = schemaRefs[0];
// 定义上下文
ContextPeriod contextPeriod = new ContextPeriod(DateTime.Parse("2020-01-01"), DateTime.Parse("2020-02-10"));
ContextEntity contextEntity = new ContextEntity("exampleIdentifierScheme", "exampleIdentifier");
Context context = new Context(contextPeriod, contextEntity);
xbrlInstance.Contexts.Add(context);
// 定义单位
Unit unit = new Unit(UnitType.Measure);
unit.MeasureQualifiedNames.Add(new QualifiedName("USD", "iso4217", "http://www.xbrl.org/2003/iso4217"));
xbrlInstance.Units.Add(unit);
// 获取概念
Concept fixedAssetsConcept = schema.GetConceptByName("fixedAssets");
if (fixedAssetsConcept != null)
{
// 为事实定义 Item 元素
Item item = new Item(fixedAssetsConcept);
item.ContextRef = context;
item.UnitRef = unit;
item.Precision = 4;
item.Value = "1444";
// 将 item 元素添加到事实
xbrlInstance.Facts.Add(item);
}
// 保存输出文件
document.Save(@"D:\Files\Finance\Output.xbrl");
获得免费许可证
您可以获得免费的临时许可证 试用该库而不受评估限制。
结论
在本文中,我们学习了如何使用 C# 创建 XBRL 文档。我们还看到了如何以编程方式将各种 XBRL 对象添加到创建的 XBRL 实例文档中。此外,您可以使用 文档 了解更多关于 Aspose.Finance for .NET API 的信息。如有任何歧义,请随时在 论坛 上与我们联系。