
有一系列以编程方式执行图像编辑和操作的场景。在图像编辑中,图像的合并是一个重要的功能,用于组合两个或多个图像,例如制作拼贴。在本文中,您将学习如何在 Java 中将多个图像合并为单个图像。我们将明确演示如何水平和垂直合并图像。
用于合并图像的 Java API - 免费下载
Aspose.Imaging for Java 是一个强大的图像处理 API,可让您处理各种图像格式。它提供了图像编辑所需的各种功能。我们将使用这个 API 来合并我们在这篇博文中的图像。您可以 下载 API 或使用以下 Maven 配置安装它。
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-imaging</artifactId>
<version>22.7</version>
</dependency>
在 Java 中合并多个图像
您可以通过以下两种方式之一合并图像:垂直和水平。在垂直合并中,图像在垂直方向上一张一张地合并。而在水平合并中,图像在水平方向上相互附加。那么让我们看看如何以两种方式合并图像。
在 Java 中水平合并图像
以下是使用 Java 水平合并图像的步骤。
- 首先,在字符串数组中指定图像的路径。
- 计算结果图像的高度和宽度。
- 创建一个 JpegOptions 类的对象并设置所需的选项。
- 创建一个 JpegImage 类的对象,并使用 JpegOptions 对象和结果图像的高度和宽度对其进行初始化。
- 遍历图像列表并使用 RasterImage 类加载每个图像。
- 为每个图像创建一个 Rectangle 并使用 JpegImage.saveArgb32Pixels() 方法将其添加到结果图像中。
- 在每次迭代中增加缝合宽度。
- 完成后,使用 JpegImage.save(string) 方法保存生成的图像。
以下代码示例展示了如何在 Java 中水平合并图像。
// 图像列表
String[] imagePaths = { "image.jpg", "image.jpg" };
// 输出图片路径
String outputPath = "output-horizontal.jpg";
String tempFilePath = "temp.jpg";
// 获取结果图像大小
int newWidth = 0;
int newHeight = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (com.aspose.imaging.RasterImage) com.aspose.imaging.Image.load(imagePath)) {
Size size = image.getSize();
newWidth += size.getWidth();
newHeight = Math.max(newHeight, size.getHeight());
}
}
// 将图像合并为新图像
try (JpegOptions options = new JpegOptions()) {
Source tempFileSource = new FileCreateSource(tempFilePath, true);
options.setSource(tempFileSource);
options.setQuality(100);
// 创建结果图像
try (JpegImage newImage = (JpegImage) Image.create(options, newWidth, newHeight)) {
int stitchedWidth = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (RasterImage) Image.load(imagePath)) {
Rectangle bounds = new Rectangle(stitchedWidth, 0, image.getWidth(), image.getHeight());
newImage.saveArgb32Pixels(bounds, image.loadArgb32Pixels(image.getBounds()));
stitchedWidth += image.getWidth();
}
}
// 保存输出图像
newImage.save(outputPath);
}
}
下图显示了水平合并两个相似图像的输出。
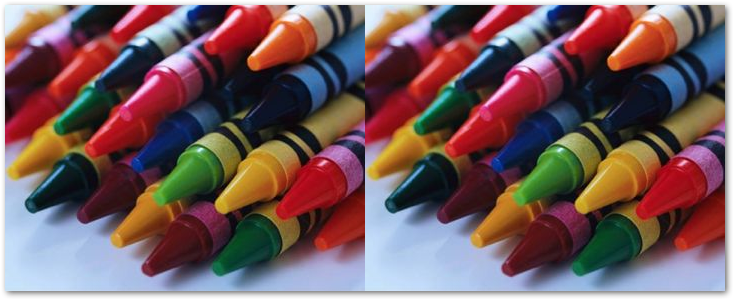
在 Java 中垂直合并图像
要垂直合并图像,只需要切换 height 和 width 属性的角色。其余代码将是相同的。以下代码示例演示了如何在 Java 中垂直合并多个图像。
// 图像列表
String[] imagePaths = { "image.jpg", "image.jpg" };
// 输出图像的路径
String outputPath = "output-vertical.jpg";
// 获取结果图像大小
int newWidth = 0;
int newHeight = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (RasterImage) Image.load(imagePath)) {
