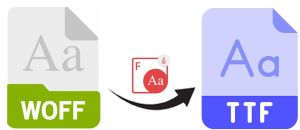
Web Open Font Format 或简称 WOFF 是一种主要用于网页的网络字体。在某些情况下,我们可能需要将 Web 字体转换为 true-type 字体。 True Type 字体或 TTF 用于所有数字平台的操作系统。在 previous post 中,我们看到了如何将 TTF 转换为 Web 字体。在本文中,我们将学习如何在 Java 中将 WOFF 转换为 TTF。
本文将涵盖以下主题:
Java WOFF 到 TTF 转换器 API
要将 WOFF 转换为 TTF,我们将使用 Aspose.Font for Java API。它允许从支持的字体类型 中加载、保存和提取信息。
API 的 FontFileDefinition 类表示字体文件定义。 FontDefinition 类允许使用字体的内部数据,例如名称、类型等。Font.open() 方法使用 FontDefinition 对象打开字体。我们可以使用 Font 类的 saveToFormat() 方法将加载的字体保存为其他格式。 FontSavingFormats 枚举中提供了保存格式。
请下载 API 的 JAR 或在基于 Maven 的 Java 应用程序中添加以下 pom.xml 配置。
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-font</artifactId>
<version>22.6</version>
</dependency>
使用 Java 将 WOFF 转换为 TTF
我们可以按照以下步骤轻松将 Web 开放字体 (WOFF) 转换为 True Type 字体 (TTF):
- 首先,使用 FileSystemStreamSource 类加载字体文件。
- 接下来,创建一个 FontFileDefinition 类的实例,文件扩展名为“woff”,源文件对象作为参数。
- 然后,初始化 Font 类型为 TTF 的 FontDefinition 类对象和 FontFileDefinition 对象。
- 之后,使用 Font.open() 方法以 FontDefinition 对象作为参数打开字体。
- 最后调用 saveToFormat() 方法保存。它以 TTF 的输出文件路径和 FontSavingFormats 作为参数。
以下代码示例演示了如何使用 Java 将 WOFF 转换为 TTF。
// 此代码示例演示如何将 WOFF 转换为 TTF。
// WOFF 文件路径
String fontPath = "C:\\Files\\font\\Montserrat-Regular.woff";
// 加载字体文件
FileSystemStreamSource source = new FileSystemStreamSource(fontPath);
// 创建字体文件定义
FontFileDefinition fileDefinition = new FontFileDefinition("woff", source);
// 创建字体定义
FontDefinition fontDefinition = new FontDefinition(FontType.TTF, fileDefinition);
// 打开字体
Font font = Font.open(fontDefinition);
// TTF 输出路径
String outPath = "C:\\Files\\font\\WoffToTtf_Out.ttf";
FileOutputStream outStream = new FileOutputStream(outPath);
// 将 WOFF 转换为 TTF
font.saveToFormat(outStream, FontSavingFormats.TTF);
使用 Java 将 WOFF2 转换为 TTF
我们也可以按照前面提到的步骤将 WOFF2 转换为 TTF。但是,我们只需在第二步中将文件扩展名设置为“woff2”。
以下代码示例演示了如何使用 Java 将 WOFF2 转换为 TTF。
// 此代码示例演示如何将 WOFF2 转换为 TTF。
// WOFF2 文件路径
String fontPath = "C:\\Files\\font\\Montserrat-Regular.woff2";
// 加载字体文件
FileSystemStreamSource source = new FileSystemStreamSource(fontPath);
// 创建字体文件定义
FontFileDefinition fileDefinition = new FontFileDefinition("woff2", source);
// 创建字体定义
FontDefinition fontDefinition = new FontDefinition(FontType.TTF, fileDefinition);
// 打开字体
Font font = Font.open(fontDefinition);
// TTF 输出路径
String outPath = "C:\\Files\\font\\Woff2ToTtf_Out.ttf";
FileOutputStream outStream = new FileOutputStream(outPath);
// 将 WOFF2 转换为 TTF
font.saveToFormat(outStream, FontSavingFormats.TTF);
获得免费许可证
您可以获得免费的临时许可证 试用该库而不受评估限制。
结论
在本文中,我们学习了如何在 Java 中将 Web Open Fonts 转换为 True Type Fonts。我们还看到了如何以编程方式将 WOFF2 保存为 TTF。此外,您可以使用 documentation 了解有关 Aspose.Font for Java API 的更多信息。如有任何歧义,请随时在我们的 论坛 上与我们联系。