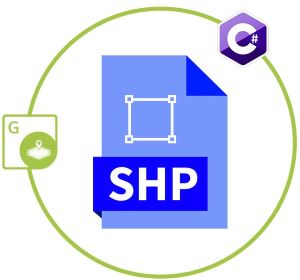
Shapefile 格式是一种地理空间矢量数据格式,用于显示地理信息。我们可以在 Shapefile 中存储点、线和多边形特征的位置、几何形状和属性。在本文中,我们将学习如何在 C# 中创建和读取 Shapefile。
本文将涵盖以下主题:
- 什么是形状文件
- 如何使用形状文件
- 创建和读取 Shapefile 的 C# API – .NET GIS 库
- 使用 C# 创建 Shapefile
- 在 C# 中向现有 ShapeFile 添加功能
- 使用 C# 读取 Shapefile
什么是形状文件
shapefile 以矢量数据的形式表示地理空间信息,供 GIS 应用程序使用。它是由 ESRI 作为开放规范开发的,以促进 ESRI 和其他软件产品之间的互操作性。它包含在地图上绘制点、线或多边形的几何数据和数据属性。 Shapefiles 可以被 ArcGIS 和 QGIS 等几种 GIS 软件程序直接读取。
如何使用形状文件
软件应用程序不能使用独立的 shapefile (.shp)。但是,可在 GIS 软件中使用的有效 shapefile 应包含以下附加必需文件:
- 形状索引文件 (.shx) - a positional index of the feature geometry;
- dBase 属性文件 (.dbf) - a dBASE file that stores all the attributes of the shapes;
- 代码页文件 (.cpg) - 用于识别字符编码的文件。
用于创建和读取 Shapefile 的 C# API – .NET GIS 库
要创建或读取 shapefile,我们将使用 Aspose.GIS for .NET API。它允许在没有额外软件的情况下渲染地图、创建、读取和转换地理数据。它支持使用 shapefile 以及其他几种 支持的文件格式。
API 的 VectorLayer 类表示一个矢量图层。它提供了各种属性和方法来处理存储在文件中的地理特征集合。此类的 Create() 方法允许创建支持的矢量图层。 Drivers 类为所有支持的格式提供驱动程序。 API 的 Feature 类表示由几何和用户定义的属性组成的地理特征。
PM> Install-Package Aspose.GIS
使用 C# 创建形状文件
我们可以按照以下步骤轻松地以编程方式创建 shapefile:
- 首先,使用 VectorLayer.Create() 方法创建一个图层。
- 接下来,将 FeatureAttributes 添加到图层的属性集合中。
- 然后,使用 ConstructFeature() 方法创建要素类的实例。
- 之后,设置不同属性的值。
- 最后,使用 Add() 方法添加功能。
以下代码示例展示了如何在 C# 中创建 shapefile:
// 此代码示例演示如何创建新的 shapefile。
// 创建一个新的形状文件
using (VectorLayer layer = VectorLayer.Create(@"C:\Files\GIS\NewShapeFile_out.shp", Drivers.Shapefile))
{
// 在添加功能之前添加属性
layer.Attributes.Add(new FeatureAttribute("name", AttributeDataType.String));
layer.Attributes.Add(new FeatureAttribute("age", AttributeDataType.Integer));
layer.Attributes.Add(new FeatureAttribute("dob", AttributeDataType.DateTime));
// 添加功能并设置值
Feature firstFeature = layer.ConstructFeature();
firstFeature.Geometry = new Point(33.97, -118.25);
firstFeature.SetValue("name", "John");
firstFeature.SetValue("age", 23);
firstFeature.SetValue("dob", new DateTime(1982, 2, 5, 16, 30, 0));
layer.Add(firstFeature);
// 添加另一个功能并设置值
Feature secondFeature = layer.ConstructFeature();
secondFeature.Geometry = new Point(35.81, -96.28);
secondFeature.SetValue("name", "Mary");