Size size = image.getSize();
newWidth = Math.max(newWidth, size.getWidth());
newHeight += size.getHeight();
}
}
// 将图像合并为新图像
try (JpegOptions options = new JpegOptions()) {
options.setSource(new StreamSource()); // empty
options.setQuality(100);
// 创建结果图像
try (JpegImage newImage = (JpegImage) Image.create(options, newWidth, newHeight)) {
int stitchedHeight = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (RasterImage) Image.load(imagePath)) {
Rectangle bounds = new Rectangle(0, stitchedHeight, image.getWidth(), image.getHeight());
newImage.saveArgb32Pixels(bounds, image.loadArgb32Pixels(image.getBounds()));
stitchedHeight += image.getHeight();
}
}
// 保存结果图像
newImage.save(outputPath);
}
}
下图显示了垂直合并两个相似图像的输出。
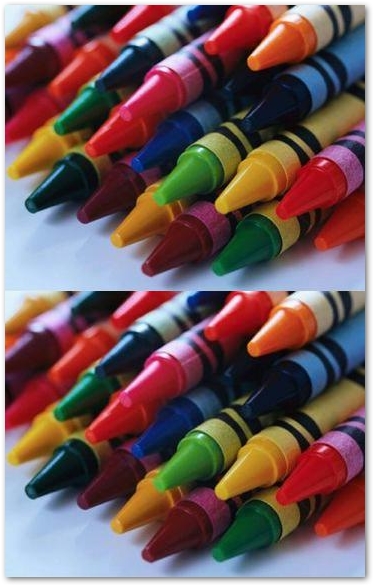
在 Java 中合并 PNG 图像
在前面的部分中,我们已经演示了如何合并 JPG 格式的图像。但是,您可能还需要合并 PNG 格式的图像。要合并 PNG 图像,只需将 JpegImage 和 JpegOptions 类分别替换为 PngImage 和 PngOptions 类,其余代码将保持不变。
以下代码示例展示了如何在 Java 中合并多个 PNG 格式的图像。
// 图像列表
String[] imagePaths = { "image.png", "image.png" };
// 输出图片路径
String outputPath = "output-horizontal.png";
String tempFilePath = "temp.png";
// 获取结果图像大小
int newWidth = 0;
int newHeight = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (com.aspose.imaging.RasterImage) com.aspose.imaging.Image.load(imagePath)) {
Size size = image.getSize();
newWidth += size.getWidth();
newHeight = Math.max(newHeight, size.getHeight());
}
}
// 将图像合并为新图像
try (PngOptions options = new PngOptions()) {
Source tempFileSource = new FileCreateSource(tempFilePath, true);
options.setSource(tempFileSource);
// 创建结果图像
try (PngImage newImage = (PngImage) Image.create(options, newWidth, newHeight)) {
int stitchedWidth = 0;
for (String imagePath : imagePaths) {
try (RasterImage image = (RasterImage) Image.load(imagePath)) {
Rectangle bounds = new Rectangle(stitchedWidth, 0, image.getWidth(), image.getHeight());
newImage.saveArgb32Pixels(bounds, image.loadArgb32Pixels(image.getBounds()));
stitchedWidth += image.getWidth();
}
}
// 保存图片
newImage.save(outputPath);
}
}
Java 图像合并 API - 获得免费许可证
您可以 获得免费的临时许可证 并合并图像而不受评估限制。
结论
在本文中,您学习了如何使用 Java 将多个图像合并为单个图像。 Java 代码示例演示了如何垂直和水平组合图像。此外,您可以使用 文档 探索有关 Java 图像处理 API 的更多信息。此外,您可以通过我们的 论坛 与我们分享您的疑问。
也可以看看
信息:Aspose 提供了一个 免费拼贴网络应用程序。使用此在线服务,您可以合并 JPG 到 JPG 或 PNG 到 PNG 图像,创建 照片网格 等等。