
图像编辑广泛用于提高图像质量。随着移动相机和图像编辑应用程序的出现,每个移动用户都知道如何编辑图像。在其他图像编辑功能中,创建拼贴是一种流行的功能,其中将多个图像组合成单个图像。因此,在本文中,我们将演示如何在 Python 中合并多个图像。如果您正在 Python 应用程序中处理图像编辑,这可能对您很有用。
用于合并图像的 Python 库
要将多个图像合并为单个图像,我们将使用 Aspose.Imaging for Python。它是一个功能丰富的图像处理库,可以轻松执行多种图像编辑操作。您可以下载该库或使用以下命令安装它。
> pip install aspose-imaging-python-net
在 Python 中合并多个图像
合并图像有两种方式:垂直合并和水平合并。您可以选择合适的方法。让我们在下面的部分中看看如何以两种方式组合图像。
在Python中水平合并图像
以下是使用 Aspose.Imaging for Python 水平合并图像的步骤。
- 首先指定数组中图像的路径。
- 然后,计算所得图像的高度和宽度。
- 创建 JpegOptions 类的对象并设置所需的选项。
- 创建 JpegImage 类的对象,并使用 JpegOptions 对象以及生成图像的高度和宽度对其进行初始化。
- 循环遍历图像列表并使用 RasterImage 类加载每个图像。
- 为每个图像创建一个矩形,并使用 JpegImage.saveargb32pixels() 方法将其添加到结果图像中。
- 增加每次迭代中的缝合宽度。
- 最后,使用 JpegImage.save(string) 方法保存生成的图像。
下面是Python中水平合并图像的代码。
import aspose.pycore as aspycore
from aspose.imaging import Image, Rectangle, RasterImage
from aspose.imaging.imageoptions import JpegOptions
from aspose.imaging.sources import FileCreateSource
from aspose.imaging.fileformats.jpeg import JpegImage
import os
# 定义文件夹
if 'TEMPLATE_DIR' in os.environ:
templates_folder = os.environ['TEMPLATE_DIR']
else:
templates_folder = r"C:\Users\USER\Downloads\templates"
delete_output = 'SAVE_OUTPUT' not in os.environ
data_dir = templates_folder
image_paths = [os.path.join(data_dir, "template.jpg"),
os.path.join(data_dir, "template.jpeg")]
output_path = os.path.join(data_dir, "result.jpg")
temp_file_path = os.path.join(data_dir, "temp.jpg")
# 获取结果图像大小
image_sizes = []
for image_path in image_paths:
with Image.load(image_path) as image:
image_sizes.append(image.size)
# 计算新尺寸
new_width = 0
new_height = 0
for size in image_sizes:
new_width += size.width
new_height = max(new_height, size.height)
# 将图像合并为新图像
temp_file_source = FileCreateSource(temp_file_path, delete_output)
with JpegOptions() as options:
options.source = temp_file_source
options.quality = 100
with aspycore.as_of(Image.create(options, new_width, new_height), JpegImage) as new_image:
stitched_width = 0
for image_path in image_paths:
with aspycore.as_of(Image.load(image_path), RasterImage) as image:
bounds = Rectangle(stitched_width, 0, image.width, image.height)
new_image.save_argb_32_pixels(bounds, image.load_argb_32_pixels(image.bounds))
stitched_width += image.width
new_image.save(output_path)
# 删除临时文件
if delete_output:
os.remove(output_path)
if os.path.exists(temp_file_path):