secondFeature.SetValue("age", 54);
secondFeature.SetValue("dob", new DateTime(1984, 12, 15, 15, 30, 0));
layer.Add(secondFeature);
}
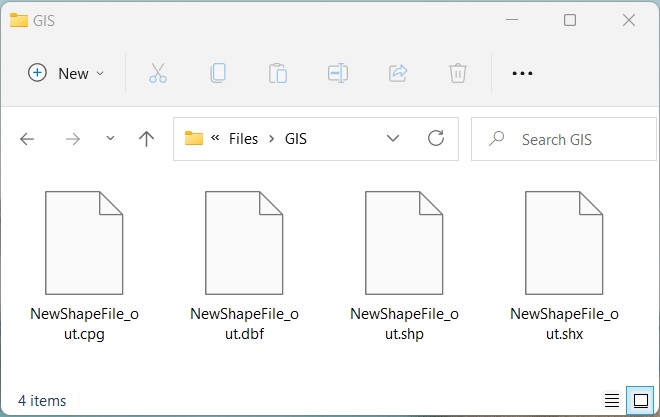
使用 C# 创建 Shapefile。
使用 C# 向现有 ShapeFile 添加功能
我们还可以按照以下步骤向现有 shapefile 添加新功能:
- 首先,使用 Drivers.Shapefile.EditLayer() 方法加载 shapefile。
- 接下来,使用 ConstructFeature() 方法创建要素类的实例。
- 之后,设置不同属性的值。
- 最后,使用 Add() 方法添加功能。
以下代码示例展示了如何使用 C# 向现有 shapefile 添加功能。
// 此代码示例演示如何编辑 shapefile。
// 文件路径
string path = Path.Combine(dataDir, "NewShapeFile_out.shp");
// 编辑 Shapefile 图层
using (var layer = Drivers.Shapefile.EditLayer(path))
{
// 添加功能
var feature = layer.ConstructFeature();
feature.Geometry = new Point(34.81, -92.28);
object[] data = new object[3] { "Alex", 25, new DateTime(1989, 4, 15, 15, 30, 0) };
feature.SetValues(data);
layer.Add(feature);
}
使用 C# 读取 Shapefile
我们可以按照以下步骤从 shapefile 中读取属性:
- 首先,使用 Drivers.Shapefile.OpenLayer() 方法加载 shapefile。
- 循环遍历层中的每个要素。
- 循环遍历属性并显示属性详细信息。
- 最后,检查点几何和读取点。
以下代码示例展示了如何使用 C# 读取 shapefile。
// 此代码示例演示如何读取新的 shapefile。
// 文件路径
string path = Path.Combine(dataDir, "NewShapeFile_out.shp");
// 打开一个图层
var layer = Drivers.Shapefile.OpenLayer(path);
foreach (Feature feature in layer)
{
foreach (var attribute in layer.Attributes)
{
// 显示属性详细信息
Console.WriteLine(attribute.Name + " : " + feature.GetValue(attribute.Name));
}
// 检查点几何
if (feature.Geometry.GeometryType == GeometryType.Point)
{
// 读点
Point point = (Point)feature.Geometry;
Console.WriteLine(point.AsText() + " X: " + point.X + " Y: " + point.Y);
Console.WriteLine("---------------------");
}
}
name : John
age : 23
dob : 1982-02-05T16:30:00
POINT (33.97 -118.25) X: 33.97 Y: -118.25
---------------------
name : Mary
age : 54
dob : 1984-12-15T15:30:00
POINT (35.81 -96.28) X: 35.81 Y: -96.28
---------------------
name : Alex
age : 25
dob : 04/15/1989 15:30:00
POINT (34.81 -92.28) X: 34.81 Y: -92.28
获得免费许可证
您可以 获得免费的临时许可证 试用该库而不受评估限制。
结论
在本文中,我们学习了如何
- 以编程方式创建一个新的 shapefile;
- 向 shapefile 添加新功能;
- 编辑 shapefile 图层;
- 打开 shapefile 层并使用 C# 读取属性。
此外,您可以探索如何使用其他几种 GIS 文件格式,并使用 文档 了解更多关于 Aspose.GIS for .NET API 的信息。如有任何歧义,请随时在我们的 论坛 上与我们联系。