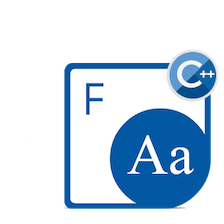
字符是解释不同单词含义的符号。您可以使用带有 Aspose.Font for C++ API 的 C++ 使用 TrueType 字体 渲染不同的文本字符。它支持不同的文本渲染功能,包括TrueType、Type1、OTF、EOT等字体。让我们通过几个简单的 API 调用来探索如何使用文本呈现功能。我们将在以下标题下讨论该主题:
C++ TrueType 字体渲染 API – 安装
考虑到 C++ 平台的普及和需求,我们在 Aspose.Font for C++ API 中引入了非常有用的功能。为了在您的应用程序中呈现 TrueType 字体,您需要通过从 New Releases 下载它来配置 API,或者您可以使用以下命令通过 NuGet 安装它:
Install-Package Aspose.Font.Cpp
使用 C++ 实现用于绘制字形以呈现 TrueType 字体的接口
首先,您需要在 Aspose.Font.Rendering 命名空间下实现 IGlyphOutlinePainter。按照以下步骤实现方法和类:
- 创建一个名为 GlyphOutlinePainter 的类
- 使用System.Drawing.Drawing2D.GraphicsPath绘制图形
下面是用于实现接口以绘制字形的 C++ 代码片段:
For complete examples and data files, please go to https://github.com/aspose-font/Aspose.Font-for-C
RenderingText::GlyphOutlinePainter::GlyphOutlinePainter(System::SharedPtr<System::Drawing::Drawing2D::GraphicsPath> path)
{
_path = path;
}
void RenderingText::GlyphOutlinePainter::MoveTo(System::SharedPtr<Aspose::Font::RenderingPath::MoveTo> moveTo)
{
_path->CloseFigure();
_currentPoint.set_X((float)moveTo->get_X());
_currentPoint.set_Y((float)moveTo->get_Y());
}
void RenderingText::GlyphOutlinePainter::LineTo(System::SharedPtr<Aspose::Font::RenderingPath::LineTo> lineTo)
{
float x = (float)lineTo->get_X();
float y = (float)lineTo->get_Y();
_path->AddLine(_currentPoint.get_X(), _currentPoint.get_Y(), x, y);
_currentPoint.set_X(x);
_currentPoint.set_Y(y);
}
void RenderingText::GlyphOutlinePainter::CurveTo(System::SharedPtr<Aspose::Font::RenderingPath::CurveTo> curveTo)
{
float x3 = (float)curveTo->get_X3();
float y3 = (float)curveTo->get_Y3();
_path->AddBezier(_currentPoint.get_X(), _currentPoint.get_Y(), (float)curveTo->get_X1(), (float)curveTo->get_Y1(), (float)curveTo->get_X2(), (float)curveTo->get_Y2(), x3, y3);
_currentPoint.set_X(x3);
_currentPoint.set_Y(y3);
}
void RenderingText::GlyphOutlinePainter::ClosePath()
{
_path->CloseFigure();
}
System::Object::shared_members_type Aspose::Font::Examples::WorkingWithTrueTypeAndOpenTypeFonts::RenderingText::GlyphOutlinePainter::GetSharedMembers()
{
auto result = System::Object::GetSharedMembers();
result.Add("Aspose::Font::Examples::WorkingWithTrueTypeAndOpenTypeFonts::RenderingText::GlyphOutlinePainter::_path", this->_path);
result.Add("Aspose::Font::Examples::WorkingWithTrueTypeAndOpenTypeFonts::RenderingText::GlyphOutlinePainter::_currentPoint", this->_currentPoint);
return result;
}
其次,请按照以下步骤创建一个名为 DrawText 的方法:
- 循环遍历文本字符串中的符号
- 获取 GID 作为每个符号标识的字形
- 创建 GlyphOutlinePainter 并传递给 GlyphOutlineRenderer 对象
- 用 Matrix 对象指定字形坐标
此外,在完成所有这些步骤之后,创建用于计算图像字体尺寸的实用方法,如以下 C++ 代码片段所述:
For complete examples and data files, please go to https://github.com/aspose-font/Aspose.Font-for-C
double RenderingText::FontWidthToImageWith(double width, int32_t fontSourceResulution, double fontSize, double dpi /* = 300*/)
{
double resolutionCorrection = dpi / 72;
// 72 是字体的内部 dpi
return (width / fontSourceResulution) * fontSize * resolutionCorrection;
}
使用 C++ 使用 TrueType 字体呈现文本
我们已经实现了使用 TrueType 字体呈现文本所需的功能。现在让我们使用 Aspose.Font for C++ API 调用方法,代码片段如下:
For complete examples and data files, please go to https://github.com/aspose-font/Aspose.Font-for-C
System::String dataDir = RunExamples::GetDataDir_Data();
System::String fileName1 = dataDir + u"Montserrat-Bold.ttf";
//带有完整路径的字体文件名
System::SharedPtr<FontDefinition> fd1 = System::MakeObject<FontDefinition>(Aspose::Font::FontType::TTF, System::MakeObject<FontFileDefinition>(u"ttf", System::MakeObject<FileSystemStreamSource>(fileName1)));
System::SharedPtr<TtfFont> ttfFont1 = System::DynamicCast_noexcept<Aspose::Font::Ttf::TtfFont>(Aspose::Font::Font::Open(fd1));
System::String fileName2 = dataDir + u"Lora-Bold.ttf";
//带有完整路径的字体文件名
System::SharedPtr<FontDefinition> fd2 = System::MakeObject<FontDefinition>(Aspose::Font::FontType::TTF, System::MakeObject<FontFileDefinition>(u"ttf", System::MakeObject<FileSystemStreamSource>(fileName2)));
System::SharedPtr<TtfFont> ttfFont2 = System::DynamicCast_noexcept<Aspose::Font::Ttf::TtfFont>(Aspose::Font::Font::Open(fd2));
DrawText(u"Hello world", ttfFont1, 14, System::Drawing::Brushes::get_White(), System::Drawing::Brushes::get_Black(), dataDir + u"hello1_montserrat_out.jpg");
DrawText(u"Hello world", ttfFont2, 14, System::Drawing::Brushes::get_Yellow(), System::Drawing::Brushes::get_Red(), dataDir + u"hello2_lora_out.jpg");
结论
在本文中,您学习了如何在 C++ 中使用 TrueType 字体呈现文本。有兴趣了解更多关于 Aspose.Font 为 C++ API 提供的功能吗?您可以通过浏览 API 参考 或 产品文档 了解更多详细信息。但是,如果您遇到任何歧义或需要帮助,请随时通过 免费支持论坛 与我们联系。我们期待与您合作!