os.remove(temp_file_path)
下面是我们水平合并图像后得到的输出图像。

在 Python 中垂直组合图像
现在让我们看看如何垂直合并多个图像。垂直合并图像的步骤与上一节中的相同。唯一的区别是,我们会交换高度和宽度属性的角色。
以下代码示例展示了如何在 Python 中合并垂直布局中的图像。
import aspose.pycore as aspycore
from aspose.imaging import Image, Rectangle, RasterImage
from aspose.imaging.imageoptions import JpegOptions
from aspose.imaging.sources import StreamSource
from aspose.imaging.fileformats.jpeg import JpegImage
from aspose.imaging.extensions import StreamExtensions
import os
import functools
# 定义文件夹
if 'TEMPLATE_DIR' in os.environ:
templates_folder = os.environ['TEMPLATE_DIR']
else:
templates_folder = r"C:\Users\USER\Downloads\templates"
delete_output = 'SAVE_OUTPUT' not in os.environ
data_dir = templates_folder
image_paths = [os.path.join(data_dir, "template.jpg"), os.path.join(data_dir, "template.jpeg")]
output_path = os.path.join(data_dir, "result.jpg")
temp_file_path = os.path.join(data_dir, "temp.jpg")
# 获取结果图像大小
image_sizes = []
for image_path in image_paths:
with Image.load(image_path) as image:
image_sizes.append(image.size)
# 计算新尺寸
new_width = 0
new_height = 0
for size in image_sizes:
new_height += size.height
new_width = max(new_width, size.width)
# 将图像合并为新图像
with StreamExtensions.create_memory_stream() as memory_stream:
output_stream_source = StreamSource(memory_stream)
with JpegOptions() as options:
options.source = output_stream_source
options.quality = 100
with aspycore.as_of(Image.create(options, new_width, new_height), JpegImage) as new_image:
stitched_height = 0
for image_path in image_paths:
with aspycore.as_of(Image.load(image_path), RasterImage) as image:
bounds = Rectangle(0, stitched_height, image.width, image.height)
new_image.save_argb_32_pixels(bounds, image.load_argb_32_pixels(image.bounds))
stitched_height += image.height
new_image.save(output_path)
# 删除临时文件
if delete_output:
os.remove(output_path)
下图显示了垂直合并两个相似图像的输出。

在 Python 中合并 PNG 图像
前面几节中提供的步骤和代码示例用于合并 JPG 图像,但是,您可能还需要合并 PNG 图像。不用担心。相同的步骤和代码示例对于 PNG 图像有效,唯一的更改是分别使用 PngImage 和 PngOptions 类而不是 JpegImage 和 JpegOptions。
获取免费的 Python 图像合并库
您可以获取免费的临时许可证并合并图像,而不受评估限制。
在线合并图像
您还可以使用我们的免费图像合并工具在线合并您的图像。该工具基于 Aspose.Imaging for Python,您无需为其创建帐户。
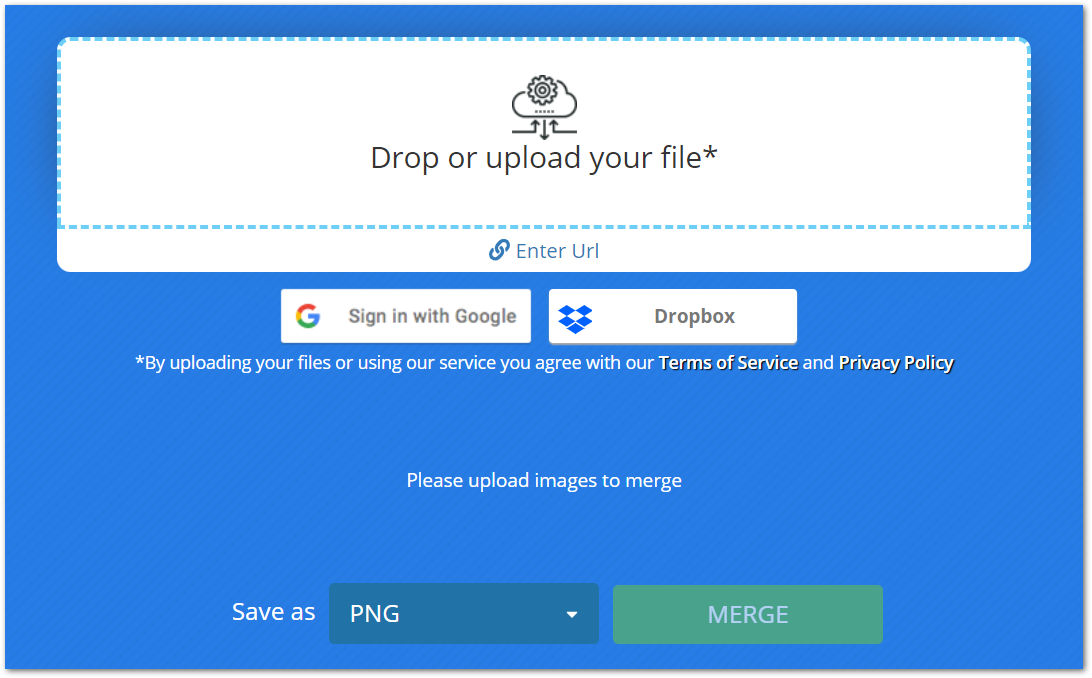
结论
本文为您提供了在 Python 中将多个图像合并为单个图像的最佳和最简单的解决方案之一。借助代码示例演示了水平和垂直图像合并。此外,我们还向您介绍了一个免费合并图像的在线工具。
您可以通过访问文档探索有关Python图像处理库的更多信息。此外,您还可以通过我们的论坛与我们分享您的疑问。